Aligning 3D Views

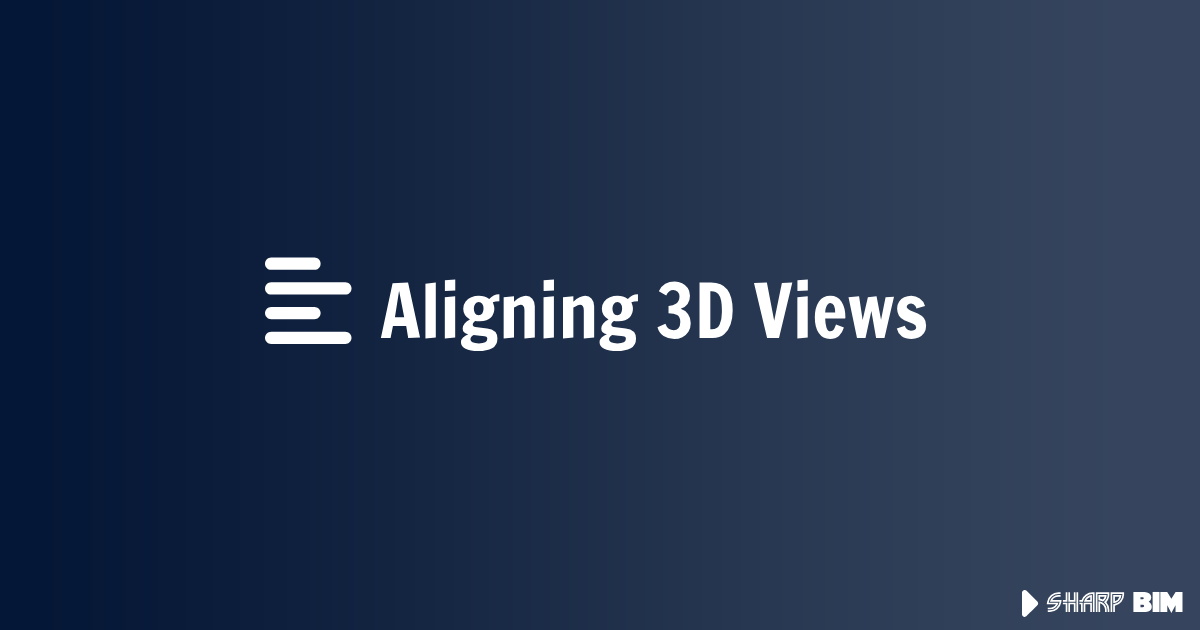
Aligning a 3D view to a specific orientation is incredibly useful during the design process. It provides better visual clarity and can help in understanding the spatial arrangement of elements. In Revit, this can be done through the user interface by right-clicking the view cube and selecting "Orient to View" or "Orient to Direction". But how can we achieve this programmatically using the Revit API? Let’s delve into this.
Understanding View3DOrientation
In the Revit API, the View3D
class contains a crucial member called View3DOrientation
. This class stores the directions of a view. Every view in Revit is defined by three vectors:
Up Direction: Corresponds to the Y direction.
Right Direction: Corresponds to the X direction.
View Direction: Corresponds to the depth direction.
By retrieving these three vector values from one view and applying them to another 3D view, we can reorient the view to match the desired orientation.
Implementing the Orientation Change
Here’s a step-by-step guide on how to reorient a 3D view using the Revit API:
Retrieve the Target View: Obtain the target view from which you want to copy the orientation.
Get the Active 3D View: This is the view you want to reorient.
Create a ViewOrientation3D Object: This object will store the orientation of the target view.
Apply the Orientation: Use a transaction to apply the orientation to the active 3D view.
Update All Open Views: Refresh the views to reflect the changes.
Code Implementation
Below is a sample code implementation in C# that demonstrates how to reorient a 3D view in Revit:
View targetView = Doc.GetElement(new ElementId(29154)) as View;
View3D v3d = UiDoc.ActiveGraphicalView as View3D;
var ori = new ViewOrientation3D(
targetView.Origin,
targetView.UpDirection,
targetView.ViewDirection
);
using (var trans = new Transaction(Doc, "Orient View"))
{
trans.Start();
v3d.SetOrientation(ori);
trans.Commit();
}
UiDoc.UpdateAllOpenViews();
Aligning to an Element Face
In addition to aligning a 3D view to another view, you can also align a 3D view to any element face. To do this, you need to extract the necessary directional values from the face of the element:
Right Direction: The X vector of the face.
Up Direction: The Y vector of the face.
View Direction: The normal vector of the face.
Here’s how you can achieve this:
Retrieve the Face: Get the face of the element you want to align to.
Extract Directional Values: Get the right, up, and view directions from the face.
Create a ViewOrientation3D Object: Use these values to create the orientation object.
Apply the Orientation: Set the orientation of the 3D view.
Code Implementation for Element Face Alignment
// Assuming 'face' is an instance of Autodesk.Revit.DB.Face
XYZ upDirection = face.YVector;
XYZ viewDirection = face.FaceNormal;
// Assuming 'origin' is a point on the face
XYZ origin = face.Evaluate(new UV(0.5, 0.5)); // Midpoint of the face as origin
var ori = new ViewOrientation3D(origin, upDirection, viewDirection);
using (var trans = new Transaction(Doc, "Align to Face"))
{
trans.Start();
v3d.SetOrientation(ori);
trans.Commit();
}
UiDoc.UpdateAllOpenViews();
Explanation
Retrieve Target View:
View targetView = Doc.GetElement(new ElementId(29154)) as View;
- This line retrieves the view with the specified ElementId. Replace
29154
with the actual ElementId of your target view.
- This line retrieves the view with the specified ElementId. Replace
Get Active 3D View:
View3D v3d = UiDoc.ActiveGraphicalView as View3D;
- This line retrieves the currently active 3D view.
Create ViewOrientation3D Object:
var ori = new ViewOrientation3D(targetView.Origin, targetView.UpDirection, targetView.ViewDirection);
- This line creates a
ViewOrientation3D
object using the origin, up direction, and view direction of the target view.
- This line creates a
Transaction to Apply Orientation:
using (var trans = new Transaction(Doc, "Orient View")) { trans.Start(); v3d.SetOrientation(ori); trans.Commit(); }
- This block of code starts a transaction, sets the orientation of the active 3D view to match the target view, and then commits the transaction.
Update Views:
UiDoc.UpdateAllOpenViews();
- This line refreshes all open views to reflect the changes.
Conclusion
Aligning 3D views to a specific orientation or matching another view can significantly enhance your workflow in Revit. By utilizing the View3DOrientation
class in the Revit API, you can programmatically achieve precise view alignments, facilitating a more efficient and visually clear design process. This approach is not only powerful but also relatively straightforward to implement. Additionally, aligning a 3D view to an element face by extracting the face’s directional values provides even greater flexibility and precision in viewing specific parts of your model.
Subscribe to my newsletter
Read articles from Moustafa Khalil directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Moustafa Khalil
Moustafa Khalil
I'm Moustafa Khalil El-Sayed, an Egyptian native with a passion for architecture and technology. After completing high school in 1999, I embarked on my journey into architecture, earning my Bachelor's degree in Architecture in 2004. My academic pursuits continued, and I later acquired my Master's degree in Architecture from Wings University. However, my thirst for knowledge extended beyond architectural design, leading me to pursue a degree in Computer Science as well. Driven by a desire to innovate, I began self-teaching computer science, which later empowered me to achieve my Associate degree in Computer Science from the University of the People more easily. Armed with dual expertise in architecture and computer science, I've cultivated a unique skill set that blends creativity with technical acumen. My career has taken me across borders, from Egypt to Oman and the UAE, where I've honed my skills as an architect. As a graduate architect and a proficient .NET BIM Solution Developer, I specialize in translating unique visions into tangible realities. My role extends beyond conventional architectural practices; I leverage technology to streamline processes and enhance project outcomes. My journey into programming began with developing script-based Excel tools to augment project management efforts, notably during the Sharm El Sheikh development. Over time, I expanded my programming prowess to address practical challenges encountered in bridging Revit and AutoCAD within my professional sphere. Several of my applications, born from this evolution, have gained recognition, with some even being featured on the Autodesk website. I am committed to pushing boundaries and continually enriching the intersection of architecture and technology, ensuring that my clients benefit from cutting-edge solutions tailored to their needs.