Stack Memory and Heap Memory in Javascript
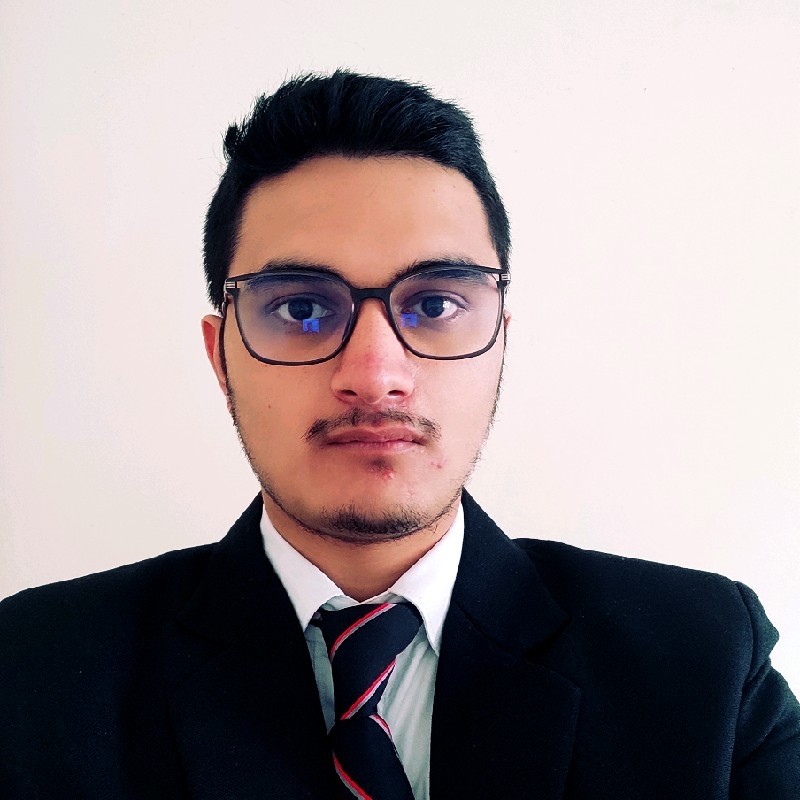
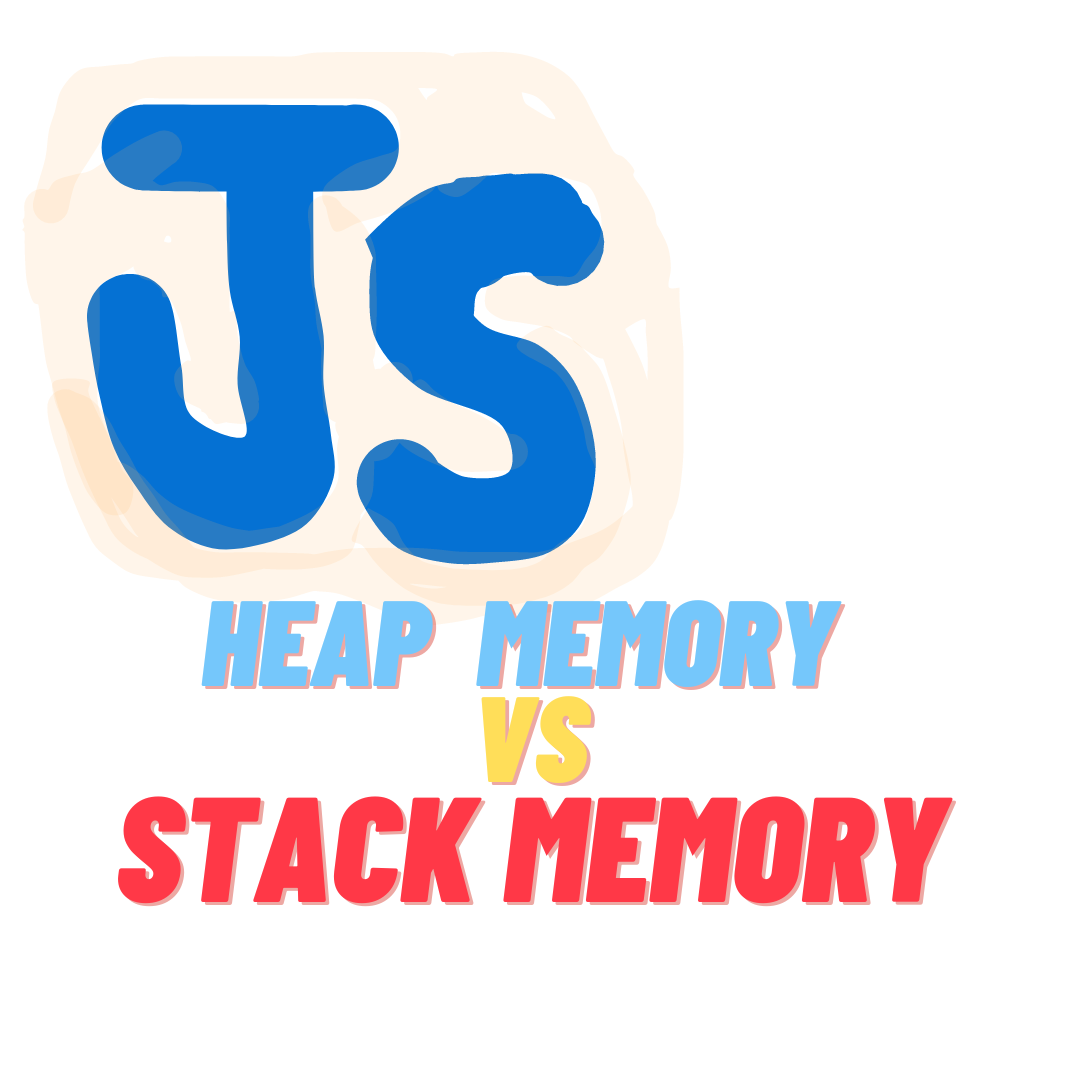
In JavaScript, stack and heap memory are used to manage the allocation and deallocation of memory for variables, objects, and functions. Here's a concise overview:
Stack Memory
Characteristics:
Fast Access: Memory allocation and deallocation follow the Last In, First Out (LIFO) principle.
Fixed Size: Typically limited in size.
Automatic Management: Managed by the JavaScript engine, automatically handling memory for function calls, variables, and execution context.
Use Cases:
Primitive Data Types: Stores variables of primitive data types (e.g.,
number
,string
,boolean
).Function Calls: Stores function arguments and local variables.
Execution Context: Keeps track of function calls and their local execution contexts.
Heap Memory
Characteristics:
Dynamic Allocation: Memory can be allocated and freed at any time.
Larger Size: Typically larger than the stack.
Manual Management: Requires garbage collection to manage memory allocation and deallocation.
Use Cases:
Objects: Stores objects, arrays, and functions.
Complex Data Types: Handles larger and more complex data structures.
Persistent Data: Keeps data that needs to persist beyond the scope of a single function call.
Example
javascriptCopy codefunction example() {
// Primitive data stored in stack
let num = 42;
let str = "Hello, World!";
// Object stored in heap
let obj = {
key: "value"
};
// Function stored in heap
let func = function() {
console.log("Function in heap");
};
}
example();
In this example, num
and str
are stored in the stack, while obj
and func
are stored in the heap. This distinction helps optimize memory usage and access times in JavaScript.
Subscribe to my newsletter
Read articles from Neelesh Joshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
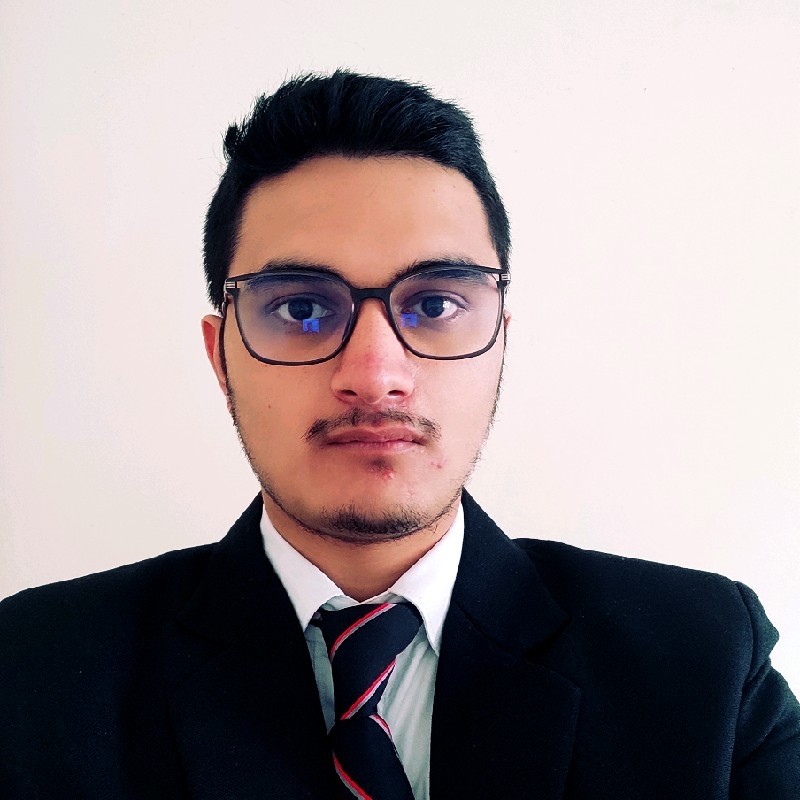
Neelesh Joshi
Neelesh Joshi
Hi, am a passionate programmer and front-end web developer I am interested in problem solving