How to Transpile JavaScript/TypeScript with Babel AST: A Guide for Functions and Classes

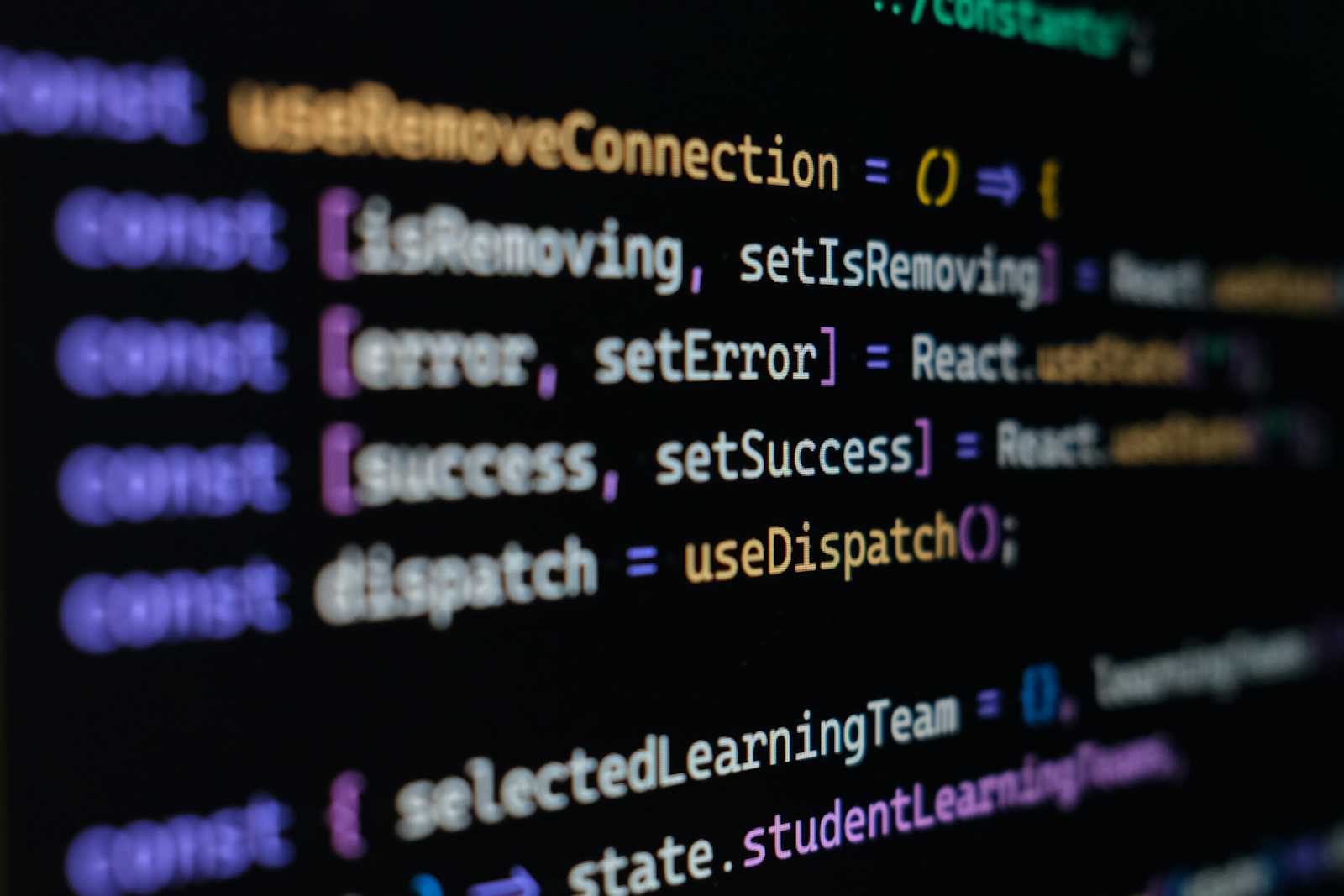
Mastering JavaScript/TypeScript Transpilation with Babel AST: From Functions to Classes
In the ever-evolving world of web development, keeping your codebase up-to-date with the latest patterns and practices is crucial. One common migration path is transitioning from function-based components to class-based components. This blog post will dive deep into using Babel's Abstract Syntax Tree (AST) to automate this process, focusing on transforming JavaScript functions containing JSX into ES6 classes.
For the full code implementation and more detailed examples, you can refer to the babel-plugin-transform-reblend-function-to-class repository on GitHub.
Understanding Babel and AST
What is Babel?
Babel is a powerful JavaScript compiler that transforms modern JavaScript code (ES6+) into backwards-compatible versions for older environments. It's an essential tool in many development workflows, enabling developers to use cutting-edge language features while maintaining broad browser compatibility.
What is an Abstract Syntax Tree (AST)?
An Abstract Syntax Tree is a tree representation of the abstract syntactic structure of source code. It's a fundamental concept in parsing and compiling programming languages. When Babel processes your code, it first converts it into an AST, manipulates this tree, and then generates new code from the modified AST.
Why Use Babel AST for Code Transformation?
Precision: ASTs allow for granular control over code structure, enabling precise transformations.
Scalability: You can apply transformations to large codebases consistently and efficiently.
Flexibility: ASTs can handle complex transformations that simple regex-based solutions cannot.
Safety: Working at the AST level reduces the risk of introducing syntax errors during transformation.
Setting Up Your Babel Environment
Before we dive into the transformation process, let's set up our development environment:
npm install --save-dev @babel/core @babel/preset-env @babel/plugin-syntax-jsx
For TypeScript support, add:
npm install --save-dev @babel/preset-typescript
The Transformation Process: From Functions to Classes
Let's break down the process of transforming a function component to a class component using our custom Babel plugin.
Example: Before and After
Input (Function Component):
import { useState } from 'reblendjs';
const MyComponent = () => {
const [count, setCount] = useState(0);
const handleClick = () => {
setCount(count + 1);
};
return <button onClick={handleClick}>Count: {count}</button>;
};
Output (Class Component):
class MyComponent extends Reblend {
static ELEMENT_NAME = "MyComponent";
constructor() {
super();
}
init() {
this.count = 0;
this.setCount = this.setCount.bind(this);
this.apply(this.setCount, "count");
this.handleClick = this.handleClick.bind(this);
}
html() {
return <button onClick={this.handleClick}>Count: {this.count}</button>;
}
}
Key Transformation Steps
Function to Class Conversion: Convert the function declaration to a class extending
Reblend
.State Management: Convert
useState
hooks to class-based state.Method Binding: Bind methods in the constructor to ensure correct
this
context.JSX Transformation: Update JSX to use class properties and methods.
Implementing the Babel Plugin
Here's the core of our Babel plugin, the replaceIdentifiers
function:
import * as t from "@babel/types";
import { NodePath } from "@babel/traverse";
type ReplaceIdentifiersOption = {
assignmentStatements: t.ExpressionStatement[];
props:
| (t.ObjectProperty | t.RestElement)[]
| (
| t.ArrayPattern
| t.AssignmentPattern
| t.Identifier
| t.RestElement
| t.TSParameterProperty
)[];
};
function replaceIdentifiers(
path: NodePath<t.Function>,
node: t.Node,
t: typeof import("@babel/types"),
{ assignmentStatements, props }: ReplaceIdentifiersOption
) {
// ... (rest of the function implementation)
}
export default replaceIdentifiers;
For the complete implementation of this function and the full plugin code, please refer to the babel-plugin-transform-reblend-function-to-class repository on GitHub.
Key Aspects of the Implementation
Type Safety: We're using TypeScript to ensure type safety throughout our plugin.
Visitor Pattern: The core of Babel plugins, allowing us to traverse and manipulate the AST.
Context Awareness: We carefully handle different contexts (e.g.,
this
expressions, assignments) to avoid incorrect transformations.Flexible Replacements: The plugin can handle both props and state, transforming them appropriately.
Advanced Features and Considerations
Hooks to Lifecycle Methods: Consider extending the plugin to convert React hooks to equivalent lifecycle methods.
Performance Optimization: For large codebases, implement caching mechanisms to speed up repeated transformations.
Error Handling: Add robust error handling to provide meaningful feedback when transformations fail.
Config Options: Allow users to customize the transformation behavior through plugin options.
Testing Your Babel Plugin
Thorough testing is crucial for a reliable Babel plugin. Consider implementing unit tests and integration tests to ensure your plugin works correctly across various scenarios.
Conclusion
Leveraging Babel's AST capabilities for code transformation opens up a world of possibilities for maintaining and evolving your codebase. This approach to migrating from function components to class components is just one example of how powerful and flexible Babel plugins can be.
By mastering these techniques, you can automate complex refactoring tasks, ensure consistency across large projects, and smoothly adopt new patterns and practices in your development workflow.
Remember, while automated tools like this are incredibly helpful, they're not infallible. Always review the transformed code and test thoroughly to ensure the desired behavior is maintained.
For more advanced use cases and the full implementation, don't forget to check out the babel-plugin-transform-reblend-function-to-class repository on GitHub.
Happy coding, and may your transformations be ever smooth!
Subscribe to my newsletter
Read articles from Emmanuel Paul Elom directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Emmanuel Paul Elom
Emmanuel Paul Elom
Experienced Full Stack Developer with expertise in JAVA, JavaScript, TypeScript, NodeJS, Rust, ExpressJS, and PHP. Passionate about open-source contributions and creating scalable solutions. Seeking a challenging role to leverage my skills in both front-end and back-end development to drive innovation and efficiency.