How to Check Palindromes in JavaScript: A Simple yet Effective Function
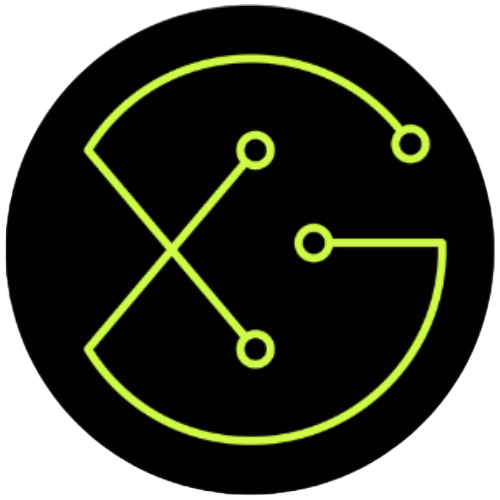
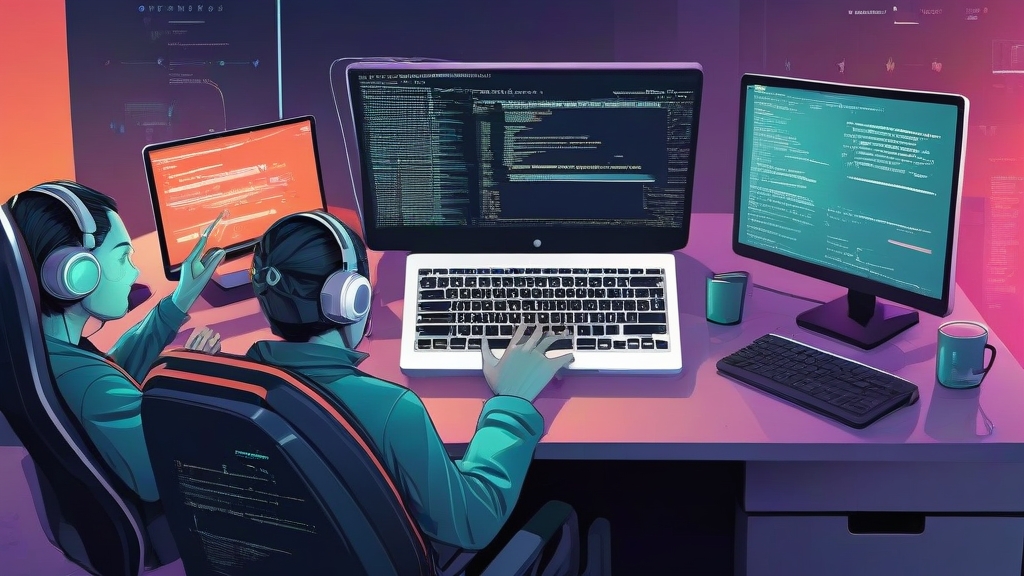
Are you interested in learning about palindromes? Perhaps you're working on a project that requires you to check if a given string is a palindrome. In this blog post, we'll explore how to create a simple JavaScript function to do just that.
What are Palindromes?
Are you interested in learning about palindromes? Perhaps you're working on a project that requires you to check if a given string is a palindrome. In this blog post, we'll explore how to create a simple JavaScript function to do just that.
Creating a JavaScript Function for Palindrome Check
To create a function in JavaScript that checks if a string is a palindrome, we can follow these steps:
Convert the input string to lowercase.
Split the string into an array of individual characters.
Reverse the character array.
Join the reversed array back into a string.
Compare the original string with the reversed string.
Here's how you could implement this in JavaScript:
function isPalindrome(str) {
str = str.toLowerCase();
return str === str.split('').reverse().join('');
}
Example Usage
Let's test our function by checking if some common palindromes and non-palindromes are indeed palindromes.
console.log(isPalindrome('radar')); // true
console.log(isPalindrome('hello')); // false
As you can see, the isPalindrome
function correctly identifies whether a given string is a palindrome or not.
Conclusion
Creating a JavaScript function to check palindromes is a straightforward process. By converting strings to lowercase and comparing them with their reversed versions, we can determine if they are palindromes. This guide has shown you how to implement such a function in your own code. Happy coding!
This is my first blog so do give me feedback :)
Subscribe to my newsletter
Read articles from Imran Mohsin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
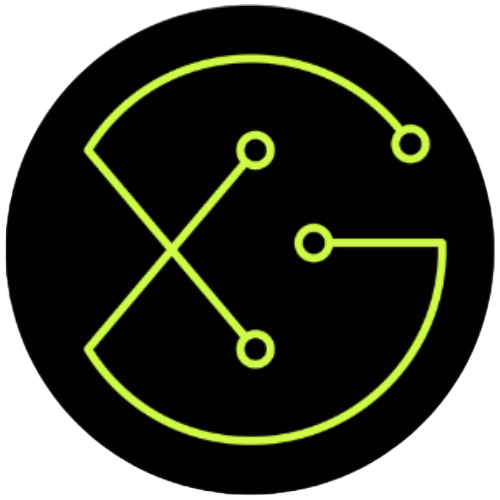
Imran Mohsin
Imran Mohsin
Just a tech nerd vibing and dropping knowledge from the internet for all my fellow geeks out there. Let's geek out together! ๐๐ป