CodeSync: Building a Real-Time Collaborative Code Editor

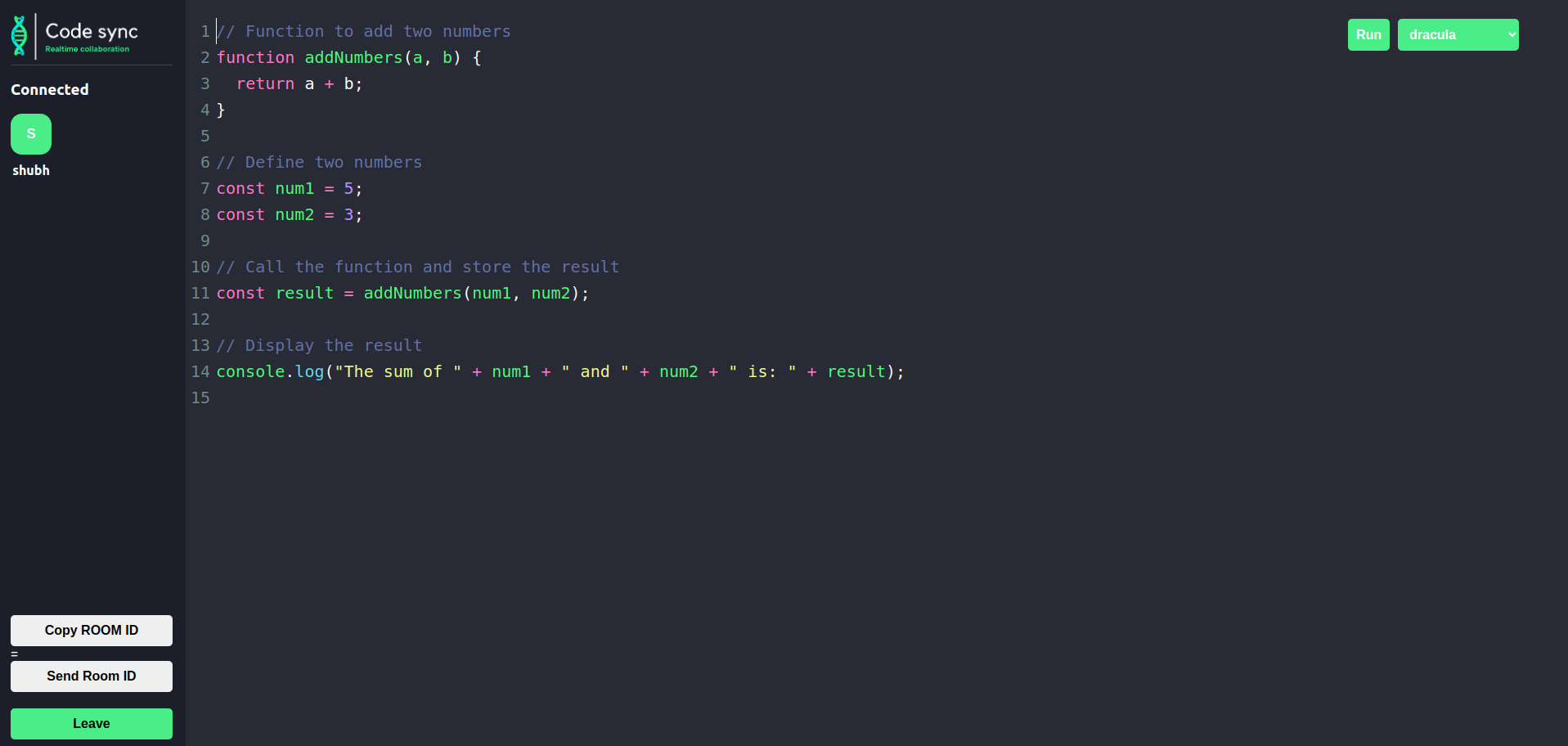
Welcome to an exciting journey where we explore the creation of CodeSync, a real-time collaborative code editor. This project allows multiple users to join, edit, and run JavaScript code in a synchronized manner. Each user can monitor the code execution and debug in real time. Notifications alert everyone when a new user joins or leaves the room. Additionally, users can toggle between ten different themes for a personalized experience.
Frontend Development
The frontend of CodeSync is built with React, providing a dynamic and responsive user interface. Here's a detailed look at the technologies and libraries used:
Technologies and Libraries
React: For building the user interface.
React Router DOM: To handle routing within the application.
Socket.ioClient: For real-time communication between the frontend and backend.
Axios: To make HTTP requests.
Codemirror: For the code editor with syntax highlighting and auto-closing brackets.
CSS: For styling the application.
React Hot Toast: To display notifications.
UUID: To generate unique IDs for each room.
Clone the repository:
git clone https://github.com/MerakiShubh/editSyncFrontend.git
Create a .env
file:
### Environment Variables
Make sure to set up the following environment variables in your
`.env` file:
```plaintext
VITE_BACKEND_URL="http://localhost:3000"
Backend Development
The backend is built with Node.js and Express, providing robust real-time capabilities. Here's a breakdown of the technologies and their roles:
Technologies and Libraries
Express: For building the server and handling API routes.
Socket.io: To manage real-time communication.
Twilio: For secure messaging services.
Redis: To cache user IDs and handle user sessions.
Features of CodeSync
Real-Time Collaboration: Users can join a room, edit code, and see changes in real time.
Run JavaScript Code: Users can run their JavaScript code, and everyone in the room can see the output.
Notifications: Best-in-class pop-up notifications when users join or leave the room.
Theme Toggle: Ten beautiful themes to choose from for a personalized coding experience.
Secure Messaging: Real-time messaging service using Twilio.
Syntax Highlighting: Enhanced code readability with beautiful syntax highlighting.
Auto-Closing Brackets: Automatically closes brackets to help prevent syntax errors.
Clone the repository:
git clone https://github.com/MerakiShubh/editSyncBackend.git
Create a .env
file:
### Environment Variables
Make sure to set up the following environment variables in your
`.env` file:
```plaintext
NODE_ENV="development",
PORT=3000
CORS_ORIGIN=*
SMS_SID=youtoken
SMS_AUTHTOKEN=yoursmstoken
SMS_FROM_NUMBER=yourregisteredphonenumber
FRONTEND_DOMAIN=http://localhost:5173
Deployment
Dockerfile
Create a Dockerfile
for the backend:
# Stage 1: Build the backend
FROM node:18-slim as build-backend
# Create and change to the app directory
WORKDIR /usr/src/app
# Copy application dependency manifests to the container image.
COPY package*.json ./
# Install production dependencies.
RUN npm install
# Copy local code to the container image.
COPY . .
# Expose the backend port
EXPOSE 3000
# Start the backend service
CMD ["npm", "run", "dev"]
Docker Compose
Create a docker-compose.yml
file to manage multiple services:
version: "3.8"
services:
backend:
build:
context: .
dockerfile: Dockerfile.backend
environment:
- NODE_ENV=production
- PORT=3000
- CORS_ORIGIN=*
- SMS_SID=${SMS_SID}
- SMS_AUTHTOKEN=${SMS_AUTHTOKEN}
- SMS_FROM_NUMBER=${SMS_FROM_NUMBER}
- FRONTEND_DOMAIN=${FRONTEND_DOMAIN}
ports:
- "3000:3000"
command: ["npm", "run", "dev"]
nginx:
build:
context: .
dockerfile: Dockerfile.nginx
ports:
- "80:80"
depends_on:
- backend
Nginx Configuration
Create an nginx.conf
file to handle requests:
events {
worker_connections 1024;
}
http {
resolver 127.0.0.11 valid=30s;
include /etc/nginx/mime.types;
default_type application/octet-stream;
server {
listen 80;
# Serve static files
location / {
root /usr/src/app/frontend/build;
try_files $uri /index.html;
}
# Proxy backend requests
location /api/ {
proxy_pass http://backend:3000/;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
proxy_set_header X-Forwarded-Proto $scheme;
}
}
}
Dockerfile.nginx
Create a Docker.nginx
file to set up Nginx:
# Stage 2: Setup NGINX
FROM nginx:latest
# Install netcat
RUN apt-get update && apt-get install -y netcat-openbsd && apt-get clean
# Copy the build files from the previous stage
COPY build /usr/src/app
# Copy your custom NGINX configuration file
COPY nginx.conf /etc/nginx/nginx.conf
# Copy the entrypoint script
COPY entrypoint.sh /entrypoint.sh
# Make the entrypoint script executable
RUN chmod +x /entrypoint.sh
# Expose the port that NGINX will run on
EXPOSE 80
# Use the entrypoint script to start NGINX
ENTRYPOINT ["/entrypoint.sh"]
Entrypoint Script
Create an entrypoint.sh
script for any initialization:
#!/bin/sh
# Wait for the backend service to be available
while ! nc -z backend 3000; do
echo "Waiting for the backend service..."
sleep 2
done
# Start NGINX
nginx -g 'daemon off;'
Conclusion
Congratulations! You've built CodeSync, a real-time collaborative code editor with a robust backend, a dynamic frontend, and efficient deployment. This project not only showcases advanced real-time features but also highlights the seamless integration of various technologies to create a cohesive user experience. Deploy this application, and enjoy coding together in real time!
Feel free to reach out if you have any questions or need further assistance.
Happy coding!
Usefull Links
Subscribe to my newsletter
Read articles from Shubham Tiwari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shubham Tiwari
Shubham Tiwari
Full-stack Software Developer and Javascript Enthusiast, Who Loves Building Things In Javascript. ๐ฅ ๐จ๐ฝโ๐ป๐