Android Mastery: Visibility Modifiers
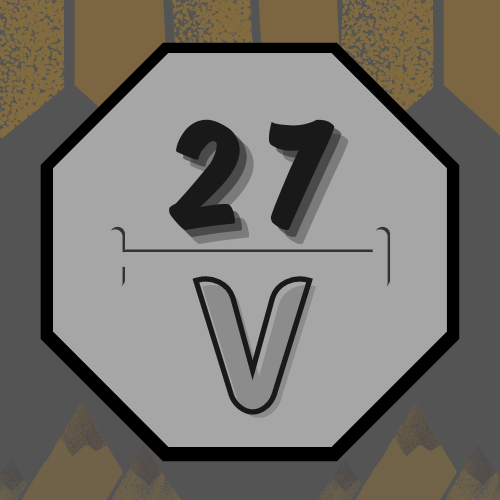
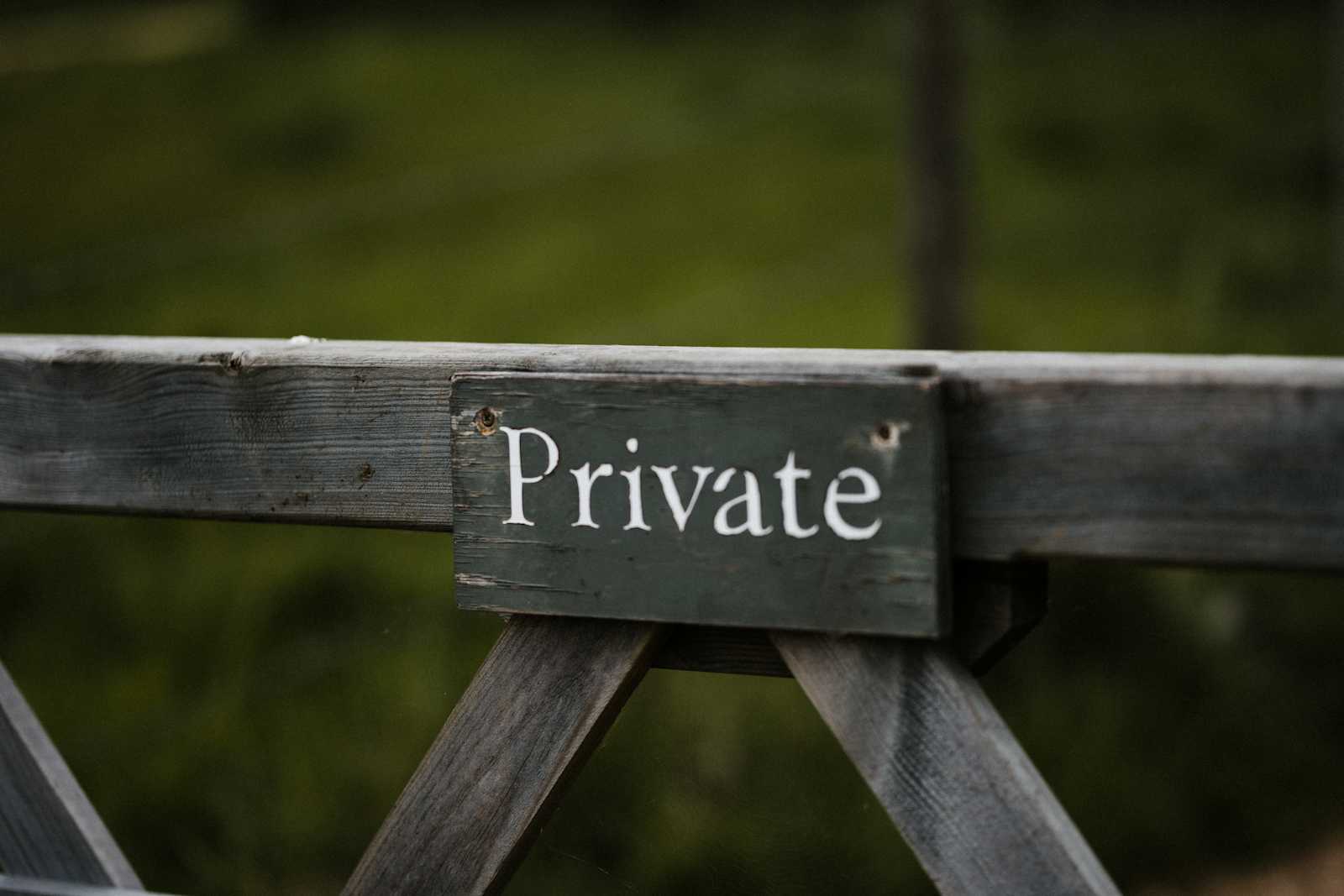
Visibility modifiers can be considered as the machine behind encapsulation.
As a quick reminder, encapsulation is the act of wrapping the properties of a class together with the methods that perform actions on those properties.
These visibility modifiers achieve encapsulation by:
Hiding a class’s properties and methods from unauthorized access outside the class.
Hiding a package’s classes and interfaces from unauthorized access outside the package.
In Kotlin, there are four visibility modifiers:
Public: the default visibility modifier allows property and method declarations accessible everywhere, meaning they are marked as public and can be used outside the lass.
Private: restricts the declarations to within the same source file or class. This is implemented for properties and methods you want to use within the class but don’t wish other classes to use.
Protected: restricts the declaration accessibility to the class and subclasses.
Internal: restricts the declaration accessibility to the same module. It is similar to private, however, the properties and methods can be accessed outside the class but within the same module.
Please Note:
Module: a collection of source files and build settings that allow for functionality unit divisions of a project. A project may have multiple modules that are built, tested, and debugged individually.
Package: similar to a directory or folder, a package is a grouping of related classes. A module can contain multiple packages.
Syntax
// [modifier] [declaration syntax]
Specifying A Visibility Modifier For Properties
// [modifier] var [name] : [data type] = [initial value]
class KotlinEngineer(name: String) :
SoftwareEngineer(name = name) {
// A Kotlin Engineer
...
/* 1. Take for example the declaration for
the kotlinExperience variable*/
private kotlinExperience: Int = 3
// 2. Can also be set to setter functions
/* PS: The visibility modifier for set and get
should match to avoid compiler errors. */
override var experience: Double = 10.5
protected set
...
}
Specifying Visibility Modifiers For Methods
/*
Syntax:
modifier fun [name]() {
[body]
}
*/
class KotlinEngineer(name: String) :
SoftwareEngineer(name = name) {
...
override var experience: Double = 10.5
protected set(value) {
if (experience > 0.0) {
field = value
}
}
protected fun checkSeniority() {
if (experience < 3.1) {
println("We have ourselves a junior Kotlin Engineer.")
}
else if (experience > 3.0 and experience < 5.1) {
println("We have ourselves a mid-level Kotlin Engineer.")
}
else if (experience > 5.0) {
println("We have ourselves a Senior Kotlin Engineer.")
}
else {
println("Please input the correct value.")
}
}
...
}
Specifying Visibility Modifiers For Constructors
/*
Syntax:
class [name] modifier constructor ([parameters]) {
[body]
}
*/
class KotlinEngineer protected constructor (name: String) :
SoftwareEngineer(name = name) {
...
}
Specifying Visibility Modifiers For Classes
/*
Syntax:
modifier class [name]() {
[body]
}
*/
internal class KotlinEngineer (name: String) :
SoftwareEngineer(name = name) {
...
}
Putting It All Together
TLDR; The following summarizes it for you lazy professional/student.
Modifier | Accessible in same class | Accessible in subclass | Accessible in same module | Accessible outside module |
private | Yes | No | No | No |
protected | Yes | Yes | No | No |
internal | Yes | Yes | Yes | No |
public | Yes | Yes | Yes | Yes |
Subscribe to my newsletter
Read articles from The Chief - Omar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
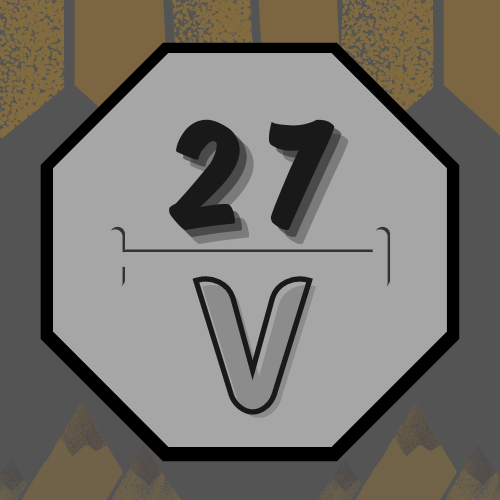
The Chief - Omar
The Chief - Omar
Bismillah I write on: Android, Python, Kotlin, Flutter, Programming, Linux, Bug Bounty, and more.