🚀Day 21/180 Find all factorial numbers less than equal to n …
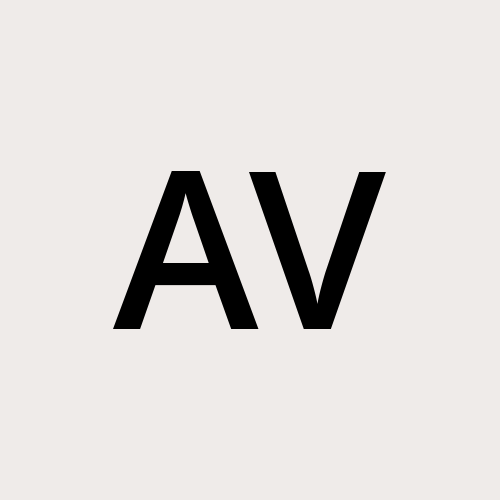
Find all factorial numbers less than equal to n …
public class Main {
public static void fact(int N,int fact,int i){
i++;
if (fact>N){
return;
}
System.out.print(fact+" ");
fact(N,fact*i,i);
}
public static void main(String args[]){
fact(100,1,1);
}
}
/*
* PS C:\Users\HP\OneDrive\Coding\Java\Practice01> javac Main.java
PS C:\Users\HP\OneDrive\Coding\Java\Practice01> java Main
1 2 6 24
*/
The provided Java code defines a recursive method fact
to compute and print the sequence of factorials until a given value ( N ). Here's an explanation of how the code works and why it produces the output 1 2 6 24
for N = 100
:
Code Explanation
Method
fact
:N
: The upper limit for the factorial sequence.fact
: The current factorial value.i
: The current multiplier.
The method performs the following steps:
Increments
i
.Checks if
fact
is greater thanN
. If true, it stops the recursion.Prints the current
fact
value.Recursively calls itself with updated values:
fact
multiplied byi
, and the incrementedi
.
Main Method:
- Calls the
fact
method with initial values:N = 100
,fact = 1
, andi = 1
.
- Calls the
Dry Run
Here's a step-by-step dry run of the code:
Initial call:
fact(100, 1, 1)
i = 1 + 1 = 2
fact = 1
1 <= 100
(true)Prints
1
Recursively calls
fact(100, 1 * 2, 2)
Second call:
fact(100, 2, 2)
i = 2 + 1 = 3
fact = 2
2 <= 100
(true)Prints
2
Recursively calls
fact(100, 2 * 3, 3)
Third call:
fact(100, 6, 3)
i = 3 + 1 = 4
fact = 6
6 <= 100
(true)Prints
6
Recursively calls
fact(100, 6 * 4, 4)
Fourth call:
fact(100, 24, 4)
i = 4 + 1 = 5
fact = 24
24 <= 100
(true)Prints
24
Recursively calls
fact(100, 24 * 5, 5)
Fifth call:
fact(100, 120, 5)
i = 5 + 1 = 6
fact = 120
120 > 100
(true)Returns without printing.
The recursion stops here as fact
exceeds N
. Hence, the printed output is 1 2 6 24
.
Output
1 2 6 24
The code correctly computes and prints the factorials 1!
, 2!
, 3!
, and 4!
that are less than or equal to 100
.
Subscribe to my newsletter
Read articles from Aniket Verma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
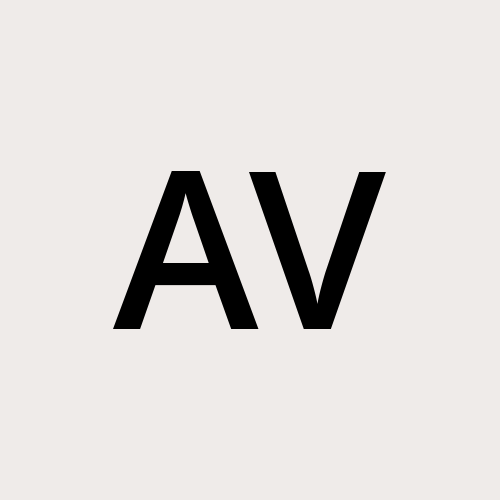