Conquering Callback Hell in JavaScript : Strategies and Solutions
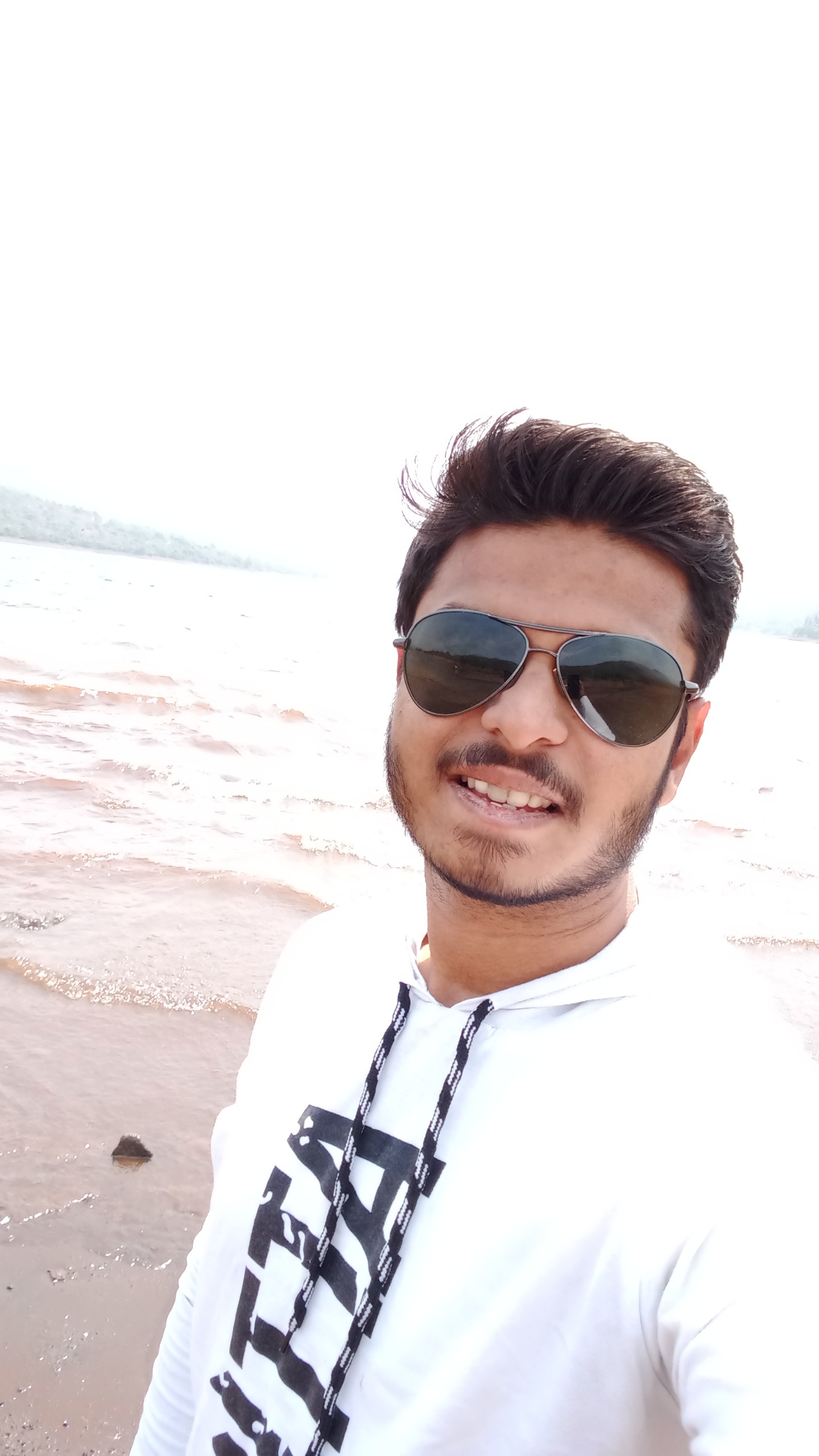
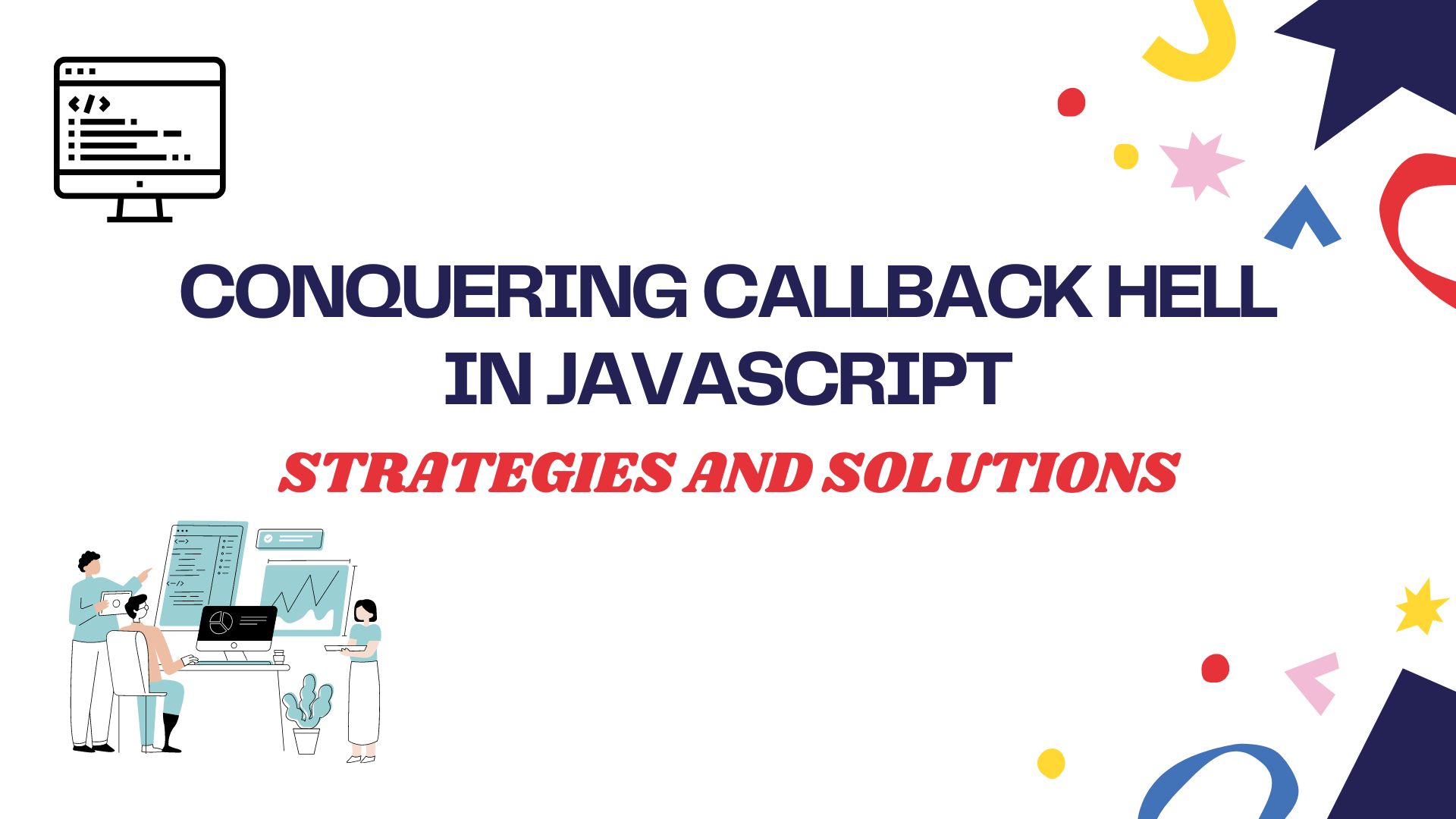
JavaScript is a versatile and powerful language, but as projects grow in complexity, managing asynchronous code can become a nightmare. This issue, often referred to as "callback hell," can make code difficult to read, maintain, and debug. In this blog post, we'll explore what callback hell is, why it happens, and how you can tame it using modern JavaScript techniques.
What is Callback Hell?
Callback hell, also known as "Pyramid of Doom," occurs when multiple nested callbacks are used to handle asynchronous operations. This leads to deeply nested code that resembles a pyramid and is hard to follow.
Example of Callback Hell
doSomething(function(err, result) {
if (err) {
// Handle error
} else {
doSomethingElse(result, function(err, result) {
if (err) {
// Handle error
} else {
doAnotherThing(result, function(err, result) {
if (err) {
// Handle error
} else {
doFinalThing(result, function(err, result) {
if (err) {
// Handle error
} else {
// Final result
}
});
}
});
}
});
}
});
In this example, each function call depends on the result of the previous one, creating a deeply nested structure that is hard to read and maintain.
Why Does Callback Hell Happen?
Callback hell typically arises from the need to perform multiple asynchronous operations in sequence, where each operation depends on the result of the previous one. As the number of operations increases, the code becomes more nested and complex.
Strategies to Avoid Callback Hell
1. Modularize Your Code
Breaking down your code into smaller, reusable functions can help reduce nesting and improve readability.
function handleResult(err, result, next) {
if (err) {
// Handle error
} else {
next(result);
}
}
doSomething(function(err, result) {
handleResult(err, result, function(result) {
doSomethingElse(result, function(err, result) {
handleResult(err, result, function(result) {
doAnotherThing(result, function(err, result) {
handleResult(err, result, function(result) {
doFinalThing(result, function(err, result) {
handleResult(err, result, function(result) {
// Final result
});
});
});
});
});
});
});
});
2. Use Promises
Promises provide a more elegant way to handle asynchronous operations. They allow you to chain operations, avoiding deep nesting.
doSomething()
.then(result => doSomethingElse(result))
.then(result => doAnotherThing(result))
.then(result => doFinalThing(result))
.then(result => {
// Final result
})
.catch(err => {
// Handle error
});
3. Utilize Async/Await
Async/await, introduced in ES8, simplifies asynchronous code, making it look and behave more like synchronous code. This reduces nesting and improves readability.
async function performTasks() {
try {
const result1 = await doSomething();
const result2 = await doSomethingElse(result1);
const result3 = await doAnotherThing(result2);
const result4 = await doFinalThing(result3);
// Final result
} catch (err) {
// Handle error
}
}
performTasks();
4. Use Async Libraries
Libraries like async provide utilities to manage asynchronous code and avoid callback hell.
const async = require('async');
async.waterfall([
function(callback) {
doSomething(callback);
},
function(result, callback) {
doSomethingElse(result, callback);
},
function(result, callback) {
doAnotherThing(result, callback);
},
function(result, callback) {
doFinalThing(result, callback);
}
], function (err, result) {
if (err) {
// Handle error
} else {
// Final result
}
});
Conclusion
Callback hell can be a significant hurdle in JavaScript development, but modern techniques and tools provide effective solutions. By modularizing your code, using promises, leveraging async/await, and utilizing async libraries, you can transform your asynchronous code from a tangled mess into a well-structured and maintainable piece of art.
Embrace these strategies to conquer callback hell and elevate your JavaScript skills to new heights.
Subscribe to my newsletter
Read articles from Shreeyog Gaikwad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
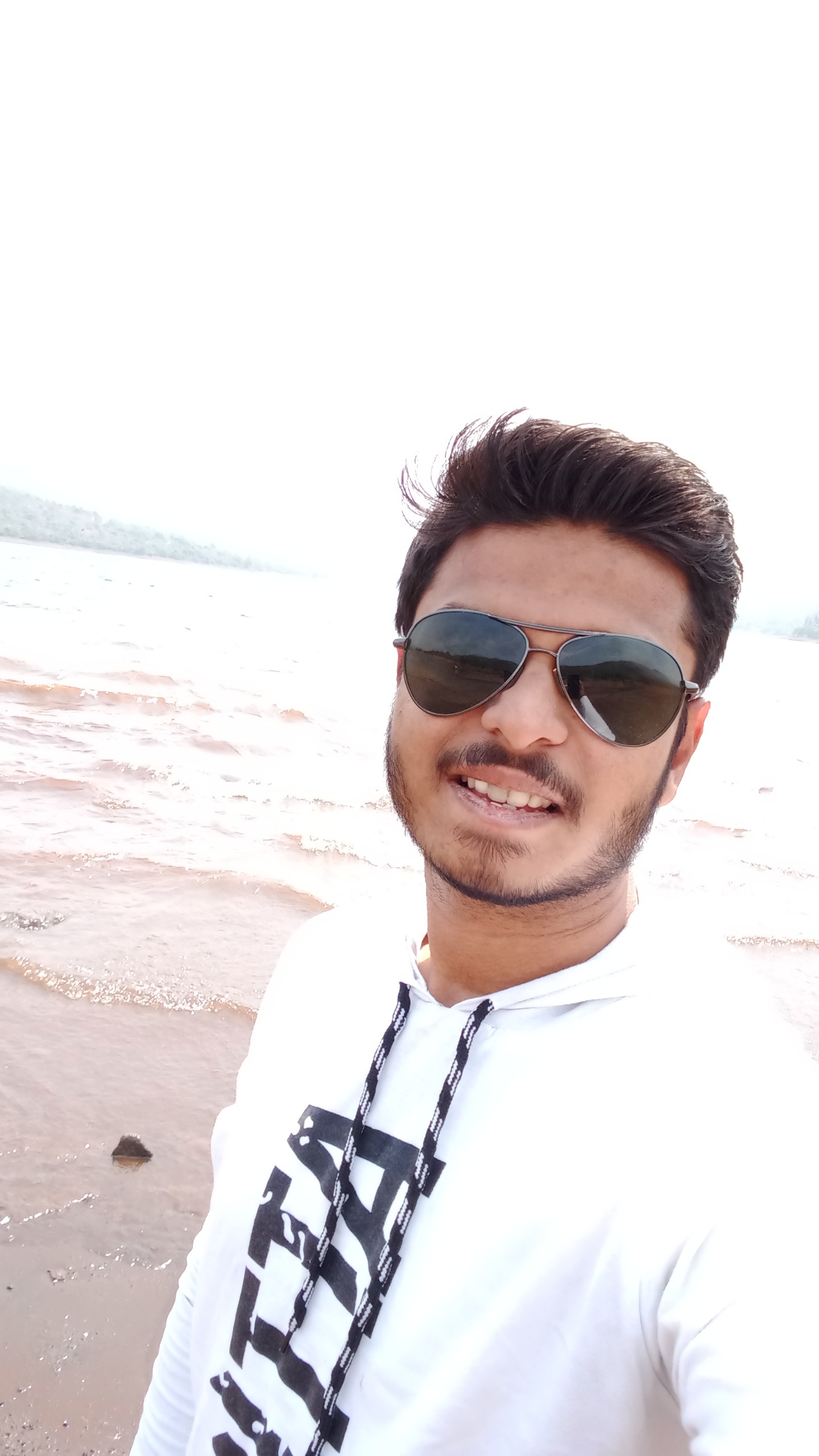
Shreeyog Gaikwad
Shreeyog Gaikwad
Passionate web developer with expertise in React, JavaScript and Node.js. Always eager to learn and share knowledge about modern web technologies. Join me on my journey of coding adventures and innovative web solutions!