Preventing Balance Transfer Exploits: Resolving a Case-Insensitive Username Comparison After a Hacker Attempt
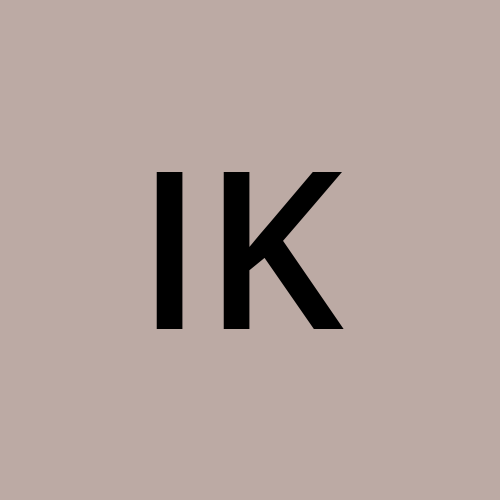
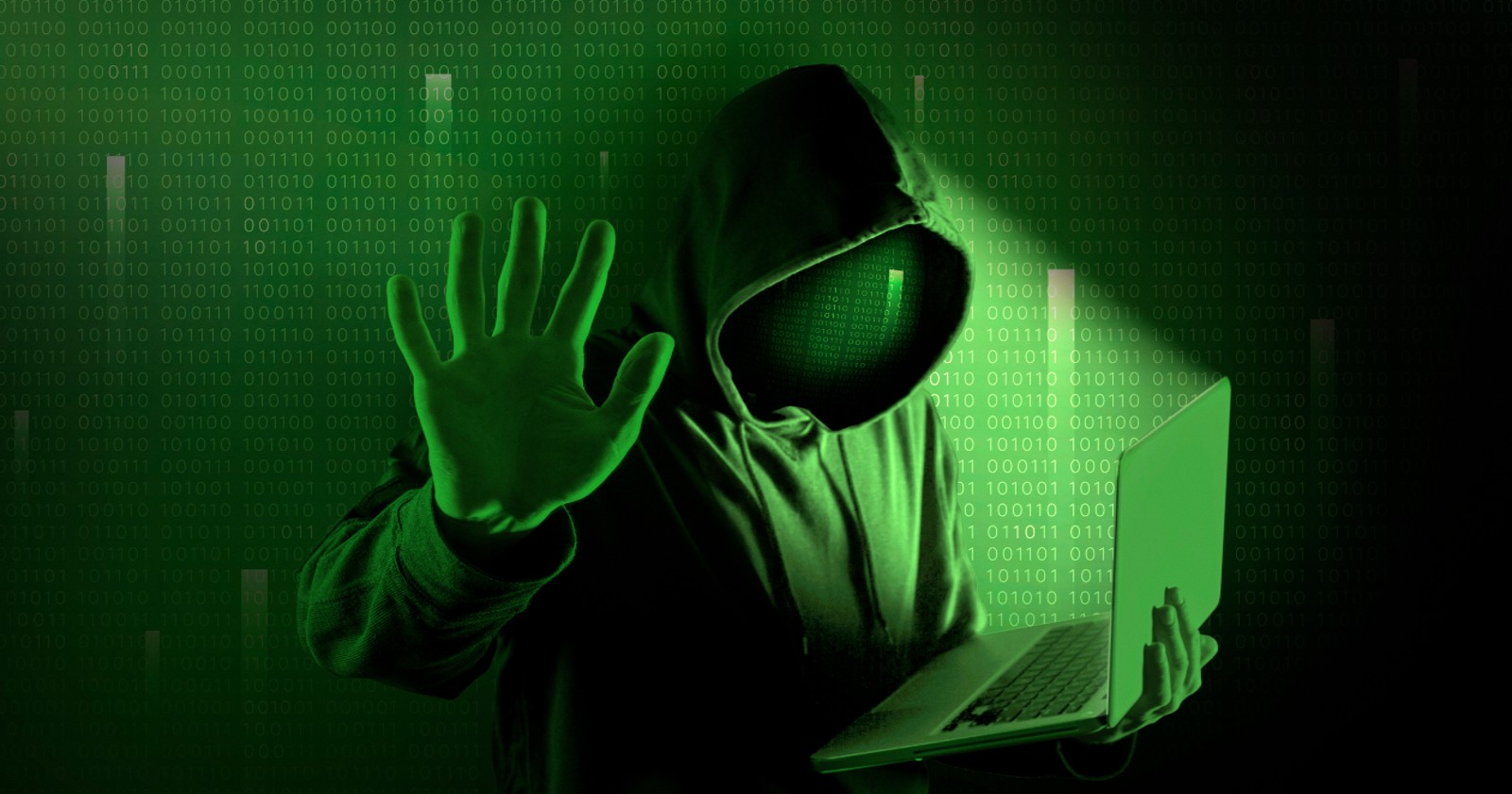
Introduction
In web development, ensuring that users cannot perform unintended actions is crucial for maintaining the integrity and security of an application. Recently, I encountered a situation where users were able to transfer balance to their own accounts despite having validation in place. In this article, I’ll share my experience and the solution I implemented to resolve the issue.
The Problem
I had implemented a feature to prevent users from transferring balance to their own account with the following validation:
$sender = auth()->user();
if ($sender->username === $receiver->username) {
$notify[] = ['error', 'You cannot transfer balance to your own account'];
return back()->withNotify($notify);
}
Despite this validation, a user was able to transfer balance to themselves. This led me to investigate further and identify the root cause of the issue.
Investigation
After reviewing the code and considering various scenarios, I found that the issue was due to case-sensitive comparison of usernames. The original validation failed to account for different cases (e.g., UserName
vs username
). Additionally, it turned out that a user, aware of bypass techniques via client-side manipulation, attempted to exploit this oversight.
The Solution: Case-Insensitive Comparison
To resolve this, I updated the validation to perform a case-insensitive comparison using strcasecmp
in PHP. Here’s the updated code:
$sender = auth()->user();
if (strcasecmp($sender->username, $receiver->username) == 0) {
$notify[] = ['error', 'You cannot transfer balance to your own account'];
return back()->withNotify($notify);
}
Why Case-Insensitive Comparison?
Case-insensitive comparison ensures that usernames are compared without considering their case, which eliminates the possibility of bypassing validation by changing the case of the username.
Client-Side and Server-Side Validation
While client-side validation is useful for providing immediate feedback to users, it is not sufficient on its own. Critical validations must be performed on the server side to prevent bypasses via client-side manipulation. In this case, despite having client-side validation tested and in place, the server-side validation needed to be robust to prevent such exploits.
Lessons Learned
Server-Side Validation: Always perform critical validations on the server side.
Case Sensitivity: Be aware of case sensitivity in comparisons and ensure that validations account for different cases.
Robust Security Measures: Implement comprehensive security measures to prevent exploits.
Conclusion
By updating the validation to use case-insensitive comparison, I was able to prevent users from transferring balance to their own accounts. It’s a small but important change that enhances the security and reliability of the application. I hope this article helps other developers facing similar issues.
Your Thoughts
Have you encountered similar issues in your projects? How did you resolve them? Share your experiences and solutions in the comments below!
Subscribe to my newsletter
Read articles from IRFAN ALI KHAN directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
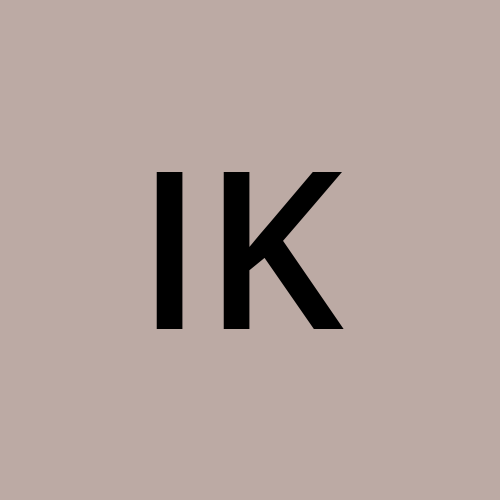