Aspect-Oriented Programming in Spring: Simplifying Cross-Cutting Concerns

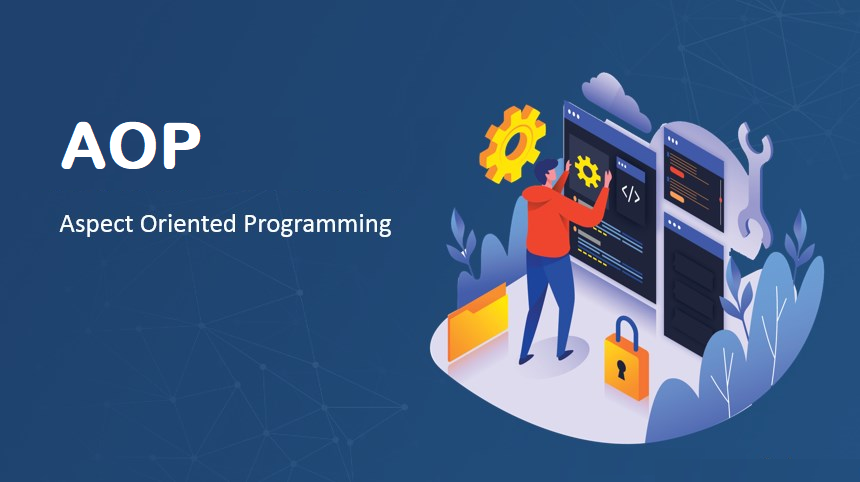
Aspect-Oriented Programming (AOP) is a programming paradigm that allows you to separate cross-cutting concerns from the main business logic of your application. This separation helps keep your code clean, modular, and easier to maintain.
Cross cutting concerns are aspects of program that affect multiple parts of an application. These are common tasks that need to be applied across different modules or layers but aren't part of the main functionality.
For Eg.
Logging: Adding logs to various parts of the application.
Security: Like checking permissions at different parts of the application. etc.
1. Importance
Here are some points that tell us why and how AOP is important in development:
Separation of Concerns
Separating cross cutting concerns from business logic.
Improved Code Readability
Due to the separation of concerns, the main code remains clean and focused on its primary function.
Easier Maintenance
Cross-cutting concerns in aspects makes it easier to update and maintain them. If you need to change your logging format, you do it in one place rather than updating multiple methods.
Reusability
Aspects can be reused across different parts of your application, promoting DRY (Don't Repeat Yourself) principles.
A security aspect that checks user permissions can be applied to various methods in different classes.
Now, lets discuss some technical terminology
2. Core Concepts of AOP
Aspect:
Core concept in Aspect-Oriented Programming (AOP) that encapsulates a cross-cutting concern.
Its a module that defines how and where certain code should be executed in relation to other parts of your application.
By defining an aspect, you can apply common behaviors, such as Logging or a security without cluttering the core business logic.
Annotation used :
@Aspect
Join Point:
Are specific points in your program where you can attach additional behavior.
- Think of them as a Check points in your code where you can add extra actions without modifying the main code directly.
Advice: Types (before, after, around, etc.)
Advice refers to the code that runs at a Join Point. Its essentially the action taken by an aspect at specific moments in your program.
Types of Advice:
Before Advice: This code runs before the Join point method executes.
@Before("execution(* com.example.service.*.*(..))") public void logBefore(JoinPoint joinPoint) { System.out.println("Before executing: " + joinPoint.getSignature().getName()); }
After Advice: This code runs after the join point method has finished executing, regardless of whether it is completed successfully or threw an exception.
@After("execution(* com.example.service.*.*(..))") public void logAfter(JoinPoint joinPoint) { System.out.println("After executing: " + joinPoint.getSignature().getName()); }
Around Advice: This code surrounds the join point method, running before and after it. It can also decide whether to proceed with the join point method or skip it.
@Around("execution(* com.example.service.*.*(..))") public Object logAround(ProceedingJoinPoint joinPoint) throws Throwable { System.out.println("Before method: " + joinPoint.getSignature().getName()); Object result = joinPoint.proceed(); // Proceed with the method System.out.println("After method: " + joinPoint.getSignature().getName()); return result; }
Summary**:**
Before Advice: Runs before the join point method.
Annotation:
@Before
After Advice: Runs after the join point method, regardless of the outcome.
Annotation:
@After
Around Advice: Runs before and after the join point method and can control whether the method proceeds.
Annotation:
@Around
After Returning Advice: Runs after the join point method successfully completes.
Annotation:
@AfterReturning
After Throwing Advice: Runs if the join point method throws an exception.
Annotation:
@AfterThrowing
Pointcut:
Pointcut in AOP is a way to specify where in your code an aspect's advice should be applied.
It defines which join points should be trigger the advice.
Examples:
Method Execution:
A pointcut that matches when certain methods are executed.
"execution(* com.example.service.*.*(..))"
matches all methods in thecom.example.service
package.
Object Instantiation:
A pointcut that matches when objects are created.
"execution(
com.example.service.User.new
(..))"
matches the creation ofUser
objects.
Field Access:
A pointcut that matches when fields are accessed or modified.
"get(* com.example.service.User.*)"
matches all field getters in theUser
class.
Annotation:
@Pointcut
3. Implementing AOP in Spring
Here's a step-by-step guide to implementing Aspect-Oriented Programming (AOP) in a Spring application:
Setting up a Spring project for AOP
Use Spring Initializer (https://start.spring.io/) to create a new Spring Boot project.
Add the following dependencies:
Spring Web
Spring AOP
Creating Aspects Class:
import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Pointcut; import org.aspectj.lang.annotation.Before; import org.springframework.stereotype.Component; @Aspect @Component public class LoggingAspect { @Pointcut("execution(* com.example.service.*.*(..))") public void serviceLayer() {} @Before("serviceLayer()") public void logBefore() { System.out.println("Executing method in service layer"); } @After("serviceLayer()") public void logAfter(JoinPoint joinPoint) { System.out.println("After executing: " + joinPoint.getSignature()); } @AfterReturning(pointcut = "serviceLayer()", returning = "result") public void logAfterReturning(JoinPoint joinPoint, Object result) { System.out.println("Method returned: " + result); } @AfterThrowing(pointcut = "serviceLayer()", throwing = "ex") public void logAfterThrowing(JoinPoint joinPoint, Exception ex) { System.out.println("Method threw: " + ex.getMessage()); } @Around("serviceLayer()") public Object logAround(ProceedingJoinPoint joinPoint) throws Throwable { System.out.println("Before executing: " + joinPoint.getSignature()); Object result = joinPoint.proceed(); System.out.println("After executing: " + joinPoint.getSignature()); return result; } }
Defining Pointcuts Expressions
execution(* com.example.service.*.*(..))
: Matches all methods in thecom.example.service
package.@Pointcut
Annotation: Used to define reusable pointcut expressions.In the example above, the
serviceLayer
method is a pointcut that matches all methods in the service layer.
Writing different types of Advice (Before, After, After Returning, After Throwing, Around)
Already explained above with example.
Create a Main Application Class
import org.springframework.boot.CommandLineRunner; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.context.annotation.Bean; @SpringBootApplication public class AopDemoApplication { public static void main(String[] args) { SpringApplication.run(AopDemoApplication.class, args); } @Bean public CommandLineRunner run(MyService myService) { return args -> { System.out.println(myService.process()); }; } }
Run the Application
Start the application and observe the console output to see the effects of the different advices.
4. AOP Use Cases
Logging
Security
Transaction management
Caching
Performance monitoring
5. Best Practices for AOP in Spring
Keeping aspects focused and modular
Single Responsibility: Each aspect should have a single responsibility. Avoid creating monolithic aspects that handle multiple concerns.
Separation of Concerns: Use aspects to handle cross-cutting concerns like logging, security, or transactions, but keep business logic separate from aspects.
Minimize Complexity
Simple Pointcuts: Write pointcuts that are as simple as possible. Complex expressions can be difficult to understand and maintain.
Avoid Overusing AOP: Not all concerns need AOP. Use it where it adds clear value, such as logging, caching, or transactions.
Optimize Performance
Avoid Expensive Operations: Be mindful of performance. Avoid placing expensive operations in advice, especially in
@Around
advice, as it can affect application performance.Benchmark and Profile: Regularly benchmark and profile your application to ensure AOP does not introduce significant performance overhead.
Be Careful with
@Around
AdviceProceed Carefully:
@Around
advice can alter the behavior of the target method. Ensure it’s tested thoroughly to avoid unexpected side effects.Control Method Execution: Ensure you call
joinPoint.proceed()
appropriately in@Around
advice to allow method execution or to skip it.
Manage Advice Execution Order
Order of Advice: Use the
@Order
annotation to control the order in which multiple advices are executed if necessary.Ordering of Aspects: In cases where multiple aspects are applied to the same join point, manage their execution order to ensure correct behavior.
Handle Exceptions Properly
Exception Handling in Advice: Handle exceptions appropriately in advice, especially in
@AfterThrowing
and@Around
advice.Avoid Swallowing Exceptions: Ensure that exceptions are not swallowed unless you have a specific reason. Log or propagate them as needed.
Test Aspects Thoroughly
Unit Testing: Write unit tests for aspects to verify that they behave as expected. Use mocks and spies to test advice behavior in isolation.
Integration Testing: Include integration tests to ensure that aspects interact correctly with the rest of your application.
Use Proper Annotation
Appropriate Annotations: Use the correct annotations (
@Before
,@After
,@AfterReturning
,@AfterThrowing
,@Around
) based on the type of advice needed.Pointcut Annotations: Use
@Pointcut
annotations to define reusable pointcut expressions for better maintainability.
Document Aspect Behavior
- Documentation: Document the purpose and behavior of each aspect clearly. This helps other developers understand the purpose of the aspect and its impact on the application.
Monitor Aspect Usage
Application Monitoring: Monitor the impact of aspects in production to ensure they are functioning correctly and not introducing issues.
Configuration Management: Use configuration to enable or disable aspects in different environments (e.g., development, testing, production).
Conclusion
- AOP improves code modularity, reusability, and maintainability by isolating concerns. Enhances code quality and flexibility, making it easier to manage and adapt to changes. Do add aspects in your application wherever applicable.
“Signing off—remember, if your code’s a mess, just call it ‘experimental’!”
Happy Coding !!!
Subscribe to my newsletter
Read articles from Prashant Bale directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Prashant Bale
Prashant Bale
With 17+ years in software development and 14+ years specializing in Android app architecture and development, I am a seasoned Lead Android Developer. My comprehensive knowledge spans all phases of mobile application development, particularly within the banking domain. I excel at transforming business needs into secure, user-friendly solutions known for their scalability and durability. As a proven leader and Mobile Architect.