AI-Powered Development: How to Feed Your Entire Project to Claude.ai in One Script
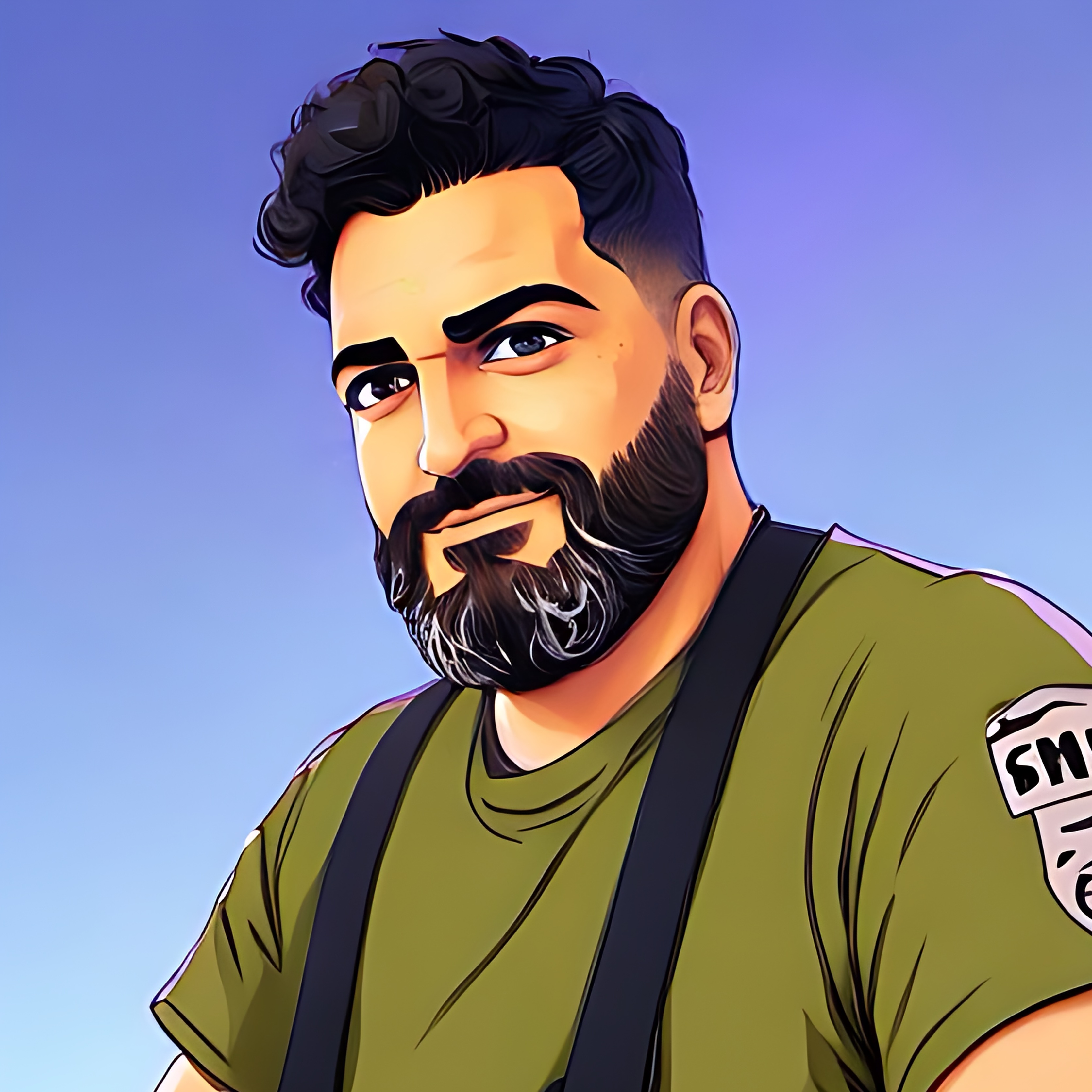
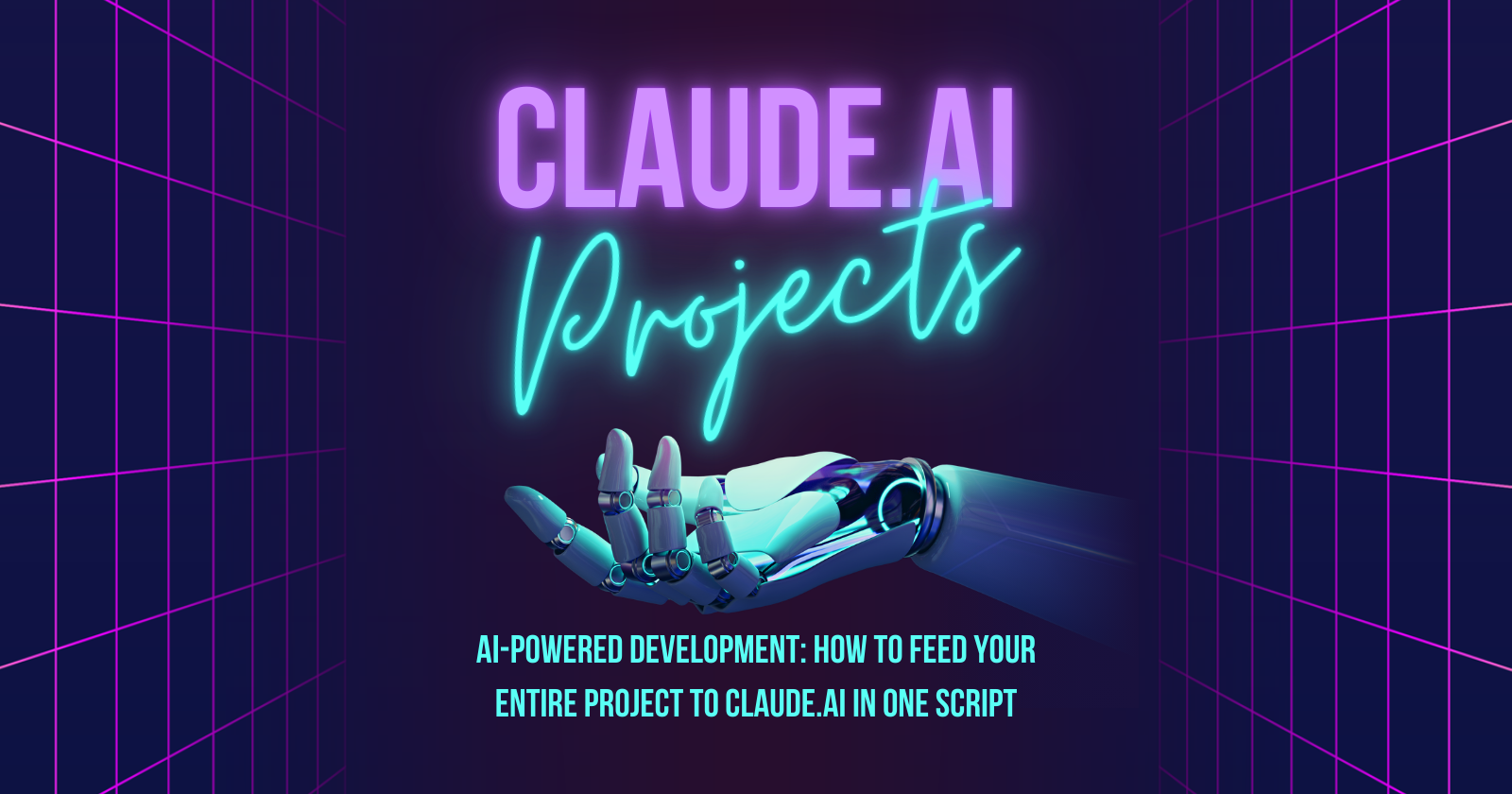
Introduction
Unless you've been coding in a cave for the past few weeks, you've probably heard about Claude.ai's new Projects feature. This game-changer allows you to add your project code into Claude.ai, creating a shared context for more accurate and personalized AI assistance. As a developer and AI enthusiast, I've been waiting for this kind of feature for ages. Nothing else currently comes close to this implementation in terms of ease of use and user experience.
The Challenge: Text File Limitations
Here's the catch: currently, you can only upload text files (pdf, docx, csv, txt, html, odt, rtf, or epub) to the project context. But hey, we're developers, right? Our whole job is creatively solving problems. (Am I right, or am I right?)
My Solution: A Custom Bash Script
In this article, I'll show you how to add your entire project into Claude.ai's context using a custom script. I'll break down a workflow I've been refining recently that's made my life so much easier. So, let's dive in! (For the TL;DR crowd, I've got a link to a repo with ready-to-use scripts for various frameworks Here. For those itching to copy-paste, your time will come. Stick with me for a few more paragraphs.)
The Power of Project Context in Claude
Before we get into the nitty-gritty, let's talk about why this is so awesome:
Personalized Responses: Claude.ai gets your project's quirks, from structure to naming conventions.
Code-Aware Suggestions: No more generic advice - get recommendations that actually fit your codebase.
Faster Problem Solving: With the full picture, Claude.ai becomes your personal code detective.
Learning Curve Reduction: New team members can get up to speed faster than you can say "onboarding". (And if you're on the Teams plan, you can share projects and enjoy a beefier context limit.)
Consistent Documentation: Keep your docs in check across the project without breaking a sweat.
Real-World Impact: A Developer's Tale
Let me paint you a picture. I'm leading a project at work using Astro with Svelte for dynamic components, TypeScript for that sweet type safety, and a custom Tailwind CSS color palette that our designers swear by. Oh, and let's not forget NanoStores for state management, because why make life easy, right?
The other day, I was knee-deep in a complex component that needed to play nice with our state management. Pre-Claude, I would've spent few hours jumping between files.
Post-Claude? I just asked, "How do I integrate this UserProfile component with our nanostores setup?" Boom! Claude spit out a code snippet that not only worked but also matched our project's naming conventions and even suggested a performance tweak.
What would've been a half-day headache turned into a 15-minute fix. And the best part? The solution was so good, I almost convinced myself I wrote it.
Script Breakdown
Now, let's break down this script:
- Creating the Copy Directory
mkdir -p copy
This creates a copy
directory. Groundbreaking stuff, I know.
- File Copying Function
copy_file() {
local src="$1"
local filename=$(basename "$src")
local dest="copy/${filename}.txt"
counter=1
while [ -e "$dest" ]; do
dest="copy/${filename}_${counter}.txt"
((counter++))
done
cp "$src" "$dest"
echo "Copied: $src to $dest"
}
export -f copy_file
This function copies files to our new directory, adding a .txt
extension. It's like a file photocopier, but fancier.
- Ignoring Specific Files and Directories
IGNORE_FILES=".DS_Store|\.env|pnpm-lock.yaml"
IGNORE_DIRS="node_modules|dist|\.git"
export IGNORE_FILES IGNORE_DIRS
Because some files should stay in Vegas... I mean, your local machine.
- Copying Root Directory Files
find . -maxdepth 1 -type f \( -name ".gitignore" -o -name "tailwind.config.js" -o -name "tsconfig.json" -o -name ".prettierrc" -o -name "next.config.js" -o -name "package.json" -o -name "README.md" \) -exec bash -c 'copy_file "$0"' {} \;
This grabs important config files from your root directory.
- Copying Files from Specific Directories
find app components lib layouts components types -type f \( -name "*.js" -o -name "*.ts" -o -name "*.jsx" -o -name "*.tsx" -o -name "*.json" -o -name "*.md" \) -not -path "*/node_modules/*" -not -path "dist" -not -path "*/.git/*" -exec bash -c 'copy_file "$0"' {} \;
This is where the magic happens. It copies all your project files, excluding the stuff we don't need.
- Generating Project Structure
tree -a -I "${IGNORE_DIRS}|${IGNORE_FILES}|copy" --dirsfirst > copy/MY_PROJECT_STRUCTURE.txt
This creates a neat tree structure of your project. It's like a map for your code jungle. The Project Structure file would look something like this:
.
├── public
│ ├── fonts
│ │ ├── Satoshi-Variable.woff
│ │ ├── Satoshi-Variable.woff2
│ │ ├── Satoshi-VariableItalic.woff
│ │ ├── Satoshi-VariableItalic.woff2
│ │ └── fonts.css
│ ├── apple-touch-icon.png
│ ├── favicon-32x32.png
│ ├── favicon-48x48.png
│ ├── favicon.svg
├── src
│ ├── components
│ │ ├── astro
│ │ │ ├── AuthInitializer.astro
│ │ │ ├── ButtonLink.astro
│ │ │ ├── Carousel.astro
│ │ │ ├── Chip.astro
│ │ │ ├── Faqs.astro
...
# Rest of the folder...
- Completion Messages
echo "Files have been copied to the 'copy' directory with .txt extension added."
echo "Project structure has been saved to copy/MY_PROJECT_STRUCTURE.txt"
Because who doesn't love a little confirmation?
After the script will finish running you should have a copy
folder in your project folder looking something like this:
The Full Script
Here's the complete script in all its glory:
mkdir -p copy
copy_file() {
local src="$1"
local filename=$(basename "$src")
local dest="copy/${filename}.txt"
counter=1
while [ -e "$dest" ]; do
dest="copy/${filename}_${counter}.txt"
((counter++))
done
cp "$src" "$dest"
echo "Copied: $src to $dest"
}
export -f copy_file
IGNORE_FILES=".DS_Store|\.env|pnpm-lock.yaml"
IGNORE_DIRS="node_modules|dist|\.git"
export IGNORE_FILES IGNORE_DIRS
find . -maxdepth 1 -type f \( -name ".gitignore" -o -name "tailwind.config.js" -o -name "tsconfig.json" -o -name ".prettierrc" -o -name "next.config.js" -o -name "package.json" -o -name "README.md" \) -exec bash -c 'copy_file "$0"' {} \;
find app components lib layouts components types -type f \( -name "*.js" -o -name "*.ts" -o -name "*.jsx" -o -name "*.tsx" -o -name "*.json" -o -name "*.md" \) -not -path "*/node_modules/*" -not -path "dist" -not -path "*/.git/*" -exec bash -c 'copy_file "$0"' {} \;
echo "Files have been copied to the 'copy' directory with .txt extension added."
tree -a -I "${IGNORE_DIRS}|${IGNORE_FILES}|copy" --dirsfirst > copy/MY_PROJECT_STRUCTURE.txt
echo "Project structure has been saved to copy/MY_PROJECT_STRUCTURE.txt"
Adapting the Script for Popular Frameworks
Different strokes for different folks, right? Here's how you can tweak the script for various frameworks:
For Astro Projects
# Add this to the file copying section
find src pages public -type f \( -name "*.astro" -o -name "*.md" -o -name "*.js" -o -name "*.ts" \) -not -path "*/node_modules/*" -exec bash -c 'copy_file "$0"' {} \;
For SvelteKit Projects
# Add this to the file copying section
find src routes static -type f \( -name "*.svelte" -o -name "*.js" -o -name "*.ts" \) -not -path "*/node_modules/*" -exec bash -c 'copy_file "$0"' {} \;
For Next.js Projects
# Add this to the file copying section
find pages api components styles -type f \( -name "*.js" -o -name "*.jsx" -o -name "*.ts" -o -name "*.tsx" -o -name "*.css" \) -not -path "*/node_modules/*" -exec bash -c 'copy_file "$0"' {} \;
For Nuxt.js Projects
# Add this to the file copying section
find pages components layouts store -type f \( -name "*.vue" -o -name "*.js" -o -name "*.ts" \) -not -path "*/node_modules/*" -exec bash -c 'copy_file "$0"' {} \;
For Python Projects
# Add this to the file copying section
find . -type f \( -name "*.py" -o -name "*.ipynb" \) -not -path "*/venv/*" -not -path "*/.*" -exec bash -c 'copy_file "$0"' {} \;
Remember to adjust the IGNORE_DIRS
variable to include framework-specific directories you want to exclude, like .next
, .nuxt
, or __pycache__
.
How to Use the Script
Save the script to your project root directory.
Run it:
bash script_name.sh
Upload the contents of the
copy
directory to your Claude.ai project.Get yourself some nice cup of coffee
Start chatting with your new, project-aware AI buddy!
[!TIP] be careful not to include sensitive information (like API keys or passwords) when uploading your project files to Claude.ai.(or anywhere)
A Quick Note on the "tree" Command
our script uses the "tree" command to generate a project structure file. If you're on a Mac and don't have it installed, you can easily get it with Homebrew: just run brew install tree
. Windows users, you're in luck – it's built-in for you. No "tree"? No worries! The script will still convert your code files just fine, but you'll miss out on that nifty project structure file that gives Claude some extra context. It's like the cherry on top of your code sundae – nice to have, but not essential.
Conclusion
I've been using Claude.ai's project feature for a few weeks now, and let me tell you, it's a game-changer. It's like having a brilliant developer that has an encyclopedic knowledge of your project.
I hope this article helps you supercharge your AI-assisted development. If you have any questions or want to share your experience, drop a comment below. I'm excited to see what the amazing team at Anthropic comes up with next!
Happy coding, and may your bugs be ever in your favor!
P.S. You can find the repository with the basic script and sample premade ones for Astro, Next.js, and Nuxt Here.
Happy coding!
Subscribe to my newsletter
Read articles from Meir-Josef Cohen directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
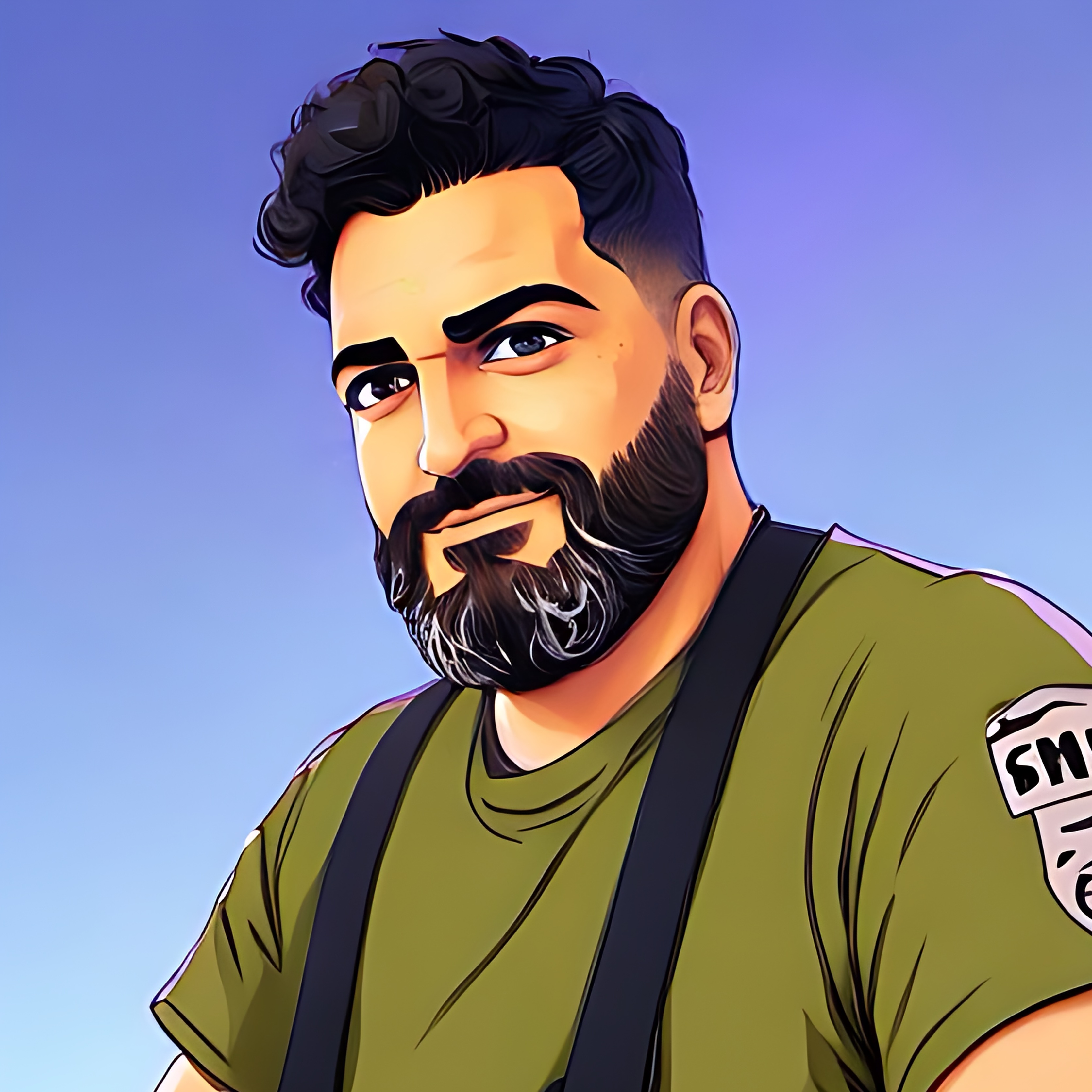
Meir-Josef Cohen
Meir-Josef Cohen
Hey there! I'm Meir J Cohen, a full-stack web developer with a passion for AI integration. With 2+ years of experience, I'm all about crafting efficient and innovative web solutions. My toolbox? Astro, Svelte, React, NextJS, JavaScript, TypeScript, and more. Currently, I'm diving deep into AI features for web apps. By day, I build end-to-end projects in Israel. By night, I'm exploring the AI frontier with a US-based company. I'm here to share insights, tips, and a bit of coding enthusiasm. Let's learn and grow together in this ever-evolving tech landscape! Got questions or just want to chat code? Drop me a line!