Simple Steps to Delete Twilio Message Logs in a Node.js Application
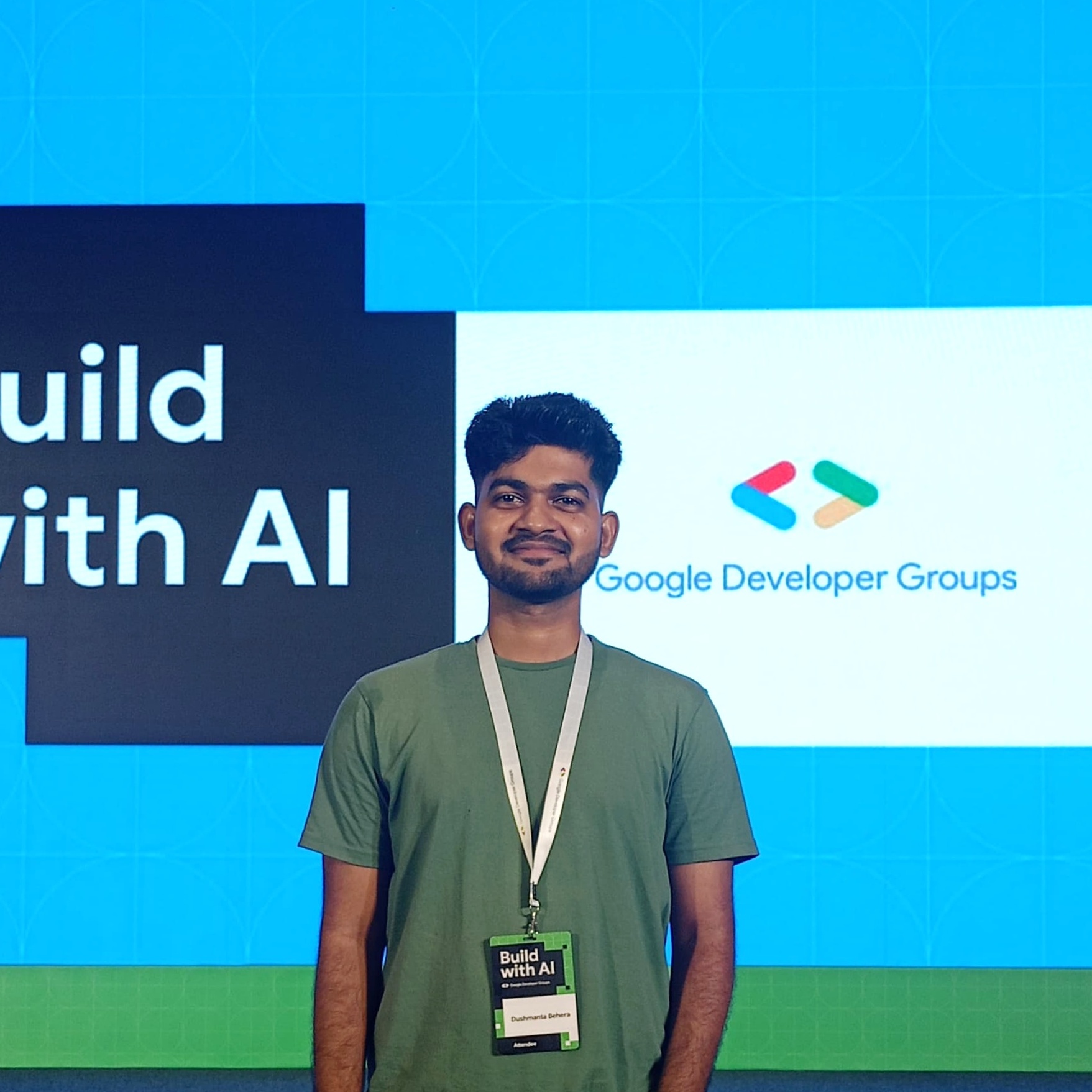
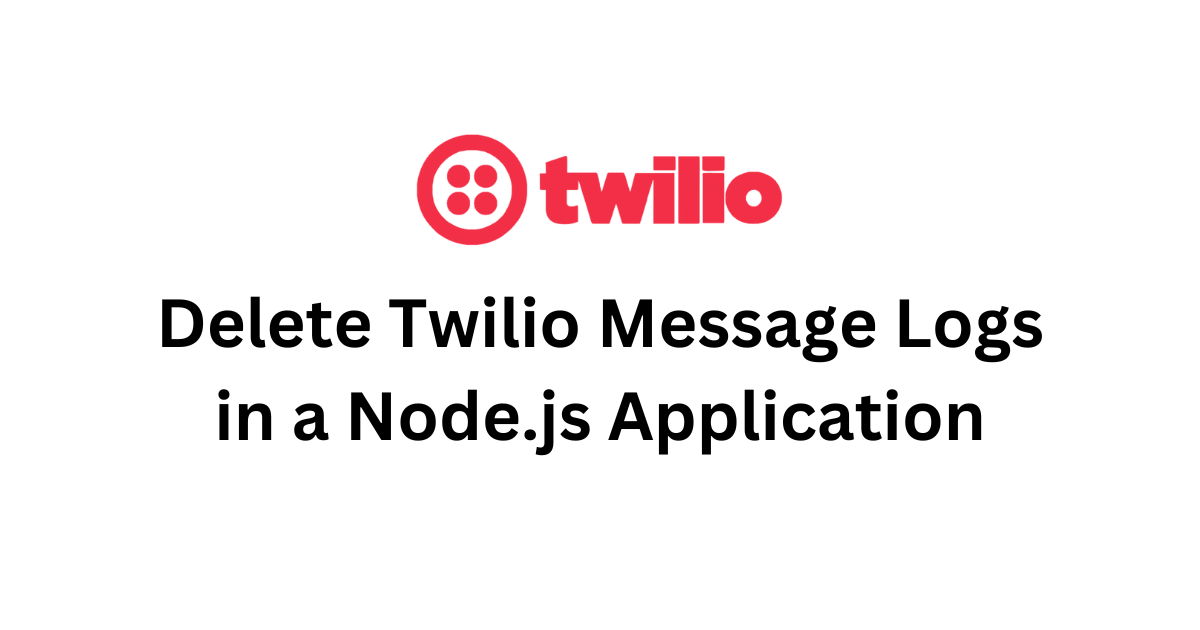
Hello developers, In this article, we'll explore how you can delete message logs from your Twilio project using Node.js
. This will help you manage your Twilio message logs efficiently.
Prerequisite: Before diving in, you should have basic knowledge of Node.js
and a Twilio account with messaging services. Make sure you have your Twiliocredentials (Account SID and Auth Token).
I won't be explaining how to create a Node.js
application, as I expect you already have a Node.js
project.
Install Twilio:
We'll be using the twilio
package for this task. First, let's install the package with the following command:
npm i twilio # npm
yarn add twilio # yarn
Implementation:
Configure Twilio Client:
Next, we need to set up the Twilio client using the Account SID and Auth Token, which you can get from the Twilio console page. Here is the code to do this:
const twilio = require("twilio");
const accountSID = "YOUR_ACCOUNT_SID";
const authToken = "YOUR_AUTH_TOKEN";
const client = twilio(accountSID, authToken);
Replace YOUR_ACCOUNT_SID
and YOUR_AUTH_TOKEN
with your Account SID and Auth Token, respectively.
Deletion Functionality:
Using the Twilio client, we can delete a specific message log by calling the remove()
method with the Message SID.
Let's look at the following code to see how this works:
const deleteMessageLog = async (sid) => {
try {
await client.messages(sid).remove();
console.log(`Message ${sid} deleted successfully`);
} catch (error) {
console.error(`Error deleting message ${sid}:`, error);
}
}
const messageSID = "MESSAGE_SID";
deleteMessageLog(messageSID);
In the above code, replace MESSAGE_SID
with the Message SID of the message log you want to delete.
If you want to delete multiple message logs at once, you can use a for loop with an array of message SIDs. Let's see how this works in the code below, where I'll use the for...of
method instead of a traditional for loop.
const deleteMessageLogs = async (messageSIDs) => {
for (const sid of messageSIDs) {
try {
await client.messages(sid).remove();
console.log(`Message ${sid} deleted successfully`);
} catch (error) {
console.error(`Error deleting message ${sid}:`, error);
}
}
}
const messageSIDs = ["MESSAGE_SID_1", "MESSAGE_SID_2", "MESSAGE_SID_3"];
deleteMessageLogs(messageSIDs);
In the above code, replace MESSAGE_SID_1
, MESSAGE_SID_2
, and MESSAGE_SID_3
with the actual Message SIDs you want to delete.
I hope this article helps you delete Twilio message logs using the twilio
package. Please share any feedback or suggestions for improvement. Thank you.
Subscribe to my newsletter
Read articles from Dushmanta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
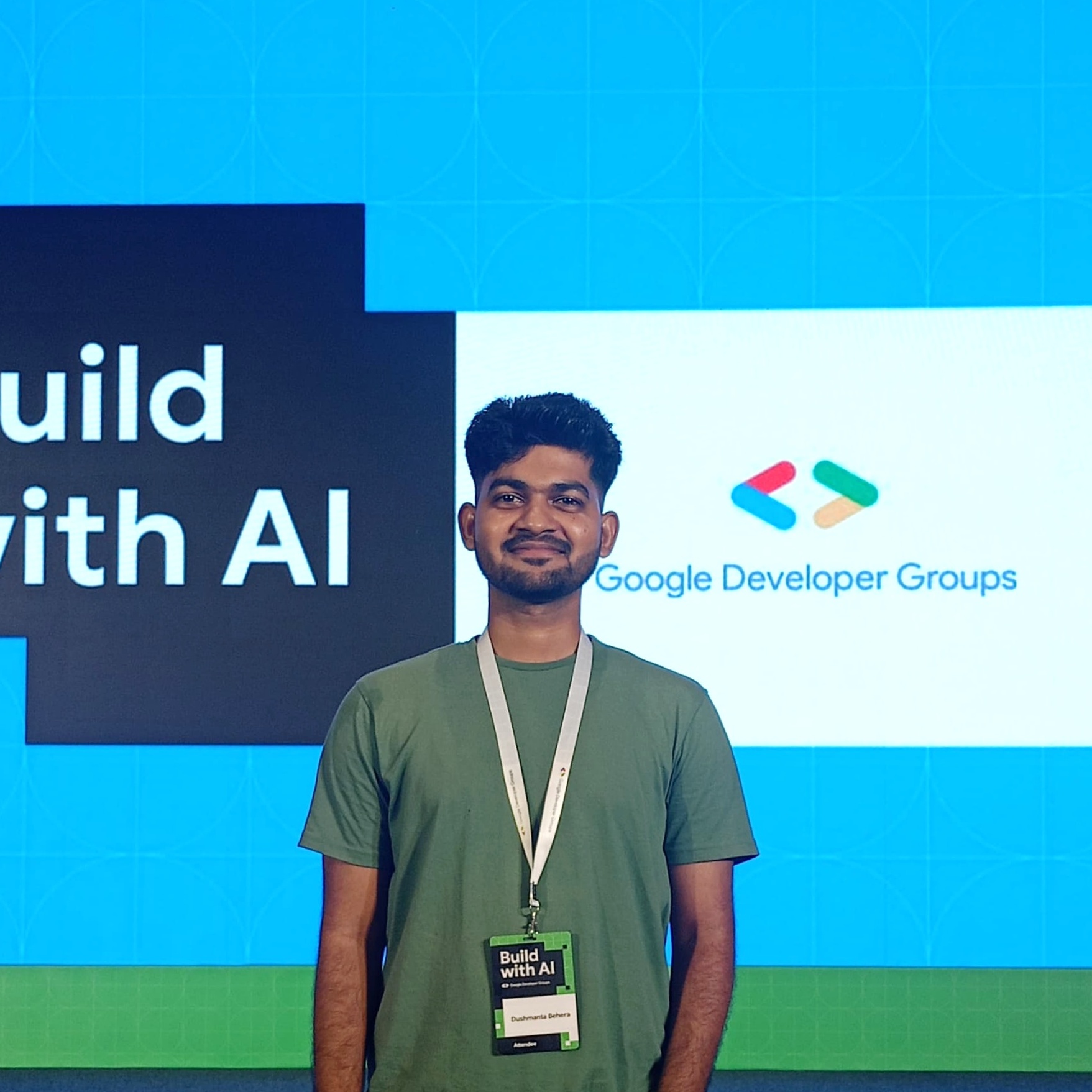
Dushmanta
Dushmanta
Passionate Software Engineer