Navigating the Magic of Resource Management in Move

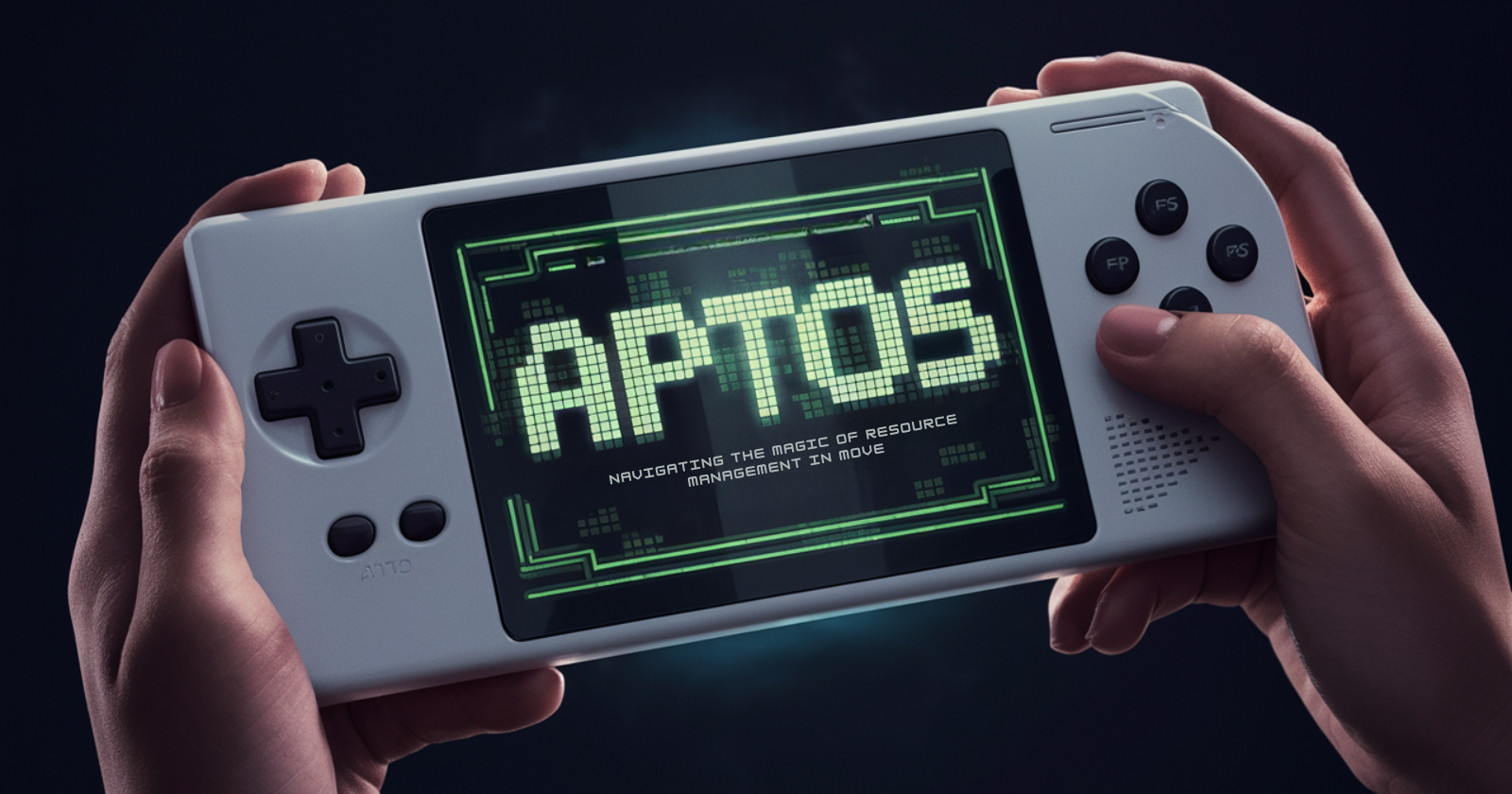
Welcome, brave developer, to the enchanted world of Move! Today, we’ll embark on an epic quest through the most challenging topic in Move: Resource Management. By the end, you'll be a wizard at managing resources in Move, ready to cast spells and create magic on the Aptos blockchain.
What is Resource Management?
In Move, resources are like magical artifacts. You can't just duplicate or throw them away without a thought. They are unique and precious, and Move ensures they stay that way.
Think of resources as legendary swords. You can't just make a copy of Excalibur, nor can you toss it in the trash. Move’s resource management ensures that your digital treasures are safe and sound.
The Basics: Ownership and Borrowing
Imagine you have a magical spellbook. Only one wizard can own the book at a time (ownership). But another wizard can borrow the book, cast a spell, and return it (borrowing).
module MagicLibrary {
resource struct SpellBook {
spells: vector<String>,
}
// Create a new SpellBook
public fun create_spellbook(spells: vector<String>): SpellBook {
SpellBook { spells }
}
// Borrow the SpellBook
public fun borrow_spellbook(book: &SpellBook): &vector<String> {
&book.spells
}
}
Move Modules: The Guilds of Magic
Modules in Move are like wizard guilds, organizing and protecting their magical resources. Each module can define, create, and manipulate these resources.
module WizardGuild {
use 0x1::MagicLibrary;
public fun create_and_use_spellbook() {
let spells = vec!["fireball".to_string(), "teleport".to_string()];
let book = MagicLibrary::create_spellbook(spells);
let borrowed_spells = MagicLibrary::borrow_spellbook(&book);
// Use the borrowed spells
}
}
The Magic of No Implicit Copying
In Move, resources are one-of-a-kind. This uniqueness is enforced by not allowing implicit copying. Imagine you have a rare potion. You can't just make another one without brewing it again from scratch.
module Alchemy {
resource struct Potion {
potency: u8,
}
// Brew a potion
public fun brew_potion(potency: u8): Potion {
Potion { potency }
}
// Attempt to copy a potion (this will fail)
public fun illegal_copy(potion: Potion): Potion {
let copied_potion = potion; // This line will cause an error
copied_potion
}
}
Practical Example: Creating a Digital Token
Let’s create a digital token on the Aptos blockchain. This example will cover defining a resource, creating and transferring tokens, and ensuring they are handled safely.
Define the Token Resource
module Token {
resource struct Token {
value: u64,
}
// Mint a new token
public fun mint_token(value: u64): Token {
Token { value }
}
}
Creating and Transferring Tokens
module TokenManager {
use 0x1::Token;
public fun create_token(value: u64): Token::Token {
Token::mint_token(value)
}
public fun transfer_token(token: Token::Token, recipient: &signer) {
// Transfer logic
}
}
Handling Errors: Avoiding Magical Mishaps
Error handling in Move ensures that resource operations are safe and predictable. Let’s add error handling to our token transfer function.
module TokenManager {
use 0x1::Token;
use 0x1::Errors;
public fun create_token(value: u64): Token::Token {
Token::mint_token(value)
}
public fun transfer_token(token: Token::Token, recipient: &signer) acquires Token::Token {
if (/* some condition */) {
move_to(recipient, token);
} else {
// Return an error if transfer conditions are not met
abort(Errors::INVALID_ARGUMENT);
}
}
}
Move Prover: Ensuring Your Magic is Error-Free
The Move Prover is a tool that helps ensure your resource management logic is correct and free from bugs.
module VerifiedToken {
resource struct Token {
value: u64,
}
public fun mint_token(value: u64): Token {
Token { value }
}
spec module {
// Ensure tokens are always created with a positive value
ensures forall(value: u64) mint_token(value).value > 0;
}
}
Tips for Mastering Move’s Magic
Understand Ownership Rules: Know the rules of ownership and borrowing to avoid common pitfalls.
Use Formal Verification: The Move Prover is your friend for verifying the correctness of your code.
Clear Naming Conventions: Use clear names for functions and resources to make your code more readable.
Resource management in Move is a unique and powerful feature that ensures the safety of digital assets on the Aptos blockchain. By mastering this concept, you can create robust and secure applications that make the most of the Move language.
Remember, practice makes perfect. Keep experimenting, and soon you'll be a grandmaster of resource management in Move. Now go forth and create some magic.
Delving Deeper into Resource Management in Move
Now that we’ve covered the basics, let’s dive deeper into the more nuanced aspects of resource management in Move. This deeper understanding will help you master the intricacies of Move and make your smart contracts more robust and efficient.
Move’s Resource Semantics
Unique Resources
Move’s resources are designed to be unique. This means that once a resource is created, it cannot be duplicated or discarded without explicit actions. This feature is crucial for maintaining the integrity of digital assets.
module Collectibles {
resource struct Collectible {
id: u64,
name: String,
}
// Create a new collectible
public fun create_collectible(id: u64, name: String): Collectible {
Collectible { id, name }
}
// Transfer a collectible
public fun transfer_collectible(collectible: Collectible, recipient: &signer) acquires Collectible {
move_to(recipient, collectible);
}
}
In this example, the Collectible
resource is unique. You cannot create a duplicate of an existing collectible, ensuring its uniqueness and value.
Ensuring Resource Safety with Move Prover
The Move Prover is a powerful tool that helps ensure your resource management logic is correct. It allows you to write specifications that can be verified to prove the correctness of your smart contracts.
Let’s enhance our Collectibles
module with formal verification:
module VerifiedCollectibles {
resource struct Collectible {
id: u64,
name: String,
}
public fun create_collectible(id: u64, name: String): Collectible {
Collectible { id, name }
}
public fun transfer_collectible(collectible: Collectible, recipient: &signer) acquires Collectible {
move_to(recipient, collectible);
}
spec module {
// Ensure collectibles are always created with a unique ID
ensures forall(id: u64, name: String) {
let collectible = create_collectible(id, name);
collectible.id == id
};
}
}
With these specifications, the Move Prover ensures that each collectible is created with the correct unique ID, preventing any inconsistencies in your smart contracts.
Advanced Resource Management: Access Control
Access control is a crucial aspect of resource management. It allows you to specify who can access and manipulate your resources. Move provides robust mechanisms for defining and enforcing access control.
Let’s add access control to our Collectibles
module:
module AccessControlledCollectibles {
resource struct Collectible {
id: u64,
name: String,
}
// Create a new collectible with access control
public fun create_collectible(id: u64, name: String): Collectible {
Collectible { id, name }
}
// Transfer a collectible with access control
public fun transfer_collectible(collectible: Collectible, recipient: &signer) acquires Collectible {
// Only allow transfer if the sender is the owner
assert!(is_owner(&signer), Errors::NOT_AUTHORIZED);
move_to(recipient, collectible);
}
fun is_owner(signer: &signer): bool {
// Define your ownership logic here
true
}
}
In this example, the transfer_collectible
function includes a check to ensure that only the owner can transfer the collectible. This access control mechanism helps prevent unauthorized actions, adding an extra layer of security to your smart contracts.
Error Handling: Keeping Your Spells in Check
Proper error handling ensures that your smart contracts behave predictably and securely, even in the face of unexpected conditions. Move’s error handling mechanisms allow you to define and handle errors gracefully.
Let’s improve our Collectibles
module with robust error handling:
module SafeCollectibles {
use 0x1::Errors;
resource struct Collectible {
id: u64,
name: String,
}
public fun create_collectible(id: u64, name: String): Collectible {
Collectible { id, name }
}
public fun transfer_collectible(collectible: Collectible, recipient: &signer) acquires Collectible {
// Check if the recipient is valid
if (!is_valid_recipient(recipient)) {
abort(Errors::INVALID_ARGUMENT);
}
move_to(recipient, collectible);
}
fun is_valid_recipient(recipient: &signer): bool {
// Define your recipient validation logic here
true
}
}
In this enhanced module, the transfer_collectible
function includes a check to validate the recipient. If the recipient is invalid, an error is raised, preventing the transfer and ensuring the integrity of the collectible.
Mastering Resource Management
By delving deeper into the advanced aspects of resource management in Move, you can create more secure, efficient, and reliable smart contracts on the Aptos blockchain. From understanding the uniqueness of resources to leveraging formal verification, access control, and error handling, these techniques will equip you to tackle any challenge.
Keep experimenting, keep learning, and soon you’ll be a grandmaster of resource management in Move. Now go forth and weave your magic on the blockchain!
May your code be bug-free and your resources well-managed—until next time, happy coding
References
Subscribe to my newsletter
Read articles from swayam sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
