Promise.all()
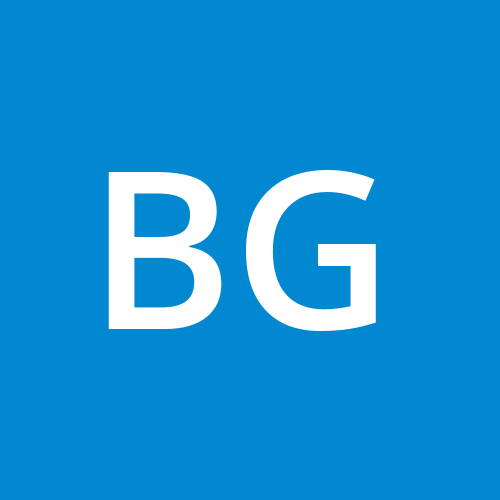
1 min read
The Promise.all() accepts an array of promises and returns a promise that resolves when all of the promises in the array are fulfilled or when the iterable contains no promises. It rejects with the reason of the first promise that rejects.
The code for Promise.all() is as follows:-
let promise1 = Promise.resolve("First completed");
let promise2 = Promise.resolve("Second incompleted");
let promise3 = new Promise((resolve,reject)=>{
setTimeout(resolve,2000,"Third completed");
})
Promise.all([promise1,promise2,promise3]).then((values)=>{
console.log(values);
}).catch(err=>console.log("Err",err))
If any promise fail to resolve, then it will return the reason of the first rejected Promise.
The polyfill for the Promise.all() is as follows:-
let promise1 = Promise.resolve("First completed");
let promise2 = Promise.resolve("Second incompleted");
let promise3 = new Promise((resolve,reject)=>{
setTimeout(resolve,2000,"Third completed");
})
//polfill for promise.all
let PromiseAll = (promises)=>{
let result = []
let count = 0;
return new Promise((resolve,reject)=>{
promises.forEach((promise,index)=>{
promise.then((value)=>{
result[index] = value;
count +=1;
if(count == promises.length){
resolve(result);
}
}).catch(err=>reject(err))
})
})
}
PromiseAll([promise1,promise2,promise3]).then((data)=>{
console.log(data);
}).catch(err=>console.log(err))
1
Subscribe to my newsletter
Read articles from Bidhubhushan Gahan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
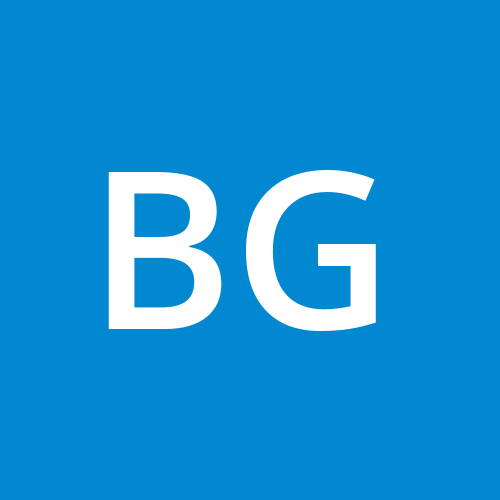