An Introduction to Activity Lifecycle in Android
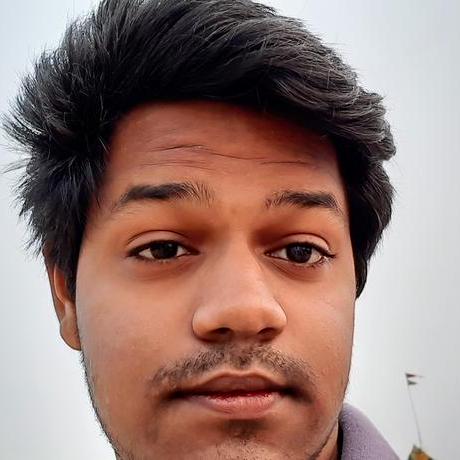
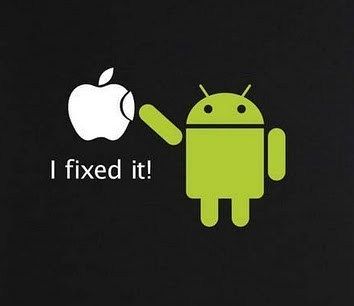
"The future of mobile is Android, and Android is the future." - Hiroshi Lockheimer, Former Senior Vice President of Android, Chrome OS & Play at Google
Introduction
Let us Imagine your phone as a stage with different apps like mini-plays. The Activity class is the stage manager, whispering to each play when things change. This lets apps pause videos (like lowering curtains) when you switch and pick up right where you left off! It keeps things running smoothly, avoiding crashes and remembering progress, for a much better app experience!
Working Concepts
Our phone's apps, like actors in a play, go through different stages managed by the Activity class. Here's how it keeps things running smoothly:
Opening an App (The Play Begins): When we open an app, the Activity class calls onCreate() to set everything up, just like preparing the stage for a play.
Switching Apps (Lowering the Curtains): As we switch apps, the Activity class helps the current app gracefully exit the stage. Here's what happens:
onPause(): The app pauses any ongoing tasks, like pausing a video.
onStop(): The app is mostly hidden, but it can still reside in memory in case we return to it quickly.
Coming Back to an App (Resuming the Play): When we return to the app, the Activity class helps it pick up right where we left off:
onStart(): The app starts preparing to come back into the foreground.
onResume(): The app resumes its tasks, like restarting the paused video.
Background Apps (The Understudies): There are limits on what background apps can do to conserve resources, but the Activity class helps them run efficiently.
Closing an App (The Final Curtain): If we completely close an app, the Activity class calls onDestroy() to release all resources associated with the app.
Lifecycle Methods (The Stage Manager's Cues): We don't necessarily need to use all the lifecycle methods provided by the Activity class, but understanding them helps our app function as users expect. Here's a reminder of the core methods:
onCreate()
onStart()
onResume()
onPause()
onStop()
onDestroy()
onCreate():
Act I: Opening the App - onCreate()
The user taps on your app icon, and the curtain rises! Behind the scenes, the Android system calls the onCreate() method, the opening act for your app's performance. This is where you set the stage and initialize everything your app needs to function.
Here's the code snippet for calling onCreate()
and using Logcat to confirm its execution:
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// Your app's initialization code goes here
// Logcat message to verify onCreate() call
Log.d("MyApp", "onCreate called!");
}
Logcat writes a debug message ("onCreate called!") with the tag "MyApp" to Logcat. This helps you verify that onCreate()
is executing as expected during development and testing. Seeing this message in Logcat confirms the curtain has risen for your app's performance!
onStart()
Act II (Part 1): The App Gains Focus - onStart()
As users navigate their phones, apps are constantly entering and exiting the foreground. When your app is pushed to the background but has the potential to return quickly (like when minimized), the system calls onStart(). This method signifies the app is no longer completely hidden, but it's not yet fully in the foreground either.
Here's the code snippet for calling onStart()
:
@Override
protected void onStart() {
super.onStart();
// Code to prepare for app visibility
Log.d("MyApp", "onStart called!");
// Logcat for verifying
}
This will write a debug message ("onStart called!") with the tag "MyApp" to Logcat, helping you track the lifecycle flow of your app.
onResume()
Act II (Part 2): Taking Center Stage - onResume()
As users navigate between apps, the one in focus takes center stage. The Android system calls onResume() to signal that your app has regained full control of the foreground. This method is like the final curtain rising, indicating your app is ready for user interaction.
Here's the code snippet for calling onResume()
and using Logcat to confirm its execution:
@Override
protected void onResume() {
super.onResume();
// Code to resume app functionality
// Logcat message to verify onResume() call
Log.d("MyApp", "onResume called!");
}
The included Log.d("MyApp", "onResume called!")
line writes a debug message ("onResume called!") with the tag "MyApp" to Logcat. Seeing this message confirms your app has successfully resumed and is ready for user interaction.
onPause()
Act II (Part 1): Stepping Backstage - onPause()
As users navigate between apps, the one in focus takes center stage. But when another app demands attention, yours gets relegated to the background. The Android system calls onPause() to signal this shift. It's like the stage lights dimming slightly, indicating a pause in the action for your app.
Here's the code snippet for calling onPause()
and using Logcat to confirm its execution:
@Override
protected void onPause() {
super.onPause();
// Code to pause app functionality
// Logcat message to verify onPause() call
Log.d("MyApp", "onPause called!");
}
The included Log.d("MyApp", "onPause called!")
line writes a debug message ("onPause called!") with the tag "MyApp" to Logcat. Seeing this message confirms your app has been paused and is ready to transition to the background.
onStop():
Act II (Part 2): Fading to Black - onStop()
As users juggle multiple apps, some inevitably get pushed completely off-screen. The Android system calls onStop() to signal this transition, where your app becomes almost invisible. Imagine the stage lights fading to near darkness, indicating the end of the current scene.
Here's the code snippet for calling onStop()
and using Logcat to confirm its execution:
@Override
protected void onStop() {
super.onStop();
// Code to release temporary resources
// Logcat message to verify onStop() call
Log.d("MyApp", "onStop called!");
}
The included Log.d("MyApp", "onStop called!")
line writes a debug message ("onStop called!") with the tag "MyApp" to Logcat. Seeing this message confirms your app has transitioned to a mostly hidden state and resources have been optimized.
onDestroy:
Act II (Part 3): The Final Curtain Call - onDestroy()
As users navigate their phones, apps transition through various states. Sometimes, an app might be completely shut down by the user or the system. The Android system calls onDestroy()` as the final farewell to your activity's lifecycle. Imagine the stage lights going completely dark, signifying the end of the play.
Here's the code snippet for calling onDestroy()
and using Logcat to confirm its execution:
@Override
protected void onDestroy() {
super.onDestroy();
// Code to clean up resources
// Logcat message to verify onDestroy() call
Log.d("MyApp", "onDestroy called!");
}
The included Log.d("MyApp", "onDestroy called!")
line writes a debug message ("onDestroy called!") with the tag "MyApp" to Logcat. Seeing this message confirms your activity has been completely destroyed and its resources have been released.
onRestart() [Optional]
There isn't a direct lifecycle method called onRestart
in the standard Android Activity lifecycle. However, there are ways to handle situations where an app might appear to "restart" and you want to take specific actions. Here are two possibilities:
Recreating the Activity:
If your app is completely destroyed by the system (e.g., due to low memory) and then relaunched by the user, the system will call
onCreate()
again to recreate the activity from scratch.You can use
onCreate()
to re-initialize your activity's state and data as needed.
System Configuration Changes:
Sometimes, the system configuration might change while your app is running in the background (e.g., screen orientation change, language change). In such cases, the system might destroy and recreate your activity to adapt to the new configuration.
The system calls
onConfigurationChanged(Configuration newConfig)
before recreating the activity. You can use this method to handle configuration changes and potentially update the UI accordingly.
Summary
The Need for Optimized Activity Management in Android Apps
The Android Activity lifecycle methods, from onCreate()
to onDestroy()
, are essential for managing your app's resources effectively. By understanding and implementing these methods, you can ensure your app uses resources efficiently throughout its lifecycle, leading to a better user experience.
Here's why optimized activity management is crucial for Android apps:
Limited Resources: Mobile devices have limited memory and processing power compared to computers. Inefficient resource usage can lead to lag, crashes, and a poor user experience. Optimized activity management helps your app function smoothly within these limitations.
Battery Efficiency: Unnecessary resource consumption can drain a user's battery quickly. By releasing resources when your app is not actively in use, you can significantly improve battery life.
Improved Performance: Efficient resource management ensures your app runs smoothly and responds quickly to user interactions. Users are more likely to enjoy using an app that performs well.
Memory Leaks Prevention: If resources are not properly released when an activity is paused or destroyed, it can lead to memory leaks. These leaks can cause performance issues and instability in the long run. Optimized activity management helps prevent memory leaks by ensuring proper resource cleanup.
Following the activity lifecycle methods allows you to:
Targeted Initialization (onCreate()): Allocate only the resources essential for your app's initial launch. This avoids unnecessary resource consumption right from the start.
Optimizing for Background States (onPause() and onStop()): When your app transitions to the background, release temporary resources that aren't critical while hidden. This conserves battery and processing power.
Clean Up (onDestroy()): When your app is completely shut down, perform a thorough cleanup by releasing all resources. This ensures your app exits gracefully and avoids memory leaks.
By effectively managing your app's activities throughout their lifecycle, you can create a more efficient, performant, and user-friendly experience on Android devices.
References:
I hope you Enjoyed reading this small blog on the basics of activity. There are tons of layers beneath this which can be dived deep into so lets continue learning and uncovering the secrets of how this magnificient tech works around us to give us the fruitful life we have today.
If you have read till the end, please checkout and follow my social handles and connect as it would mean a lot : ) , See Yaa
Linkedin:- Arpan Padhi
Twitter:-Beingtechsavy
Github:- Beingtechsavy
Hashnode:- beingtechsavy.hashnode.dev
Subscribe to my newsletter
Read articles from Arpan Padhi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
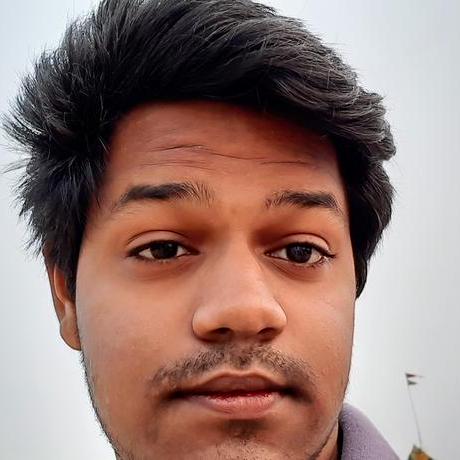
Arpan Padhi
Arpan Padhi
Android Developer • MLSA- Alpha • Podcasting at Cogent Labs • Ex-CCO • Founder @Tech & Talk