Implementing User Registration in ASP.NET Core with Identity
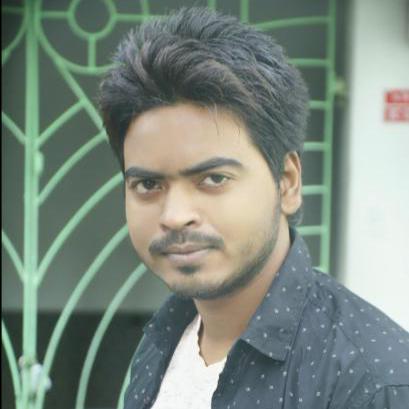
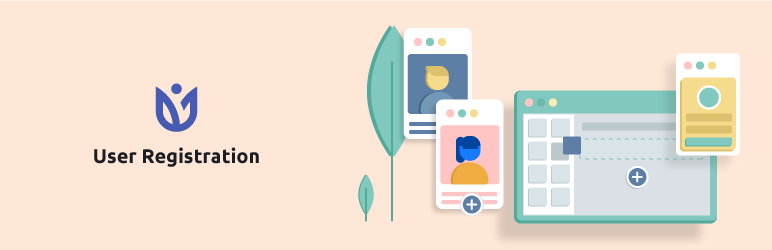
In this post, we will explore how to implement user registration in an ASP.NET Core application using Identity. The provided code snippet demonstrates a controller that handles user registration, including role assignment.
Code Explanation
The AuthController
class is responsible for managing authentication-related actions. Here's a breakdown of the key components:
Dependencies: The controller depends on
UserManager<ApplicationUser>
,RoleManager<IdentityRole>
, and a secret key from the configuration.Constructor: The constructor initializes these dependencies.
Register Endpoint: The
Register
method handles user registration. It performs the following steps:Checks if the username already exists.
Creates a new user if the username is available.
Assigns roles to the user based on the provided role in the registration request.
Code
namespace RedMango_API.Controllers
{
[Route("api/auth")]
[ApiController]
public class AuthController : ControllerBase
{
private readonly UserManager<ApplicationUser> _userManager;
private readonly RoleManager<IdentityRole> _roleManager;
private readonly string _secretKey;
public AuthController(IConfiguration configuration,
UserManager<ApplicationUser> userManager, RoleManager<IdentityRole> roleManager)
{
_secretKey = configuration.GetValue<string>("ApiSettings:Secret");
_userManager = userManager;
_roleManager = roleManager;
}
[HttpPost("register")]
public async Task<IActionResult> Register([FromBody] RegisterRequestDTO model)
{
var userFromDb = await _userManager.FindByNameAsync(model.UserName.ToLower());
if (userFromDb != null)
{
return BadRequest(new { StatusCode = HttpStatusCode.BadRequest, IsSuccess = false, ErrorMessages = new[] { "Username already exists" } });
}
var newUser = new ApplicationUser
{
UserName = model.UserName,
Email = model.UserName,
NormalizedEmail = model.UserName.ToUpper(),
Name = model.Name
};
var result = await _userManager.CreateAsync(newUser, model.Password);
if (result.Succeeded)
{
if (!await _roleManager.RoleExistsAsync(SD.Role_Admin))
{
await _roleManager.CreateAsync(new IdentityRole(SD.Role_Admin));
await _roleManager.CreateAsync(new IdentityRole(SD.Role_Customer));
}
if (model.Role.ToLower() == SD.Role_Admin)
{
await _userManager.AddToRoleAsync(newUser, SD.Role_Admin);
}
else
{
await _userManager.AddToRoleAsync(newUser, SD.Role_Customer);
}
return Ok(new { StatusCode = HttpStatusCode.OK, IsSuccess = true });
}
return BadRequest(new { StatusCode = HttpStatusCode.BadRequest, IsSuccess = false, ErrorMessages = new[] { "Error while registering" } });
}
}
}
Hashtags
#ASPNetCore #Identity #UserRegistration #WebDevelopment #DotNet
Explanation
The AuthController
class is designed to handle user registration in an ASP.NET Core application. It uses ASP.NET Core Identity to manage user information and roles. The Register
method checks if a user already exists, creates a new user if not, and assigns the appropriate role based on the registration request. This setup ensures that user management is handled securely and efficiently within the application.
Subscribe to my newsletter
Read articles from Md Asif Alam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
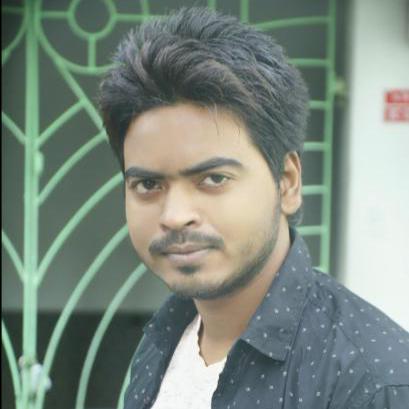
Md Asif Alam
Md Asif Alam
๐ Full Stack .NET Developer & React Enthusiast ๐จโ๐ป About Me: With 3+ years of experience, I'm passionate about crafting robust solutions and seamless user experiences through code. ๐ผ Expertise: Proficient in .NET Core API, ASP.NET MVC, React.js, and SQL. Skilled in backend architecture, RESTful APIs, and frontend development. ๐ Achievements: Led projects enhancing scalability by 50%, delivered ahead of schedule, and contributed to open-source initiatives. ๐ Future Focus: Eager to embrace new technologies and drive innovation in software development. ๐ซ Let's Connect: Open to new opportunities and collaborations. Reach me on LinkedIn or GitHub!