Advanced Topics and Tips

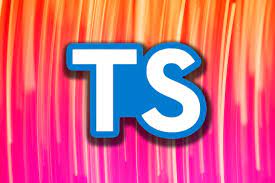
Welcome to the final day of our TypeScript series!
Today, we’ll dive into advanced TypeScript topics and share some tips to enhance your TypeScript development experience. We'll cover topics such as advanced type manipulation, type inference, performance considerations, and more. These insights will help you leverage TypeScript's full potential and write robust, efficient, and maintainable code.
1. Advanced Type Manipulation
1.1 Mapped Types
Mapped types allow you to create new types by transforming existing types.
Example:
type User = {
id: number;
name: string;
email: string;
};
type ReadOnlyUser = {
readonly [K in keyof User]: User[K];
};
const user: ReadOnlyUser = {
id: 1,
name: 'Alice',
email: 'alice@example.com',
};
// user.id = 2; // Error: Cannot assign to 'id' because it is a read-only property
1.2 Conditional Types
Conditional types enable you to create types that depend on a condition.
Example:
type IsString<T> = T extends string ? true : false;
type A = IsString<string>; // true
type B = IsString<number>; // false
1.3 Utility Types
TypeScript provides several utility types that simplify common type transformations.
Example:
type User = {
id: number;
name: string;
email?: string;
};
type PartialUser = Partial<User>; // All properties are optional
type RequiredUser = Required<User>; // All properties are required
type ReadOnlyUser = Readonly<User>; // All properties are read-only
type PickUser = Pick<User, 'id' | 'name'>; // Pick specific properties
type OmitUser = Omit<User, 'email'>; // Omit specific properties
2. Type Inference and Type Guards
2.1 Type Inference
TypeScript can often infer the types of variables and functions, reducing the need for explicit type annotations.
Example:
let x = 10; // TypeScript infers x as number
function add(a: number, b: number) {
return a + b; // TypeScript infers the return type as number
}
2.2 Type Guards
Type guards allow you to narrow down the type of a variable within a conditional block.
Example:
function printId(id: number | string) {
if (typeof id === 'string') {
// id is of type string here
console.log(id.toUpperCase());
} else {
// id is of type number here
console.log(id.toFixed(2));
}
}
3. Generics
Generics provide a way to create reusable components that work with a variety of types.
Example:
function identity<T>(arg: T): T {
return arg;
}
let output1 = identity<string>('Hello'); // output1 is of type string
let output2 = identity<number>(42); // output2 is of type number
Generics can also be used in classes and interfaces.
Example:
class GenericNumber<T> {
zeroValue: T;
add: (x: T, y: T) => T;
constructor(zeroValue: T, add: (x: T, y: T) => T) {
this.zeroValue = zeroValue;
this.add = add;
}
}
let myGenericNumber = new GenericNumber<number>(0, (x, y) => x + y);
console.log(myGenericNumber.add(5, 10)); // Output: 15
4. Performance Considerations
4.1 Compiler Options
TypeScript provides several compiler options that can affect performance. Some key options include:
incremental
: Enables incremental compilation for faster rebuilds.skipLibCheck
: Skips type checking of declaration files for faster compilation.strict
: Enables all strict type-checking options for improved code quality.
Example:
// tsconfig.json
{
"compilerOptions": {
"incremental": true,
"skipLibCheck": true,
"strict": true
}
}
4.2 Type-Only Imports and Exports
Use type-only imports and exports to avoid including unnecessary code in the compiled output.
Example:
// Use 'import type' for type-only imports
import type { User } from './types';
// Use 'export type' for type-only exports
export type { User };
5. Working with Legacy Code
Gradually introducing TypeScript to a legacy JavaScript codebase can be challenging. Here are some tips:
Start with TypeScript: Rename a few
.js
files to.ts
and fix any type errors.Use
any
Sparingly: Whileany
can help you get started, gradually replace it with more specific types.Incremental Adoption: Convert one module or component at a time to TypeScript.
Leverage Type Declarations: Create type declaration files (
.d.ts
) for third-party libraries that lack TypeScript support.
6. TypeScript with Modern JavaScript
6.1 ES6+ Features
TypeScript supports modern JavaScript features like async/await
, destructuring, and spread/rest operators.
Example:
// Async/Await
async function fetchData(url: string): Promise<any> {
const response = await fetch(url);
return response.json();
}
// Destructuring
const user = { id: 1, name: 'Alice' };
const { id, name } = user;
// Spread Operator
const userWithAge = { ...user, age: 25 };
6.2 Decorators
Decorators are a powerful feature for meta-programming in TypeScript.
Example:
function Log(target: any, key: string) {
let value = target[key];
const getter = () => {
console.log(`Get: ${key} => ${value}`);
return value;
};
const setter = (newVal: any) => {
console.log(`Set: ${key} => ${newVal}`);
value = newVal;
};
Object.defineProperty(target, key, {
get: getter,
set: setter,
enumerable: true,
configurable: true,
});
}
class Person {
@Log
public name: string;
constructor(name: string) {
this.name = name;
}
}
const person = new Person('Alice');
person.name = 'Bob';
console.log(person.name);
7. Tips and Tricks
Type Aliases: Use type aliases to create more readable and maintainable code.
type Point = { x: number; y: number }; type ID = number | string;
Union and Intersection Types: Combine multiple types using union (
|
) and intersection (&
) operators.type Admin = { role: 'admin'; privileges: string[] }; type User = { name: string }; type AdminUser = Admin & User;
Type Assertions: Use type assertions to override inferred types when necessary.
let someValue: any = 'this is a string'; let strLength: number = (someValue as string).length;
Non-null Assertion Operator: Use the non-null assertion operator (
!
) to assert that a value is non-null.let value: string | null = 'Hello'; console.log(value!.toUpperCase());
Summary
Today, we covered advanced TypeScript topics and shared practical tips to enhance your development experience. From advanced type manipulation and generics to performance considerations and modern JavaScript features, these insights will help you write robust, efficient, and maintainable TypeScript code. As you continue your TypeScript journey, remember to leverage these techniques and best practices to unlock the full potential of this powerful language.
Thank you for following along with this series! Happy coding!
Subscribe to my newsletter
Read articles from Anuj Kumar Upadhyay directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Anuj Kumar Upadhyay
Anuj Kumar Upadhyay
I am a developer from India. I am passionate to contribute to the tech community through my writing. Currently i am in my Graduation in Computer Application.