Go Series Part 8: Time in Go

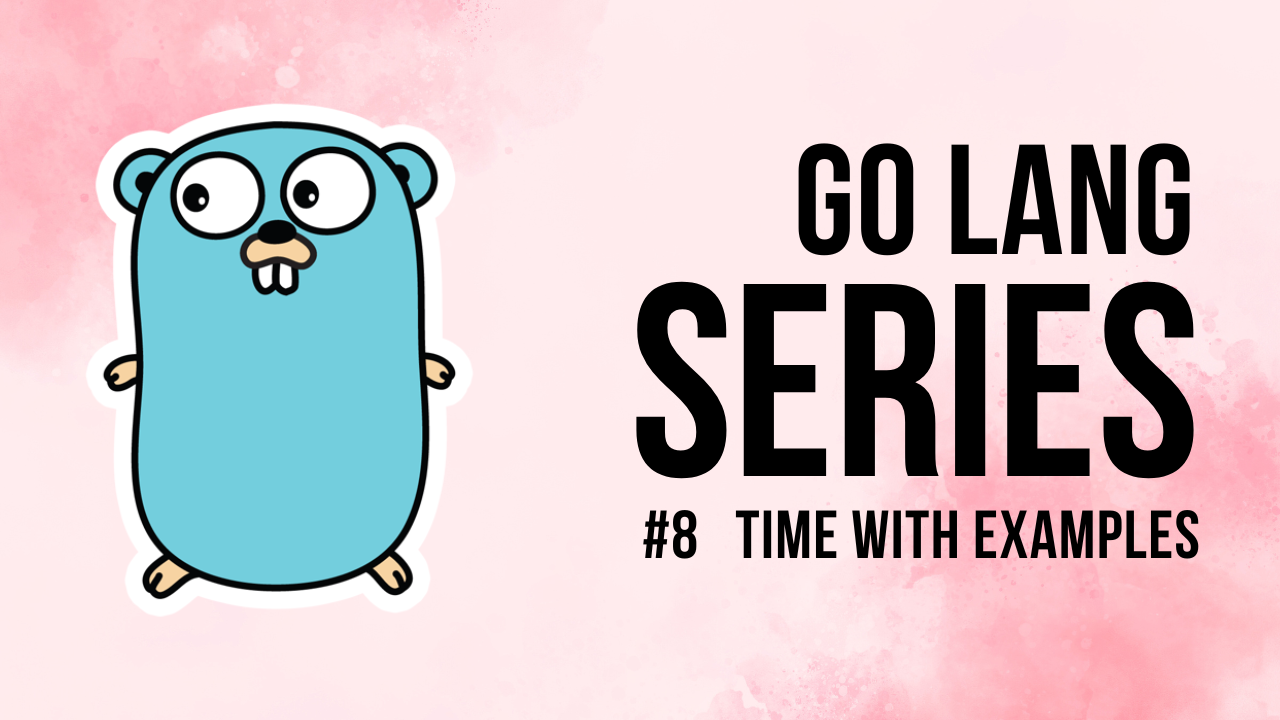
Introduction
Handling time is a common requirement in many applications. Go provides a robust time
package that allows you to work with dates and times efficiently. In this part of our series, we will explore various aspects of working with time in Go, including getting the current time, formatting dates, parsing strings into time objects, and calculating durations.
Example 1: Getting the Current Time
Let's start with a basic example of getting the current date and time.
package main
import (
"fmt"
"time"
)
func main() {
currentTime := time.Now()
fmt.Println("Current Time:", currentTime)
}
Output:
Current Time: 2024-07-20 15:04:05.999999999 -0700 MST
Explanation:
time.Now
()
returns the current local time.The
fmt.Println
function prints the current time.
Example 2: Formatting Time
You can format time in various ways using the Format
method with predefined layout constants.
package main
import (
"fmt"
"time"
)
func main() {
currentTime := time.Now()
formattedTime := currentTime.Format("2006-01-02 15:04:05")
fmt.Println("Formatted Time:", formattedTime)
}
Output:
Formatted Time: 2024-07-20 15:04:05
Explanation:
currentTime.Format("2006-01-02 15:04:05")
formats the time according to the provided layout.The layout uses the reference time
Mon Jan 2 15:04:05 MST 2006
.
Example 3: Parsing Time
Parsing a string into a time.Time
object is useful for working with date and time inputs.
package main
import (
"fmt"
"time"
)
func main() {
timeString := "2024-07-20 15:04:05"
parsedTime, err := time.Parse("2006-01-02 15:04:05", timeString)
if err != nil {
fmt.Println("Error parsing time:", err)
} else {
fmt.Println("Parsed Time:", parsedTime)
}
}
Output:
Parsed Time: 2024-07-20 15:04:05 +0000 UTC
Explanation:
time.Parse("2006-01-02 15:04:05", timeString)
parses the string into atime.Time
object.The layout must match the format of the input string.
Example 4: Calculating Durations
You can calculate the duration between two times using the Sub
method.
package main
import (
"fmt"
"time"
)
func main() {
startTime := time.Now()
time.Sleep(2 * time.Second)
endTime := time.Now()
duration := endTime.Sub(startTime)
fmt.Println("Duration:", duration)
}
Output:
Duration: 2s
Explanation:
time.Sleep(2 * time.Second)
pauses the program for 2 seconds.endTime.Sub(startTime)
calculates the duration betweenstartTime
andendTime
.
Example 5: Working with Time Zones
You can work with different time zones using the time.LoadLocation
function.
package main
import (
"fmt"
"time"
)
func main() {
location, err := time.LoadLocation("America/New_York")
if err != nil {
fmt.Println("Error loading location:", err)
return
}
currentTime := time.Now().In(location)
fmt.Println("Current Time in New York:", currentTime)
}
Output:
Current Time in New York: 2024-07-20 18:04:05 -0400 EDT
Explanation:
time.LoadLocation("America/New_York")
loads the time zone location.time.Now
().In(location)
gets the current time in the specified time zone.
Conclusion
Working with time in Go is straightforward with the time
package. You can easily get the current time, format dates, parse strings into time objects, calculate durations, and work with different time zones. These examples cover some of the most common time-related tasks you might encounter in Go.
Next, we'll continue to explore more advanced topics in Go. If you have any questions or need further clarification, feel free to ask!
Subscribe to my newsletter
Read articles from Navya A directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Navya A
Navya A
๐ Welcome to my Hashnode profile! I'm a passionate technologist with expertise in AWS, DevOps, Kubernetes, Terraform, Datree, and various cloud technologies. Here's a glimpse into what I bring to the table: ๐ Cloud Aficionado: I thrive in the world of cloud technologies, particularly AWS. From architecting scalable infrastructure to optimizing cost efficiency, I love diving deep into the AWS ecosystem and crafting robust solutions. ๐ DevOps Champion: As a DevOps enthusiast, I embrace the culture of collaboration and continuous improvement. I specialize in streamlining development workflows, implementing CI/CD pipelines, and automating infrastructure deployment using modern tools like Kubernetes. โต Kubernetes Navigator: Navigating the seas of containerization is my forte. With a solid grasp on Kubernetes, I orchestrate containerized applications, manage deployments, and ensure seamless scalability while maximizing resource utilization. ๐๏ธ Terraform Magician: Building infrastructure as code is where I excel. With Terraform, I conjure up infrastructure blueprints, define infrastructure-as-code, and provision resources across multiple cloud platforms, ensuring consistent and reproducible deployments. ๐ณ Datree Guardian: In my quest for secure and compliant code, I leverage Datree to enforce best practices and prevent misconfigurations. I'm passionate about maintaining code quality, security, and reliability in every project I undertake. ๐ Cloud Explorer: The ever-evolving cloud landscape fascinates me, and I'm constantly exploring new technologies and trends. From serverless architectures to big data analytics, I'm eager to stay ahead of the curve and help you harness the full potential of the cloud. Whether you need assistance in designing scalable architectures, optimizing your infrastructure, or enhancing your DevOps practices, I'm here to collaborate and share my knowledge. Let's embark on a journey together, where we leverage cutting-edge technologies to build robust and efficient solutions in the cloud! ๐๐ป