What is Flutter Secure Storage? Reasons to Use It and Best Practices
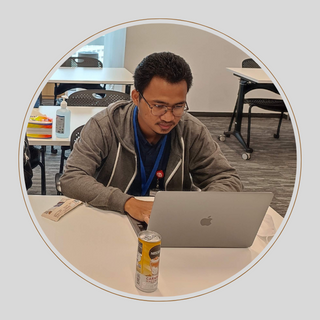
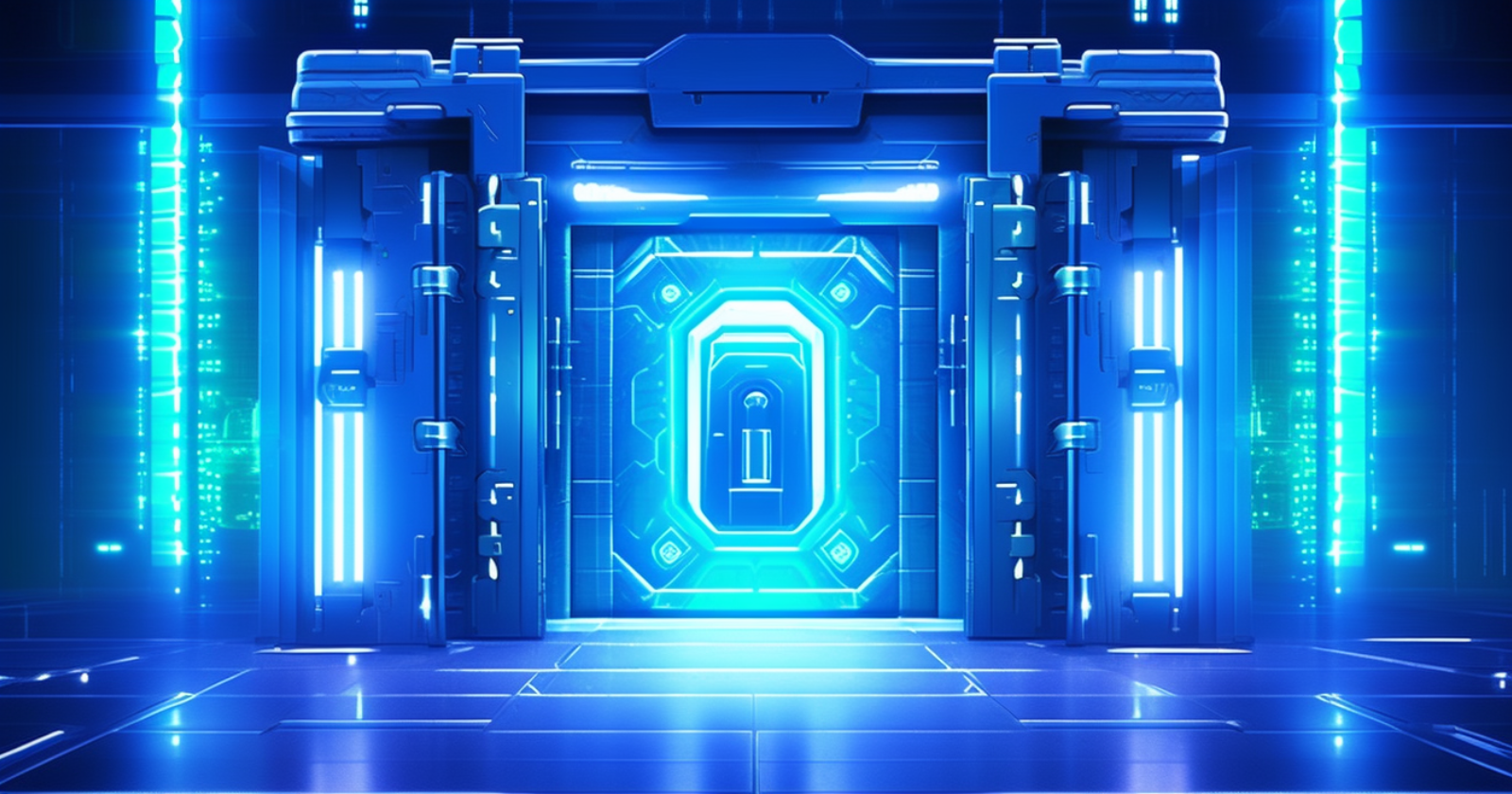
In mobile application development, securely storing sensitive data such as API keys, tokens, and user credentials is crucial. Flutter Secure Storage is a powerful package designed to securely store data in Flutter applications. This article will explore what Flutter Secure Storage is, the reasons why you should use it, how to install it, and best practices for its use.
What is Flutter Secure Storage?
Flutter Secure Storage is a Flutter package that provides a way to securely store key-value pairs on both iOS and Android. It leverages platform-specific APIs to ensure that the stored data is encrypted and protected from unauthorized access.
How Does Flutter Secure Storage Work?
iOS: Uses the Keychain services, which is a secure storage API provided by Apple to store sensitive data.
Android: Uses the Keystore system and EncryptedSharedPreferences to store data securely.
Reasons to Use Flutter Secure Storage
1. Enhanced Security
Flutter Secure Storage ensures that sensitive data is stored securely by encrypting it. This is critical for protecting user credentials, tokens, and other private information from being accessed by unauthorized parties.
2. Cross-Platform Support
The package provides a unified API for both iOS and Android, simplifying the development process. Developers can use the same codebase to handle secure storage on both platforms.
3. Ease of Use
Flutter Secure Storage offers a straightforward API for storing and retrieving key-value pairs, making it easy to integrate into Flutter applications. Its simplicity allows developers to focus on other aspects of the application without worrying about data security.
4. Compliance with Security Standards
Using platform-specific secure storage mechanisms ensures compliance with security standards and best practices. This is important for applications that need to adhere to regulatory requirements such as GDPR or HIPAA.
Use Cases for Flutter Secure Storage
1. Storing User Credentials
Securely storing user credentials (such as usernames and passwords) is essential for protecting user accounts. Flutter Secure Storage can be used to store these credentials securely and retrieve them when needed for authentication purposes.
2. Managing Session Tokens
Many applications use session tokens or API tokens to manage user sessions and authenticate API requests. Flutter Secure Storage ensures that these tokens are stored securely, preventing unauthorized access.
3. Storing Encryption Keys
If your application uses encryption for data protection, storing encryption keys securely is crucial. Flutter Secure Storage provides a safe place to store these keys, ensuring that encrypted data remains secure.
4. Storing User Preferences
While not as sensitive as credentials or tokens, user preferences can still benefit from secure storage. Flutter Secure Storage can be used to store settings and preferences that should not be easily accessible or modifiable by users or other apps.
How to Install Flutter Secure Storage
Step 1: Add Dependency
Add the flutter_secure_storage
package to your pubspec.yaml
file:
dependencies:
flutter:
sdk: flutter
flutter_secure_storage: ^9.2.2
Run flutter pub get
to install the package.
Step 2: Configure iOS
For iOS, you need to add the following permissions to your ios/Runner/Info.plist
file:
<key>NSFaceIDUsageDescription</key>
<string>We need access to your Face ID for secure authentication</string>
Step 3: Configure Android
For Android, ensure that your minSdkVersion
in android/app/build.gradle
is set to at least 18:
android {
...
defaultConfig {
...
minSdkVersion 18
...
}
...
}
Sample Code for Using Flutter Secure Storage
Here is a sample Flutter application demonstrating how to use Flutter Secure Storage to store and retrieve data securely.
Example Application
import 'package:flutter/material.dart';
import 'package:flutter_secure_storage/flutter_secure_storage.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Secure Storage Example',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: SecureStorageDemo(),
);
}
}
class SecureStorageDemo extends StatefulWidget {
@override
_SecureStorageDemoState createState() => _SecureStorageDemoState();
}
class _SecureStorageDemoState extends State<SecureStorageDemo> {
final _storage = FlutterSecureStorage();
final _keyController = TextEditingController();
final _valueController = TextEditingController();
String _storedValue = '';
Future<void> _saveData() async {
await _storage.write(key: _keyController.text, value: _valueController.text);
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(content: Text('Data saved successfully')),
);
}
Future<void> _readData() async {
String? value = await _storage.read(key: _keyController.text);
setState(() {
_storedValue = value ?? 'No value found';
});
}
Future<void> _deleteData() async {
await _storage.delete(key: _keyController.text);
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(content: Text('Data deleted successfully')),
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Flutter Secure Storage Example'),
),
body: Padding(
padding: EdgeInsets.all(16.0),
child: Column(
children: [
TextField(
controller: _keyController,
decoration: InputDecoration(labelText: 'Key'),
),
TextField(
controller: _valueController,
decoration: InputDecoration(labelText: 'Value'),
),
SizedBox(height: 20),
Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: [
ElevatedButton(
onPressed: _saveData,
child: Text('Save'),
),
ElevatedButton(
onPressed: _readData,
child: Text('Read'),
),
ElevatedButton(
onPressed: _deleteData,
child: Text('Delete'),
),
],
),
SizedBox(height: 20),
Text('Stored Value: $_storedValue'),
],
),
),
);
}
}
Best Practices for Using Flutter Secure Storage
1. Use Unique Keys
When storing data, always use unique keys to avoid conflicts and ensure that each piece of data can be retrieved correctly.
final storage = new FlutterSecureStorage();
await storage.write(key: 'api_token', value: 'your_api_token');
2. Handle Exceptions
Secure storage operations can fail for various reasons, such as missing permissions or platform-specific issues. Always handle exceptions to ensure that your application can respond gracefully.
dartCopy codetry {
await storage.write(key: 'api_token', value: 'your_api_token');
} catch (e) {
print('Error storing data: $e');
}
3. Avoid Storing Large Amounts of Data
Flutter Secure Storage is designed for storing small pieces of sensitive data. Avoid using it for large datasets or files. For larger data, consider using secure file storage methods.
4. Secure Your App Further
While Flutter Secure Storage provides encryption, it's essential to secure your application as a whole. Use additional security measures such as obfuscation, code signing, and secure network communications (e.g., HTTPS).
5. Regularly Update Dependencies
Keep your dependencies up-to-date to ensure that you benefit from the latest security patches and improvements. Regularly check for updates to the Flutter Secure Storage package and other related dependencies.
6. Test on All Supported Platforms
Ensure that your secure storage implementation works correctly on all supported platforms (iOS and Android). Test your application thoroughly to identify and resolve any platform-specific issues.
Conclusion
Flutter Secure Storage is a vital tool for securely storing sensitive data in Flutter applications. By leveraging platform-specific secure storage mechanisms, it ensures that data remains protected from unauthorized access. Whether you're storing user credentials, session tokens, encryption keys, or user preferences, Flutter Secure Storage provides a simple and effective solution.
By following best practices such as using unique keys, handling exceptions, and securing your application further, you can ensure that your sensitive data is stored safely. Integrate Flutter Secure Storage into your Flutter projects today to enhance security and protect user data.
Subscribe to my newsletter
Read articles from Anaz S. Aji directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
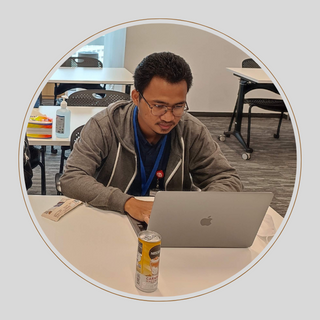
Anaz S. Aji
Anaz S. Aji
I am a dedicated Mobile Developer with a strong passion for exploring the latest advancements in technology and the dynamic world of cryptocurrency. My curiosity and enthusiasm drive me to continuously seek out and experiment with innovative solutions, ensuring that I stay at the forefront of industry trends and developments.