Go Series Part 9: Pointers in Go

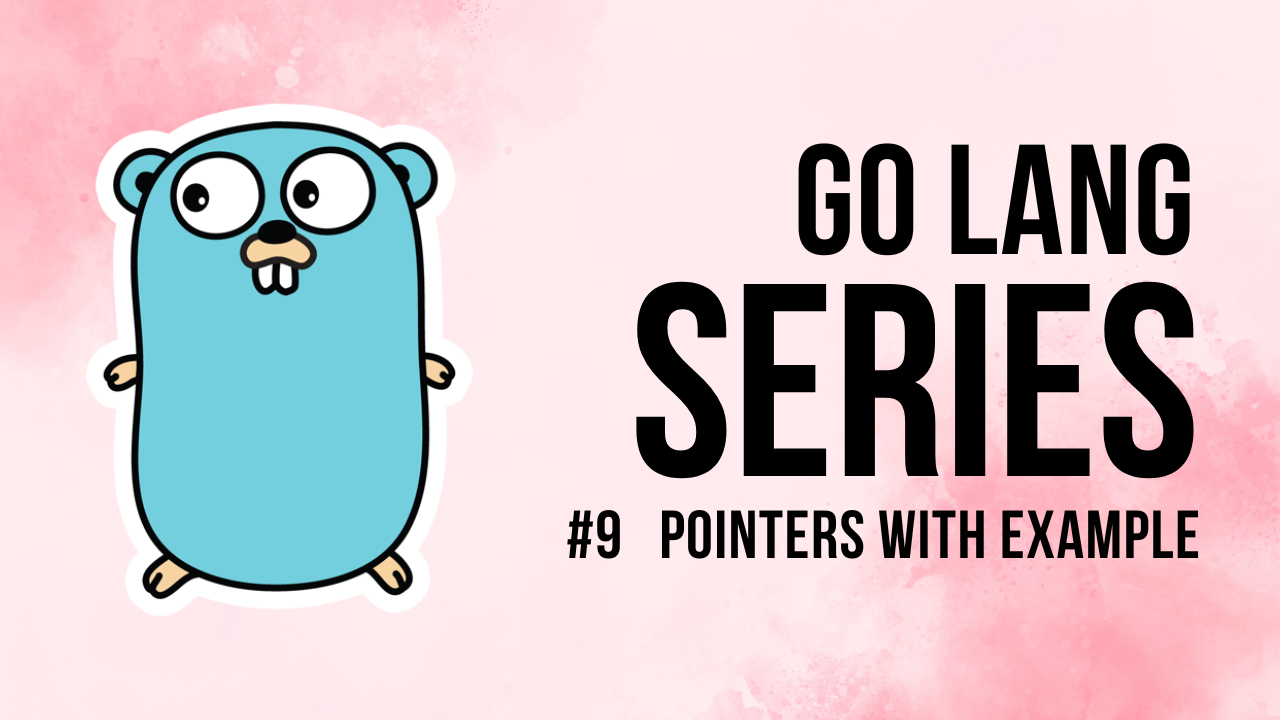
Introduction
Pointers are a fundamental concept in programming, allowing you to directly manipulate memory addresses. In Go, pointers provide a powerful way to optimize performance and manage memory effectively. This part of our series will explain how pointers work in Go, followed by examples and clear explanations.
How Pointers Work in Go
A pointer is a variable that stores the memory address of another variable. Instead of holding a data value, a pointer holds the address where the data is stored. You can use pointers to share data between functions without copying the entire data structure.
Key concepts:
Pointer Declaration: A pointer is declared using the
*
operator.Address Operator (
&
): The&
operator returns the memory address of a variable.Dereference Operator (
*
): The*
operator is used to access the value stored at the memory address.
Example 1: Basic Pointer Usage
Let's start with a simple example of pointer usage.
package main
import (
"fmt"
)
func main() {
var a int = 42
var p *int = &a
fmt.Println("Value of a:", a)
fmt.Println("Address of a:", &a)
fmt.Println("Value of p (Address of a):", p)
fmt.Println("Value at address p (Dereferencing p):", *p)
}
Output:
Value of a: 42
Address of a: 0xc0000140a0
Value of p (Address of a): 0xc0000140a0
Value at address p (Dereferencing p): 42
Explanation:
We declare an integer variable
a
with a value of 42.We declare a pointer
p
that stores the address ofa
using the&
operator.We print the value of
a
, the address ofa
, the value ofp
(which is the address ofa
), and the value at the addressp
(dereferencingp
).
Example 2: Pointers and Functions
Pointers are particularly useful when you want to modify a variable within a function.
package main
import (
"fmt"
)
func main() {
var a int = 42
fmt.Println("Before: a =", a)
modifyValue(&a)
fmt.Println("After: a =", a)
}
func modifyValue(p *int) {
*p = 100
}
Output:
Before: a = 42
After: a = 100
Explanation:
We declare an integer variable
a
with a value of 42.We pass the address of
a
to themodifyValue
function.Inside the function, we use the dereference operator
*
to modify the value stored at the addressp
.
Example 3: Pointer to a Struct
Pointers can also be used with structs to manage complex data structures efficiently.
package main
import (
"fmt"
)
type Person struct {
name string
age int
}
func main() {
p := Person{name: "Alice", age: 30}
fmt.Println("Before:", p)
modifyPerson(&p)
fmt.Println("After:", p)
}
func modifyPerson(p *Person) {
p.age = 31
}
Output:
Before: {Alice 30}
After: {Alice 31}
Explanation:
We define a
Person
struct withname
andage
fields.We create a
Person
instance and print its initial state.We pass the address of the
Person
instance to themodifyPerson
function.Inside the function, we modify the
age
field of thePerson
struct using the pointerp
.
Conclusion
Pointers are a powerful feature in Go that allow you to work directly with memory addresses, enabling efficient data manipulation and sharing between functions. Understanding pointers can significantly improve the performance and flexibility of your Go programs.
Stay tuned for the next part of our series, where we'll dive into more advanced topics. If you have any questions or need further clarification, feel free to ask!
Subscribe to my newsletter
Read articles from Navya A directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Navya A
Navya A
๐ Welcome to my Hashnode profile! I'm a passionate technologist with expertise in AWS, DevOps, Kubernetes, Terraform, Datree, and various cloud technologies. Here's a glimpse into what I bring to the table: ๐ Cloud Aficionado: I thrive in the world of cloud technologies, particularly AWS. From architecting scalable infrastructure to optimizing cost efficiency, I love diving deep into the AWS ecosystem and crafting robust solutions. ๐ DevOps Champion: As a DevOps enthusiast, I embrace the culture of collaboration and continuous improvement. I specialize in streamlining development workflows, implementing CI/CD pipelines, and automating infrastructure deployment using modern tools like Kubernetes. โต Kubernetes Navigator: Navigating the seas of containerization is my forte. With a solid grasp on Kubernetes, I orchestrate containerized applications, manage deployments, and ensure seamless scalability while maximizing resource utilization. ๐๏ธ Terraform Magician: Building infrastructure as code is where I excel. With Terraform, I conjure up infrastructure blueprints, define infrastructure-as-code, and provision resources across multiple cloud platforms, ensuring consistent and reproducible deployments. ๐ณ Datree Guardian: In my quest for secure and compliant code, I leverage Datree to enforce best practices and prevent misconfigurations. I'm passionate about maintaining code quality, security, and reliability in every project I undertake. ๐ Cloud Explorer: The ever-evolving cloud landscape fascinates me, and I'm constantly exploring new technologies and trends. From serverless architectures to big data analytics, I'm eager to stay ahead of the curve and help you harness the full potential of the cloud. Whether you need assistance in designing scalable architectures, optimizing your infrastructure, or enhancing your DevOps practices, I'm here to collaborate and share my knowledge. Let's embark on a journey together, where we leverage cutting-edge technologies to build robust and efficient solutions in the cloud! ๐๐ป