Advanced Scenario-Based Terraform Interview Questions and Answers
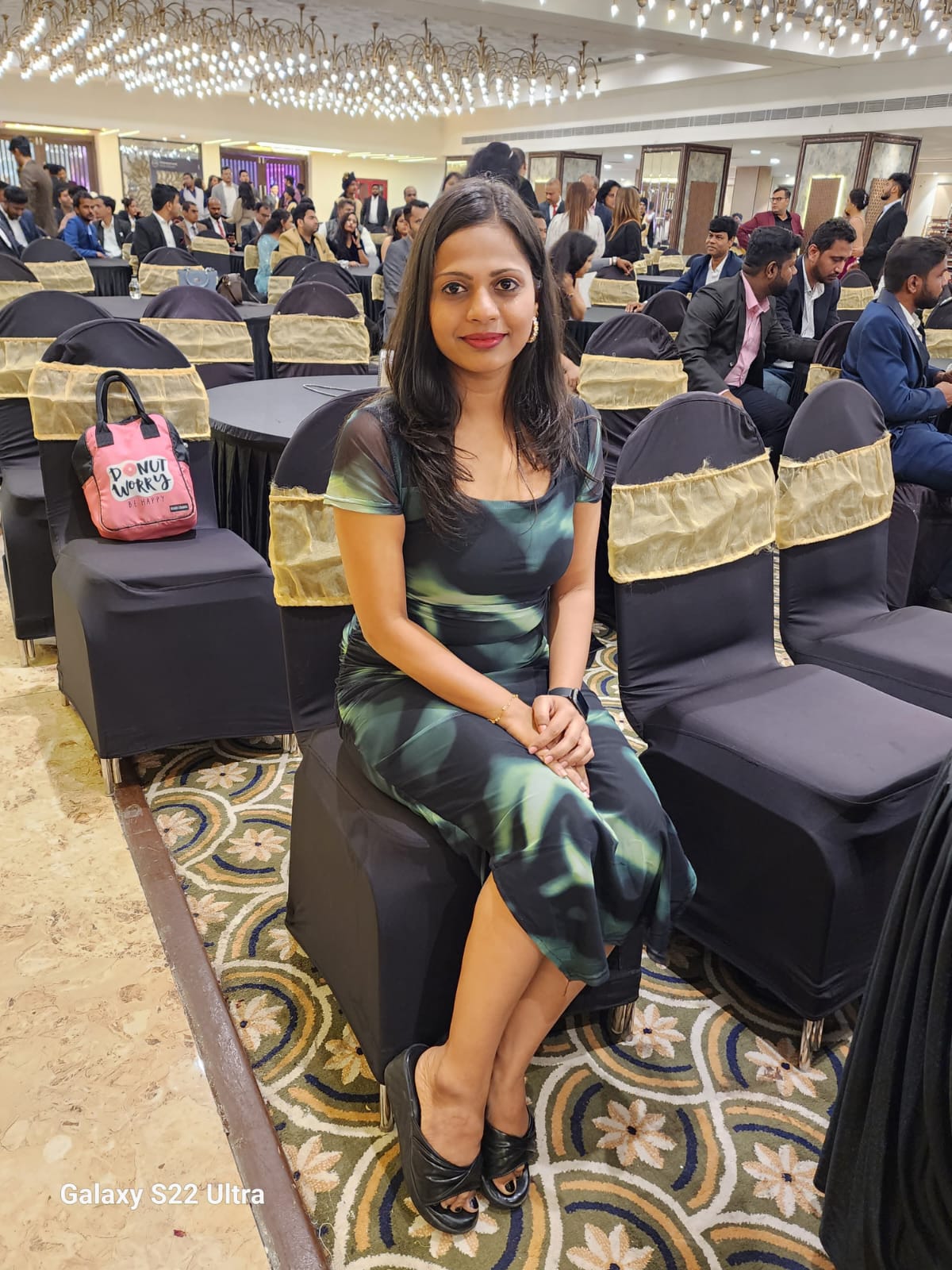
These advanced scenario-based questions cover a wide range of complex situations you might encounter while managing infrastructure with Terraform, ensuring you can demonstrate in-depth knowledge and practical problem-solving skills.
Scenario 1 : You need to manage infrastructure in a multi-cloud environment, ensuring high availability and failover capabilities.
Question: How do you design and implement such a solution using Terraform?
Answer:
Multi-cloud Provider Setup: Define multiple providers for different clouds (e.g., AWS, Azure, GCP).
Infrastructure Deployment: Use modules to encapsulate infrastructure definitions for each cloud. Deploy similar resources (like databases, VMs) across providers.
High Availability and Failover: Implement load balancers and DNS failover mechanisms (e.g., AWS Route 53, Azure Traffic Manager) to route traffic between clouds.
Example:
provider "aws" {
region = "us-west-2"
}
provider "google" {
project = "my-project"
region = "us-central1"
}
module "aws_infra" {
source = "./modules/aws"
providers = {
aws = aws
}
}
module "gcp_infra" {
source = "./modules/gcp"
providers = {
google = google
}
}
State Management: Use a remote backend like Terraform Cloud or Consul for state synchronization and locking across the environments.
Scenario 2 : You need to implement continuous delivery of infrastructure changes while ensuring minimal downtime.
Question: How do you integrate Terraform with a CI/CD pipeline to achieve this?
Answer:
Pipeline Setup: Integrate Terraform commands into your CI/CD tool (e.g., Jenkins, GitLab CI).
Infrastructure Testing: Use terraform plan in a staging environment before applying changes to production.
Blue-Green Deployments: Implement blue-green deployments to minimize downtime. Deploy changes to a "blue" environment, test, and switch traffic using a load balancer.
Example:
stages:
- plan
- apply
plan:
script:
- terraform init
- terraform plan -out=tfplan
apply:
script:
- terraform apply "tfplan"
Monitoring and Rollback: Implement monitoring and alerting to detect issues post-deployment. Use version control to rollback changes if necessary.
Scenario 3 : You need to implement a disaster recovery plan for your infrastructure managed by Terraform.
Question: How do you configure Terraform to handle disaster recovery scenarios?
Answer:
Multi-Region Deployment: Deploy critical resources in multiple regions.
Data Replication: Use cloud-native solutions for data replication (e.g., AWS RDS Multi-AZ, GCP Cloud SQL Replicas).
Automated Failover: Configure automated failover mechanisms using health checks and routing policies.
Example:
resource "aws_rds_cluster" "example" {
engine = "aurora"
master_username = "foo"
master_password = "bar"
availability_zones = ["us-west-2a", "us-west-2b"]
}
resource "aws_route53_record" "failover" {
zone_id = "Z1234567890"
name = "example.com"
type = "A"
set_identifier = "primary"
failover_routing_policy {
type = "PRIMARY"
}
alias {
name = aws_elb.example.dns_name
zone_id = aws_elb.example.zone_id
evaluate_target_health = true
}
}
Regular Drills: Schedule regular disaster recovery drills to ensure the plan works as expected.
Scenario 4 : You need to ensure compliance with security policies and standards in your Terraform-managed infrastructure.
Question: How do you enforce and monitor compliance using Terraform?
Answer:
Policy as Code: Use Sentinel or Open Policy Agent (OPA) to enforce security policies.
Configuration Validation: Implement pre-commit hooks and CI/CD checks to validate configurations against policies.
Example:
policy "no_public_s3_buckets" {
rule {
all tfplan.resource_changes as , resourcechanges {
all resource_changes as resource_change {
resource_change.type == "aws_s3_bucket" and resource_change.change.after.acl != "public-read"
}
}
}
}
Automated Audits: Use tools like terraform-compliance or Checkov to automate compliance audits.
Continuous Monitoring: Implement continuous monitoring and alerting for policy violations using cloud-native tools (e.g., AWS Config, Azure Policy).
Scenario 5 : You need to migrate a large, complex infrastructure setup to Terraform without downtime.
Question: What steps do you take to perform this migration smoothly?
Answer:
Incremental Import: Use terraform import to bring existing resources into Terraform management incrementally.
State File Management: Ensure the state file accurately reflects the existing infrastructure.
Resource Mapping: Map existing resources to Terraform configurations without altering current infrastructure.
Example:
terraform import aws_instance.example i-1234567890abcdef0
Testing and Validation: Test the imported resources in a staging environment to ensure no discrepancies.
Phased Rollout: Roll out Terraform management in phases to minimize impact on production environments.
Scenario 6 : You need to optimize cost and resource utilization in your Terraform-managed infrastructure.
Question: How do you identify and implement cost-saving measures using Terraform?
Answer:
Resource Tagging: Implement tagging policies to categorize and track resource usage.
Auto-scaling: Configure auto-scaling for dynamic resource allocation based on demand.
Spot Instances: Use spot instances for non-critical workloads to reduce costs.
Example:
resource "aws_autoscaling_group" "example" {
launch_configuration = aws_launch_configuration.example.id
min_size = 1
max_size = 10
tag {
key = "Environment"
value = "Production"
propagate_at_launch = true
}
}
resource "aws_spot_instance_request" "example" {
spot_price = "0.03"
ami = "ami-0abcdef1234567890"
instance_type = "t2.micro"
}
Cost Management Tools: Integrate with cloud-native cost management tools (e.g., AWS Cost Explorer, Azure Cost Management) to monitor and analyze costs.
Scenario 7 : Your Terraform configuration needs to manage secrets securely across multiple environments.
Question: How do you manage and secure secrets in Terraform?
Answer:
Environment Variables: Pass secrets as environment variables during Terraform runs.
Secret Management Services: Use cloud-native secret management services (e.g., AWS Secrets Manager, Azure Key Vault) to store and retrieve secrets.
Example:
data "aws_secretsmanager_secret" "example" {
name = "my-secret"
}
data "aws_secretsmanager_secret_version" "example" {
secret_id = data.aws_secretsmanager_secret.example.id
}
resource "aws_db_instance" "example" {
identifier = "example"
username = "foo"
password = data.aws_secretsmanager_secret_version.example.secret_string
}
Encrypted Storage: Ensure state files and sensitive data are encrypted using Terraform’s built-in encryption mechanisms.
Scenario 8 : You need to ensure that your Terraform configurations are reusable and maintainable.
Question: What best practices do you follow to achieve this?
Answer:
Modularization: Break down configurations into reusable modules.
Version Control: Use version control for module management and versioning.
Documentation: Document modules and configurations comprehensively.
Example:
module "vpc" {
source = "./modules/vpc"
cidr = "10.0.0.0/16"
}
DRY Principle: Follow the DRY (Don’t Repeat Yourself) principle to avoid redundancy in configurations.
Scenario 9 : You need to handle a large number of dynamic resources in your Terraform configuration.
Question: How do you efficiently manage dynamic resource creation?
Answer:
Loops: Use loops with for_each or count to dynamically create resources.
Maps and Lists: Utilize maps and lists to manage dynamic inputs and iterate over them.
Example:
variable "subnets" {
type = map(string)
default = {
subnet1 = "10.0.1.0/24"
subnet2 = "10.0.2.0/24"
}
}
resource "aws_subnet" "example" {
for_each = var.subnets
cidr_block = each.value
vpc_id = aws_vpc.example.id
}
Templates: Use templates to dynamically generate configurations based on inputs.
Scenario 10 : Your organization requires a multi-tenant architecture with isolated environments for each tenant.
Question: How do you design and implement this using Terraform?
Answer:
Provider Aliases: Use provider aliases to manage multiple environments.
Resource Isolation: Create separate resources (VPCs, subnets) for each tenant to ensure isolation.
provider "aws" {
alias = "tenant1"
region = "us-west-2"
}
provider "aws" {
alias = "tenant2"
region = "us-east-1"
}
resource "aws_vpc" "tenant1" {
provider = aws.tenant1
cidr_block = "10.0.0.0/16"
}
resource "aws_vpc" "tenant2" {
provider = aws.tenant2
cidr_block = "10.1.0.0/16"
}
State Separation: Use separate state files or workspaces for each tenant to manage state independently.
Example Domain
Subscribe to my newsletter
Read articles from Shraddha Suryawanshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
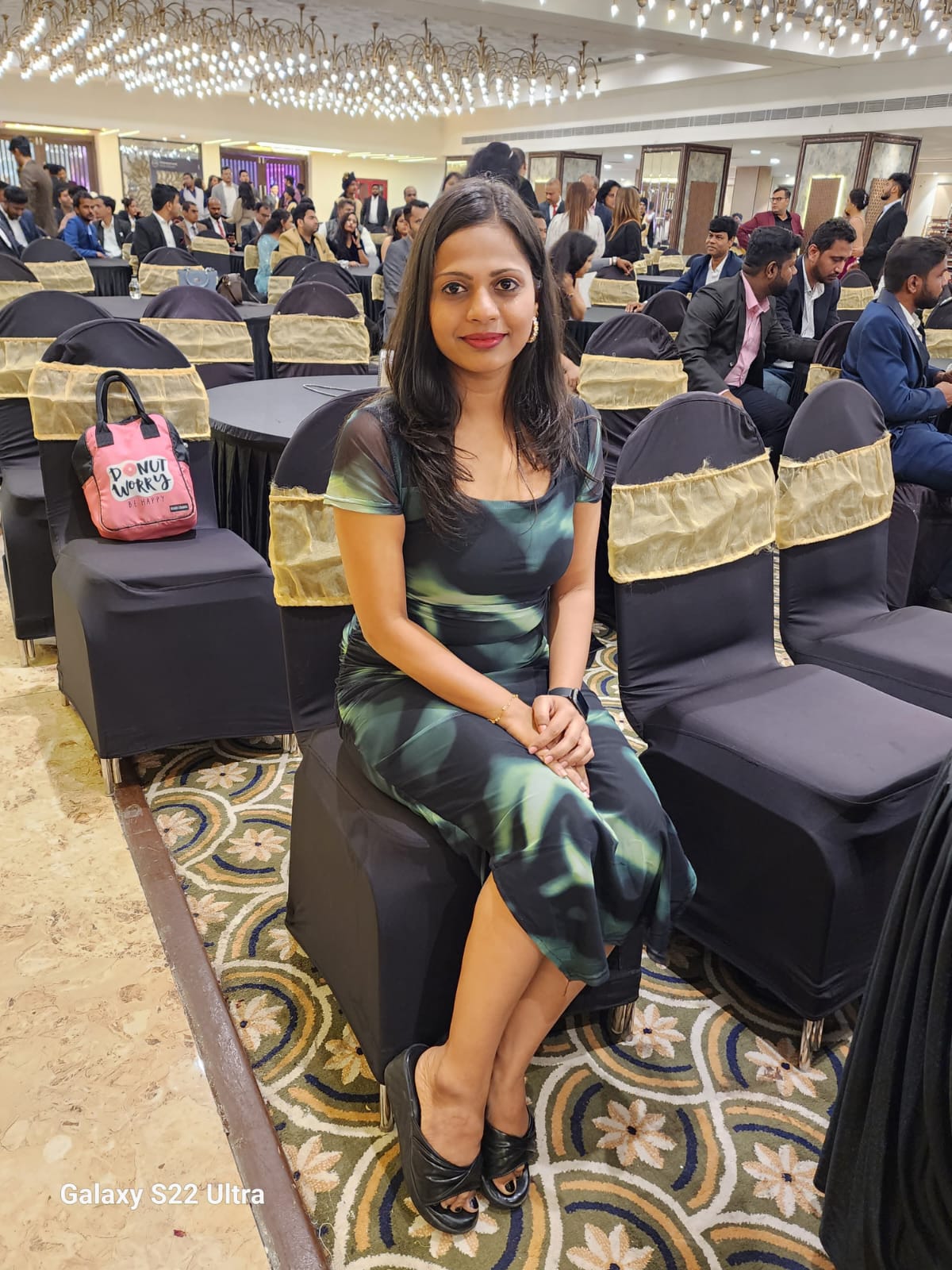