Implementing a game guide using a knowledge-based AI chatbot
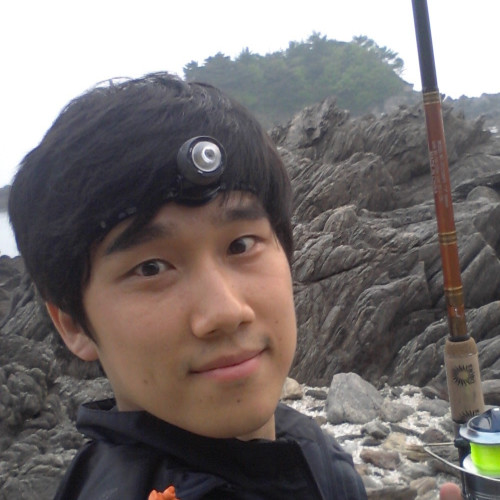
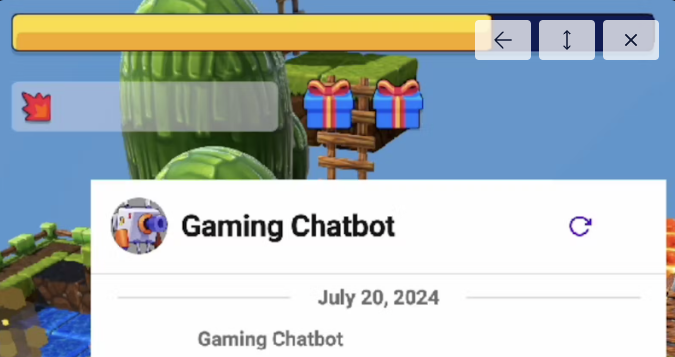
Since game systems are very complex, it is important to provide various tutorials and guides for the convenience of gamers. However, creating tutorials takes a lot of time, and it can be challenging to update them whenever the system changes.
I implemented a solution using a knowledge-based AI chatbot that allows gamers to easily access official information from the developers. This approach reduces the resources needed for developing tutorials by utilizing Unity WebView and Sendbird AI chatbot.
By providing the chatbot with appropriate information, it can answer questions like
How can I craft legendary items?
What is a good hunting ground for level 10?
What is the strongest sword in terms of attack power? Where can I obtain it?
How do I defeat the boss in stage 10?
With this AI chatbot, gamers can easily obtain the information they need at any time, greatly enhancing their gaming experience and immersion.
Preparation requirements
Unity 6
Creating a PDF file with game information
Entire process
Unity Project
Add an HTML file to connect the chatbot.
Add the WebView package to open the HTML file.
Add a MonoBehaviour script to open the WebView.
-
Access the dashboard, create an account, and create a new application.
Create an AI chatbot and upload the PDF file with game information.
Copy the Application ID and Bot ID from the dashboard and apply them to the HTML file.
Verify by playing or building in the Unity Editor.
Implementation
Add HTML file to Unity project
Add the
Chatbot.html
file to theAssets/StreamingAssets
folder and write the following<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>Chatbot</title> <script crossorigin src="https://unpkg.com/react@18.2.0/umd/react.development.js"></script> <script crossorigin src="https://unpkg.com/react-dom@18.2.0/umd/react-dom.development.js"></script> <script>process = { env: { NODE_ENV: '' } }</script> <script crossorigin src="https://unpkg.com/@sendbird/chat-ai-widget@latest/dist/index.umd.js"></script> <link href="https://unpkg.com/@sendbird/chat-ai-widget@latest/dist/style.css" rel="stylesheet" /> <script src="https://unpkg.com/@babel/standalone/babel.min.js"></script> <style> html,body { height:100% } #aichatbot-widget-close-icon { display: none } #scale-container { transform: scale(1); transform-origin: bottom right; height: 100%; zoom: 3; } </style> </head> <body> <div id="root"></div> <script type="text/babel"> const { ChatWindow } = window.ChatAiWidget const App = () => { <!-- Replace YOUR_APPLICATION_ID and YOUR_BOT_ID with the actual application ID and bot ID you intend to use. --> return ( <div id="scale-container"> <ChatWindow applicationId="YOUR_APPLICATION_ID" botId="YOUR_BOT_ID" /> </div> ) } ReactDOM.createRoot(document.querySelector('#root')).render(<App/>); </script> </body> </html>
Add Unity WebView Package
Click on
Install package from git URL…
in the top left menu of the Package Manager.Enter
https://github.com/gree/unity-webview.git?path=/dist/package
and click the Install button to install it.
Write MonoBehaviour Script to Open WebView
Add the
ChatbotWebView.cs
file to theAssets/Scripts
folder and write the script to load the HTML file when a button is clicked as followspublic class ChatbotWebView : UnityEngine.MonoBehaviour { private UnityEngine.UI.Button _openButton; private WebViewObject _webViewObject; private void Start() { _openButton = base.transform.GetComponent<UnityEngine.UI.Button>(); _openButton.onClick.AddListener(OnOpenChatbot); } private void OnOpenChatbot() { string strUrl = $"file://{UnityEngine.Application.streamingAssetsPath}/Chatbot.html"; _webViewObject = new UnityEngine.GameObject("ChatbotWebViewObject").AddComponent<WebViewObject>(); _webViewObject.Init(); _webViewObject.LoadURL(strUrl); _webViewObject.SetVisibility(true); _webViewObject.SetMargins(200, 400, 50, 400); } }
Create Button UI and Add ChatbotWebView Script
Create
Button
Add the
ChatbotWebView
component to the createdButton
.
Create a Sendbird App and AI chatbot (30-day free trial).
Create an Application.
In the left tab, click on AI chatbot -> Click the Get started button to create it.
If necessary, change the settings and click the Next button.
Upload the PDF file containing game information (example PDF)
Dungeon 1: Cave of Fire • Difficulty: Easy • Monster Types: • Flame Slime • HP: 100 • MP: 50 • Attack Power: 30 • Defense: 10 Dungeon 2: Fortress of Ice • Difficulty: Medium • Monster Types: • Ice Golem • HP: 300 • MP: 100 • Attack Power: 50 • Defense: 40 Ice Sword • Materials: • Ice Shard x3 (Obtained from: Ice Golem, Glacier Wolf) • Glacier Core x2 (Obtained from: Ice Spirit, Frost Witch) • Steel Fragment x1 (Obtained from: Dungeon Shop) • Combination Method: • Infuse the Ice Shards and Glacier Cores into the Steel Fragment, then cool and temper the mixture.
Creation is complete.
6. Copy the Application ID and Bot ID and apply them to the HTML file.
Copy the
Application ID
and replaceYOUR_APPLICATION_ID
in theStreamingAssets/Chatbot.html
file.Copy the
Bot ID
and replaceYOUR_BOT_ID
in theStreamingAssets/Chatbot.html
file.
7. In the Unity Editor, click Play or Build to verify.
Subscribe to my newsletter
Read articles from HANBYEOL LEE directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
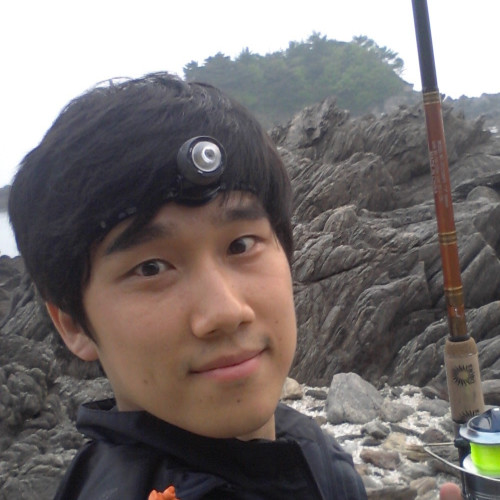