Must-Know Features in Next.js 14: Top 20 Highlights
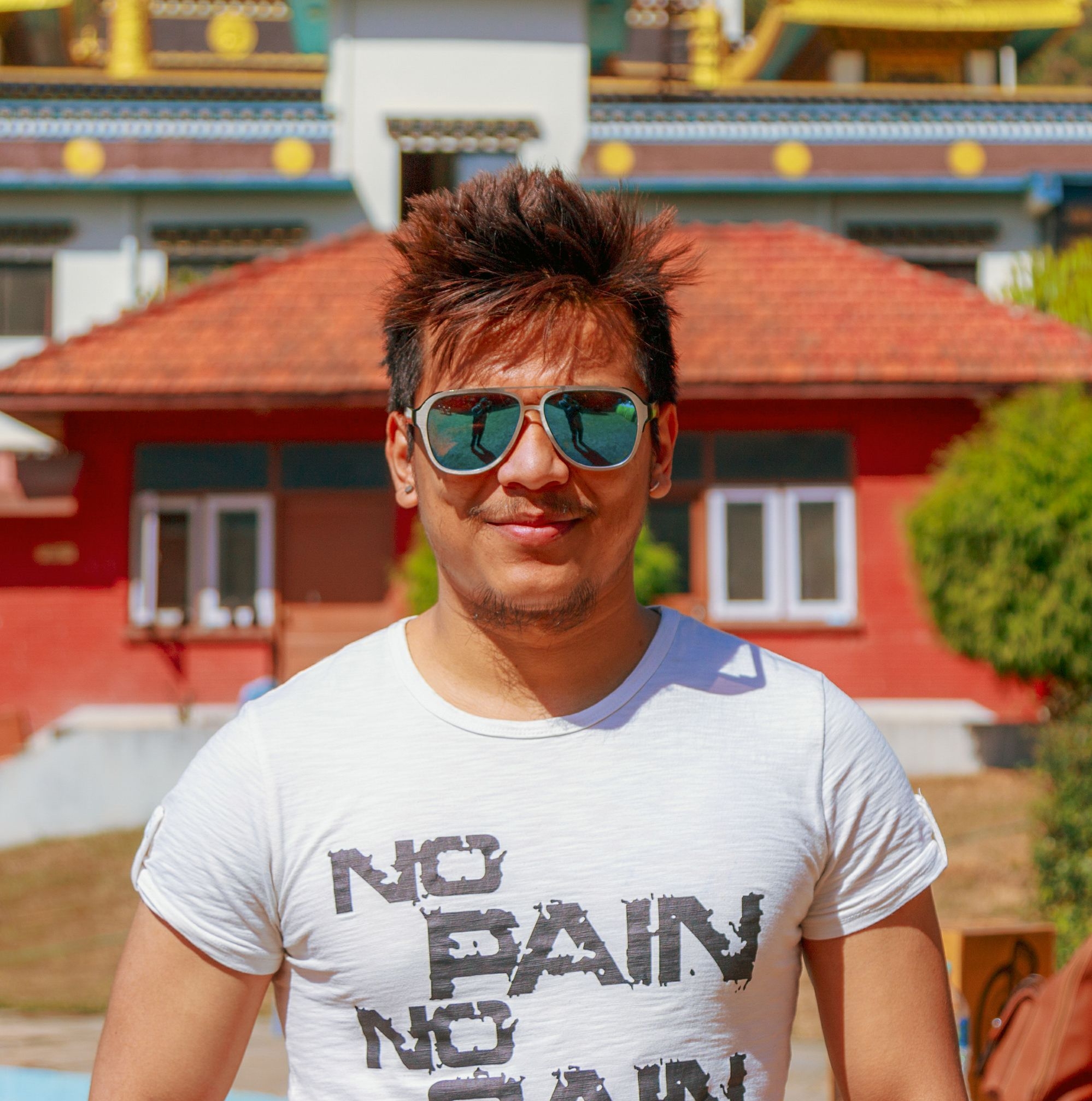
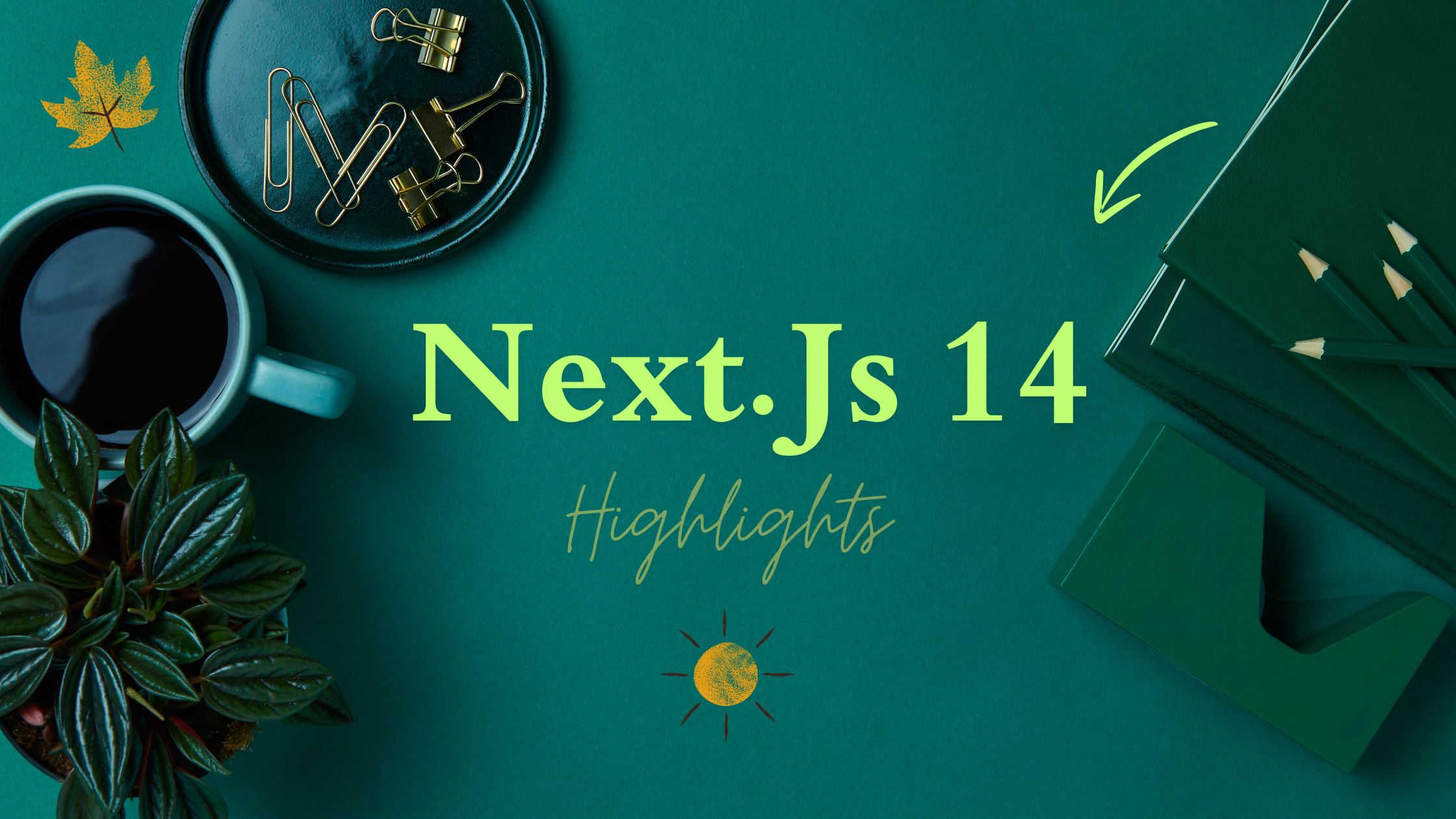
Next.js 14, released in October 2023, introduces many powerful features and improvements to the popular React framework. This version aims to enhance performance, developer experience, and application architecture. In this blog post, we'll explore the top 20 features that make Next.js 14 a game-changer for web development.
1. Turbopack (Beta): Lightning-Fast Development
Turbopack, now in beta, is a Rust-based bundler that greatly improves development speed. It provides updates up to 700 times faster than webpack, leading to almost instant hot module replacement (HMR).
// next.config.js
module.exports = {
experimental: {
turbo: {
loaders: {
// Add custom loaders here
},
},
},
}
By enabling Turbopack, you'll enjoy faster build times and more responsive development servers.
2. Server Actions: Simplified Form Handling
Server Actions let you manage form submissions and data changes directly on the server, often removing the need for API routes.
// app/submit-form.js
'use server'
export async function submitForm(formData) {
const name = formData.get('name')
const email = formData.get('email')
// Process the form data on the server
// ...
}
// app/page.js
import { submitForm } from './submit-form'
export default function Form() {
return (
<form action={submitForm}>
<input type="text" name="name" />
<input type="email" name="email" />
<button type="submit">Submit</button>
</form>
)
}
This feature simplifies form handling and reduces client-side JavaScript, improving performance and security.
3. Partial Pre-rendering (Preview)
Partial Pre-rendering lets you mix static and dynamic content on one page, optimizing both performance and data freshness.
import { unstable_noStore as noStore } from 'next/cache'
export default async function Page() {
noStore()
const data = await fetchData()
return <div>{data}</div>
}
This feature is particularly useful for pages with a mix of static and dynamic content, allowing you to serve static content immediately while loading dynamic parts asynchronously.
4. Improved Image Component
The Next.js Image component now supports native lazy loading and automatic srcset generation, improving image loading and performance.
import Image from 'next/image'
export default function MyComponent() {
return (
<Image
src="/my-image.jpg"
width={500}
height={300}
alt="Description"
sizes="(max-width: 768px) 100vw, (max-width: 1200px) 50vw, 33vw"
/>
)
}
This improvement makes it easier to create responsive and high-performance image-heavy applications with minimal effort.
5. App Router: Enhanced Routing and Layouts
The App Router, introduced in version 13 and improved in version 14, offers a more intuitive way to organize your application with nested layouts and better routing.
app/
├─ layout.js
├─ page.js
├─ about/
│ ├─ layout.js
│ └─ page.js
└─ blog/
├─ layout.js
└─ [slug]/
└─ page.js
This structure allows for better code organization and easier management of shared layouts across different parts of your application.
6. Server Components: Improved Performance
Server Components, now a stable feature in Next.js 14, let you render complex, data-fetching components on the server, which reduces the amount of JavaScript sent to the client.
// app/ServerComponent.js
async function ServerComponent() {
const data = await fetch('https://api.example.com/data')
const result = await data.json()
return <div>{result.map(item => <p key={item.id}>{item.name}</p>)}</div>
}
// app/page.js
import ServerComponent from './ServerComponent'
export default function Page() {
return (
<div>
<h1>My Page</h1>
<ServerComponent />
</div>
)
}
This approach significantly improves initial page load times and reduces the JavaScript bundle size.
7. Metadata API: Enhanced SEO
The Metadata API offers a powerful method to handle your application's SEO directly within your components.
// app/layout.js
export const metadata = {
title: 'My Website',
description: 'Welcome to my website',
openGraph: {
title: 'My Open Graph Title',
description: 'My Open Graph Description',
images: ['/og-image.jpg'],
},
}
export default function RootLayout({ children }) {
return (
<html lang="en">
<body>{children}</body>
</html>
)
}
This API allows for dynamic metadata generation, improving your application's search engine optimization and social media sharing capabilities.
8. Improved Error Overlay
Next.js 14 features an improved error overlay that offers more detailed and helpful error messages during development.
// This will trigger the improved error overlay in development
export default function BrokenComponent() {
throw new Error('This is a demonstration of the improved error overlay')
}
The new overlay offers better stack traces, more contextual information, and improved suggestions for fixing errors, enhancing the debugging experience.
9. Built-in Font Optimization
Next.js 14 includes built-in font optimization, which automatically inlines font CSS and preloads font files to enhance performance.
import { Inter } from 'next/font/google'
const inter = Inter({ subsets: ['latin'] })
export default function RootLayout({ children }) {
return (
<html lang="en" className={inter.className}>
<body>{children}</body>
</html>
)
}
This feature removes the need for external font loading scripts and reduces layout shifts caused by font loading.
10. Enhanced Middleware
Middleware in Next.js 14 has been enhanced to support more use cases and offer better performance.
// middleware.js
import { NextResponse } from 'next/server'
export function middleware(request) {
// Example: Redirect based on user's country
const country = request.geo.country
if (country === 'US') {
return NextResponse.redirect(new URL('/us', request.url))
}
return NextResponse.next()
}
export const config = {
matcher: '/:path*',
}
This allows for more complex routing logic, authentication checks, and request/response modifications at the edge.
11. Improved TypeScript Support
Next.js 14 improves TypeScript integration, offering better type inference and more accurate type checking throughout your application.
// app/page.tsx
import { Metadata } from 'next'
type Props = {
params: { id: string }
}
export async function generateMetadata({ params }: Props): Promise<Metadata> {
return { title: `Item ${params.id}` }
}
export default function Page({ params }: Props) {
return <div>Item: {params.id}</div>
}
This improved TypeScript support
helps catch errors earlier in the development process and provides better autocompletion in IDEs.
12. Automatic 404 Pages
Next.js 14 automatically generates 404 pages for non-existent routes, enhancing user experience and SEO.
// app/not-found.js
export default function NotFound() {
return (
<div>
<h2>Not Found</h2>
<p>Could not find requested resource</p>
</div>
)
}
You can customize this page to match your application's design and provide helpful navigation options for users.
13. Dynamic OG Image Generation
Next.js 14 supports dynamic Open Graph image generation, enabling the creation of custom social media preview images on-the-fly.
// app/opengraph-image.js
import { ImageResponse } from 'next/server'
export const runtime = 'edge'
export async function GET() {
return new ImageResponse(
(
<div
style={{
fontSize: 128,
background: 'white',
width: '100%',
height: '100%',
display: 'flex',
alignItems: 'center',
justifyContent: 'center',
}}
>
Hello, World!
</div>
),
{
width: 1200,
height: 630,
}
)
}
This feature enables personalized and context-aware social media previews, which can increase engagement with shared links.
14. Incremental Static Regeneration (ISR) Improvements
Next.js 14 enhances Incremental Static Regeneration, providing finer control over content revalidation.
// app/page.js
export const revalidate = 60 // revalidate every 60 seconds
export default async function Page() {
const res = await fetch('https://api.example.com/data', { next: { revalidate: 60 } })
const data = await res.json()
return <div>{data.content}</div>
}
This improvement helps balance between static generation performance and content freshness.
15. Enhanced API Routes
Next.js 14 introduces better typing and more flexible routing options for API routes.
// app/api/hello/route.js
import { NextResponse } from 'next/server'
export async function GET(request: Request) {
const { searchParams } = new URL(request.url)
const name = searchParams.get('name')
return NextResponse.json({ message: `Hello, ${name}!` })
}
These enhancements make it easier to build robust, type-safe APIs within your Next.js application.
16. Improved CSS Support
Next.js 14 offers better CSS support, including enhanced CSS Modules and support for CSS-in-JS libraries.
// app/styles.module.css
.heading {
color: blue;
font-size: 24px;
}
// app/page.js
import styles from './styles.module.css'
export default function Page() {
return <h1 className={styles.heading}>Welcome to Next.js 14</h1>
}
This feature allows for more flexible and maintainable styling solutions in your Next.js applications.
17. Enhanced Build Output
Next.js 14 provides more detailed and informative build output, helping developers understand their application's performance and structure.
$ next build
info - Creating an optimized production build...
info - Compiled successfully
info - Collecting page data...
info - Generating static pages (3/3)
info - Finalizing page optimization...
Route (app) Size First Load JS
┌ ○ / 5.3 kB 87.4 kB
├ ○ /about 4.2 kB 86.3 kB
└ ○ /blog/[slug] 5.1 kB 87.2 kB
+ First Load JS shared by all 82.1 kB
├ chunks/framework-8883d1e9be70c3da.js 45.1 kB
├ chunks/main-57bbc28a4b75d459.js 35.2 kB
└ chunks/pages/_app-1534f180665c855f.js 1.8 kB
○ (Static) automatically rendered as static HTML (uses no initial props)
This detailed output helps developers optimize their applications and understand the impact of their changes on build size and performance.
18. Improved Error Handling
Next.js 14 introduces better error handling mechanisms, including more informative error messages and improved error boundaries.
// app/error.js
'use client'
export default function Error({ error, reset }) {
return (
<div>
<h2>Something went wrong!</h2>
<button onClick={() => reset()}>Try again</button>
</div>
)
}
This feature helps create more resilient applications by gracefully handling and recovering from errors.
19. Enhanced Static Export
Next.js 14 improves the static export functionality, allowing for more complex static sites with dynamic routes.
// next.config.js
module.exports = {
output: 'export',
// Other config options...
}
This enhancement makes Next.js a powerful option for creating static sites with the benefits of a React-based framework.
20. Improved Documentation and Learning Resources
While not a code feature, Next.js 14 comes with significantly improved documentation and learning resources, making it easier for developers to leverage all these new features effectively.
Official Website: https://nextjs.org/
For more information visit https://nextjs.org/blog/next-14
Conclusion
Next.js 14 marks a big step forward for React-based web development frameworks. It focuses on performance optimization, better developer experience, and enhanced architectural capabilities, providing a robust toolkit for building modern, scalable web applications.
By adopting Next.js 14, developers can use these advanced features to create faster, more responsive, and easier-to-maintain web applications. Whether you're working on a small personal project or a large-scale enterprise application, Next.js 14 has the tools and capabilities to bring your vision to life.
Are you ready to elevate your web development with Next.js 14? Dive in, explore these features, and take your React applications to the next level!
👏👏 Congratulations on making it this far! I hope you can implement this awesome counter animated effect in your project. Give it a try and enjoy it!
Feel free to share your thoughts and opinions, and leave a comment if you have any problems or questions.
Until then, keep on hacking. Cheers!
Subscribe to my newsletter
Read articles from Nirajan Basnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
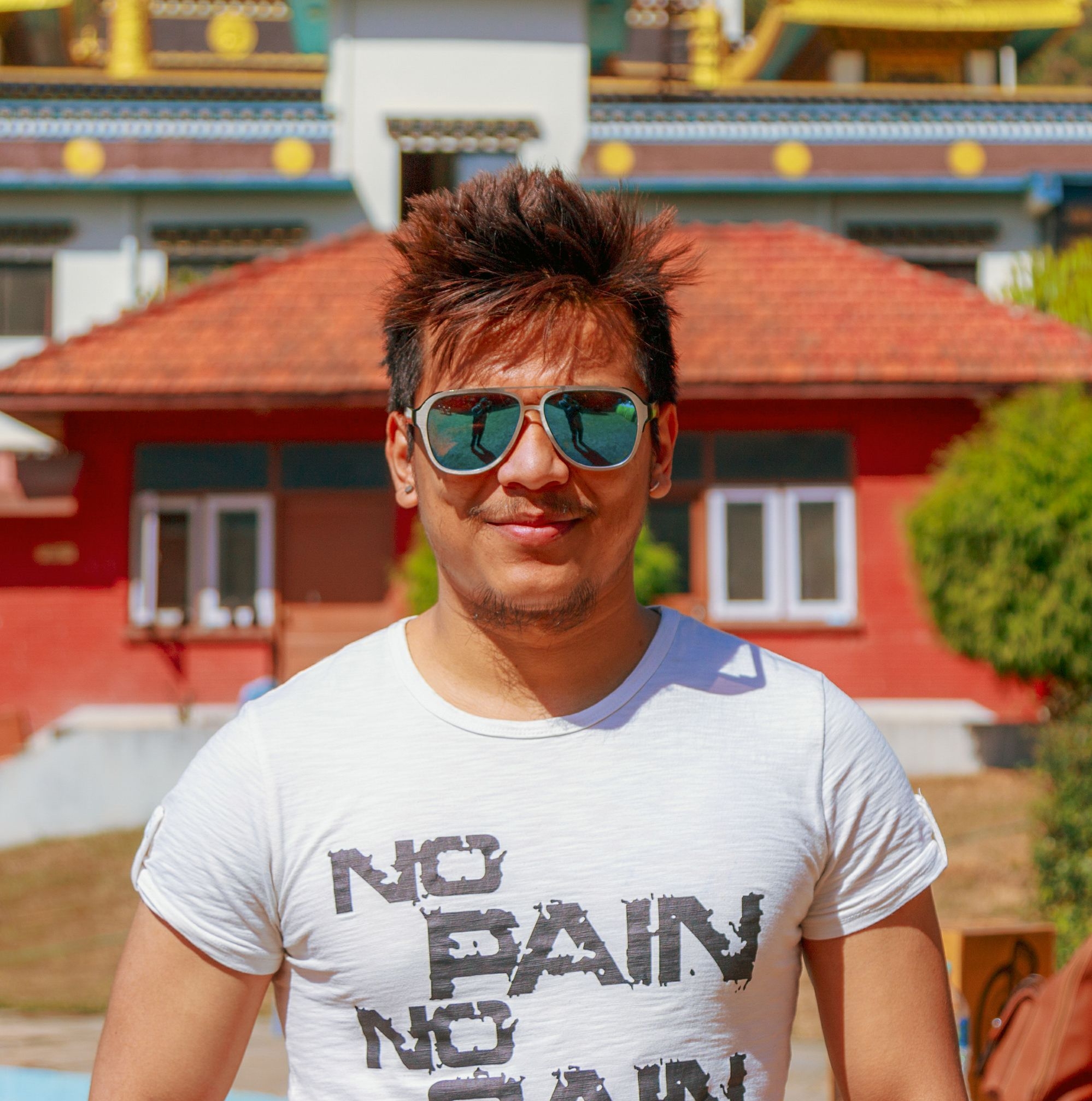
Nirajan Basnet
Nirajan Basnet
Exploring the new tools and techniques on frontend development. Loves to meet up with new people and participate in the community. I do interesting stuff on codepen https://codepen.io/nirazanbasnet