Introduction to PHP Arrays: What You Need to Know

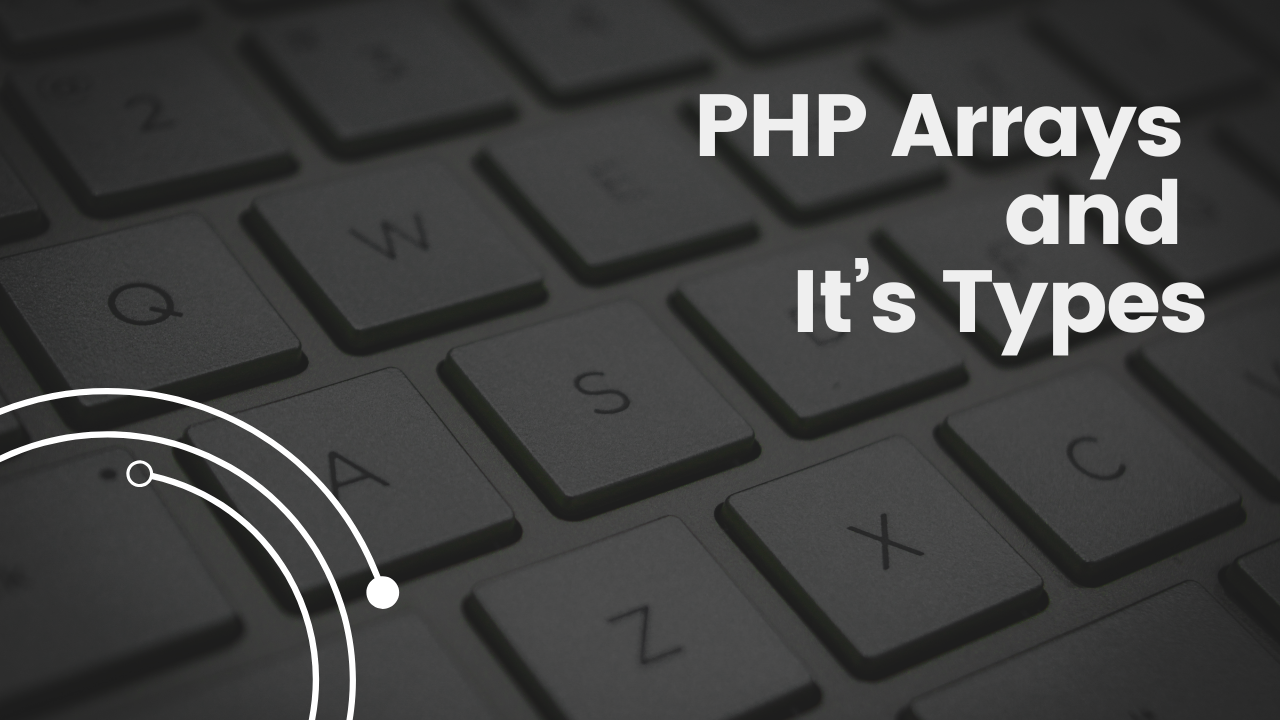
Arrays are fundamental data structures in PHP that allow you to store multiple values in a single variable. This makes them extremely useful for handling and managing data collections efficiently. In this blog, we'll delve into the definition of arrays, explore various methods to create them, discuss the best techniques for creating arrays, and provide practical examples.
Definition:
An array in PHP is a special variable that can hold more than one value at a time. Each value in an array is called an element, and each element can be accessed using an index or a key.
Creating Arrays
There are several ways to create arrays in PHP. Here are the primary methods:
1. Indexed Arrays
Indexed arrays use numeric indices to access their elements.
Method 1: Using array()
Function
<?php
// Creating Indexed Array
$fruits = array("Apple", "Banana", "Orange");
// Displaying the entire array
print_r($fruits);
echo "<br><br>";
// Displaying Array Elements
echo "First fruit: " . $fruits[0] . "<br>"; // Outputs: First fruit: Apple
echo "Second fruit: " . $fruits[1] . "<br>"; // Outputs: Second fruit: Banana
echo "Third fruit: " . $fruits[2] . "<br>"; // Outputs: Third fruit: Orange
?>
Method 2: Using Short Array Syntax (Recommended)
<?php
// Creating Indexed Array
$fruits = ["Apple", "Banana", "Orange"];
// Displaying the entire array
print_r($fruits);
echo "<br><br>";
// Displaying Array Elements
echo "First fruit: " . $fruits[0] . "<br>"; // Outputs: First fruit: Apple
echo "Second fruit: " . $fruits[1] . "<br>"; // Outputs: Second fruit: Banana
echo "Third fruit: " . $fruits[2] . "<br>"; // Outputs: Third fruit: Orange
?>
Method 3: Adding Elements Individually
<?php
// Creating Indexed Array
$fruits = [];
$fruits[] = "Apple";
$fruits[] = "Banana";
$fruits[] = "Orange";
// Displaying the entire array
print_r($fruits);
echo "<br><br>";
// Displaying Array Elements
echo "First fruit: " . $fruits[0] . "<br>"; // Outputs: First fruit: Apple
echo "Second fruit: " . $fruits[1] . "<br>"; // Outputs: Second fruit: Banana
echo "Third fruit: " . $fruits[2] . "<br>"; // Outputs: Third fruit: Orange
?>
2. Associative Arrays
Associative arrays use named keys to access their elements.
Method 1: Using array()
Function
<?php
// Creating Associative Array
$ages = array("John" => 25, "Jane" => 30, "Bob" => 35);
// Displaying the entire array
print_r($ages);
echo "<br><br>";
// Displaying Array Elements
echo "John's age: " . $ages['John'] . "<br>"; // Outputs: John's age: 25
echo "Jane's age: " . $ages['Jane'] . "<br>"; // Outputs: Jane's age: 30
echo "Bob's age: " . $ages['Bob'] . "<br>"; // Outputs: Bob's age: 35
?>
Method 2: Using Short Array Syntax (Recommended)
<?php
// Creating Associative Array
$ages = ["John" => 25, "Jane" => 30, "Bob" => 35];
// Displaying the entire array
print_r($ages);
echo "<br><br>";
// Displaying Array Elements
echo "John's age: " . $ages['John'] . "<br>"; // Outputs: John's age: 25
echo "Jane's age: " . $ages['Jane'] . "<br>"; // Outputs: Jane's age: 30
echo "Bob's age: " . $ages['Bob'] . "<br>"; // Outputs: Bob's age: 35
?>
Method 3: Adding Elements Individually
<?php
// Creating Associative Array
$ages = [];
$ages["John"] = 25;
$ages["Jane"] = 30;
$ages["Bob"] = 35;
// Displaying the entire array
print_r($ages);
echo "<br><br>";
// Displaying Array Elements
echo "John's age: " . $ages['John'] . "<br>"; // Outputs: John's age: 25
echo "Jane's age: " . $ages['Jane'] . "<br>"; // Outputs: Jane's age: 30
echo "Bob's age: " . $ages['Bob'] . "<br>"; // Outputs: Bob's age: 35
?>
3. Multidimensional Arrays
Multidimensional arrays contain one or more arrays within them.
Method 1: Using array()
Function
<?php
// Creating Multidimensional Array
$products = array(
"Electronics" => array("Laptop", "Mobile", "Tablet"),
"Furniture" => array("Sofa", "Chair", "Table")
);
// Displaying the entire array
print_r($products);
echo "<br><br>";
// Displaying a specific array element
echo $products['Electronics'][0]; // Outputs: Laptop
?>
Method 2: Using Short Array Syntax (Recommended)
<?php
// Creating Multidimensional Array
$products = [
"Electronics" => ["Laptop", "Mobile", "Tablet"],
"Furniture" => ["Sofa", "Chair", "Table"]
];
// Displaying the entire array
print_r($products);
echo "<br><br>";
// Displaying a specific array element
echo $products['Electronics'][0]; // Outputs: Laptop
?>
Method 3: Adding Elements Individually
<?php
// Creating Multidimensional Array
$products = [];
$products["Electronics"] = ["Laptop", "Mobile", "Tablet"];
$products["Furniture"] = ["Sofa", "Chair", "Table"];
// Displaying the entire array
print_r($products);
echo "<br><br>";
// Displaying a specific array element
echo $products['Electronics'][0]; // Outputs: Laptop
?>
Best Techniques for Creating Arrays
Use Associative Arrays for Key-Value Pairs: If you need to store data with a relationship between keys and values, associative arrays are ideal.
Multidimensional Arrays for Complex Data: Use multidimensional arrays for storing more complex, hierarchical data structures.
Conclusion
Arrays are versatile and powerful tools in PHP for managing collections of data. Whether you're dealing with simple lists, key-value pairs, or complex data structures, understanding how to create and manipulate arrays is crucial. By mastering arrays and their functions, you can write more efficient and maintainable PHP code.
Subscribe to my newsletter
Read articles from Saurabh Damale directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Saurabh Damale
Saurabh Damale
Hello there! ๐ I'm Saurabh Kailas Damale, a passionate Full Stack Developer with a strong background in creating dynamic and responsive web applications. I thrive on turning complex problems into elegant solutions, and I'm always eager to learn new technologies and tools to enhance my development skills.