Creating Animations in .NET MAUI - A Comprehensive Guide
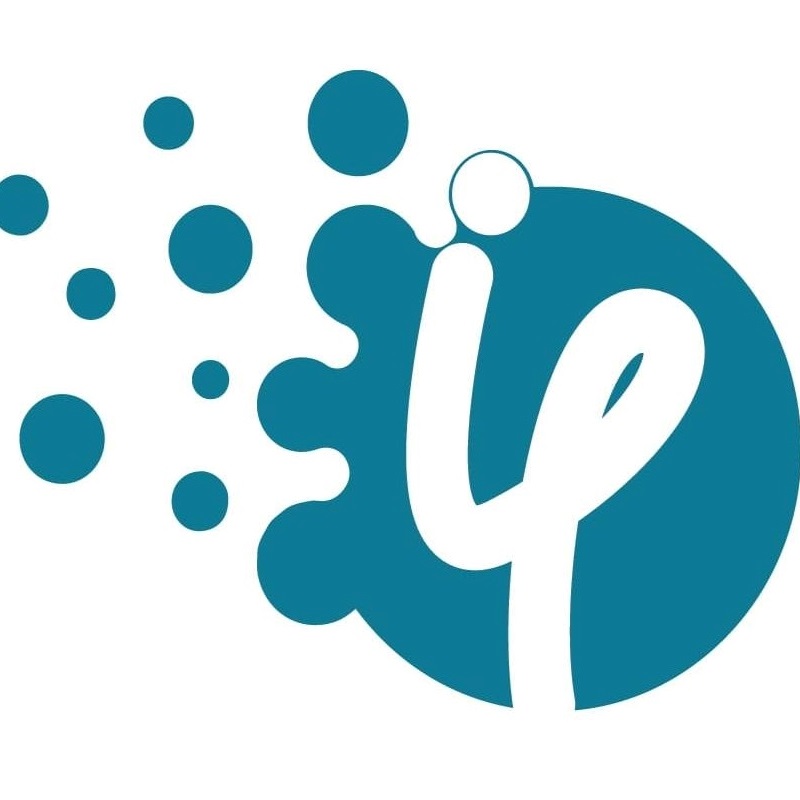
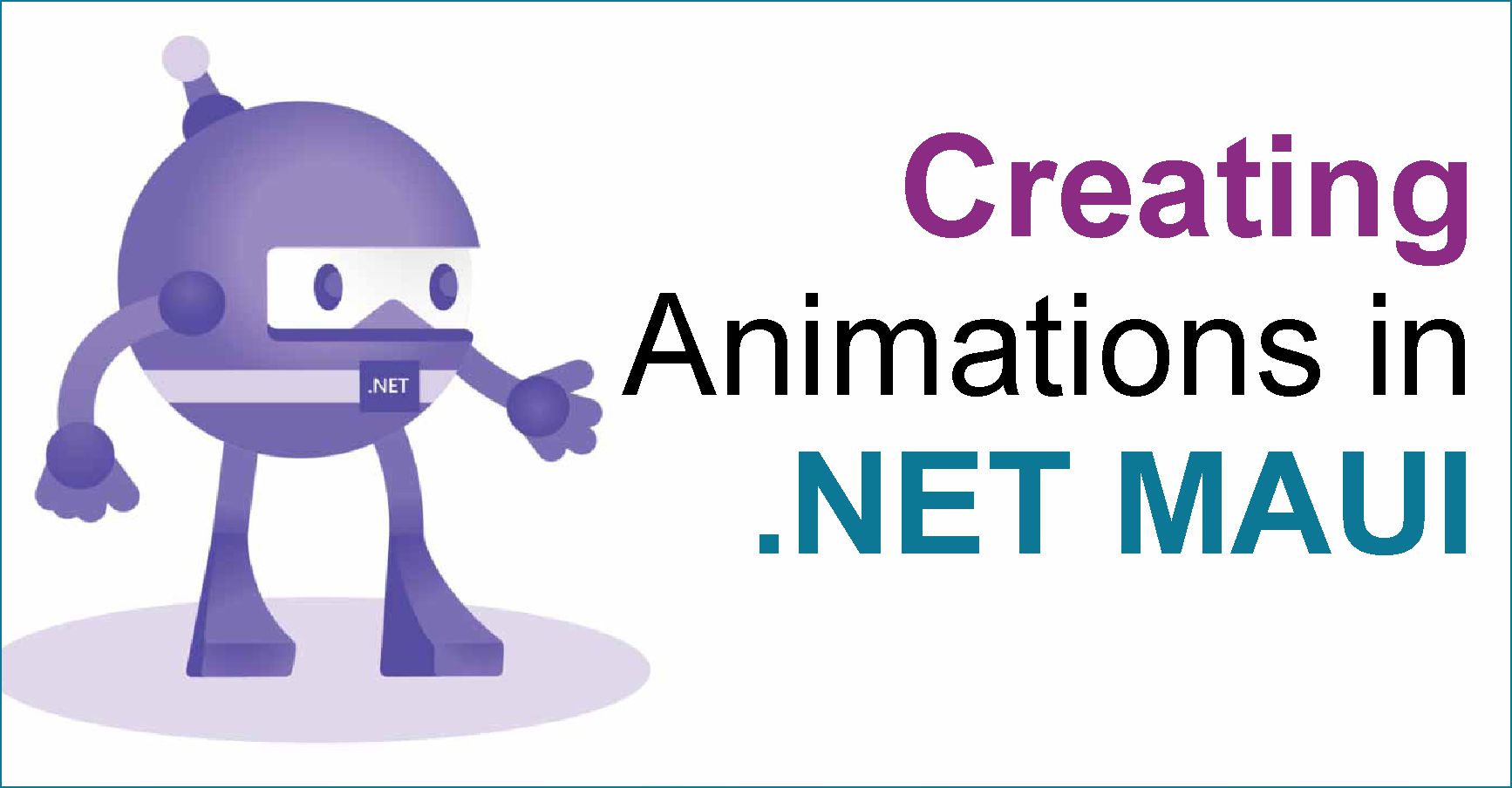
In the world of mobile and desktop applications, animations play a crucial role in enhancing user experience by making interactions more intuitive and engaging. .NET Multi-platform App UI (.NET MAUI) is a powerful framework for building cross-platform applications, and it provides robust support for creating rich animations. In this comprehensive guide, we will explore how to create animations in .NET MAUI, from basic to advanced techniques. If you're looking for professional assistance, many companies offer .NET MAUI app development services to help bring your ideas to life.
Understanding Animations in .NET MAUI
Animations are visual effects that add motion to UI elements, helping to create a more dynamic and responsive user interface. In .NET MAUI, animations can range from simple transitions to complex sequences. The framework supports several types of animations, including basic animations (like fading and scaling), transformations, and keyframe animations.
Getting Started with Animations
Before diving into creating animations, ensure you have a .NET MAUI project set up. If you haven’t already, you can create a new .NET MAUI project using Visual Studio. Make sure you have the necessary SDKs and tools installed.
Basic Animations
Fading Animation
A fading animation can make an element appear or disappear smoothly. Here’s how to create a simple fade-in and fade-out animation:
csharpCopy code// Fading in
await myElement.FadeTo(1, 1000); // Fade in over 1 second
// Fading out
await myElement.FadeTo(0, 1000); // Fade out over 1 second
Scaling Animation
Scaling animations change the size of an element. Here’s an example of scaling an element up and down:
csharpCopy code// Scaling up
await myElement.ScaleTo(1.5, 1000); // Scale to 1.5 times its original size over 1 second
// Scaling down
await myElement.ScaleTo(1, 1000); // Scale back to original size over 1 second
Transformations and Effects
Transformations involve rotating, translating, or scaling elements to create more complex animations.
Rotating Animation
Rotating an element can add a dynamic effect to your UI. Here’s how to create a rotation animation:
csharpCopy codeawait myElement.RotateTo(360, 2000); // Rotate 360 degrees over 2 seconds
Combining Transformations
You can combine multiple transformations to create a more complex animation:
csharpCopy codeawait myElement.ScaleTo(1.5, 1000);
await myElement.RotateTo(360, 1000);
await myElement.TranslateTo(50, 50, 1000);
Keyframe Animations
Keyframe animations allow for more complex and detailed animations by specifying multiple points (keyframes) in the animation sequence.
csharpCopy codevar animation = new Animation();
animation.WithConcurrent((f) => myElement.Opacity = f, 0, 1, Easing.Linear);
animation.WithConcurrent((f) => myElement.Scale = f, 1, 1.5, Easing.SpringIn);
animation.WithConcurrent((f) => myElement.Rotation = f, 0, 360);
await myElement.AnimateAsync("keyframeAnimation", animation, 16, 2000);
Custom Animations
For more advanced scenarios, you might need to create custom animations. .NET MAUI allows you to extend its animation capabilities with your own logic.
csharpCopy codepublic async Task CustomAnimation(View element)
{
var animation = new Animation(v => element.Opacity = v, 0, 1);
animation.Commit(element, "CustomAnimation", 16, 1000, Easing.Linear, (v, c) => element.Opacity = 1);
await Task.Delay(1000);
}
Performance Considerations
Optimizing animations is crucial for ensuring smooth performance, especially on lower-end devices. Here are some tips:
Limit the number of concurrent animations: Too many animations running simultaneously can slow down your app.
Use hardware-accelerated animations: Ensure your animations are offloaded to the GPU when possible.
Profile your app: Use profiling tools to identify and fix performance bottlenecks.
Read More: WPF vs MAUI: Key Differences that Businesses Should Know
Real-World Examples
Example 1: Bouncing Button
Create a button that scales up and down to create a bouncing effect when clicked.
csharpCopy codeprivate async void OnButtonClick(object sender, EventArgs e)
{
await myButton.ScaleTo(1.2, 100);
await myButton.ScaleTo(1, 100);
}
Example 2: Sliding Menu
Create a sliding menu that slides in from the left when a button is clicked.
csharpCopy codeprivate async void OnMenuButtonClick(object sender, EventArgs e)
{
await myMenu.TranslateTo(0, 0, 500, Easing.SpringOut);
}
Debugging and Troubleshooting
Common issues with animations include performance lag, incorrect timing, or visual glitches. Here are some troubleshooting tips:
Check animation timings: Ensure the duration and delays are set correctly.
Use easing functions: Easing functions can smooth out animations and make them look more natural.
Test on multiple devices: Animations may perform differently on various devices, so test on a range of hardware.
Conclusion
Animations in .NET MAUI can significantly enhance the user experience of your applications. By understanding the basics and exploring more advanced techniques, you can create dynamic, responsive, and visually appealing interfaces. Experiment with different types of animations and combine them to create unique effects.
Subscribe to my newsletter
Read articles from Harshal Suthar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
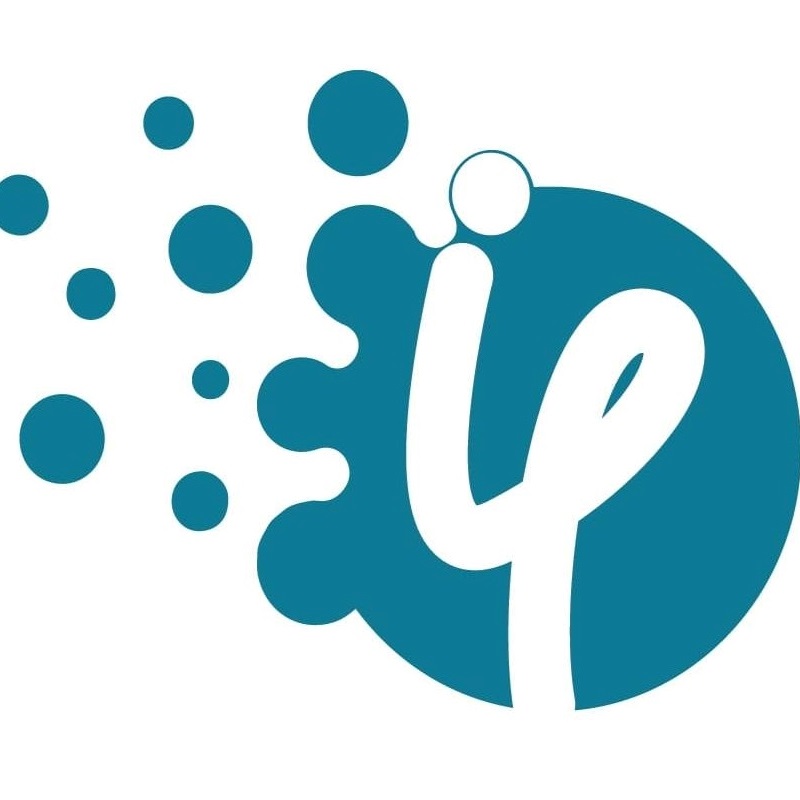