Mastering Full Stack Development: Guide to Acing MERN Stack, React Native and AWS Interviews
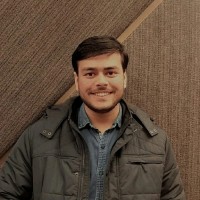
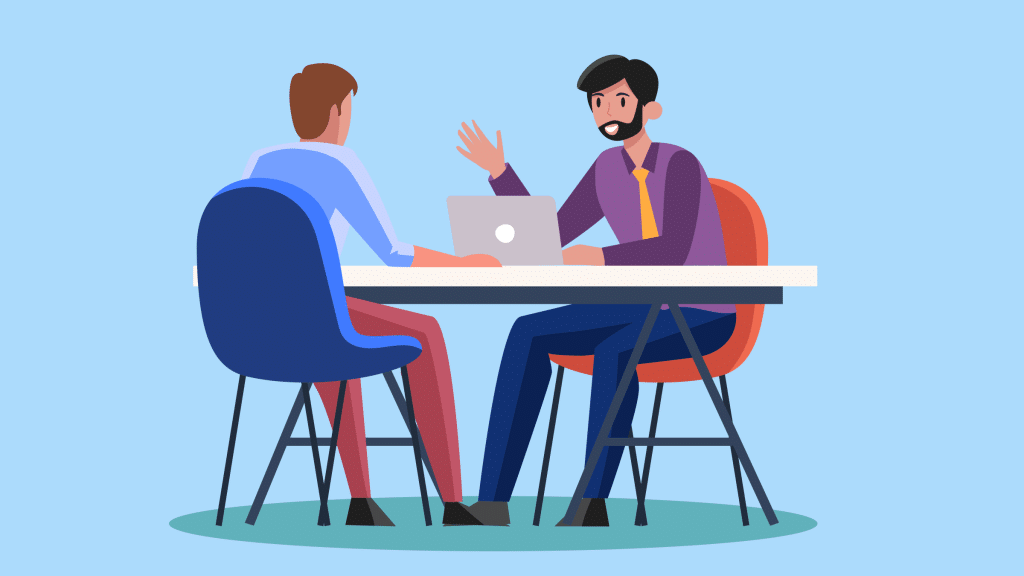
In the rapidly evolving world of software development, the role of a Full Stack Developer has become more critical than ever. Imagine yourself at the forefront of technology, seamlessly integrating both the front-end and back-end to create powerful, efficient, and user-friendly applications. This is the essence of a Full Stack Developer, and mastering this role requires a deep understanding of the MERN stack—MongoDB, Express.js, React, and Node.js—along with the versatility of React Native for mobile development.
Visualize yourself as a key player in a dynamic team, where every day brings new challenges and opportunities to innovate. You're not just writing code; you're building solutions that enhance user experiences and drive business success. From designing intuitive user interfaces with React to implementing robust server-side logic with Node.js, your role encompasses a broad spectrum of technologies. Managing databases with MongoDB, creating APIs with Express.js, and developing cross-platform mobile applications with React Native are all part of your daily routine. You’re not just developing software; you’re crafting the future of digital experiences.
But it’s not just about the technical skills. Being a Full Stack Developer means working in a collaborative and supportive environment. You’ll engage with cross-functional teams, gather requirements, and implement solutions that meet business objectives. The satisfaction of seeing your work make a real impact is unparalleled.
As you prepare for this exciting journey, it’s crucial to be well-equipped with knowledge and expertise in key areas. In the following sections, we will delve into essential questions and answers that cover topics like Node.js, React, React Native, MongoDB, and AWS. Whether you’re preparing for an interview or looking to enhance your skills, this comprehensive guide will provide you with valuable insights and practical examples to help you excel.
Node.js Questions and Answers
What is Node.js, and how does it work?
- Node.js is an open-source, cross-platform JavaScript runtime environment that executes JavaScript code outside a web browser. It uses the V8 JavaScript engine developed by Google.
Explain the event-driven architecture of Node.js.
- Node.js operates on a single-threaded event loop. It uses non-blocking I/O operations, allowing it to handle thousands of concurrent connections without being blocked.
How does the Node.js event loop work?
- The event loop is the heart of Node.js. It processes events and executes callbacks. It has phases like timers, pending callbacks, idle, prepare, poll, check, and close callbacks.
What are the main differences between Node.js and traditional server-side technologies?
- Node.js is asynchronous and non-blocking, while traditional server-side technologies are often synchronous and blocking. Node.js uses a single-threaded event loop, whereas traditional servers use multiple threads.
How do you handle asynchronous operations in Node.js?
- Asynchronous operations in Node.js can be handled using callbacks, Promises, or async/await.
// Using async/await
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error(error);
}
}
fetchData();
Explain the concept of callbacks in Node.js.
- A callback is a function passed as an argument to another function, which is then invoked inside the outer function to complete some kind of routine or action.
function fetchData(callback) {
setTimeout(() => {
callback(null, 'Data retrieved');
}, 1000);
}
fetchData((err, data) => {
if (err) {
console.error(err);
} else {
console.log(data);
}
});
What are Promises and async/await in Node.js?
- Promises represent a value that may be available now, or in the future, or never. async/await is syntax sugar built on top of Promises to handle asynchronous operations in a more synchronous manner.
// Using Promises
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
// Using async/await
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error(error);
}
}
fetchData();
Describe the use of streams in Node.js.
- Streams are objects that let you read data from a source or write data to a destination in a continuous fashion. They are instances of EventEmitter.
const fs = require('fs');
const stream = fs.createReadStream('path/to/file.txt');
stream.on('data', chunk => {
console.log(`Received ${chunk.length} bytes of data.`);
});
stream.on('end', () => {
console.log('No more data.');
});
How do you handle errors in Node.js?
- Errors in Node.js can be handled using try/catch blocks, event listeners, or callback functions.
// Using try/catch
try {
const data = fs.readFileSync('/path/to/file');
console.log(data.toString());
} catch (err) {
console.error(err);
}
// Using callback
fs.readFile('/path/to/file', (err, data) => {
if (err) {
console.error(err);
} else {
console.log(data.toString());
}
});
What is middleware in Express.js, and how is it used?
- Middleware functions are functions that have access to the request object (req), the response object (res), and the next middleware function in the application’s request-response cycle.
const express = require('express');
const app = express();
const myMiddleware = (req, res, next) => {
console.log('Middleware executed');
next();
};
app.use(myMiddleware);
app.get('/', (req, res) => {
res.send('Hello World');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Explain the purpose of the package.json file.
- The package.json file is a manifest file that contains metadata about the project, such as the name, version, dependencies, and scripts.
How do you manage dependencies in Node.js projects?
- Dependencies are managed using npm or yarn. You can install dependencies using
npm install <package-name>
and save them in the package.json file.
- Dependencies are managed using npm or yarn. You can install dependencies using
What is npm, and how do you use it?
- npm (Node Package Manager) is the default package manager for Node.js. It is used to install, update, and manage packages and dependencies.
What are some common Node.js modules, and how do you use them?
- Common Node.js modules include fs (file system), http (HTTP server), path (file and directory paths), and events (EventEmitter).
const fs = require('fs');
const data = fs.readFileSync('/path/to/file');
console.log(data.toString());
How do you create a RESTful API using Node.js and Express?
- A RESTful API can be created using Express by defining routes and handling HTTP methods like GET, POST, PUT, and DELETE.
const express = require('express');
const app = express();
app.use(express.json());
app.get('/api/items', (req, res) => {
res.send('GET request to the homepage');
});
app.post('/api/items', (req, res) => {
res.send('POST request to the homepage');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
What is the role of the cluster module in Node.js?
- The cluster module allows you to create child processes (workers) that share the same server port, making it possible to take advantage of multi-core systems.
How do you handle file uploads in Node.js?
- File uploads can be handled using middleware like multer.
const express = require('express');
const multer = require('multer');
const app = express();
const upload = multer({ dest: 'uploads/' });
app.post('/upload', upload.single('file'), (req, res) => {
res.send('File uploaded successfully');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Explain how to secure a Node.js application.
- Security practices include using HTTPS, validating user input, sanitizing data, using environment variables for configuration, and keeping dependencies up to date.
How do you handle authentication and authorization in Node.js?
- Authentication and authorization can be handled using libraries like Passport.js or JSON Web Tokens (JWT).
const jwt = require('jsonwebtoken');
const express = require('express');
const app = express();
app.post('/login', (req, res) => {
const token = jwt.sign({ id: user.id }, 'secret', { expiresIn: '1h' });
res.json({ token });
});
const authenticate = (req, res, next) => {
const token = req.headers['authorization'];
if (token) {
jwt.verify(token, 'secret', (err, decoded) => {
if (err) {
return res.status(403).send('Token is not valid');
} else {
req.user = decoded;
next();
}
});
} else {
return res.status(403).send('No token provided');
}
};
app.get('/protected', authenticate, (req, res) => {
res.send('This is a protected route');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
What are some best practices for deploying a Node.js application?
- Best practices include using a process manager like PM2, setting up environment variables, monitoring the application, ensuring proper error handling, and automating the deployment process with CI/CD tools.
React Questions and Answers
What is React, and why is it used for front-end development?
- React is a JavaScript library for building user interfaces. It allows developers to create reusable UI components and manage the state efficiently.
Explain the concept of the virtual DOM in React.
- The virtual DOM is a lightweight representation of the real DOM. React updates the virtual DOM and then efficiently updates the real DOM by computing the minimal set of changes needed.
How does React Js component-based architecture work?
- React applications are built using components, which are reusable pieces
of the UI. Components can be class-based or functional and can have their own state and lifecycle methods.
What are React hooks, and how do you use them?
- React hooks are functions that let you use state and lifecycle features in functional components. Common hooks include useState, useEffect, and useContext.
import React, { useState, useEffect } from 'react';
function Example() {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `You clicked ${count} times`;
}, [count]);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
Explain the use of useState and useEffect hooks.
- useState is used to declare state variables in functional components, while useEffect is used to perform side effects like data fetching or updating the DOM.
How do you manage state in a React application?
- State can be managed using useState, useReducer, Context API, or state management libraries like Redux.
What is the context API in React?
- The context API allows you to pass data through the component tree without having to pass props down manually at every level.
const ThemeContext = React.createContext('light');
function App() {
return (
<ThemeContext.Provider value="dark">
<Toolbar />
</ThemeContext.Provider>
);
}
function Toolbar() {
return (
<div>
<ThemedButton />
</div>
);
}
function ThemedButton() {
const theme = useContext(ThemeContext);
return <button style={{ background: theme }}>Theme</button>;
}
How do you handle forms in React?
- Forms in React can be handled using controlled or uncontrolled components. Controlled components have their state managed by React, while uncontrolled components manage their own state.
function MyForm() {
const [value, setValue] = useState('');
const handleChange = (event) => {
setValue(event.target.value);
};
const handleSubmit = (event) => {
event.preventDefault();
alert('A name was submitted: ' + value);
};
return (
<form onSubmit={handleSubmit}>
<label>
Name:
<input type="text" value={value} onChange={handleChange} />
</label>
<input type="submit" value="Submit" />
</form>
);
}
Explain the difference between controlled and uncontrolled components.
- Controlled components have their state managed by React, while uncontrolled components use the DOM to manage their state.
What are higher-order components (HOCs) in React?
- HOCs are functions that take a component and return a new component, allowing you to reuse component logic.
function withLogging(WrappedComponent) {
return function(props) {
useEffect(() => {
console.log('Component mounted');
}, []);
return <WrappedComponent {...props} />;
};
}
const EnhancedComponent = withLogging(MyComponent);
How do you optimize the performance of a React application?
- Performance optimization techniques include using React.memo, useMemo, useCallback, code splitting, and lazy loading.
const MemoizedComponent = React.memo(MyComponent);
const memoizedValue = useMemo(() => computeExpensiveValue(a, b), [a, b]);
const memoizedCallback = useCallback(() => {
doSomething(a, b);
}, [a, b]);
What are React fragments, and how do you use them?
- React fragments allow you to group multiple elements without adding extra nodes to the DOM.
return (
<React.Fragment>
<h1>Title</h1>
<p>Paragraph</p>
</React.Fragment>
);
How do you handle routing in a React application?
- Routing in React can be handled using libraries like React Router.
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
function App() {
return (
<Router>
<Switch>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
</Switch>
</Router>
);
}
Explain the concept of lazy loading in React.
- Lazy loading allows you to load components only when they are needed, reducing the initial load time.
import React, { Suspense } from 'react';
const LazyComponent = React.lazy(() => import('./LazyComponent'));
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
);
}
How do you test React components?
- React components can be tested using testing libraries like Jest and React Testing Library.
import { render, screen } from '@testing-library/react';
import App from './App';
test('renders learn react link', () => {
render(<App />);
const linkElement = screen.getByText(/learn react/i);
expect(linkElement).toBeInTheDocument();
});
What is Redux, and how does it integrate with React?
- Redux is a state management library for JavaScript applications. It integrates with React using the
react-redux
library.
- Redux is a state management library for JavaScript applications. It integrates with React using the
import { createStore } from 'redux';
import { Provider } from 'react-redux';
import rootReducer from './reducers';
import App from './App';
const store = createStore(rootReducer);
function Root() {
return (
<Provider store={store}>
<App />
</Provider>
);
}
Explain the concept of props drilling and how to avoid it.
- Props drilling refers to passing props through multiple levels of components. It can be avoided using the Context API or state management libraries like Redux.
How do you handle side effects in React applications?
- Side effects can be handled using useEffect or middleware like Redux Thunk or Redux Saga.
useEffect(() => {
fetchData();
}, []);
What are React portals, and when should you use them?
- React portals allow you to render a component's children into a DOM node outside of the parent component.
import ReactDOM from 'react-dom';
function PortalComponent() {
return ReactDOM.createPortal(
<div>Portal Content</div>,
document.getElementById('portal-root')
);
}
Explain the difference between class components and functional components in React.
- Class components are ES6 classes that extend
React.Component
, while functional components are plain JavaScript functions that return JSX.
- Class components are ES6 classes that extend
React Native Questions and Answers
What is React Native, and how does it differ from React?
- React Native is a framework for building native mobile applications using React. Unlike React, which is for web applications, React Native uses native components for rendering.
How do you set up a React Native project?
- You can set up a React Native project using the React Native CLI or Expo CLI.
npx react-native init MyApp
# or
npx expo-cli init MyApp
Explain the use of Flexbox for layout in React Native.
- Flexbox is used for layout in React Native, allowing you to design responsive and flexible layouts.
const styles = StyleSheet.create({
container: {
flex: 1,
flexDirection: 'column',
justifyContent: 'center',
alignItems: 'center',
},
});
How do you handle navigation in a React Native app?
- Navigation in React Native can be handled using libraries like React Navigation.
import { NavigationContainer } from '@react-navigation/native';
import { createStackNavigator } from '@react-navigation/stack';
const Stack = createStackNavigator();
function App() {
return (
<NavigationContainer>
<Stack.Navigator>
<Stack.Screen name="Home" component={HomeScreen} />
<Stack.Screen name="Details" component={DetailsScreen} />
</Stack.Navigator>
</NavigationContainer>
);
}
What are some common performance issues in React Native, and how do you address them?
- Common performance issues include slow rendering, memory leaks, and large bundle sizes. They can be addressed by optimizing images, using FlatList, and minimizing re-renders.
How do you manage state in a React Native application?
- State can be managed using useState, useReducer, Context API, or state management libraries like Redux.
What are the differences between React Native and traditional native development?
- React Native uses JavaScript and React to build applications, while traditional native development uses languages like Swift/Objective-C for iOS and Java/Kotlin for Android.
How do you handle platform-specific code in React Native?
- Platform-specific code can be handled using the
Platform
module or platform-specific file extensions.
- Platform-specific code can be handled using the
javascript import { Platform } from 'react-native';
const styles = StyleSheet.create({ container: { paddingTop: Platform.OS === 'ios' ? 20 : 0, }, });
What are some common libraries used in React Native development?
- Common libraries include React Navigation, Redux, Axios, and Native Base.
How do you debug a React Native application?
- React Native applications can be debugged using tools like React Native Debugger, Flipper, or the built-in debugging tools in Chrome.
Explain the use of async storage in React Native.
- Async Storage is a simple, unencrypted, asynchronous, persistent, key-value storage system that is global to the app.
import AsyncStorage from '@react-native-async-storage/async-storage';
const storeData = async (value) => {
try {
await AsyncStorage.setItem('@storage_Key', value);
} catch (e) {
// saving error
}
};
const getData = async () => {
try {
const value = await AsyncStorage.getItem('@storage_Key');
if (value !== null) {
// value previously stored
}
} catch (e) {
// error reading value
}
};
How do you handle animations in React Native?
- Animations can be handled using the Animated API, Reanimated library, or Lottie.
import { Animated } from 'react-native';
const fadeAnim = useRef(new Animated.Value(0)).current;
useEffect(() => {
Animated.timing(fadeAnim, {
toValue: 1,
duration: 1000,
useNativeDriver: true,
}).start();
}, [fadeAnim]);
return (
<Animated.View style={{ opacity: fadeAnim }}>
<Text>Fading in</Text>
</Animated.View>
);
What are React Native bridges, and how do you use them?
- Bridges in React Native allow you to write native code that interacts with JavaScript code. They are used when you need to access native APIs or functionality not available in React Native.
Explain the use of Expo in React Native development.
- Expo is a framework and platform for universal React applications. It provides a set of tools and services for building, deploying, and quickly iterating on React Native projects.
How do you handle push notifications in a React Native app?
- Push notifications can be handled using libraries like Firebase Cloud Messaging (FCM) or OneSignal.
What is the role of Metro bundler in React Native?
- Metro bundler is the JavaScript bundler for React Native. It takes care of transforming, bundling, and serving the JavaScript code.
How do you handle images and media in a React Native application?
- Images and media can be handled using the
Image
component and libraries like react-native-video.
- Images and media can be handled using the
What are some best practices for styling in React Native?
- Best practices include using StyleSheet, avoiding inline styles, and using libraries like styled-components or Emotion.
How do you handle device permissions in a React Native app?
- Device permissions can be handled using the
PermissionsAndroid
module for Android andreact-native-permissions
library for both iOS and Android.
- Device permissions can be handled using the
import { PermissionsAndroid } from 'react-native';
async function requestCameraPermission() {
try {
const granted = await PermissionsAndroid.request(
PermissionsAndroid.PERMISSIONS.CAMERA,
{
title: 'Cool Photo App Camera Permission',
message:
'Cool Photo App needs access to your camera ' +
'so you can take awesome pictures.',
buttonNeutral: 'Ask Me Later',
buttonNegative: 'Cancel',
buttonPositive: 'OK',
}
);
if (granted === PermissionsAndroid.RESULTS.GRANTED) {
console.log('You can use the camera');
} else {
console.log('Camera permission denied');
}
} catch (err) {
console.warn(err);
}
}
How do you handle different screen sizes and orientations in React Native?
- Different screen sizes and orientations can be handled using Flexbox, Dimensions API, and libraries like react-native-responsive-screen.
MongoDB Questions and Answers
What is MongoDB, and why is it used?
- MongoDB is a NoSQL database that uses a document-oriented data model. It is used for its flexibility, scalability, and ease of use.
Explain the difference between SQL and NoSQL databases.
- SQL databases are relational and use structured query language, while NoSQL databases are non-relational and can use various data models, including document, key-value, graph, and column-family.
How do you define a schema in MongoDB?
- MongoDB is schema-less, but you can define a schema using Mongoose in Node.js.
const mongoose = require('mongoose');
const userSchema = new mongoose.Schema({
name: String,
age: Number,
email: String,
});
const User = mongoose.model('User', userSchema);
What are collections in MongoDB?
- Collections are groups of MongoDB documents, similar to tables in relational databases.
How do you insert a document into a MongoDB collection?
- You can insert a document using the
insertOne
orinsertMany
methods.
- You can insert a document using the
const { MongoClient } = require('mongodb');
async function run() {
const client = new MongoClient('mongodb://localhost:27017');
await client.connect();
const db = client.db('mydatabase');
const collection = db.collection('users');
const result = await collection.insertOne({ name: 'John', age: 30, email: 'john@example.com' });
console.log(`New user created with the following id: ${result.insertedId}`);
await client.close();
}
run().catch(console.dir);
How do you query a document in MongoDB?
- You can query a document using the
find
method.
- You can query a document using the
const { MongoClient } = require('mongodb');
async function run() {
const client = new MongoClient('mongodb://localhost:27017');
await client.connect();
const db = client.db('mydatabase');
const collection = db.collection('users');
const user = await collection.findOne({ name: 'John' });
console.log(user);
await client.close();
}
run().catch(console.dir);
What is the difference between findOne and find in MongoDB?
findOne
returns the first document that matches the query, whilefind
returns a cursor to all matching documents.
How do you update a document in MongoDB?
- You can update a document using the
updateOne
orupdateMany
methods.
- You can update a document using the
const { MongoClient } = require('mongodb');
async function run() {
const client = new MongoClient('mongodb://localhost:27017');
await client.connect();
const db = client.db('mydatabase');
const collection = db.collection('users');
const result = await collection.updateOne({ name: 'John' }, { $set: { age: 31 } });
console.log(`${result.matchedCount} document(s) matched the filter, updated ${result.modifiedCount} document(s)`);
await client.close();
}
run().catch(console.dir);
How do you delete a document in MongoDB?
- You can delete a document using the
deleteOne
ordeleteMany
methods.
- You can delete a document using the
const { MongoClient } = require('mongodb');
async function run() {
const client = new MongoClient('mongodb://localhost:27017');
await client.connect();
const db = client.db('mydatabase');
const collection = db.collection('users');
const result = await collection.deleteOne({ name: 'John' });
console.log(`${result.deletedCount} document(s) was/were deleted`);
await client.close();
}
run().catch(console.dir);
Explain the use of indexes in MongoDB.
- Indexes are used to improve the performance of search operations by providing faster access to documents.
const { MongoClient } = require('mongodb');
async function run() {
const client = new MongoClient('mongodb://localhost:27017');
await client.connect();
const db = client.db('mydatabase');
const collection = db.collection('users');
await collection.createIndex({ name: 1 });
await client.close();
}
run().catch(console.dir);
How do you handle transactions in MongoDB?
- Transactions in MongoDB allow you to execute multiple operations in a sequence as a single atomic operation.
const { MongoClient } = require('mongodb');
async function run() {
const client = new MongoClient('mongodb://localhost:27017');
await client.connect();
const session = client.startSession();
try {
session.startTransaction();
const db = client.db('mydatabase');
const collection = db.collection('users');
await collection.insertOne({ name: 'Jane', age: 25, email: 'jane@example.com' }, { session }); await collection.updateOne({ name: 'John' }, { $set: { age: 32 } }, { session });
await session.commitTransaction(); } catch (error) { await session.abortTransaction(); throw error; } finally { session.endSession(); await client.close(); } }
run().catch(console.dir);
What are the aggregation pipelines in MongoDB?
- Aggregation pipelines are a framework for performing data aggregation operations, such as filtering, grouping, and transforming data.
const { MongoClient } = require('mongodb');
async function run() {
const client = new MongoClient('mongodb://localhost:27017');
await client.connect();
const db = client.db('mydatabase');
const collection = db.collection('users');
const pipeline = [
{ $match: { age: { $gte: 30 } } },
{ $group: { _id: '$age', count: { $sum: 1 } } },
{ $sort: { count: -1 } },
];
const result = await collection.aggregate(pipeline).toArray();
console.log(result);
await client.close();
}
run().catch(console.dir);
How do you perform data validation in MongoDB?
- Data validation can be performed using JSON schema validation in MongoDB.
const { MongoClient } = require('mongodb');
async function run() {
const client = new MongoClient('mongodb://localhost:27017');
await client.connect();
const db = client.db('mydatabase');
const collection = db.collection('users');
await db.command({
collMod: 'users',
validator: {
$jsonSchema: {
bsonType: 'object',
required: ['name', 'age', 'email'],
properties: {
name: {
bsonType: 'string',
description: 'must be a string and is required',
},
age: {
bsonType: 'int',
minimum: 0,
description: 'must be an integer and is required',
},
email: {
bsonType: 'string',
pattern: '^.+@.+$',
description: 'must be a string and match the regular expression pattern',
},
},
},
},
});
await client.close();
}
run().catch(console.dir);
What are the advantages and disadvantages of using MongoDB?
Advantages: Scalability, flexibility, high performance, and ease of use.
Disadvantages: Lack of ACID transactions (prior to version 4.0), potential for data inconsistency, and limited support for complex queries compared to SQL databases.
How do you backup and restore a MongoDB database?
- You can backup and restore a MongoDB database using the
mongodump
andmongorestore
utilities.
- You can backup and restore a MongoDB database using the
# Backup
mongodump --db=mydatabase --out=/path/to/backup
# Restore
mongorestore --db=mydatabase /path/to/backup/mydatabase
Explain sharding in MongoDB.
- Sharding is a method for distributing data across multiple servers to handle large datasets and high throughput operations.
What is the replica set in MongoDB?
- A replica set is a group of MongoDB instances that maintain the same data set, providing redundancy and high availability.
How do you monitor the performance of a MongoDB database?
- Performance can be monitored using tools like MongoDB Atlas, MMS, and the built-in monitoring commands.
What is the use of the $lookup operator in MongoDB?
- The
$lookup
operator is used for performing left outer joins to retrieve documents from another collection.
- The
Explain the use of GridFS in MongoDB.
- GridFS is a specification for storing and retrieving large files, such as images, videos, and other binary data, in MongoDB.
AWS Questions and Answers
What are the core services provided by AWS?
- The core services provided by AWS include Compute (EC2, Lambda), Storage (S3, EBS), Databases (RDS, DynamoDB), Networking (VPC, Route 53), and Security (IAM, KMS).
How do you deploy a Node.js application on AWS?
- You can deploy a Node.js application on AWS using services like Elastic Beanstalk, EC2, or Lambda. For example, using Elastic Beanstalk, you simply upload your application, and AWS handles the deployment, scaling, and monitoring.
Explain how to use AWS Lambda and its use cases.
- AWS Lambda is a serverless compute service that allows you to run code without provisioning servers. Use cases include data processing, real-time file processing, and back-end services for web and mobile applications.
How do you set up a database on AWS (e.g., RDS, DynamoDB)?
- To set up an RDS database, you create a DB instance, configure settings like engine type, instance class, and storage, and then connect to it. For DynamoDB, you create a table, define its primary key, and manage its throughput settings.
What is the AWS Well-Architected Framework?
- The AWS Well-Architected Framework provides best practices and guidelines for designing and operating reliable, secure, efficient, and cost-effective systems in the cloud. It consists of five pillars: Operational Excellence, Security, Reliability, Performance Efficiency, and Cost Optimization.
How do you manage IAM roles and policies in AWS?
- IAM roles and policies are managed through the IAM service. You create roles with specific permissions and attach policies that define what actions are allowed or denied.
What is Amazon S3, and how do you use it?
- Amazon S3 is an object storage service that allows you to store and retrieve any amount of data. You use it by creating buckets, uploading files, and setting permissions for access.
Explain the concept of VPC in AWS.
- A Virtual Private Cloud (VPC) is a virtual network dedicated to your AWS account. It allows you to launch AWS resources in a logically isolated network and control network settings like IP addresses, subnets, route tables, and security groups.
How do you set up and use CloudFront in AWS?
- AWS CloudFront is a Content Delivery Network (CDN) service. To set it up, you create a distribution, specify the origin server (e.g., an S3 bucket or an EC2 instance), and configure settings like caching behavior and security.
What is AWS CloudFormation, and how does it work?
- AWS CloudFormation allows you to define and provision infrastructure as code. You create templates that describe the resources and their configurations, and CloudFormation handles the provisioning and deployment.
How do you monitor AWS resources using CloudWatch?
- AWS CloudWatch monitors resources by collecting and tracking metrics, setting alarms, and logging data. You can create dashboards to visualize metrics and configure alerts to notify you of issues.
What are AWS Elastic Beanstalk and its use cases?
- AWS Elastic Beanstalk is a service for deploying and managing applications. It abstracts the infrastructure, allowing you to focus on code. Use cases include web applications, APIs, and background worker processes.
How do you use AWS Fargate with ECS?
- AWS Fargate is a serverless compute engine for containers. To use it with ECS, you create an ECS cluster, define task definitions, and run tasks without managing the underlying infrastructure.
What is the difference between EC2 and Lambda?
- EC2 provides virtual servers that you manage, offering full control over the operating system and software stack. Lambda is a serverless service that runs code in response to events, without managing servers.
How do you handle data backup and recovery in AWS?
- Data backup and recovery can be managed using services like AWS Backup, which automates backups of AWS resources, or by creating manual snapshots of EBS volumes, RDS instances, and using S3 for data storage.
What are AWS security groups, and how do they work?
- AWS security groups act as virtual firewalls for your instances. They control inbound and outbound traffic by defining rules based on IP addresses, protocols, and ports.
How do you set up auto-scaling in AWS?
- Auto-scaling in AWS is set up using Auto Scaling groups. You define scaling policies based on metrics like CPU utilization, and AWS automatically adjusts the number of instances to maintain performance.
Explain the use of AWS Route 53.
- AWS Route 53 is a scalable Domain Name System (DNS) service. It translates domain names into IP addresses and can be used to route end users to applications, ensuring high availability and reliability.
How do you optimize costs in AWS?
- Cost optimization in AWS involves using cost-effective services, rightsizing instances, leveraging reserved instances and spot instances, and using AWS Cost Explorer and Trusted Advisor to identify savings opportunities.
What is AWS CodePipeline, and how is it used for CI/CD?
- AWS CodePipeline is a continuous integration and continuous delivery (CI/CD) service. It automates the build, test, and deploy phases of your release process, integrating with services like CodeCommit, CodeBuild, and CodeDeploy.
Interview Preparation Tips:
Understand the Basics: Make sure you have a solid understanding of the core concepts.
Practice Coding: Solve problems on platforms like LeetCode, HackerRank, or CodeSignal.
Build Projects: Showcase your skills through projects that demonstrate your abilities.
Mock Interviews: Practice with mock interviews to get comfortable with the format.
Review Fundamentals: Brush up on data structures, algorithms, and system design.
Stay Updated: Keep up with the latest trends and updates in the technologies you work with.
Soft Skills: Communication, problem-solving, and teamwork are also crucial.
By the end of this guide, you will have a deeper understanding of the technologies and practices that drive successful full stack development. You'll be prepared to tackle complex challenges, contribute meaningfully to your team, and play a pivotal role in the world of software development. So, let’s embark on this journey together and master the art of Full Stack Development for a thriving tech future.
Subscribe to my newsletter
Read articles from Narottam Choudhary directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
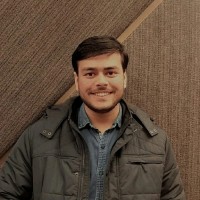
Narottam Choudhary
Narottam Choudhary
Not only Just a Coder Having skills in tech field and Also Having a great touch in music And a Music producer