How to Boost Performance and Scalability in PHP and Laravel Apps

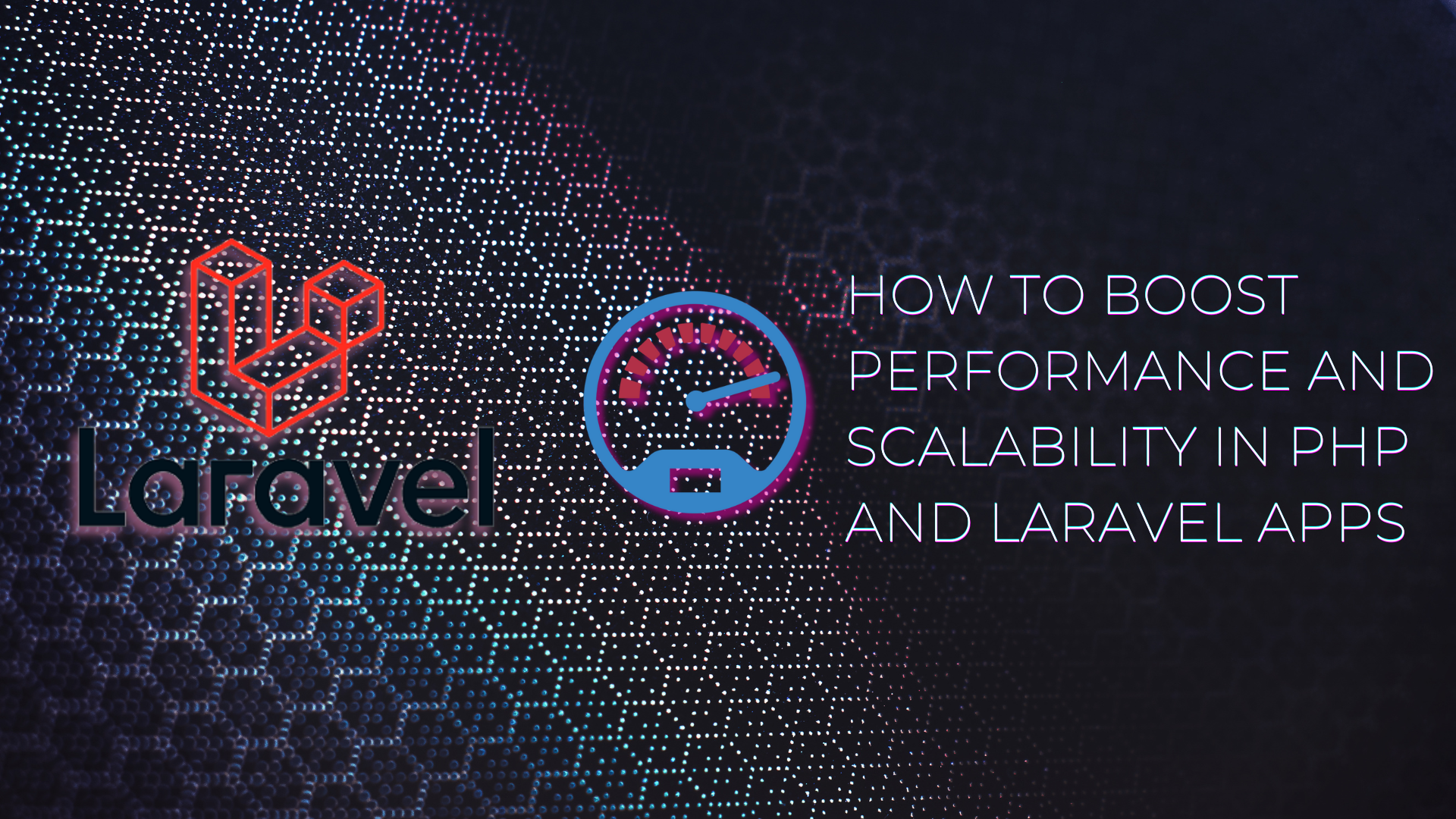
In the fast-paced world of web development, making sure your application is quick and can handle growth is super important. Users want fast and responsive interfaces, and if your app is slow, they might get frustrated and leave. Laravel, a popular PHP framework, has lots of tools and best practices to help you boost performance and ensure scalability. In this friendly guide, we'll explore advanced techniques and best practices for optimizing Laravel applications, including database optimization, caching strategies, and code profiling. By the end, you'll have a better grasp of how to make your Laravel app run faster and handle more users.
Database Optimization
Databases are often the backbone of web applications, and their performance directly impacts the overall application speed. Proper database optimization can significantly reduce latency and improve responsiveness.
Indexing
Indexes are critical for speeding up database queries. Without indexes, the database must perform a full table scan, which can be slow for large datasets. Ensure that your tables have proper indexes on columns frequently used in WHERE
, JOIN
, and ORDER BY
clauses.
Example: Adding an index to a migration
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class AddEmailIndexToUsersTable extends Migration
{
public function up()
{
Schema::table('users', function (Blueprint $table) {
$table->index('email');
});
}
public function down()
{
Schema::table('users', function (Blueprint $table) {
$table->dropIndex(['email']);
});
}
}
Query Optimization
Avoid N+1 query problems by using eager loading. Eloquent's lazy loading can lead to multiple database queries, slowing down performance.
Example: Eager Loading
// Lazy Loading (Inefficient)
$books = Author::find(1)->books;
// Eager Loading (Efficient)
$books = Author::with('books')->find(1);
Eager loading retrieves related models as part of the initial query, reducing the number of queries executed and significantly improving performance.
Eloquent ORM Performance
While Eloquent ORM is convenient, it can be slow for complex operations. Use raw queries for more complex operations and avoid using get()
when exists()
or count()
can suffice.
Example: Using raw queries
$users = DB::select('SELECT * FROM users WHERE active = ?', [1]);
Using raw SQL queries can be more efficient for complex operations that might otherwise require multiple Eloquent queries.
Caching Strategies
Caching is one of the most effective ways to improve application performance. By storing the results of expensive operations and reusing them, you can reduce the load on your database and application servers.
Config and Route Caching
Configuration and route caching can significantly reduce the loading time of your Laravel application.
Command to cache configurations
php artisan config:cache
Command to cache routes
php artisan route:cache
These commands compile all configurations and routes into a single file, reducing the time it takes Laravel to bootstrap your application.
Query Caching
Laravel provides an easy way to cache database queries.
Example: Query Caching
$users = Cache::remember('users', 60, function() {
return DB::table('users')->get();
});
In this example, the query results are cached for 60 minutes, reducing the need to run the same query multiple times.
Object Caching with Redis and Memcached
Redis and Memcached are in-memory data stores that can be used to cache data, significantly improving performance.
Example: Using Redis for caching
Cache::store('redis')->put('key', 'value', 600);
Using Redis or Memcached, you can cache objects and data, reducing the load on your database and speeding up your application.
Code Profiling and Monitoring
Profiling and monitoring your application can help identify performance bottlenecks and areas that need optimization.
Profiling with Xdebug and Blackfire
Profiling tools like Xdebug and Blackfire can help identify performance bottlenecks in your code.
Using Xdebug
# Install Xdebug via PECL
pecl install xdebug
# Enable Xdebug in php.ini
zend_extension="xdebug.so"
Xdebug provides detailed profiling information that can help you understand which parts of your code are slow and need optimization.
Monitoring with Laravel Telescope
Laravel Telescope provides insights into your application's performance, including requests, jobs, and exceptions.
Installing Laravel Telescope
composer require laravel/telescope
php artisan telescope:install
php artisan migrate
php artisan telescope:publish
Telescope is an excellent tool for monitoring your Laravel application and identifying performance issues in real-time.
Other Performance Tips
Optimizing Blade Templates
Blade templates are powerful but can be optimized further to improve performance.
Example: Caching views
php artisan view:cache
Caching views compiles Blade templates into plain PHP code, reducing the overhead of parsing Blade templates on each request.
Efficient Use of Middleware
Middleware can affect performance if overused. Only use middleware where necessary and consider using route groups to apply middleware selectively.
Example: Route groups with middleware
Route::middleware(['auth', 'throttle:60,1'])->group(function () {
Route::get('/dashboard', 'DashboardController@index');
});
By applying middleware selectively, you can reduce the performance overhead and improve the overall speed of your application.
Job Queues and Background Processing
Use Laravel queues to handle time-consuming tasks in the background, improving the responsiveness of your application.
Example: Creating a job
php artisan make:job ProcessPodcast
// In the job class
public function handle()
{
// Process the podcast...
}
Dispatching a job
ProcessPodcast::dispatch($podcast);
Configuring the queue
# Start a queue worker
php artisan queue:work
By offloading time-consuming tasks to background jobs, you can ensure that your application remains responsive and performs well under load.
Conclusion
Optimizing Laravel applications for performance and scalability involves a multi-faceted approach, from database optimization and caching to code profiling and background processing. By implementing these advanced techniques and best practices, you can ensure that your Laravel applications remain fast, responsive, and scalable as they grow.
Remember, optimization is an ongoing process. Regularly profile your application, monitor performance, and keep up-to-date with the latest Laravel features and community best practices. By doing so, you can continuously improve your application's performance and provide a better experience for your users.
In this guide, we've covered various strategies to optimize Laravel applications, including indexing, query optimization, eager loading, caching strategies, code profiling, and efficient use of middleware and job queues. Each of these techniques contributes to the overall performance and scalability of your application.
As you continue to develop and maintain your Laravel applications, keep these best practices in mind. Regularly review your code and database queries, profile your application, and make use of Laravel's built-in tools and features to ensure optimal performance.
By following these guidelines, you can create robust, high-performing Laravel applications that scale effectively and provide a seamless experience for your users.
Subscribe to my newsletter
Read articles from Hamza Kachachi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Hamza Kachachi
Hamza Kachachi
Hello! My namesake is Hamza Kachachi. I come from Fes, Morocco. I am engaged in creating powerful web and mobile applications with Application Interfaces in NodeJS and ReactJS. I am also able to use PHP, Laravel and MongoDB. Furthermore, I know both front-end and back-end programming languages enough to feel comfortable with Agile methodologies. In my free time I enjoy playing basketball, chess or football or simply listening to music. Let’s connect and create something amazing together!