Basic Scenario-Based Terraform Interview Questions and Answers
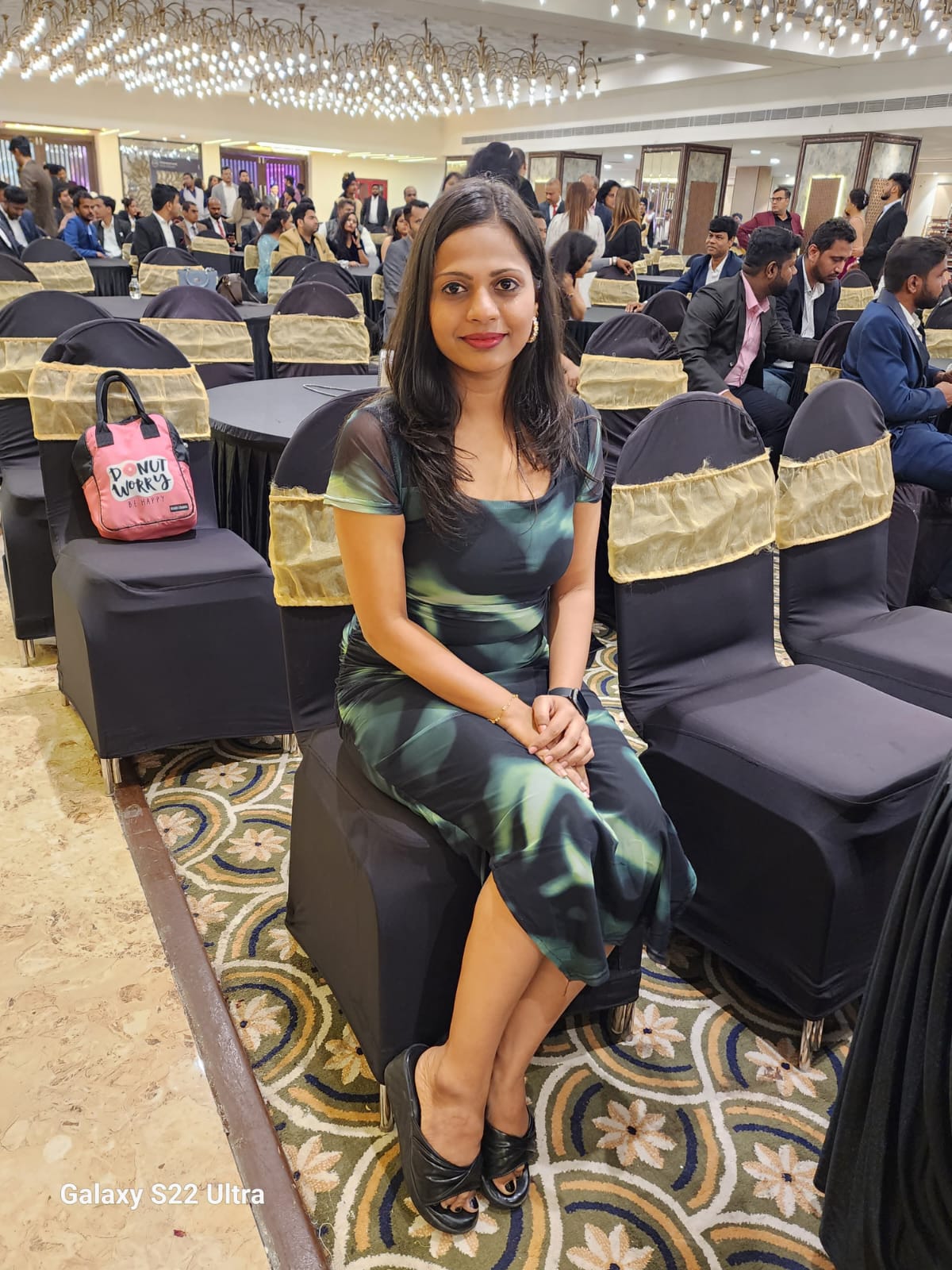
Scenario: You need to create a VPC with multiple subnets in different availability zones.
Question: How would you define this in Terraform?
Answer: You would use the aws_vpc, aws_subnet, and aws_availability_zone resources, utilizing modules for reusability and defining subnets in a loop.
Scenario: Your Terraform configuration needs to create an EC2 instance and attach an existing security group.
Question: How do you reference the existing security group?
Answer: Use the data block to fetch the existing security group and reference it in your aws_instance resource.
Scenario: You are asked to migrate an existing infrastructure managed outside Terraform into Terraform.
Question: How would you approach this task?
Answer: Use terraform import to bring the existing resources into Terraform management and ensure the state file reflects the current infrastructure state.
Scenario: Multiple team members need to work on the same Terraform configuration.
Question: How do you manage the state file to avoid conflicts?
Answer: Use remote state storage with state locking enabled, such as Terraform Cloud, AWS S3 with DynamoDB, or Azure Blob Storage with a shared key.
Scenario: A Terraform plan indicates that certain resources will be destroyed and recreated.
Question: How do you investigate the cause and prevent it?
Answer: Check for changes in immutable properties or dependencies in the configuration. Use lifecycle blocks with ignore_changes to prevent recreation if necessary.
Scenario: You need to deploy resources across multiple cloud providers.
Question: How would you structure your Terraform configuration?
Answer: Create provider configurations for each cloud provider and use modules to encapsulate the resource definitions for each provider.
Scenario: Your Terraform apply failed halfway through.
Question: What steps do you take to resolve the situation?
Answer: Investigate the error message, ensure resources are in the expected state, and use terraform apply again. Check the state file if necessary.
Scenario: You need to create infrastructure for multiple environments (dev, staging, prod).
Question: How do you manage different configurations?
Answer: Use workspaces or separate state files and configuration files for each environment, ensuring modularization and parameterization.
Scenario: A critical security patch needs to be applied to all EC2 instances.
Question: How do you ensure all instances are updated?
Answer: Update the AMI or configuration in the Terraform code, apply the changes, and use a rolling update strategy to minimize downtime.
Scenario: A team member accidentally deleted the Terraform state file.
Question: What do you do to recover?
Answer: Restore the state file from a backup or remote state storage, and use terraform refresh to update the state file with current resource statuses.
Scenario: Your Terraform configuration has hardcoded values that need to be changed frequently.
Question: How do you make it more flexible?
Answer: Use input variables to parameterize the configuration, allowing values to be changed without modifying the code.
Scenario: You are tasked with ensuring infrastructure compliance with a set of security policies.
Question: How do you enforce these policies in Terraform?
Answer: Implement policy as code using tools like Sentinel or Open Policy Agent (OPA) integrated with Terraform.
Scenario: You need to create resources that depend on each other in a specific order.
Question: How do you manage the dependencies?
Answer: Use implicit dependencies by referencing resource attributes or explicit dependencies with the depends_on meta-argument.
Scenario: Your Terraform apply command fails due to rate limiting by the cloud provider.
Question: How do you handle this?
Answer: Implement retries with exponential backoff, and use batching or throttling techniques in your configuration.
Scenario: You need to scale infrastructure based on load.
Question: How do you configure auto-scaling in Terraform?
Answer: Use resources like aws_autoscaling_group and aws_launch_configuration, and define scaling policies based on metrics.
Scenario: You need to perform a blue-green deployment.
Question: How do you implement this with Terraform?
Answer: Create separate environments (blue and green) and switch traffic between them using a load balancer or DNS.
Scenario: Your infrastructure needs to be resilient to regional failures.
Question: How do you design it with Terraform?
Answer: Deploy resources across multiple regions and use global services or cross-region replication.
Scenario: You need to update the Terraform provider to the latest version.
Question: What steps do you take to ensure a smooth update?
Answer: Check the provider's release notes, update the provider version constraint in the configuration, and run terraform init -upgrade.
Scenario: A resource configuration change is required but should not affect existing resources.
Question: How do you apply this change?
Answer: Use the lifecycle block with the ignore_changes argument to prevent specific attributes from triggering resource replacement.
Scenario: You need to create a resource only if it doesn't already exist.
Question: How do you handle this in Terraform?
Answer: Use the count or for_each meta-arguments with conditional expressions to control resource creation based on existence checks.
Scenario: You are asked to integrate Terraform with a CI/CD pipeline.
Question: How do you implement this?
Answer: Use Terraform commands in your CI/CD tool (e.g., Jenkins, GitLab CI) to run terraform init, plan, and apply as part of the deployment process.
Scenario: Your Terraform state file grows too large.
Question: What can you do to manage it?
Answer: Break the state file into multiple smaller files by using modules and workspaces, and store state remotely.
Scenario: A new team member needs access to manage Terraform infrastructure.
Question: How do you grant access securely?
Answer: Use role-based access control (RBAC) in your remote state backend and limit access with least privilege principles.
Scenario: You need to debug a Terraform configuration issue.
Question: What tools or methods do you use?
Answer: Use terraform plan to check for discrepancies, terraform validate for syntax errors, and logging/debugging output from Terraform commands.
Scenario: Your Terraform configuration must comply with organizational naming conventions.
Question: How do you enforce naming standards?
Answer: Use modules with predefined naming conventions and validate naming patterns with policies or scripts.
Scenario: You need to create a resource that is dependent on an output from another resource.
Question: How do you reference this output?
Answer: Use output variables to expose values from one module or resource and reference them in another.
Scenario: You need to create resources conditionally based on input variables.
Question: How do you achieve this in Terraform?
Answer: Use conditional expressions with the count or for_each meta-arguments to create resources based on input variable conditions.
Scenario: You need to version control your Terraform configurations.
Question: What best practices do you follow?
Answer: Use a version control system like Git, modularize your code, maintain clear commit messages, and implement CI/CD for automatic testing and deployment.
Scenario: Your organization adopts a multi-cloud strategy.
Question: How do you manage infrastructure across different cloud providers?
Answer: Use Terraform’s multi-provider capabilities, create provider configurations for each cloud, and define modules to encapsulate cloud-specific resource definitions.
Scenario: You need to roll back infrastructure to a previous state.
Question: How do you perform a rollback in Terraform?
Answer: Use versioned state files or revert to a previous commit in your version control system, and run terraform apply to restore the desired state.
Subscribe to my newsletter
Read articles from Shraddha Suryawanshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
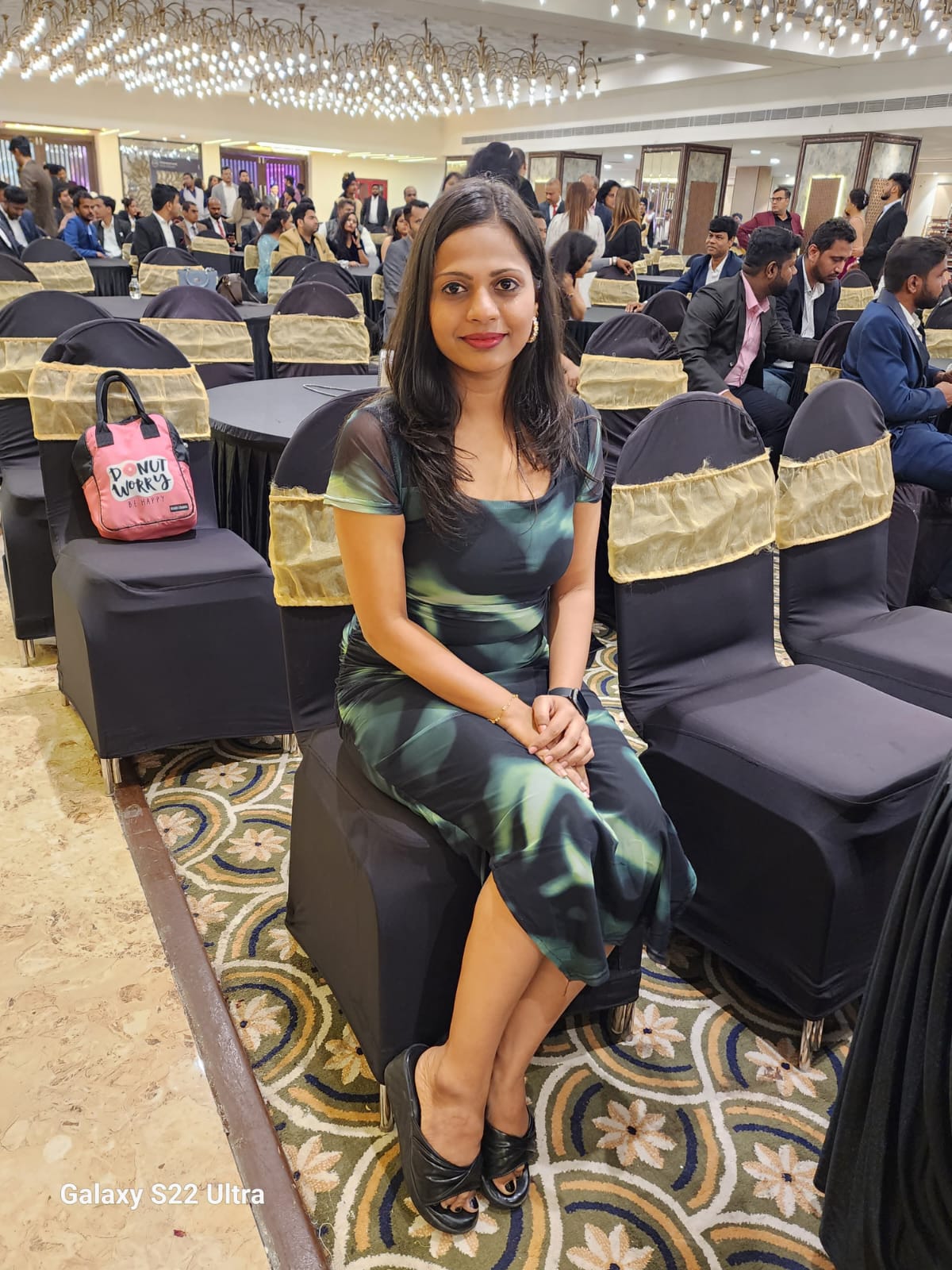