Star Patterns and Basic Logic Questions in PHP

Table of contents
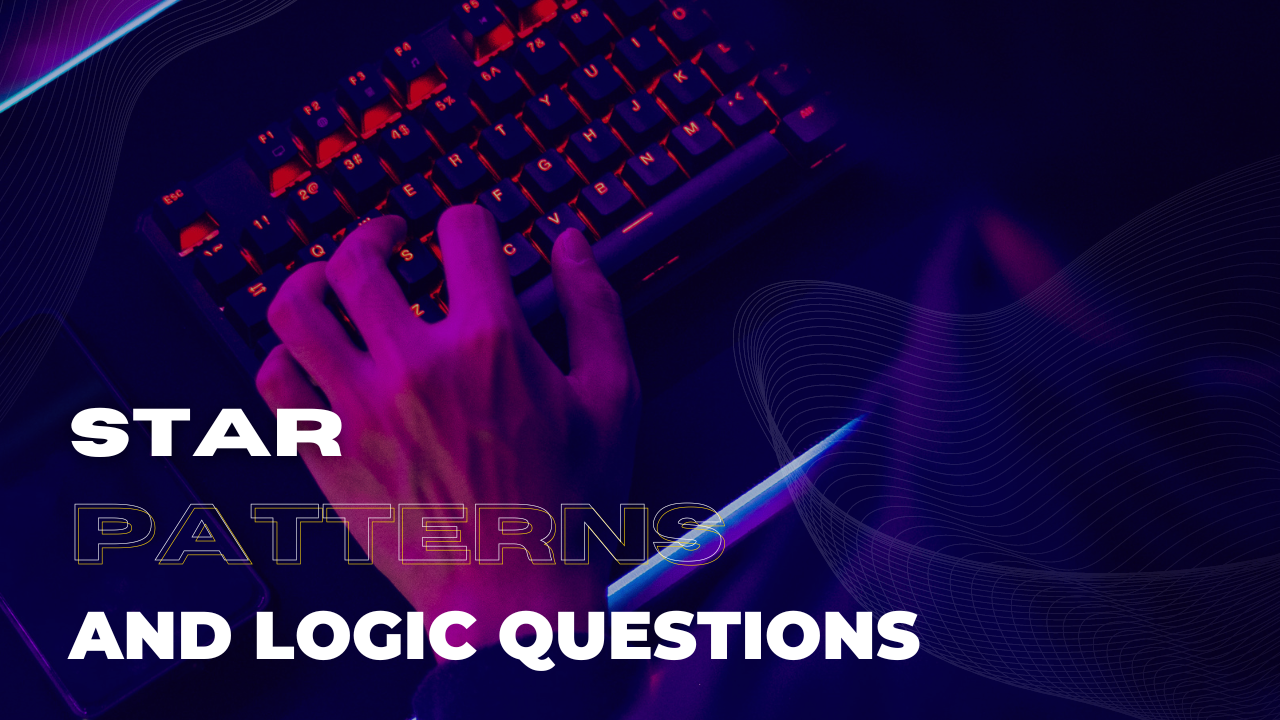
Welcome to a special blog post where we delve into some interesting PHP challenges: star patterns and basic logic questions. These exercises are great for honing your programming skills and understanding loops, and conditionals in PHP.
Star Patterns
Star patterns are a common exercise in programming that help you understand nested loops. Here are some examples:
Right-Angled Triangle:
<?php $n = 5; // Number of rows for ($i = 1; $i <= $n; $i++) { for ($j = 1; $j <= $i; $j++) { echo "* "; } echo "<br>"; } // Outputs: // * // * * // * * * // * * * * // * * * * * ?>
Inverted Right-Angled Triangle:
<?php $n = 5; for ($i = $n; $i >= 1; $i--) { for ($j = 1; $j <= $i; $j++) { echo "* "; } echo "<br>"; } // Outputs: // * * * * * // * * * * // * * * // * * // * ?>
Left-Angled Triangle:
<?php $n = 5; for ($i = 1; $i <= $n; $i++) { for ($j = $i; $j < $n; $j++) { echo " "; } for ($j = 1; $j <= $i; $j++) { echo "* "; } echo "<br>"; } // Output: // * // * * // * * * // * * * * // * * * * * ?>
Inverted Left-Angled Triangle:
<?php $n = 5; for ($i = $n; $i >= 1; $i--) { for ($j = $i; $j < $n; $j++) { echo " "; } for ($j = 1; $j <= $i; $j++) { echo "* "; } echo "<br>"; } // Output: // * * * * * // * * * * // * * * // * * // * ?>
Pyramid:
<?php $n = 5; for ($i = 1; $i <= $n; $i++) { for ($j = $i; $j < $n; $j++) { echo " "; // Two spaces } for ($j = 1; $j <= (2 * $i - 1); $j++) { echo "* "; } echo "<br>"; } // Outputs: // * // * * * // * * * * * // * * * * * * * // * * * * * * * * * ?>
Diamond:
<?php $n = 5; for ($i = 1; $i <= $n; $i++) { for ($j = $i; $j < $n; $j++) { echo " "; } for ($j = 1; $j <= (2 * $i - 1); $j++) { echo "* "; } echo "<br>"; } for ($i = $n-1; $i >= 1; $i--) { for ($j = $n; $j > $i; $j--) { echo " "; } for ($j = 1; $j <= (2 * $i - 1); $j++) { echo "* "; } echo "<br>"; } // Outputs: // * // * * * // * * * * * // * * * * * * * // * * * * * * * * * // * * * * * * * // * * * * * // * * * // * ?>
Sandglass Pattern:
<?php $n = 5; // Upper part for ($i = $n; $i >= 1; $i--) { for ($j = $i; $j < $n; $j++) { echo " "; } for ($j = 1; $j <= (2 * $i - 1); $j++) { echo "* "; } echo "<br>"; } // Lower part for ($i = 2; $i <= $n; $i++) { for ($j = $i; $j < $n; $j++) { echo " "; } for ($j = 1; $j <= (2 * $i - 1); $j++) { echo "* "; } echo "<br>"; } // Output: // * * * * * * * * * // * * * * * * * // * * * * * // * * * // * // * * * // * * * * * // * * * * * * * // * * * * * * * * * ?>
Basic Logic Questions
- Swapping of Two Variables:
<?php
$a = 5;
$b = 10;
// Swapping without using a third variable
echo "Before swapping: a = $a, b = $b<br>";
$a = $a + $b; // a becomes 15
$b = $a - $b; // b becomes 5 (15 - 10)
$a = $a - $b; // a becomes 10 (15 - 5)
echo "After swapping: a = $a, b = $b<br>";
?>
- Find the Largest Number Among Three Numbers:
<?php
$num1 = 10;
$num2 = 20;
$num3 = 30;
if ($num1 >= $num2 && $num1 >= $num3) {
echo "$num1 is the largest number.";
} elseif ($num2 >= $num1 && $num2 >= $num3) {
echo "$num2 is the largest number.";
} else {
echo "$num3 is the largest number.";
}
?>
- Check if a Number is a Leap Year or Not:
<?php
$year = 2024;
if (($year % 4 == 0 && $year % 100 != 0) || ($year % 400 == 0)) {
echo "$year is a leap year.";
} else {
echo "$year is not a leap year.";
}
?>
- Write a Program to Find the Area of a Circle:
<?php
$radius = 5;
$area = 3.14 * ($radius * $radius);
echo "The area of the circle with radius $radius is " . round($area, 2) . ".";
?>
Conclusion
In this blog post, we covered various star patterns and basic logic questions in PHP. These exercises help solidify your understanding of loops and conditionals, which are fundamental concepts in PHP programming. Try implementing these patterns and logic questions on your own to further enhance your coding skills.
Subscribe to my newsletter
Read articles from Saurabh Damale directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Saurabh Damale
Saurabh Damale
Hello there! ๐ I'm Saurabh Kailas Damale, a passionate Full Stack Developer with a strong background in creating dynamic and responsive web applications. I thrive on turning complex problems into elegant solutions, and I'm always eager to learn new technologies and tools to enhance my development skills.