Exploring Laravel Eloquent ORM in Depth
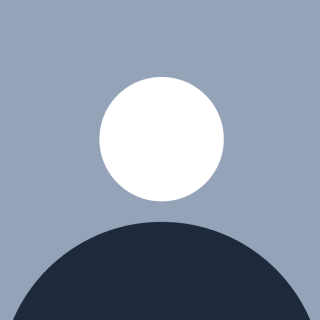
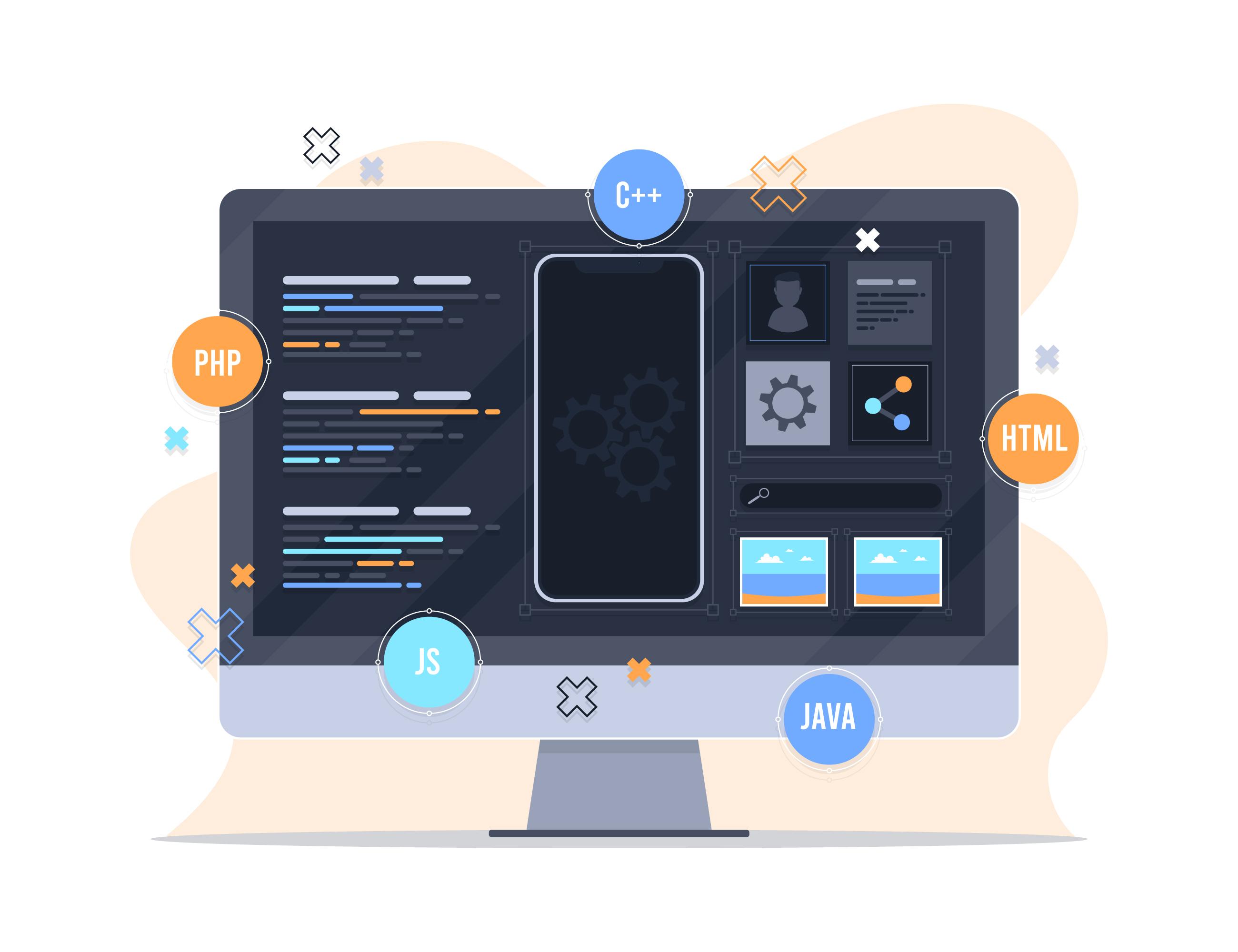
When I first started working with Laravel, I was immediately struck by the simplicity and elegance of its Eloquent ORM (Object-Relational Mapping). In this article, I’ll take you through what Eloquent is, how it evolved over time, and why it’s such a valuable tool for developers and development companies.
What is Eloquent ORM?
Eloquent ORM is Laravel’s built-in solution for interacting with databases using an object-oriented approach. Instead of writing raw SQL queries, Eloquent allows you to interact with your database using PHP syntax, making your code cleaner and more readable. Essentially, it abstracts the complexity of SQL and lets you work with database records as if they were simple PHP objects.
When Was Eloquent First Released?
Eloquent was first introduced in Laravel 4, which was released in May 2013. It was designed to offer a more developer-friendly way to work with databases compared to traditional methods. Since then, it has become one of the core features of Laravel and has undergone numerous updates to enhance its functionality and ease of use.
Uses of Eloquent ORM
For me, the real power of Eloquent lies in its ability to simplify database interactions. Here’s how it’s useful:
Readable Code: Eloquent provides a clear, readable syntax that’s easy to understand. Instead of crafting complex SQL queries, you can use methods like find(), where(), and save() to manage your data.
Relationships: Eloquent supports various types of relationships, such as one-to-many, many-to-many, and polymorphic relationships. This feature is invaluable for modeling real-world data relationships in your applications.
Mass Assignment: With Eloquent, you can quickly assign multiple attributes to your models using arrays. This is much more efficient than setting each attribute individually.
Soft Deletes: Eloquent includes built-in support for soft deletes, which allows you to keep records in the database even after they've been marked as deleted.
Updates Over the Years
Eloquent has seen several updates since its inception. Each version of Laravel has brought improvements and new features to Eloquent. Here’s a brief overview of some key updates:
Laravel 5.0: Introduced the concept of model factories for testing, along with improvements in relationship handling.
Laravel 5.1: Added support for eager loading constraints, making it easier to optimize complex queries.
Laravel 5.4: Brought new features like model events for handling model lifecycle events and custom casts for transforming model attributes.
Laravel 6.x and Beyond: Added features such as subquery support and lazy collections, which enhance performance and flexibility.
For more details, you can check out the DigitalOcean's Tutorial.
How Eloquent Benefits Developers and Companies
Eloquent’s benefits are numerous, particularly for developers and development companies:
Increased Productivity: Eloquent’s intuitive syntax reduces the amount of code you need to write, speeding up development time.
Consistency: By using Eloquent, you maintain a consistent coding style and structure throughout your project, which is crucial for team-based development.
Reduced Errors: The abstraction provided by Eloquent helps reduce the likelihood of SQL injection attacks and other common database errors.
Easier Maintenance: With Eloquent, your database queries are embedded in your PHP code, making it easier to manage and update as your application evolves.
Enhancing Your Projects with Laravel Development Services
Utilizing Laravel web development services correctly ensures efficient use of Eloquent ORM, streamlining database operations and enhancing project performance. Discover how expert services can optimize your Laravel applications.
What Laravel Eloquent ORM Offers
To illustrate Eloquent’s capabilities, here are some key features along with code examples:
Basic Operations
Operation | Code Example |
Retrieve All Records | User::all(); |
Find a Record by ID | User::find(1); |
Create a New Record | User::create(['name' => 'John', 'email' => 'john@example.com']); |
Update a Record | $user = User::find(1); $user->name = 'Jane'; $user->save(); |
Delete a Record | $user = User::find(1); $user->delete(); |
Relationships
Relationship Type | Code Example |
One-to-Many | public function posts() { return $this->hasMany(Post::class); } |
Many-to-Many | public function roles() { return $this->belongsToMany(Role::class); } |
Polymorphic | public function imageable() { return $this->morphTo(); } |
Comparing Eloquent with Other ORMs
Eloquent isn’t the only ORM available. Let’s compare it with some alternatives:
Eloquent vs. Doctrine
Feature | Eloquent | Doctrine |
Ease of Use | Very user-friendly, especially for beginners. | More complex, with a steeper learning curve. |
Performance | Generally good for most applications. | Can be more performant in complex scenarios due to advanced caching. |
Flexibility | Highly flexible, but relies on Laravel conventions. | Offers more advanced features and customizability. |
Configuration | Simple and straightforward. | Requires more configuration and setup. |
Eloquent vs. Propel
Feature | Eloquent | Propel |
Integration | Seamlessly integrates with Laravel. | Requires additional setup for integration. |
Active Record vs. Data Mapper | Uses Active Record pattern. | Uses Data Mapper pattern, which can offer more flexibility. |
Ease of Learning | Easier for Laravel developers. | Can be more challenging to learn due to its different approach. |
Conclusion
In my experience, Eloquent ORM has been a game-changer. It simplifies database interactions, making my code more readable and maintainable. The ability to define relationships easily and perform complex queries without writing raw SQL is incredibly valuable. While there are other ORMs available, Eloquent’s integration with Laravel and its ease of use make it a strong choice for many projects.
Whether you’re a seasoned developer or just starting, Eloquent offers a powerful and intuitive way to manage your database interactions. It’s continually evolving, and its updates reflect the needs and feedback of the developer community. So, if you’re working with Laravel, Eloquent is definitely worth exploring further.
Frequently Asked Questions (FAQs)
1. What is Laravel Eloquent ORM?
Eloquent ORM is Laravel's built-in database abstraction layer. It lets you interact with your database using PHP syntax instead of raw SQL, simplifying database management.
2. How do you define a relationship in Eloquent?
In Eloquent, relationships like one-to-many or many-to-many are defined in model classes using methods such as hasMany() and belongsToMany(). This simplifies querying related records.
3. What is the difference between create() and update() methods in Eloquent?
create() inserts a new record into the database, while update() modifies an existing record. Both methods are used to manage data, but update() requires an existing model instance.
4. How does Eloquent handle database migrations?
Eloquent does not handle migrations directly; Laravel's migration system manages schema changes. Eloquent models work with these migrations to interact with the database efficiently.
5. Can Eloquent ORM handle complex queries efficiently?
Yes, Eloquent ORM can handle complex queries using methods like where(), join(), and eager loading. For very complex queries, you might use raw SQL for better performance.
Subscribe to my newsletter
Read articles from Arthur Rivera directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by