Unlocking Advanced Git Skills for Full Stack Development

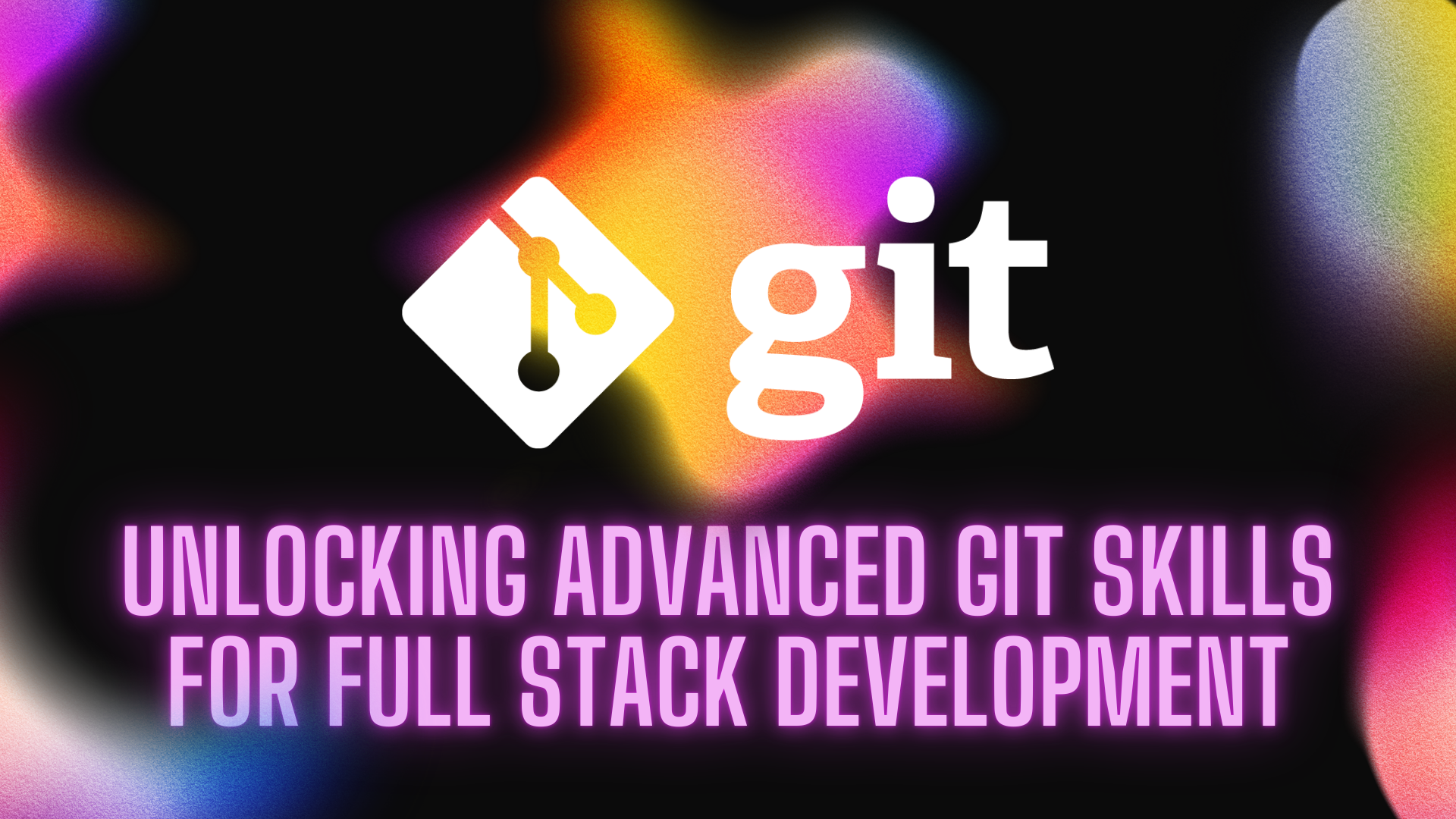
Git is an essential tool in the modern full-stack developer's toolkit. While basic commands like git add
, git commit
, and git push
are commonly used, there are advanced features of Git that can significantly enhance workflow efficiency. In this blog post, we'll explore advanced Git techniques such as rebase, cherry-pick, and submodules, complete with command examples to help you master these features.
Introduction to Advanced Git Techniques
As full-stack developers, we often work on complex projects with multiple branches and collaborators. Understanding advanced Git techniques can help manage code more effectively, resolve conflicts smoothly, and maintain a cleaner project history. Let’s dive into these advanced Git commands and see how they can improve your workflow.
1. Git Rebase: Streamlining Your Commit History
What is Git Rebase?
Git rebase is a powerful command that allows you to move or combine a sequence of commits to a new base commit. This helps in creating a linear, easy-to-read project history, which is especially useful when working with feature branches.
Benefits of Git Rebase
Cleaner History: A linear commit history makes it easier to understand the project’s evolution.
Easier Merges: Reduces the complexity of merging branches by eliminating unnecessary merge commits.
How to Use Git Rebase
Let's say you have a feature branch feature-branch
and you want to rebase it onto the main
branch:
git checkout feature-branch
git rebase main
If there are conflicts, Git will pause the rebase and allow you to resolve them. Once resolved, you can continue the rebase with:
git rebase --continue
If you need to abort the rebase process:
git rebase --abort
2. Git Cherry-Pick: Applying Specific Commits
What is Git Cherry-Pick?
Git cherry-pick allows you to apply the changes introduced by specific commits from one branch into another. This is useful when you want to apply bug fixes or features from one branch without merging the entire branch.
Benefits of Git Cherry-Pick
Selective Commit Application: Apply only the necessary changes without merging entire branches.
Targeted Updates: Ideal for applying hotfixes to production branches.
How to Use Git Cherry-Pick
First, identify the commit hash you want to cherry-pick. Then, apply the commit to your current branch:
git checkout target-branch
git cherry-pick <commit-hash>
For example:
git cherry-pick abc1234
If conflicts occur, resolve them and continue the cherry-pick with:
git cherry-pick --continue
To abort the cherry-pick:
git cherry-pick --abort
3. Git Submodules: Managing Dependencies
What are Git Submodules?
Git submodules allow you to keep a Git repository as a subdirectory of another Git repository. This is useful for managing dependencies or including libraries while keeping their history and version control separate.
Benefits of Git Submodules
Modular Development: Manage dependencies as separate repositories, maintaining their individual histories.
Reuse Code: Easily include and update shared libraries across multiple projects.
How to Use Git Submodules
To add a submodule to your repository:
git submodule add <repository-url> <path>
For example:
git submodule add https://github.com/example/library.git lib/library
To initialize and update submodules:
git submodule update --init --recursive
To pull the latest changes from the submodule:
cd lib/library
git pull origin main
To remove a submodule:
- Remove the submodule entry from
.gitmodules
:
git rm --cached <path>
- Delete the submodule directory:
rm -rf <path>
- Commit the changes:
git commit -m "Removed submodule"
4. Git Stash: Saving Changes Temporarily
What is Git Stash?
Git stash allows you to save changes in your working directory temporarily without committing them. This is useful when you need to switch branches or pull changes without committing your current work.
Benefits of Git Stash
Save Work in Progress: Save your work temporarily and return to it later.
Keep Working Directory Clean: Maintain a clean working directory while working on different tasks.
How to Use Git Stash
To stash changes:
git stash
To list stashed changes:
git stash list
To apply the latest stash:
git stash apply
To apply a specific stash:
git stash apply stash@{index}
For example:
git stash apply stash@{0}
To drop a specific stash:
git stash drop stash@{index}
To pop the latest stash (apply and remove it from the list):
git stash pop
5. Git Interactive Rebase: Editing Commits
What is Interactive Rebase?
Interactive rebase allows you to rewrite commits, change commit messages, combine commits, or reorder them. This is particularly useful for cleaning up your commit history before merging a feature branch.
Benefits of Interactive Rebase
Edit Commit History: Modify commit messages, combine commits, or remove unnecessary commits.
Enhanced Collaboration: Create a cleaner commit history for easier collaboration and code reviews.
How to Use Interactive Rebase
To start an interactive rebase:
git rebase -i <base-commit>
For example, to rebase the last 5 commits:
git rebase -i HEAD~5
In the interactive rebase interface, you can pick, edit, squash, or reword commits. To squash (combine) commits, replace pick
with squash
:
pick abc1234 Commit message 1
squash def5678 Commit message 2
Save and exit the editor to complete the rebase.
6. Git Bisect: Debugging with Binary Search
What is Git Bisect?
Git bisect helps you find the commit that introduced a bug by performing a binary search through your commit history. This is useful for debugging and pinpointing problematic changes.
Benefits of Git Bisect
Efficient Debugging: Quickly identify the commit that introduced a bug.
Automated Process: Git guides you through the binary search, simplifying the debugging process.
How to Use Git Bisect
To start a bisect session:
git bisect start
Mark the current commit as bad:
git bisect bad
Mark an earlier commit as good:
git bisect good <commit-hash>
Git will check out a commit halfway between the good and bad commits. Test the code and mark the commit as good or bad:
git bisect good
# or
git bisect bad
Continue this process until Git identifies the first bad commit. To end the bisect session:
git bisect reset
Conclusion
Mastering advanced Git techniques like rebase, cherry-pick, submodules, stash, interactive rebase, and bisect can significantly enhance your workflow as a full-stack developer. These commands not only help maintain a cleaner project history but also streamline collaboration and debugging processes. By integrating these advanced features into your daily development routine, you can manage complex projects more efficiently and effectively.
Remember, practice is key to becoming proficient with these advanced Git commands. Experiment with them in your projects, and soon you'll find yourself navigating version control with greater confidence and ease. Happy coding!
Subscribe to my newsletter
Read articles from Hamza Kachachi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Hamza Kachachi
Hamza Kachachi
Hello! My namesake is Hamza Kachachi. I come from Fes, Morocco. I am engaged in creating powerful web and mobile applications with Application Interfaces in NodeJS and ReactJS. I am also able to use PHP, Laravel and MongoDB. Furthermore, I know both front-end and back-end programming languages enough to feel comfortable with Agile methodologies. In my free time I enjoy playing basketball, chess or football or simply listening to music. Let’s connect and create something amazing together!