Day 10: Shell Script for CPU, Memory, Disk, and Service Monitoring

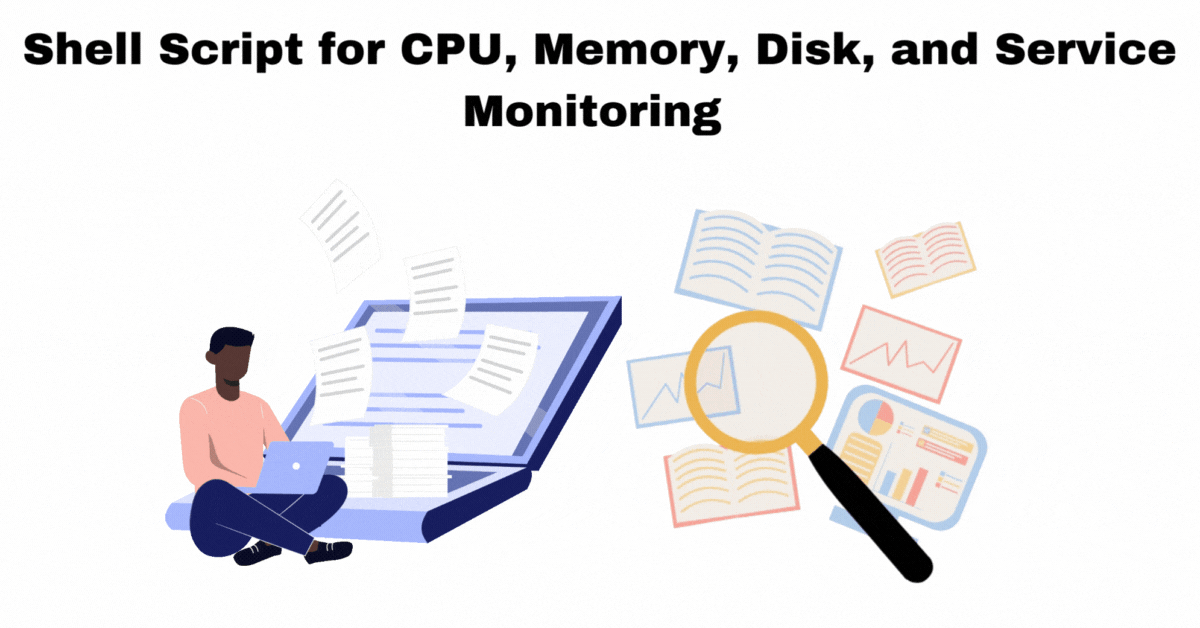
In this article I have share the shell script that monitors system metrics like CPU usage, memory usage, and disk space usage. The script will provide a user-friendly interface, allow continuous monitoring with a specified sleep interval, and extend its capabilities to monitor specific services like Nginx.
#!/bin/bash
function cpu_usage {
echo "CPU Usage is:"
top -bn1 | grep "Cpu(s)" | awk '{print $2}'
}
function memory_usage {
free -h | awk 'NR==2{printf "Memory Usage: %s, Free: %s\n", $3, $4}'
}
function disk_usage {
df -h | awk '$NF=="/"{printf "Used: %d/%d %s\n", $3, $2, $5}'
}
function monitor_service(){
local service_name=$1
if sudo systemctl is-active --quiet $service_name;then
echo "Service: $service_name is running"
else
echo "Service: $service_name is not running"
echo "Do you want to start the service $service_name (y/n): "
read option
if [[ $option == 'y' || $option == 'Y' ]]; then
sudo systemtl start $service_name
if sudo systemctl is-active --quiet $service_name;then
echo "Service: $service_name has been started sucessfully"
else
echo "Error: Failed to start service"
fi
fi
fi
}
function display_menu(){
echo "1. Show CPU Usage"
echo "2. Show Memory Usage"
echo "3. Show Disk Usage"
echo "4. Monitor Service"
echo "5. Exit"
echo -n "Please Enter the option from 1 to 5:"
}
function monitor_metrics(){
while true; do
clear
echo "Monitoring Metrics"
echo "------------------"
display_menu
read user_selection
case $user_selection in
1)
cpu_usage
;;
2)
memory_usage
;;
3)
disk_usage
;;
4)
echo "Please enter the service name"
read service_name
monitor_service $service_name
;;
5)
echo "Exiting ..."
break
;;
*)
echo "Incorrect Input: Please enter valid choice"
;;
esac
echo "Enter the sleep interval before the next update (or q for quit):"
read sleep_interval
if [[ $sleep_interval == 'q' || $sleep_interval == 'Q' ]]; then
echo "Exiting ..."
break
elif ! [[ $sleep_interval =~ ^[0-9]+$ ]]; then
echo "Incorrect value: Please enter only numeric value"
else
sleep $sleep_interval
fi
done
}
monitor_metrics
Create Cpu_usage() function:
Here I have first created a function named cpu_usage, This function will return only the cpu used. From top command we are trying to exact the highlighted values in the snapshot and for doing so we will run command top -bn1 | grep "Cpu(s)" | awk '{print $2}' .
Create memory_usage() function:
In this function i am trying to print memory used and free memory command
free -h | awk 'NR==2{printf "Memory Usage: %s, Free: %s\n", $3, $4}'
Create disk_usage function:
In this function I am trying to print disk used out of total available disk space followed by the percentage of used disk space using the command
df -h | awk '$NF=="/"{printf "Used: %d/%d %s\n", $3, $2, $5}'
monitor_service function:
Here in this function we are trying to check if the service name provided by user is active and return a message to the user if not active asking to whether or not to start the service as it not running/active
Next display_menu function:
Here i am just printing the menu of task that has to be performed using echo command
monitor_metrics:
This is actually kinda main function where i am going to use all the functions created earlier using the use-case and one the actions are complete we ask for sleep interval and then again print the same menu using the while loop.
Snapshot of Output:
Subscribe to my newsletter
Read articles from Vandana Pandit directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vandana Pandit
Vandana Pandit
๐ฉโ๐ป I am currently working as Infrastructure Engineer. ๐ญ Iโm currently preparing for CKA certification. ๐ Do check my linked post I keep posting articles related to DevOps