Using Ansible for Configuration Management
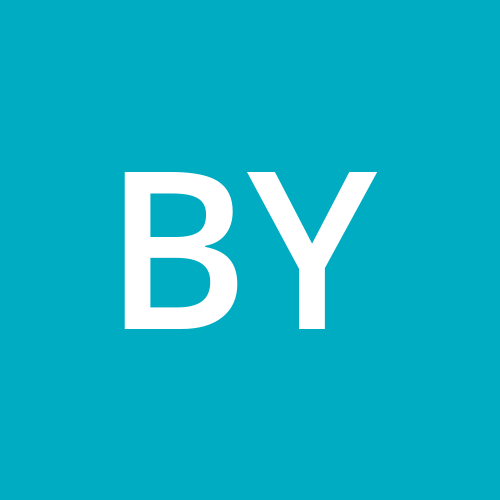
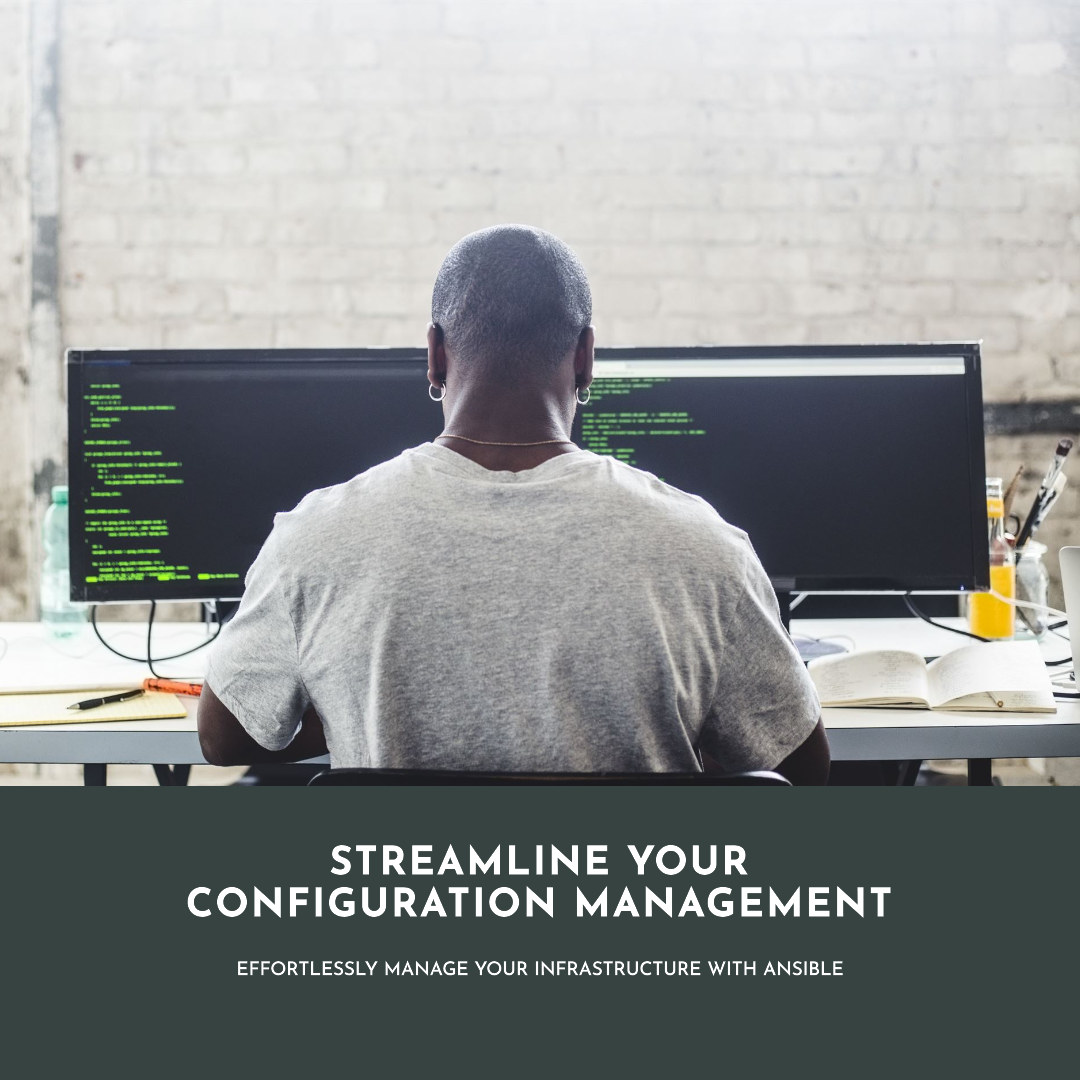
While Terraform is great for provisioning infrastructure, Ansible excels in configuration management and application deployment. Ansible is an open-source automation tool that uses simple, human-readable YAML syntax to define tasks. In this article, we’ll explore the fundamentals of Ansible, including its key concepts, how to install it, and a step-by-step guide to creating your first Ansible playbook.
Key Concepts of Ansible
Playbooks: Playbooks are Ansible’s configuration, deployment, and orchestration language. They describe a set of tasks to be executed on a group of hosts.
Inventories: Inventories are lists of managed hosts, defined in plain text files. They can be static or dynamic and group hosts logically for easier management.
Modules: Modules are reusable, standalone scripts that Ansible runs on your behalf. They perform actions such as installing packages, managing services, and copying files.
Roles: Roles provide a way to group tasks and variables into reusable units. They help organize playbooks and facilitate sharing and reuse of Ansible code.
Tasks: Tasks are the individual units of work in a playbook. They use modules to perform actions on hosts.
Installing Ansible : To get started with Ansible, you need to install it on your local machine. Follow these steps to install Ansible:
- Install Ansible: On most systems, you can install Ansible using a package manager.
# On Ubuntu
sudo apt update
sudo apt install ansible
# On macOS
brew install ansible
2. Verify Installation: Verify the installation by running the following command:
ansible --version
Your First Ansible Playbook
Let’s create a simple Ansible playbook to install and start the Nginx web server on a remote host.
- Create a Project Directory: Create a new directory for your Ansible playbook.
mkdir my-ansible-project
cd my-ansible-project
- Define the Inventory: Create an inventory file with the IP address or hostname of your remote host.
[web]
192.168.1.100 # Replace with the IP address of your remote host
- Create the Playbook: Create a file named playbook.yml and add the following content:
```yaml
---
- name: Install and start Nginx
hosts: web
become: yes
tasks:
- name: Install Nginx
apt:
name: nginx
state: present
- name: Start Nginx
service:
name: nginx
state: started
enabled: yes
- Run the Playbook: Run the playbook using the ansible-playbook command.
ansible-playbook -i inventory playbook.yml
- Verify the Installation: SSH into your remote host and verify that Nginx is installed and running.
ssh user@192.168.1.100 # Replace with your remote host's SSH details
sudo systemctl status nginx
Advanced Ansible Techniques
- Using Variables: Variables make your playbooks more flexible and reusable. Define variables in the playbook or in separate variable files.
---
- name: Install and start Nginx
hosts: web
become: yes
vars:
package_name: nginx
tasks:
- name: Install Nginx
apt:
name: "{{ package_name }}"
state: present
- name: Start Nginx
service:
name: "{{ package_name }}"
state: started
enabled: yes
2. Creating Roles: Roles help organize your playbooks into reusable components. Create a role directory structure and move tasks, variables, and files into it.
ansible-galaxy init nginx_role
- Update your playbook to use the role:
---
- name: Apply Nginx role
hosts: web
become: yes
roles:
- nginx_role
3. Handlers:
- Handlers are tasks that are triggered by other tasks using the notify directive. They are typically used for restarting services after configuration changes.
---
- name: Install and start Nginx
hosts: web
become: yes
tasks:
- name: Install Nginx
apt:
name: nginx
state: present
notify:
- Restart Nginx
handlers:
- name: Restart Nginx
service:
name: nginx
state: restarted
Best Practices for Ansible Playbooks
Idempotency: Ensure tasks are idempotent, meaning they can be run multiple times without changing the system state after the first application.
Modularity: Break down playbooks into smaller, reusable roles and tasks.
Documentation: Use comments and meaningful task names to document your playbooks.
Error Handling: Use conditionals and error handling to make playbooks robust.
Security: Avoid hardcoding sensitive information. Use Ansible Vault to encrypt secrets.
ansible-vault encrypt vars.yml
Ansible is a powerful tool for configuration management and application deployment. By understanding its key concepts and advanced techniques, you can automate complex workflows and ensure consistency across your infrastructure. Happy automating!
Subscribe to my newsletter
Read articles from Brijesh Yadav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
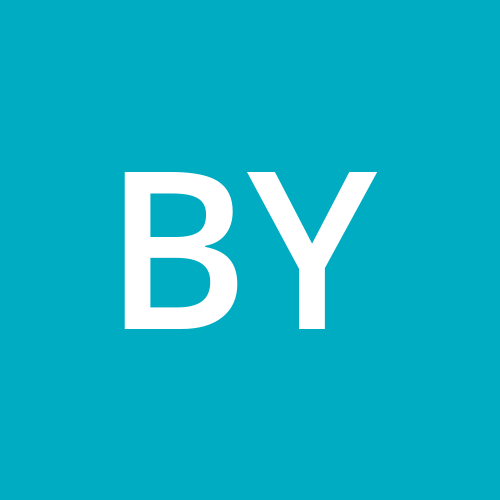