From Rust to Move: A Guide for Rust Developers Transitioning to Move
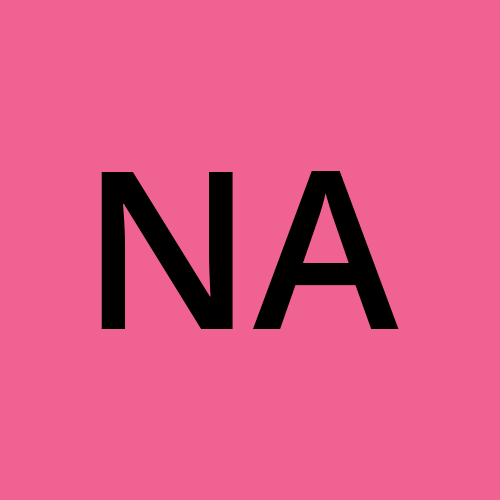
As a Rust developer, you're already accustomed to a language that emphasizes safety, concurrency, and performance. Rust's system-level control and memory safety guarantees make it a powerful tool for building reliable and efficient software. If you're looking to transition to developing smart contracts on the Aptos blockchain, you'll find Move to be an intriguing and complementary language. Move, designed specifically for blockchain applications, shares some similarities with Rust but also introduces unique concepts tailored for secure digital asset management.
In this article, we'll explore the key differences and similarities between Rust and Move, and provide practical examples to help you make the transition smoothly. We'll also cover some fundamental blockchain concepts to give you a better understanding of what it takes to be an Aptos smart contract developer.
Introduction to Blockchain Concepts
Blockchain
A blockchain is a decentralized and distributed digital ledger that records transactions across multiple computers. This ensures that the recorded transactions cannot be altered retroactively, providing a secure and transparent way to track assets.
Smart Contracts
Smart contracts are self-executing contracts with the terms of the agreement directly written into code. They run on a blockchain, allowing for automated, secure, and transparent transactions without the need for intermediaries.
Deployment of Smart Contracts
Deploying a smart contract involves writing the contract's code, compiling it, and then publishing it to the blockchain network.
Aptos SDK
The Aptos SDK (Software Development Kit) provides tools and libraries for developers to interact with the Aptos blockchain. It simplifies the process of writing, testing, and deploying smart contracts, making it easier to build decentralized applications (DApps).
Tokens
Tokens are digital assets on a blockchain that can represent various assets or utilities like currency or voting rights. They are divided into two types:
Fungible Tokens: Identical in value and interchangeable, e.g., Bitcoin (BTC), Ethereum (ETH) and of course Aptos (APT).
Non-Fungible Tokens (NFTs): Unique and not interchangeable, ideal for representing ownership of unique items like digital art or real estate.
Why Move?
Move was created to address the specific needs of blockchain development, focusing on safety, flexibility, and resource management. Unlike general-purpose languages, Move introduces the concept of resources that represent assets, ensuring they cannot be duplicated or discarded unintentionally. This resource-oriented programming model is designed to prevent common vulnerabilities and ensure the integrity of digital assets.
Similarities Between Rust and Move
Memory Safety
Both Rust and Move prioritize memory safety. Rust achieves this through its ownership model and borrowing rules, preventing data races and null pointer dereferencing. Move, while not dealing with memory management directly, ensures safety by treating resources as first-class citizens that must be explicitly created, transferred, and destroyed.
Strong Typing
Rust and Move both enforce strict type safety, reducing the likelihood of runtime errors. In Rust, the type system helps catch errors at compile time, ensuring that variables are used correctly. Move extends this concept to its resource-oriented programming model, ensuring that resources are used safely and predictably.
Modular Design
Rust's module system allows for the organization and reuse of code, making it easier to manage complex projects. Similarly, Move's modular design encourages the creation of reusable and composable modules, enabling developers to build complex smart contracts from simpler components.
Key Differences Between Rust and Move
Resource-Oriented Programming
The most significant difference between Rust and Move is the latter's focus on resource-oriented programming. In Move, resources represent assets that cannot be duplicated or discarded. This is crucial for managing digital assets on the blockchain, ensuring their integrity and security.
Execution Model
Rust is a general-purpose programming language designed for a wide range of applications, from system programming to web development. Move, on the other hand, is specifically designed for writing smart contracts. Move's execution model is built around transactions and scripts, allowing for secure and flexible interactions with the blockchain.
Standard Library and Ecosystem
Rust has a rich standard library and a thriving ecosystem with crates for virtually every use case. Move, being a more specialized language, has a smaller standard library focused on blockchain-related functionality. However, this specialization ensures that the available libraries and tools are highly optimized for smart contract development.
Practical Examples
To illustrate the transition from Rust to Move, let's look at some practical examples.
Example 1: Basic Structure
In Rust, a basic program might look like this:
fn main() {
println!("Hello, Rust!");
}
In Move, a similar program would be a module with a public function:
module addr::HelloWorld {
public fun say_hello() {
}
}
Example 2: Defining a Struct
In Rust, you define a struct and implement methods on it:
struct Point {
x: i32,
y: i32,
}
impl Point {
fn new(x: i32, y: i32) -> Point {
Point { x, y }
}
fn change(&mut self, new_x: i32, new_y: i32) {
self.x = new_x;
self.y = new_y;
}
}
In Move, you define a resource and functions that operate on it:
module addr::PointModule {
struct Point has key {
x: u64,
y: u64,
}
public fun create_point(x: u64, y: u64): Point acquires Point {
Point { x, y }
}
public fun change_point(point: &mut Point, new_x: u64, new_y: u64) {
point.x = new_x;
point.y = new_y;
}
}
Example 3: Ownership and Borrowing
Rust's ownership model ensures that each value has a single owner, preventing data races:
fn main() {
let mut s = String::from("hello");
let r1 = &s;
let r2 = &s;
println!("{} and {}", r1, r2);
}
Move's resource-oriented programming model similarly ensures safe handling of resources:
module addr::OwnershipExample {
struct MyResource {
value: u64,
}
public fun create_resource(value: u64): MyResource {
MyResource { value }
}
public fun use_resource(res: MyResource) {
// Resource is moved here, cannot be used elsewhere.
}
}
Example 4: Error Handling
Rust uses the Result
type for error handling:
fn divide(a: i32, b: i32) -> Result<i32, String> {
if b == 0 {
Err(String::from("Division by zero"))
} else {
Ok(a / b)
}
}
Move uses a similar approach with error codes and abort statements:
module addr::ErrorHandling {
use std::error;
const E_DIVISION_BY_ZERO: u64 = 1;
public fun divide(a: u64, b: u64): u64 acquires Error {
if (b == 0) {
error::invalid_argument(E_DIVISION_BY_ZERO);
}
a / b
}
}
Conclusion
Transitioning from Rust to Move involves understanding the unique features of Move, particularly its resource-oriented programming model and its focus on blockchain-specific functionality. While there are significant differences, your familiarity with Rust's emphasis on safety, strong typing, and modular design will serve you well.
By grasping these core concepts and examining practical examples, you'll be well-equipped to develop secure and efficient smart contracts on the Aptos blockchain. As you continue to explore Move, you'll find that its design principles and language features align closely with the needs of blockchain development, providing a robust foundation for building the next generation of decentralized applications.
Explore More with Video Workshops
If you're a visual learner or prefer to follow along with video tutorials, check out these YouTube workshops that dive deeper into Aptos development:
Daniel: Provides a detailed overview of Aptos Move, designed for secure programming in managing and transferring assets with a focus on security and protection against attacks. He explains the key features of Aptos, emphasizing low latency, instant finality, and built-in standards for tokens and coins. Daniel also delves into the specifics of the Move language, showcasing a simple program for creating and transferring coins while focusing on safety and correctness in code writing.
Brian: Guides participants on getting started with Aptos, discussing the similarities between frontend development in Aptos and JavaScript/TypeScript, highlighting the ease of migration. The importance of indexer support, developer documentation quality, and utilizing 'create-aptos-dapp’ tool for project initiation is emphasized. The tutorial covers Move code unique aspects, error handling, view functions, and unit testing.
Angie: Focuses on the concept of objects and capabilities like delete, extend, transfer, and create signer. It explores the efficiency of separating ownership and data location in Aptos objects, emphasizing simplification in updates and implementations. The discussion includes creating fungible assets, NFTs, and the impact of token standards on interactive applications.
Subscribe to my newsletter
Read articles from Nad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
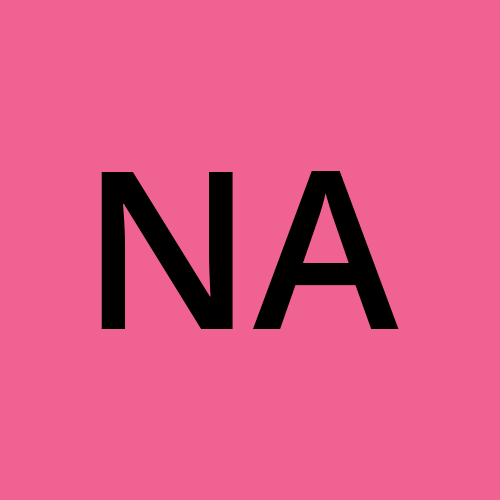
Nad
Nad
I am a Smart Contract Engineer.