TypeScript and Tooling

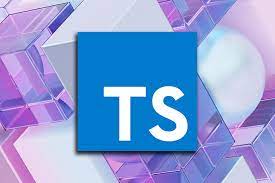
Welcome back to our TypeScript series!
Today, we’ll delve into the ecosystem of tools that enhance your TypeScript development experience. From IDE support to build tools and linters, understanding how to leverage these tools will significantly improve your productivity and code quality.
1. Integrated Development Environment (IDE) Support
TypeScript’s rich type system and static analysis capabilities are best experienced with the right IDE. Here are some popular options:
1.1 Visual Studio Code (VSCode)
VSCode is one of the most popular editors for TypeScript due to its extensive support and rich extensions.
TypeScript Language Features: Built-in support for TypeScript, including IntelliSense, type checking, and refactoring tools.
Extensions: Enhance your development experience with extensions like
ESLint
,Prettier
, andTypeScript Hero
.
Example Configuration:
Install extensions:
ESLint
: For lintingPrettier
: For code formattingTypeScript Hero
: For managing TypeScript imports
1.2 WebStorm
WebStorm by JetBrains is another powerful IDE for TypeScript development.
Smart Code Assistance: Advanced code completion, navigation, and refactoring tools.
Built-in Tools: Integrated version control, terminal, and debugging tools.
Example Configuration:
Enable TypeScript in the project settings.
Install and configure ESLint and Prettier plugins.
2. Build Tools
TypeScript can be integrated with various build tools to streamline the development process. Here are some popular options:
2.1 Webpack
Webpack is a powerful bundler for JavaScript and TypeScript applications.
- ts-loader: A TypeScript loader for Webpack that compiles TypeScript files.
Example Configuration:
npm install webpack webpack-cli ts-loader typescript --save-dev
webpack.config.js:
const path = require('path');
module.exports = {
entry: './src/index.ts',
module: {
rules: [
{
test: /\.ts$/,
use: 'ts-loader',
exclude: /node_modules/,
},
],
},
resolve: {
extensions: ['.ts', '.js'],
},
output: {
filename: 'bundle.js',
path: path.resolve(__dirname, 'dist'),
},
};
2.2 Parcel
Parcel is a zero-configuration web application bundler.
Example Configuration:
npm install -D parcel-bundler typescript
Update package.json
scripts:
{
"scripts": {
"build": "parcel build src/index.html",
"start": "parcel src/index.html"
}
}
3. Linting and Formatting
Ensuring code quality and consistency is crucial in any project. Here’s how you can use ESLint and Prettier with TypeScript:
3.1 ESLint
ESLint is a popular linter for identifying and fixing problems in your JavaScript and TypeScript code.
Example Configuration:
npm install eslint @typescript-eslint/parser @typescript-eslint/eslint-plugin --save-dev
.eslintrc.json:
{
"parser": "@typescript-eslint/parser",
"extends": [
"eslint:recommended",
"plugin:@typescript-eslint/recommended"
],
"parserOptions": {
"ecmaVersion": 2020,
"sourceType": "module"
},
"rules": {
// Add custom rules here
}
}
2.2 Prettier
Prettier is an opinionated code formatter that supports TypeScript.
Example Configuration:
npm install --save-dev prettier eslint-config-prettier eslint-plugin-prettier
.eslintrc.json (extended):
{
"extends": [
"plugin:@typescript-eslint/recommended",
"prettier/@typescript-eslint",
"plugin:prettier/recommended"
],
"plugins": ["@typescript-eslint", "prettier"],
"rules": {
"prettier/prettier": "error"
}
}
4. Testing
Testing is an integral part of software development. Here’s how you can set up testing in a TypeScript project:
4.1 Jest
Jest is a delightful JavaScript testing framework with built-in support for TypeScript.
Example Configuration:
npm install --save-dev jest ts-jest @types/jest
jest.config.js:
module.exports = {
preset: 'ts-jest',
testEnvironment: 'node',
testPathIgnorePatterns: ['/node_modules/'],
};
Example Test:
// src/sum.ts
export function sum(a: number, b: number): number {
return a + b;
}
// src/sum.test.ts
import { sum } from './sum';
test('adds 1 + 2 to equal 3', () => {
expect(sum(1, 2)).toBe(3);
});
4.2 Mocha and Chai
Mocha is a feature-rich JavaScript test framework, and Chai is an assertion library.
The assertion library is a testing tool used in software development to verify that certain conditions in your code are true. It allows developers to write tests and check if specific assumptions about their code hold during execution.
Example Configuration:
npm install --save-dev mocha chai ts-node @types/mocha @types/chai
Example Test:
// src/sum.test.ts
import { expect } from 'chai';
import { sum } from './sum';
describe('sum function', () => {
it('should add 1 and 2 to equal 3', () => {
expect(sum(1, 2)).to.equal(3);
});
});
5. Build and CI/CD Integration
Integrating TypeScript with your CI/CD pipeline ensures your code is always tested and built correctly.
5.1 GitHub Actions
GitHub Actions allows you to automate your workflow directly from your GitHub repository.
Example Workflow:
name: CI
on: [push, pull_request]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Setup Node.js
uses: actions/setup-node@v2
with:
node-version: '14'
- run: npm install
- run: npm run build
- run: npm test
Summary
Today, we explored the rich ecosystem of tools that enhance TypeScript development. From powerful IDE support and efficient build tools to rigorous linting, formatting, and testing frameworks, TypeScript’s tooling helps ensure high-quality, maintainable code. Integrating these tools into your workflow will significantly improve your development experience and productivity.
Next time, we’ll dive into real-world TypeScript patterns and best practices. Stay tuned!
Subscribe to my newsletter
Read articles from Anuj Kumar Upadhyay directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Anuj Kumar Upadhyay
Anuj Kumar Upadhyay
I am a developer from India. I am passionate to contribute to the tech community through my writing. Currently i am in my Graduation in Computer Application.