Java Heaps Explained: What You Need to Know

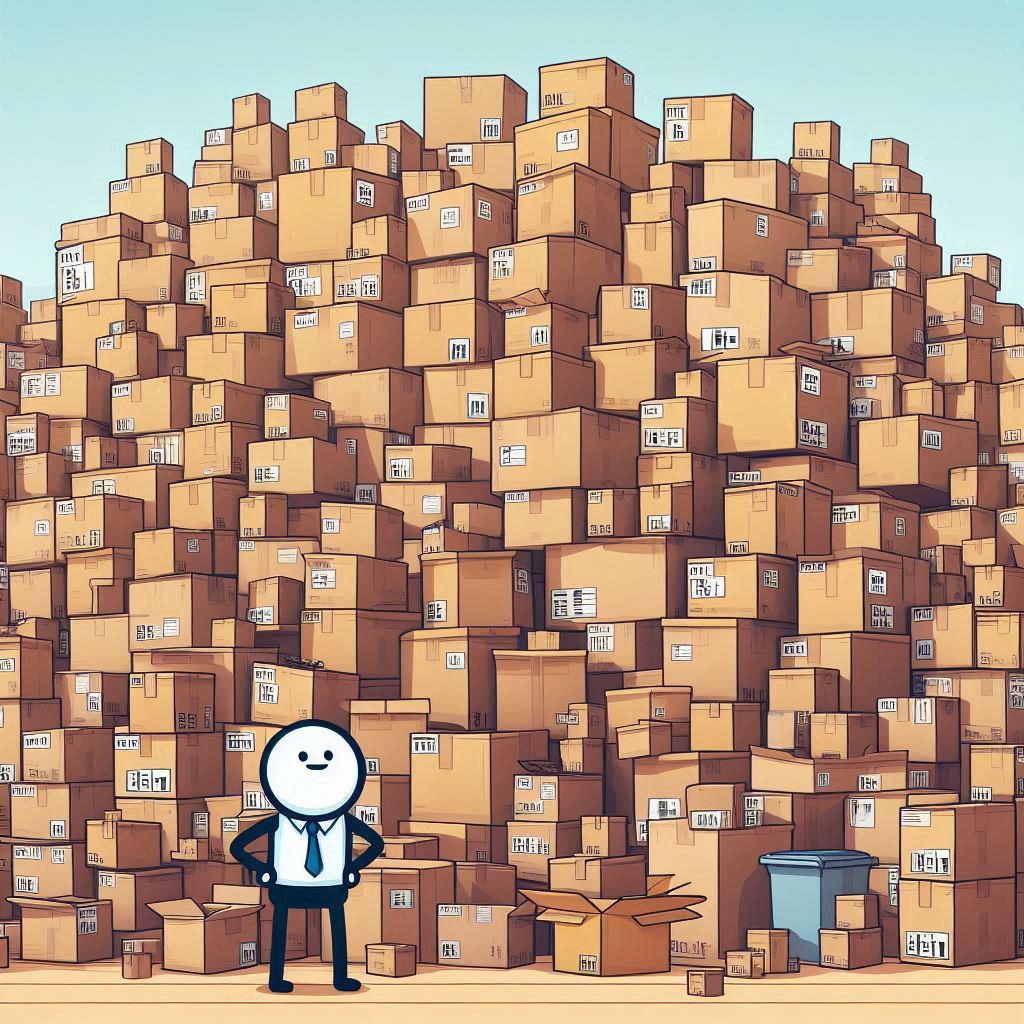
Table of Contents
Introduction to Memory in Java
Definition of Heap
What Does the Heap Store?
What Does the Stack Store?
Initial and Maximum Heap Size Parameters
Relation Between Heap Size and Garbage Collection
Performance optimizing
References
1. Introduction to Memory in Java
Java applications run inside a Java Virtual Machine (JVM) that manages memory efficiently and automatically. Memory management is crucial in Java as it directly affects the performance and stability of applications. The JVM divides memory into two main parts: the heap and the stack. Each of these memory areas serves a distinct purpose and works in tandem to ensure the smooth operation of Java applications.
2. Definition of Heap
The heap is a region of memory used for dynamic memory allocation where Java objects and instance variables are stored. Unlike the stack, which follows a Last In, First Out (LIFO) structure, the heap is more flexible and allows for more complex memory management operations.
3. What Does the Heap Store?
The heap stores:
Instance Variables: These are non-static variables defined within a class but outside any method, constructor, or block. Each object created in Java has its own copy of instance variables.
Objects: Whenever you create an object using the
new
keyword, it is allocated memory on the heap.
Example:
public class MyClass {
int instanceVariable; // Stored in the heap
public MyClass() {
instanceVariable = 10;
}
public static void main(String[] args) {
MyClass obj = new MyClass(); // Reference 'obj' is stored in the stack, the actual object is in the heap
}
}
In contrast to the stack, which is used for short-lived, method-specific storage, the heap is used for long-lived data and objects that need to be accessed globally within the application.
4. What Does the Stack Store?
The stack is a smaller region of memory compared to the heap and is used for:
Methods: Each method call creates a new frame in the stack. This frame contains method-specific data including parameters, local variables, and the return address.
Local Variables: Variables declared inside a method, including method parameters, are stored on the stack.
Example:
javaCopy codepublic class StackExample {
public static void main(String[] args) {
int localVar = 5; // Stored in the stack
method1(localVar);
}
public static void method1(int param) {
int methodLocalVar = param + 10; // Stored in the stack
}
}
The stack is highly efficient for method execution because it operates in a LIFO manner, allowing quick allocation and deallocation of memory as methods are called and returned.
5. Initial and Maximum Heap Size Parameters
The JVM allows you to set the initial and maximum heap size to control memory allocation. These parameters can be configured using command-line options:
Initial Heap Size (-Xms): This parameter sets the initial size of the heap memory. For example,
-Xms512m
sets the initial heap size to 512 megabytes.Maximum Heap Size (-Xmx): This parameter sets the maximum size to which the heap can grow. For instance,
-Xmx1024m
limits the heap size to 1024 megabytes.
Setting these parameters helps in tuning the performance of the JVM. The initial heap size ensures that the JVM starts with a sufficient amount of memory, while the maximum heap size prevents the application from consuming too much memory, which could affect system stability.
6. Relation Between Heap Size and Garbage Collection
Garbage Collection (GC) is the process by which the JVM reclaims memory occupied by objects that are no longer in use. The heap size directly impacts the frequency and duration of garbage collection:
Small Heap Size: With a smaller heap, garbage collection occurs more frequently because the JVM needs to free up memory to accommodate new objects. Frequent GC can affect performance due to the overhead of the collection process.
Large Heap Size: A larger heap reduces the frequency of GC because there is more space available for object allocation. However, when GC does occur, it takes longer to complete because it has to scan and manage a larger area of memory.
7. Performance optimizing
To optimize performance, the Garbage Collector aims to keep heap occupancy between 40% and 70%. Here's why:
Heap Occupancy Greater Than 70%: When heap occupancy exceeds 70%, the JVM triggers more frequent GC cycles to reclaim memory. These frequent GC cycles can degrade performance due to the constant interruption of application execution to perform memory cleanup. You can adjust this behavior by setting the
-Xminf
option, which specifies the minimum free space ratio.Heap Occupancy Less Than 40%: When heap occupancy is below 40%, GC cycles are less frequent, which might seem beneficial at first glance. However, these infrequent GC cycles tend to be longer because a larger amount of memory needs to be scanned and compacted. This can result in longer pause times, negatively impacting application performance. The
-Xmaxf
option allows you to adjust the maximum free space ratio, influencing how the JVM handles heap occupancy.
Optimizing the heap size and configuring these parameters help in achieving a balance where the application runs efficiently without excessive GC overhead. By understanding and tuning the heap size and GC behavior, developers can ensure their Java applications perform optimally under various conditions.
8. References
Subscribe to my newsletter
Read articles from Omar Mohamed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
