Mastering CSS Grid for Beginners: A Step-by-Step Guide

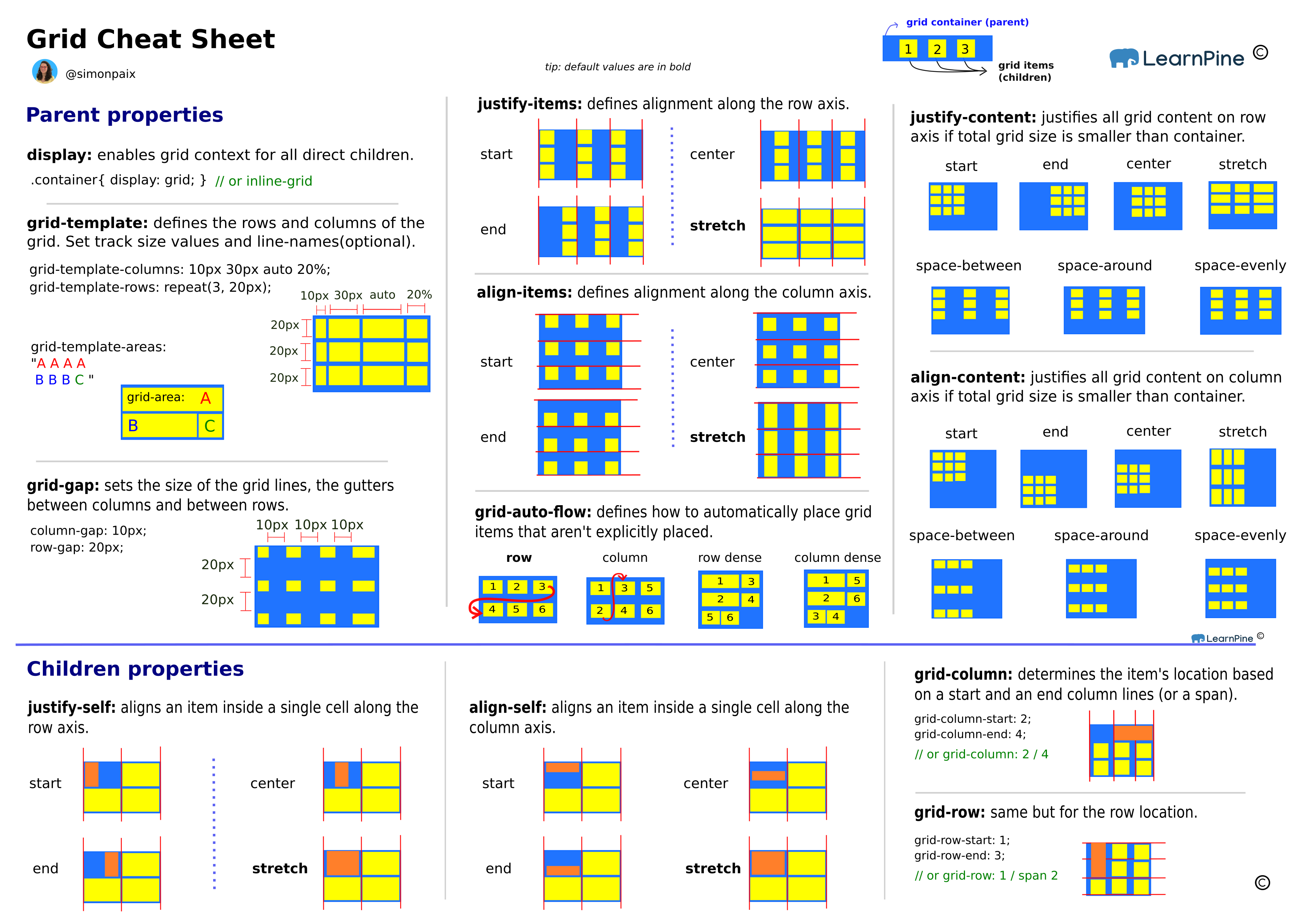
Introduction
In today's digital world, web design is more crucial than ever, and mastering layout techniques is a key part of that. One of the most powerful layout tools in modern CSS is the CSS Grid. Whether you're building complex web layouts or just starting with your first website, understanding CSS Grid will give you the flexibility and control needed to create stunning designs. This guide will take you through the basics of CSS Grid, complete with examples, exercises, and resources to make learning fun and engaging.
Table of Contents
1. What is CSS Grid?
CSS Grid is a two-dimensional layout system for the web. It allows you to create complex layouts with rows and columns, offering a much more intuitive way to design responsive web pages compared to older layout techniques like floats and tables.
Key Features of CSS Grid:
Two-Dimensional Layout: Control both rows and columns.
Responsive Design: Easily adapt to different screen sizes.
Flexible Alignment: Align items vertically and horizontally within the grid.
2. Basic Concepts of CSS Grid
Before diving into examples, let's understand some fundamental concepts of CSS Grid:
Grid Container: The element on which
display: grid
is applied. It's the parent of all grid items.Grid Item: The direct children of the grid container.
Grid Line: The lines dividing the grid into rows and columns.
Grid Track: The space between two grid lines (a row or a column).
Grid Cell: The smallest unit on the grid, where a row and a column intersect.
Grid Area: A rectangular space composed of one or more grid cells.
Here's a simple diagram to visualize these concepts:
+---------------------------+
| |
| Grid Container |
| +--------+--------+ |
| | | | |
| | Item | Item | |
| | | | |
| +--------+--------+ |
| |
+---------------------------+
3. Creating a Simple Grid Layout
Let's create a basic grid layout to understand how the CSS Grid works. Below is an example of a simple grid with two columns and two rows:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Simple CSS Grid Layout</title>
<style>
.grid-container {
display: grid;
grid-template-columns: 1fr 1fr;
grid-template-rows: 100px 100px;
gap: 10px;
background-color: #f9f9f9;
padding: 10px;
}
.grid-item {
background-color: #8ca0ff;
border: 2px solid #6b86e5;
border-radius: 5px;
padding: 20px;
font-size: 18px;
text-align: center;
}
</style>
</head>
<body>
<div class="grid-container">
<div class="grid-item">1</div>
<div class="grid-item">2</div>
<div class="grid-item">3</div>
<div class="grid-item">4</div>
</div>
</body>
</html>
Explanation:
Grid Container: The
.grid-container
class is the grid container. We define it withdisplay: grid
.Grid Template Columns: The
grid-template-columns: 1fr 1fr;
defines two columns, each taking up equal space.Grid Template Rows: The
grid-template-rows: 100px 100px;
specifies two rows, each with a fixed height of 100px.Gap: The
gap: 10px;
adds spacing between grid items.
Live Demo:
You can play with the example above using the following CodePen link: CSS Grid Simple Example.
4. Understanding Grid Properties
Now that we've seen a basic example, let's delve into some of the essential CSS Grid properties and how they can be used to create more complex layouts.
a. Grid Container Properties
display
The
display
property is used to define the element as a grid container..grid-container { display: grid; }
grid-template-columns and grid-template-rows
These properties define the number of columns and rows in the grid. You can specify fixed values, percentages, or the
fr
unit, which represents a fraction of the available space..grid-container { grid-template-columns: 100px 200px 1fr; grid-template-rows: 150px auto; }
Fixed Size:
100px
,200px
Fractional Unit:
1fr
Auto: Automatically sizes based on content.
grid-column-gap and grid-row-gap
These properties set the gap between columns and rows. The shorthand property
gap
can be used to set both simultaneously..grid-container { grid-column-gap: 20px; grid-row-gap: 10px; /* Shorthand */ gap: 20px 10px; }
grid-template-areas
This property allows you to name grid areas and layout items using a visual grid template.
.grid-container { grid-template-areas: "header header" "sidebar content" "footer footer"; }
justify-items and align-items
These properties align grid items within their grid cells.
.grid-container { justify-items: center; /* Aligns items horizontally */ align-items: center; /* Aligns items vertically */ }
b. Grid Item Properties
grid-column and grid-row
These properties define the starting and ending positions of a grid item within the grid.
.grid-item { grid-column: 1 / 3; /* Span from column 1 to column 3 */ grid-row: 1 / 2; /* Span from row 1 to row 2 */ }
grid-area
Assigns a name to a grid item, allowing it to be placed using
grid-template-areas
..grid-item { grid-area: header; }
justify-self and align-self
These properties align a specific grid item within its grid cell.
.grid-item { justify-self: start; /* Aligns the item horizontally at the start */ align-self: end; /* Aligns the item vertically at the end */ }
5. Practical Examples
Let's explore a few practical examples of CSS Grid to understand its real-world applications.
Example 1: Responsive Card Layout
A responsive card layout is a common design pattern that can be easily achieved with CSS Grid.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Responsive Card Layout</title>
<style>
.card-container {
display: grid;
grid-template-columns: repeat(auto-fill, minmax(200px, 1fr));
gap: 20px;
padding: 20px;
}
.card {
background-color: #fff;
border: 1px solid #ddd;
border-radius: 8px;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.1);
overflow: hidden;
transition: transform 0.2s ease;
}
.card:hover {
transform: translateY(-5px);
}
.card img {
width: 100%;
height: 150px;
object-fit: cover;
}
.card-content {
padding: 15px;
}
.card-title {
font-size: 20px;
font-weight: bold;
margin-bottom: 10px;
}
.card-text {
color: #555;
line-height: 1.5;
}
</style>
</head>
<body>
<div class="card-container">
<div class="card">
<img src="
https://via.placeholder.com/300x150" alt="Card Image">
<div class="card-content">
<h3 class="card-title">Card Title 1</h3>
<p class="card-text">Lorem ipsum dolor sit amet, consectetur adipiscing elit. Vivamus lacinia odio vitae vestibulum vestibulum.</p>
</div>
</div>
<div class="card">
<img src="https://via.placeholder.com/300x150" alt="Card Image">
<div class="card-content">
<h3 class="card-title">Card Title 2</h3>
<p class="card-text">Cras pulvinar mattis nunc sed blandit libero volutpat. Aenean et tortor at risus viverra adipiscing.</p>
</div>
</div>
<div class="card">
<img src="https://via.placeholder.com/300x150" alt="Card Image">
<div class="card-content">
<h3 class="card-title">Card Title 3</h3>
<p class="card-text">Integer quis auctor elit sed vulputate mi sit amet mauris commodo quis imperdiet massa.</p>
</div>
</div>
<div class="card">
<img src="https://via.placeholder.com/300x150" alt="Card Image">
<div class="card-content">
<h3 class="card-title">Card Title 4</h3>
<p class="card-text">Fusce id velit ut tortor pretium viverra suspendisse potenti nullam ac tortor vitae purus.</p>
</div>
</div>
</div>
</body>
</html>
Explanation:
Responsive Design: The
grid-template-columns: repeat(auto-fill, minmax(200px, 1fr));
creates a responsive grid that adapts to different screen sizes.Hover Effect: The
transform: translateY(-5px);
adds a subtle hover effect to each card.
Live Demo:
Check out this CodePen for the responsive card layout: Responsive Card Layout.
Example 2: Complex Web Page Layout
Here's an example of how you can use CSS Grid to create a complex web page layout.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Complex Web Page Layout</title>
<style>
.grid-container {
display: grid;
grid-template-areas:
"header header header"
"sidebar content content"
"footer footer footer";
grid-template-columns: 1fr 2fr 2fr;
grid-template-rows: 100px auto 50px;
gap: 10px;
height: 100vh;
}
.header {
grid-area: header;
background-color: #8ca0ff;
display: flex;
align-items: center;
justify-content: center;
font-size: 24px;
color: white;
}
.sidebar {
grid-area: sidebar;
background-color: #f4f4f4;
padding: 20px;
font-size: 18px;
border-right: 1px solid #ddd;
}
.content {
grid-area: content;
padding: 20px;
background-color: #ffffff;
}
.footer {
grid-area: footer;
background-color: #8ca0ff;
display: flex;
align-items: center;
justify-content: center;
font-size: 18px;
color: white;
}
</style>
</head>
<body>
<div class="grid-container">
<div class="header">Header</div>
<div class="sidebar">Sidebar</div>
<div class="content">Content</div>
<div class="footer">Footer</div>
</div>
</body>
</html>
Explanation:
Grid Template Areas: The
grid-template-areas
property is used to define named grid areas, making it easy to visualize and manage complex layouts.Responsive Layout: The layout is defined using fractions, allowing it to adapt to different screen sizes.
Live Demo:
Explore the complex web page layout on CodePen: Complex Web Page Layout.
6. Additional Resources
To further deepen your understanding of CSS Grid, here are some valuable resources and tutorials:
CSS Grid Layout Module - MDN Web Docs: Comprehensive documentation and examples from MDN.
CSS-Tricks: A Complete Guide to Grid: An extensive guide covering all aspects of CSS Grid.
CSS Grid Garden: An interactive game to practice CSS Grid concepts.
7. Interactive Practice and Games
Practicing CSS Grid can be both fun and educational with these interactive games and exercises:
CSS Grid Garden
URL: CSS Grid Garden
Description: An engaging game that teaches CSS Grid through gardening puzzles. You'll solve different challenges to water the garden using grid properties.
Grid Critters
URL: Grid Critters
Description: A paid interactive course that turns learning CSS Grid into a fun adventure with creatures and challenges. It's highly engaging and offers a unique way to understand grid concepts.
Flexbox Froggy
URL: Flexbox Froggy
Description: Although it's focused on Flexbox, this game is a great companion to CSS Grid learning. It teaches Flexbox concepts through fun puzzles with frogs.
CSS Grid Generator
URL: CSS Grid Generator
Description: A helpful tool to visually create grid layouts and generate CSS code. It's perfect for experimenting with different grid configurations.
8. Conclusion
CSS Grid is a powerful and versatile tool for creating responsive and dynamic web layouts. By mastering its properties and practicing with real-world examples and interactive games, you can unlock endless design possibilities for your web projects. Start experimenting with CSS Grid today, and elevate your web design skills to new heights!
If you enjoyed this guide, don't forget to share it with your friends and colleagues. Feel free to leave a comment below with your thoughts or questions. Happy designing!
Subscribe to my newsletter
Read articles from Trilokesh Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
