What are tRPC's and why they are better than RESTFUL API'S
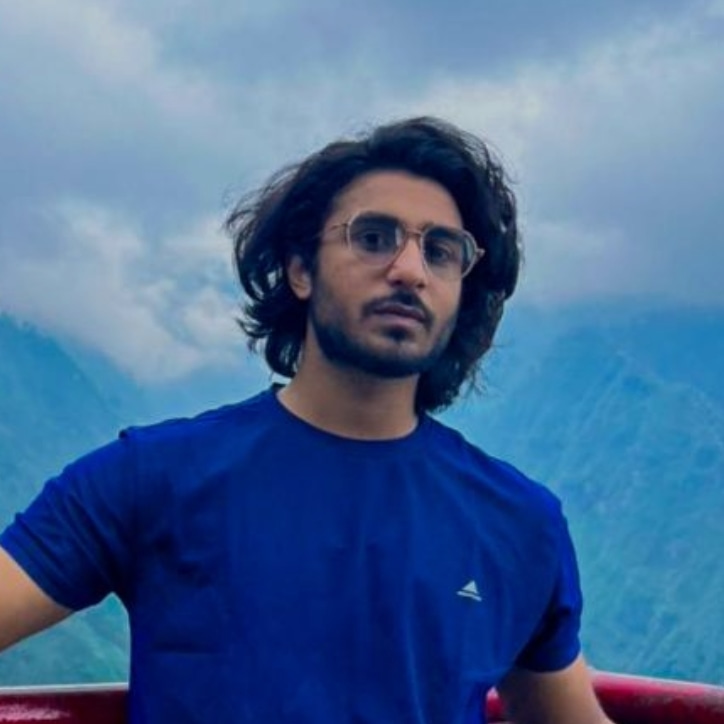
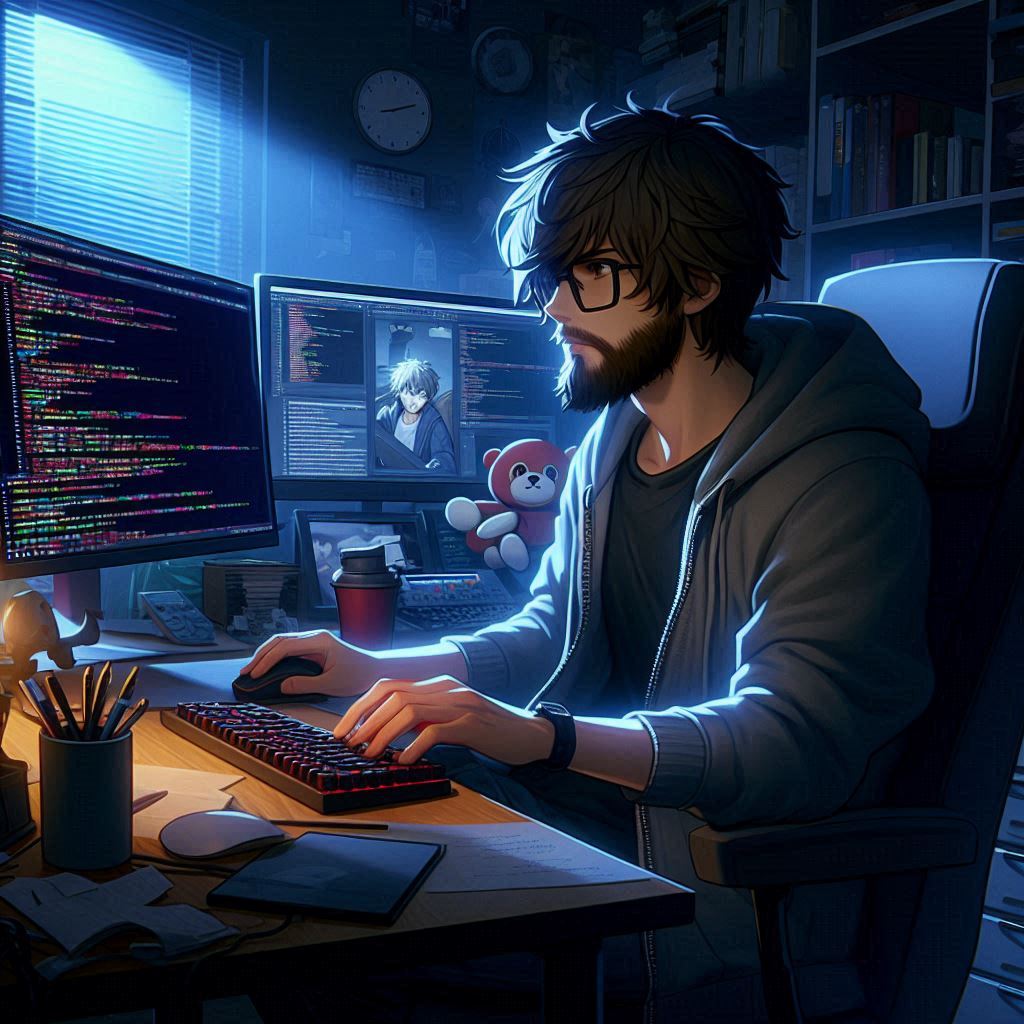
In order to understand tRPC'S first we need to understand what are RPC'S
What are RPC'S
RPC's stands for remote procedure calls and as the name suggests it is a protocol used in network programming that allows a program to execute a procedure (a block of code) on a remote server as if it were a local call. This abstraction simplifies the process of inter-process communication over a network, enabling seamless interaction between client and server applications.
RPC simplifies network communication by abstracting the complexities involved in remote interactions, allowing developers to invoke procedures on remote servers as if they were local function calls. Here are the key ways RPC achieves this simplification:
Why use RPC's
how backends talk to each other in a http request.
In this approach we have some flaws : -
the type of data is undefined.
You might be able to share types between 2 Node.js backends somehow, but if the other backend is in Rust, then you cant get back the types from it
We have to know what axios is , or what fetch is . We need to understand HTTP and how to call it
whereas, using tRPC'S(typed remote procedure calls) eliminates the ambiguity of types. And using tRPC'S removes the usage of "fetch" and "axios".
what is tRPC
tRPC stands for TypeScript Remote Procedure Call. It is a framework that helps developers create APIs in a simple and efficient way using TypeScript through remote function calls directly to the server from client side.
Advantages of tRPC
Easy to Use: RPC lets you ask another computer to do something just like asking a friend to help you, making it simple for programmers.
Fast: RPC can be quicker than other ways of talking to computers because it sends messages in a special way that makes them smaller and faster (FUNCTIONS).
Safe and Clear: With RPC, you know exactly what kind of information you’re sending and getting back, which helps avoid mistakes.
Flexible: RPC lets you send lots of information all at once, so you don’t have to make many trips back and forth.
Asynchronous Programming: Some RPCs let you keep doing things while you wait for answers, making your programs feel faster and more fun.
Interoperability: RPC can help different types of computers and programs talk to each other, even if they are not the same.
No need to call api endpoints again and again: RPC makes it easier for programmers to build and fix things with the help of functions so they can increase their workflow.
Offers Type-Safety: having type safe code make it less error prone.
How to create a simple tRPC Server
To create a simple tRPC server, follow these steps:
Initialize a new Node.js project:
mkdir trpc-server cd trpc-server npm init -y
Install necessary dependencies:
npm install @trpc/server @trpc/client zod express
Set up the server: Create a file named
server.ts
and add the following code:import express from 'express'; import * as trpc from '@trpc/server'; import * as trpcExpress from '@trpc/server/adapters/express'; import { z } from 'zod'; // Define the router const appRouter = trpc.router() .query('hello', { input: z .object({ name: z.string().nullish(), }) .nullish(), resolve({ input }) { return `Hello ${input?.name ?? 'world'}`; }, }); // Create the express app const app = express(); // Create the tRPC endpoint app.use( '/trpc', trpcExpress.createExpressMiddleware({ router: appRouter, createContext: () => null, }), ); // Start the server app.listen(4000, () => { console.log('Server is running on http://localhost:4000'); }); export type AppRouter = typeof appRouter;
Create a client to test the server: Create a file named
client.ts
and add the following code:import { createTRPCClient } from '@trpc/client'; import type { AppRouter } from './server'; const client = createTRPCClient<AppRouter>({ url: 'http://localhost:4000/trpc', }); async function main() { const result = await client.query('hello', { name: 'tRPC' }); console.log(result); // Output: Hello tRPC } main();
Subscribe to my newsletter
Read articles from Lakshay vaishnav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
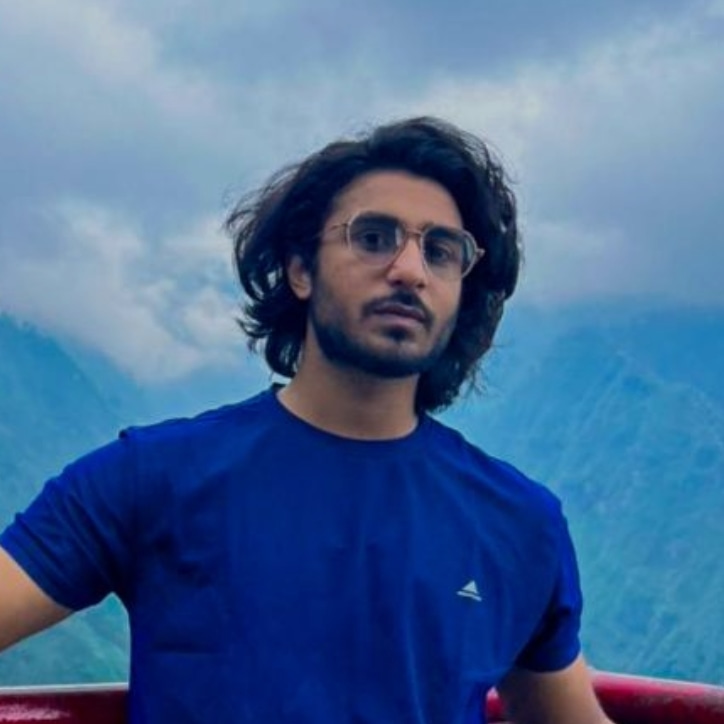