Javascript String methods
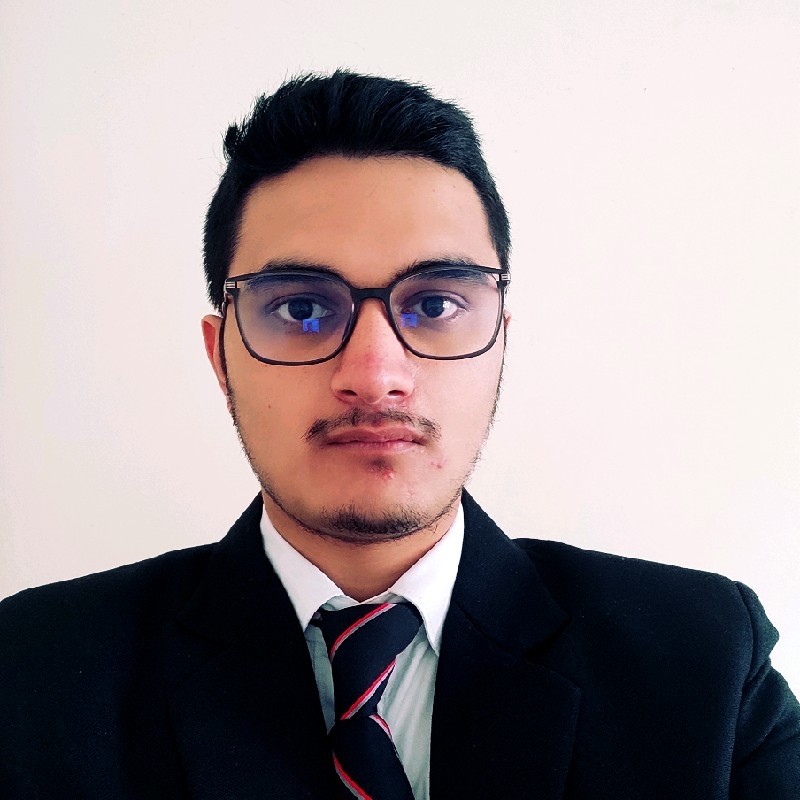
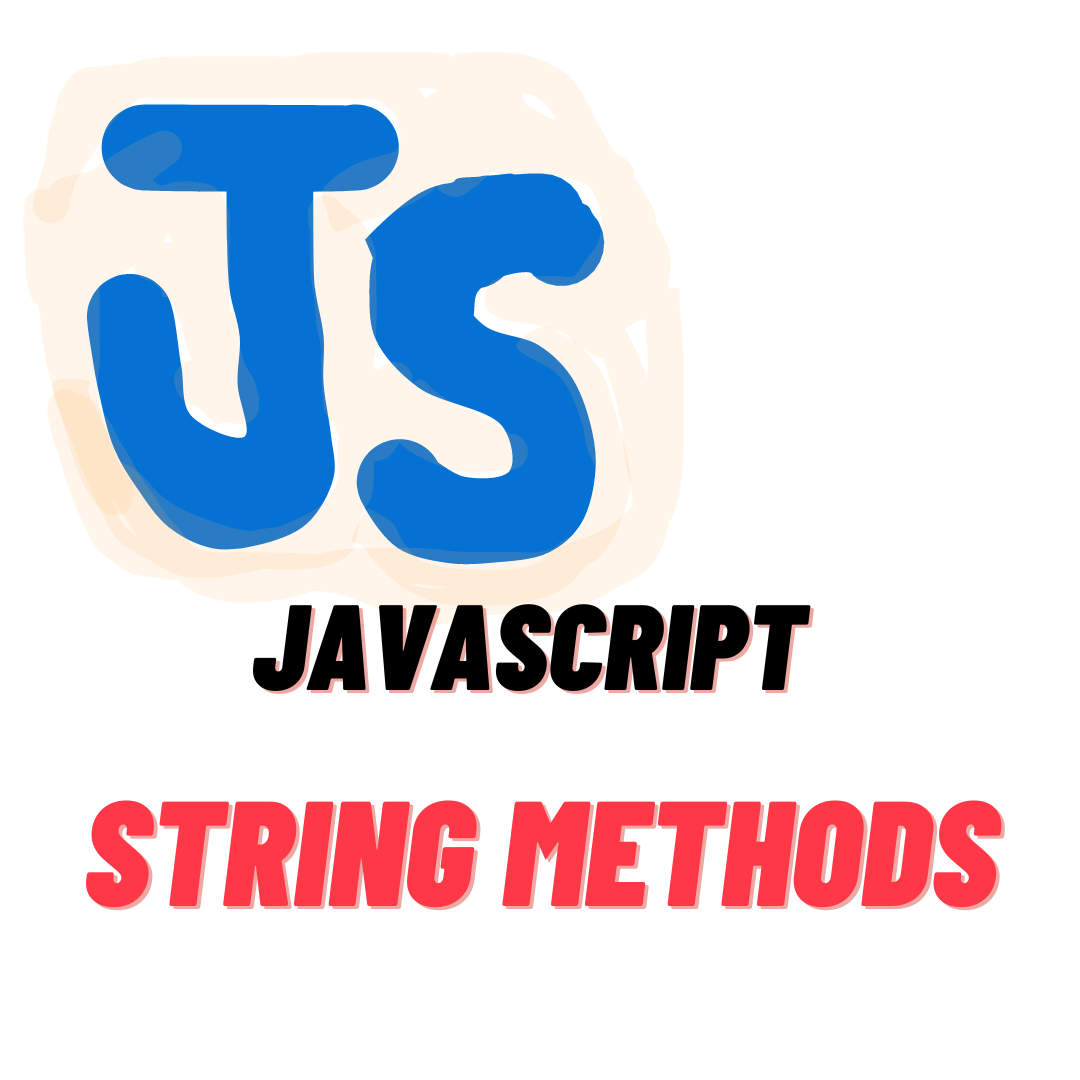
JavaScript provides a variety of methods for manipulating and working with strings. Here is a comprehensive list of the commonly used string methods:
String Creation Methods
String()
: Creates a new String object.
Character Methods
charAt(index)
: Returns the character at the specified index.charCodeAt(index)
: Returns the Unicode of the character at the specified index.codePointAt(index)
: Returns the Unicode code point of the character at the specified index.
String Manipulation Methods
concat(string1, string2, ...)
: Joins two or more strings and returns a new string.includes(searchString, position)
: Determines whether a string contains the characters of a specified string.endsWith(searchString, length)
: Determines whether a string ends with the characters of a specified string.startsWith(searchString, position)
: Determines whether a string begins with the characters of a specified string.indexOf(searchValue, fromIndex)
: Returns the index of the first occurrence of a specified text in a string.lastIndexOf(searchValue, fromIndex)
: Returns the index of the last occurrence of a specified text in a string.match(regex)
: Searches a string for a match against a regular expression and returns the matches.matchAll(regex)
: Returns an iterator of all results matching a string against a regular expression.replace(searchValue, newValue)
: Replaces a specified value with another value in a string.replaceAll(searchValue, newValue)
: Replaces all occurrences of a specified value with another value in a string.slice(start, end)
: Extracts a part of a string and returns a new string.split(separator, limit)
: Splits a string into an array of substrings.substring(start, end)
: Extracts characters from a string, between two specified indices.toLowerCase()
: Converts a string to lowercase letters.toUpperCase()
: Converts a string to uppercase letters.trim()
: Removes whitespace from both ends of a string.trimStart()
ortrimLeft()
: Removes whitespace from the beginning of a string.trimEnd()
ortrimRight()
: Removes whitespace from the end of a string.
String Conversion Methods
toString()
: Returns the value of a String object.valueOf()
: Returns the primitive value of a String object.
Locale-Sensitive Methods
localeCompare(compareString)
: Compares two strings in the current locale.toLocaleLowerCase(locale)
: The characters within a string are converted to lowercase while respecting the current locale.toLocaleUpperCase(locale)
: The characters within a string are converted to uppercase while respecting the current locale.
String Padding Methods
padStart(targetLength, padString)
: Pads the current string from the start with a given string until the resulting string reaches the given length.padEnd(targetLength, padString)
: Pads the current string from the end with a given string until the resulting string reaches the given length.
Raw String Methods
String.raw()
: A tag function of template literals, it returns a string created from a raw template string.
Example Usage
let str = "Hello, World!";
console.log(str.charAt(0)); // "H"
console.log(str.charCodeAt(0)); // 72
console.log(str.concat(" How are you?")); // "Hello, World! How are you?"
console.log(str.includes("World")); // true
console.log(str.endsWith("!")); // true
console.log(str.startsWith("Hello")); // true
console.log(str.indexOf("o")); // 4
console.log(str.lastIndexOf("o")); // 8
console.log(str.match(/o/g)); // ["o", "o"]
console.log(str.replace("World", "Universe")); // "Hello, Universe!"
console.log(str.slice(0, 5)); // "Hello"
console.log(str.split(", ")); // ["Hello", "World!"]
console.log(str.substring(0, 5)); // "Hello"
console.log(str.toLowerCase()); // "hello, world!"
console.log(str.toUpperCase()); // "HELLO, WORLD!"
console.log(str.trim()); // "Hello, World!"
console.log(str.padStart(15, "*")); // "**Hello, World!"
console.log(str.padEnd(15, "*")); // "Hello, World!**"
These methods provide a powerful toolkit for string manipulation and are essential for effective JavaScript programming.
Subscribe to my newsletter
Read articles from Neelesh Joshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
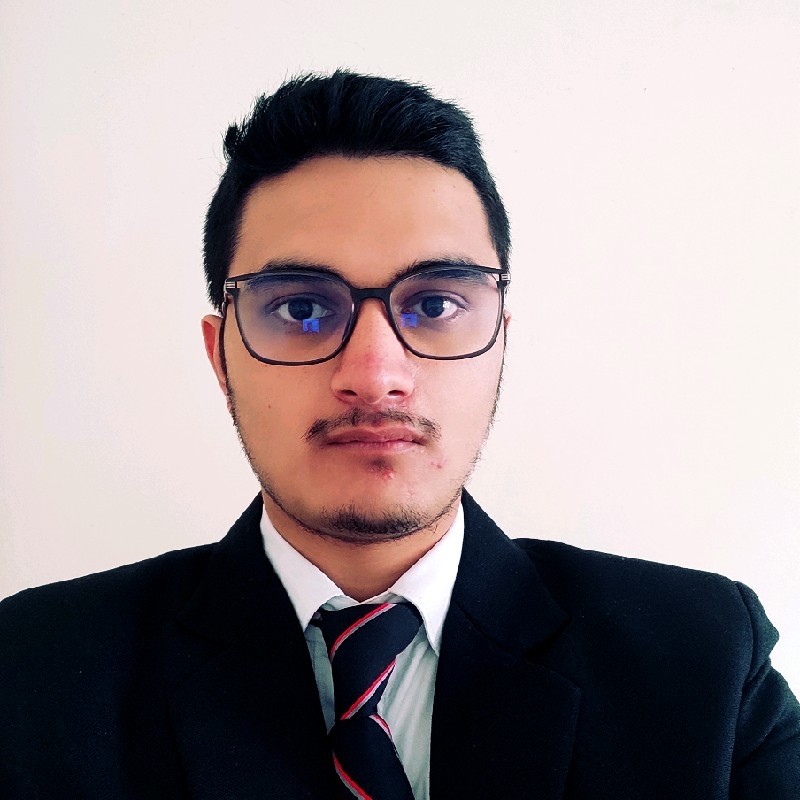
Neelesh Joshi
Neelesh Joshi
Hi, am a passionate programmer and front-end web developer I am interested in problem solving