Schema Validation with Zod .

Table of contents
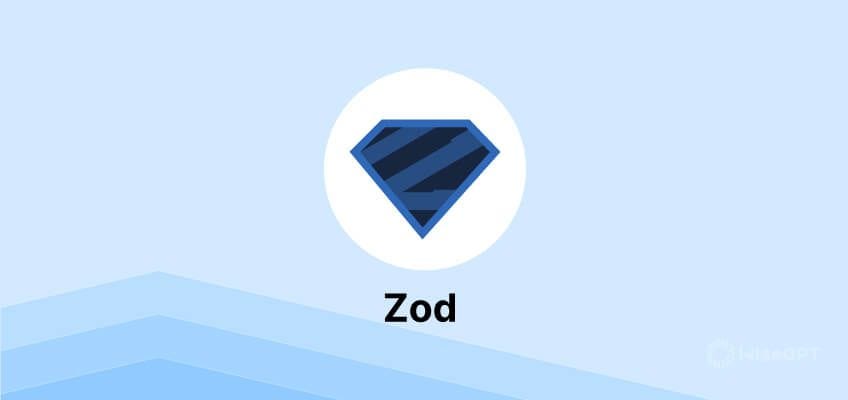
What is Zod ?
Zod is a TypeScript library used for creating schemas to validate data types. It helps ensure that the data our program receives or works with matches the expected format, like checking if a variable is a number, a string, or a more complex object with specific properties. Zod is particularly useful because it's designed with TypeScript's type system in mind. It allows developers to define schemas that not only validate the shape and type of data at runtime but also automatically infer TypeScript types from these schemas.
In Simple words Zod is a JavaScript and TypeScript library that allows developers to define schemas for their data. In this context, a schema is a blueprint that describes the shape and constraints of data.
Why Use Zod ?
Type Safety : After using Zod , You don't need to think about the type safety of the Schema in your application.Zod shines with TypeScript because it lets you infer types from schemas, so you don't have to write the same definition twice. But it can also be used in JavaScript codebases, where you can get runtime type safety even without TypeScript
simplified validation Logic : Zod provides a consistent and easy-to-use API for defining and validating schemas. This reduces boilerplate code and makes your validation logic more maintainable.
Installation
npm install zod # npm
yarn add zod # yarn
bun add zod # bun
pnpm add zod # pnpm
Basic usage
creating a simple string schema
import { z } from "zod";
// creating a schema for strings
const mySchema = z.string();
const messageSchema = z.string().min(3,"message has atleast 3 character ")
.max(100,"message must be no longer than 100 character")
// creating a schema for User
const UserSchema = z.object({
username : z.string()
.min(3,"username must have minimum 3 character")
.max(20 , "username must have less than 20 characters")
.regex(/^[a-zA-Z0-9_]+$/, "Username must not contain special characters"),
password: z.string().min(6,{message:"password must have minimum 6 character"}),
email: z.string().email()
})
Notice how Zod automatically inferred the type User out of UserSchema. Why is that a big deal? Because it creates a single source of truth for all our types and enforces any external input to confirm to that source of truth. Other schema validation libraries will force us to manually define types and keep those types in sync with our schemas.
Subscribe to my newsletter
Read articles from Sajan Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
