How to Use Filter and Map Methods in JavaScript
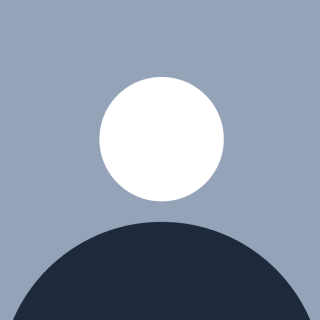
Filter
The filter
method creates a new array with all elements that pass a test implemented by the provided function. It doesn't change the original array but returns a new one based on the specified condition.
Example:
const products = [
{ id: 1, name: "Laptop", category: "Electronics", price: 999.99, inStock: true },
{ id: 4, name: "Running Shoes", category: "Sports", price: 89.99, inStock: true },
{ id: 5, name: "Coffee Maker", category: "Home", price: 79.99, inStock: true },
{ id: 6, name: "Tennis Racket", category: "Sports", price: 129.99, inStock: false },
{ id: 7, name: "Smart Watch", category: "Electronics", price: 299.99, inStock: true },
];
// Filter function to get in-stock electronics under $300
const filterProducts = (products) => {
return products.filter(product =>
product.category === "Electronics" &&
product.price < 300 &&
product.inStock
);
};
const filteredProducts = filterProducts(products);
console.log(filteredProducts);
[
{ id: 7, name: "Smart Watch", category: "Electronics", price: 299.99, inStock: true }
]
In this example, the filter
method goes through each number in the numbers
array. If the number is even (number % 2 === 0
), it is added to the new array evenNumbers
.
const users = [
{ name: "Alice", age: 25 },
{ name: "Bob", age: 30 },
{ name: "Charlie", age: 35 },
{ name: "David", age: 20 }
];
// Filter users older than 25
const olderUsers = users.filter(user => user.age > 25);
console.log(olderUsers);
// Output: [{ name: "Bob", age: 30 }, { name: "Charlie", age: 35 }]
Map
The map
method creates a new array with the results of applying a provided function to each element in the original array. It doesn't change the original array but transforms each element based on the function's logic.
Example:
Suppose you have an array of numbers and you want to double each number:
const numbers = [1, 2, 3, 4, 5];
const doubledNumbers = numbers.map(number => number * 2);
console.log(doubledNumbers);
// Output: [2, 4, 6, 8, 10]
In this example, the map
method goes through each number in the numbers
array and multiplies it by 2, creating a new array doubledNumbers
with the results.
const products = [
{ name: "Laptop", price: 1000, category: "Electronics" },
{ name: "Shirt", price: 50, category: "Clothing" },
{ name: "Phone", price: 500, category: "Electronics" },
{ name: "Shoes", price: 80, category: "Clothing" }
];
// Filter electronics and extract their names
const electronicProductNames = products
.filter(product => product.category === "Electronics")
.map(product => product.name);
console.log(electronicProductNames);
// Output: ["Laptop", "Phone"]
Subscribe to my newsletter
Read articles from Pawan S directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Pawan S
Pawan S
Understanding technology.