Mastering Laravel Debugging: Understanding Query Logs

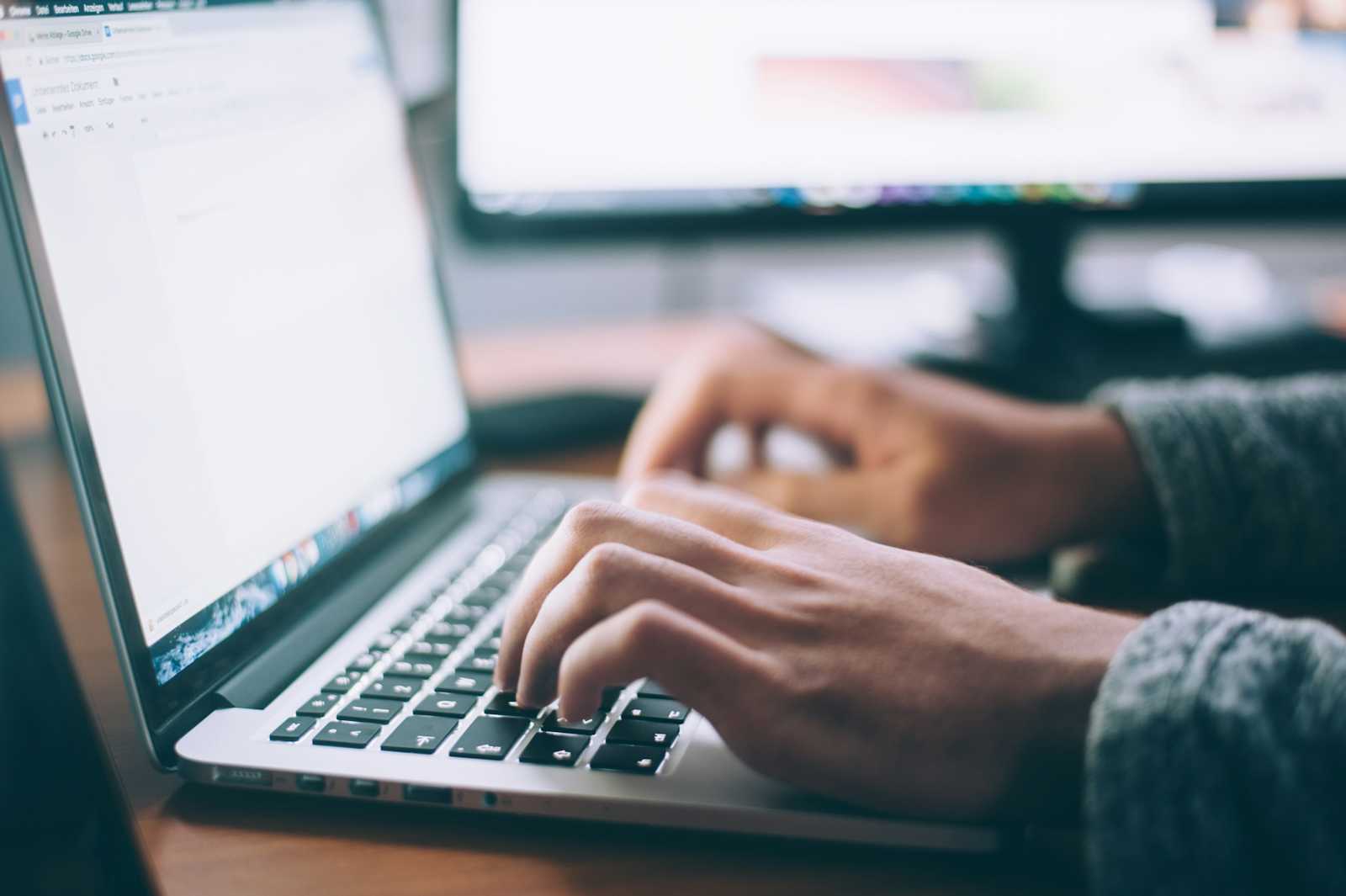
Optimizing and debugging database queries is crucial for building efficient and scalable applications. Laravel makes this process straightforward with its robust query-logging features. Here’s a detailed guide on how to enable and inspect query logs in Laravel:
Step-by-Step Guide:
1. Enable Query Logging
First, you need to enable the query log. This command will tell Laravel to start logging all SQL queries that are executed:
DB::enableQueryLog();
2. Execute Your Query
Next, run the query you want to debug. For example, let's retrieve all records from the users
table:
User::all();
3. Retrieve and Display the Logs
Finally, retrieve the log of executed queries and display them using the dd()
function, which dumps the content and stops the script execution:
$logs = DB::getQueryLog();
dd($logs);
Example Output:
After running the above code, the output might look like this:
array:1 [
0 => array:3 [
"query" => "select * from `users`"
"bindings" => []
"time" => 1.25
]
]
Detailed Breakdown:
"query": The actual SQL query executed. In this case,
select * from users
."bindings": Any bound parameters. It's empty here because there are no parameters in our query.
"time": The execution time of the query in milliseconds. For instance, 1.25ms in this example.
Real-World Use Case:
Imagine you have a complex query that's causing performance issues. By enabling query logging, you can pinpoint the exact query and understand how long it takes to execute.
For example, you might find a query like this in your logs:
array:1 [
0 => array:3 [
"query" => "select * from `users` where `email` = ?"
"bindings" => [
0 => "example@example.com"
]
"time" => 120.45
]
]
Here, the query is searching for a user by their email, and it takes 120.45ms. This might be a sign that the email
column needs an index for faster lookups.
Optimizing the Query:
To optimize, you can add an index to the email
column in your database:
ALTER TABLE users ADD INDEX(email);
Advanced Usage:
You can also log more complex queries, like joins or subqueries. For instance:
DB::enableQueryLog();
$users = User::join('posts', 'users.id', '=', 'posts.user_id')
->where('posts.created_at', '>', now()->subDays(30))
->get();
$logs = DB::getQueryLog();
dd($logs);
This might produce a log like:
array:1 [
0 => array:3 [
"query" => "select * from `users` inner join `posts` on `users`.`id` = `posts`.`user_id` where `posts`.`created_at` > ?"
"bindings" => [
0 => "2024-06-23 12:00:00"
]
"time" => 145.67
]
]
Analyzing the Logs:
From the log, you can see:
The exact query being run.
Any bindings (parameters) being used.
The time taken to execute the query.
With this information, you can take steps to optimise your queries and improve your application's performance.
Conclusion:
Leveraging Laravel's query logging helps you gain valuable insights into your application's database interactions. This not only aids in debugging but also enhances performance optimisation efforts.
#Laravel #Debugging #WebDevelopment #DatabaseOptimization #CodeTips
Subscribe to my newsletter
Read articles from Asfia Aiman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Asfia Aiman
Asfia Aiman
Hey Hashnode community! I'm Asfia Aiman, a seasoned web developer with three years of experience. My expertise includes HTML, CSS, JavaScript, jQuery, AJAX for front-end, PHP, Bootstrap, Laravel for back-end, and MySQL for databases. I prioritize client satisfaction, delivering tailor-made solutions with a focus on quality. Currently expanding my skills with Vue.js. Let's connect and explore how I can bring my passion and experience to your projects! Reach out to discuss collaborations or learn more about my skills. Excited to build something amazing together! If you like my blogs, buy me a coffee here https://www.buymeacoffee.com/asfiaaiman