Deploy nodejs app on VPS (AWS EC2) using pm2
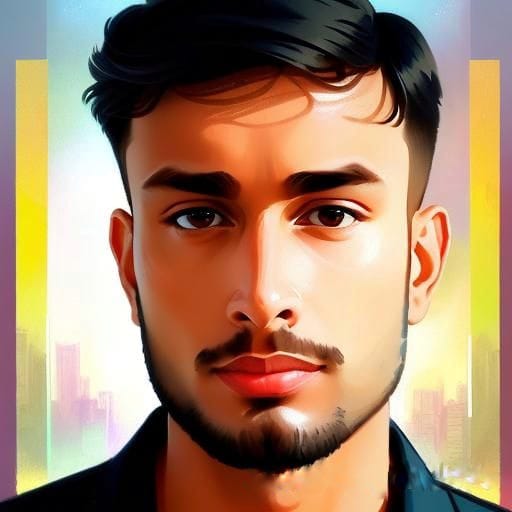
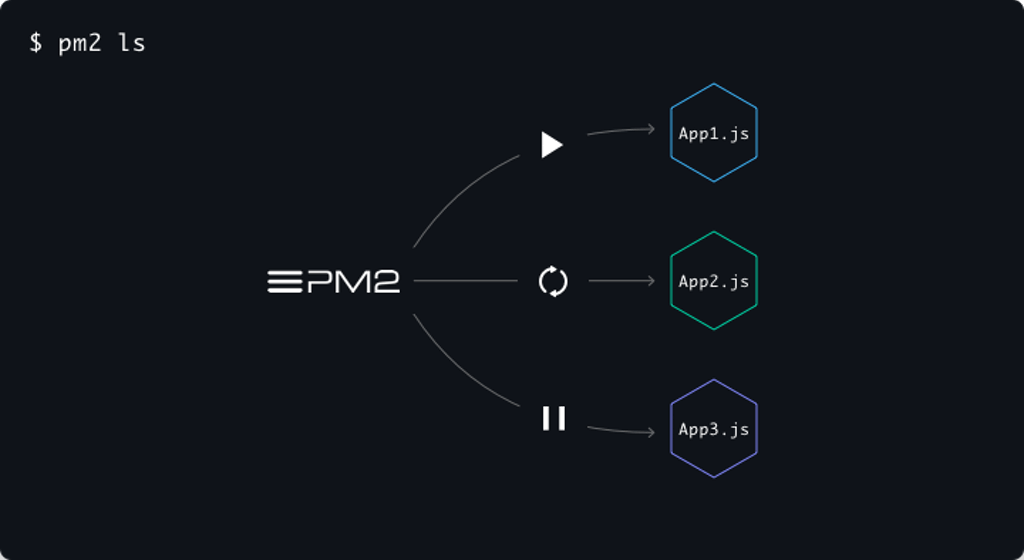
As deployment is an important part of development and every developer should know how to make their application available to the outside world.
In this blog, we're going to see how to create and deploy a nodejs http server to VPS.
Create http server in nodejs
install nodejs if not already, from nodejs website.
open terminal (powershell in windows) and navigate to your preferred directory where you want to create nodejs server.
initialize node project by running following command
npm init -y
create a index.js file in this directory and open index.js file with your favourite text editor
put below content in index.js file
const http = require("http"); http .createServer(function (req, res) { res.writeHead(200, { "Content-Type": "application/json" }); res.write(JSON.stringify({ message: "hello from server" })); res.end(); }) .listen(8080, () => console.log("Server is running on PORT: 8080"));
save the file.
Upload to github repository
make sure you have a github account and setup ssh key with your device see connect using ssh.
create new repository on github (make sure its visibility is public)
open terminal again in same directory and initialize git repository
git init
add remote url to git repository
git remote add origin <remote-ssh-url>
add, commit changes and push to github.
git add . git commit -m "http server" git push origin <branch-name>
NOTE:- you can check your git branch using command
# get git branch git branch
Create EC2 instance
make sure you have AWS account.
go to services and choose EC2 from all services
choose launch instance
fill out details
name: node server
choose OS as ubuntu
instance type: t2.micro
create a new key-pair and store it at a safe place as it will be required to login into our EC2 instance shell
create security group with allow ssh, http and https
configure storage: 10 Gb, type: gp3
click on launch instance
click on services and choose EC2 then click on instances(running)
here, you will see your newly created instance. Copy public IPv4 Address of EC2 instance
Login using SSH into it
its time to login into our instance shell, open terminal and type
ssh -i <key-pair_file> ubuntu@<IPv4_Address>
Install nodejs and pm2 package
install nodejs using nvm
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.39.3/install.sh | bash source ~/.bashrc nvm install v20.15.1
check if node is installed by running
node -v npm -v
if got version as output then node is installed
install pm2 as global package
npm install -g pm2
Deploy nodejs app
copy github clone url from github repository
create new directory nodeapp and change directory into it
mkdir nodeapp cd nodeapp
clone github repo
git clone <repo-url> cd <repo-name>
install packages (if any)
npm install
start nodejs app
pm2 start index.js --name http-server
Accessing server
open browser and go to
you will see 'site can't be reached'.
now type following command into instance terminal
curl http://localhost:8080
here, you can see our app is running.
So, what's the problem?
As you can see, we can access our app inside our EC2 instance and not outside of it as we haven't opened port 8080.
Allow listening on port from outside
open browser and go to instances section
choose (tick) your instance and you will see many configuration options below
choose security
in inbound rules, click on security groups link
tick security group from available list of security groups
configuration options will be shown at below of them
choose edit inbound rules from inbound rules section
a set of available rules will be shown, click on Add rule
fill out details for rule:
type: custom tcp
port range: 8080
source: custom
access url: 0.0.0.0/0
click on save rules
now, again try to open url into browser
Hurray!, you deployed your first nodejs app.
Remember to manage your instance. If not in use, delete it as it may incur charges.
Subscribe to my newsletter
Read articles from Ankit Yadav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
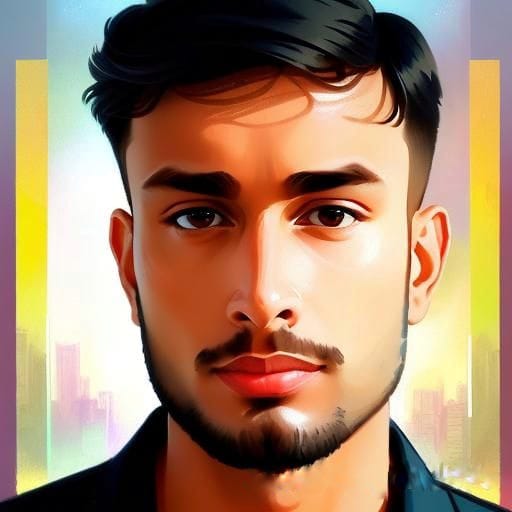
Ankit Yadav
Ankit Yadav
I am a student from India exploring about tech and its power