Scheduling Tasks in Node.js with the Cronjob
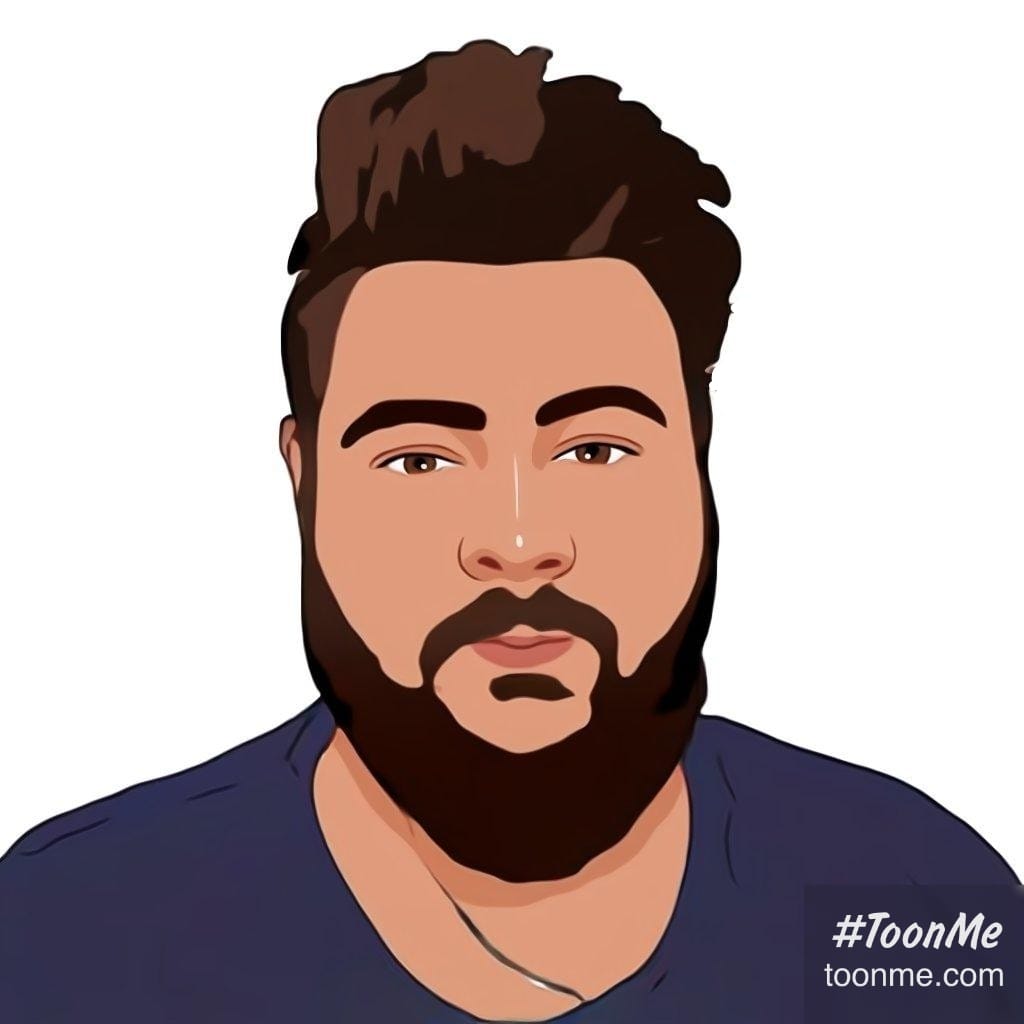
Table of contents
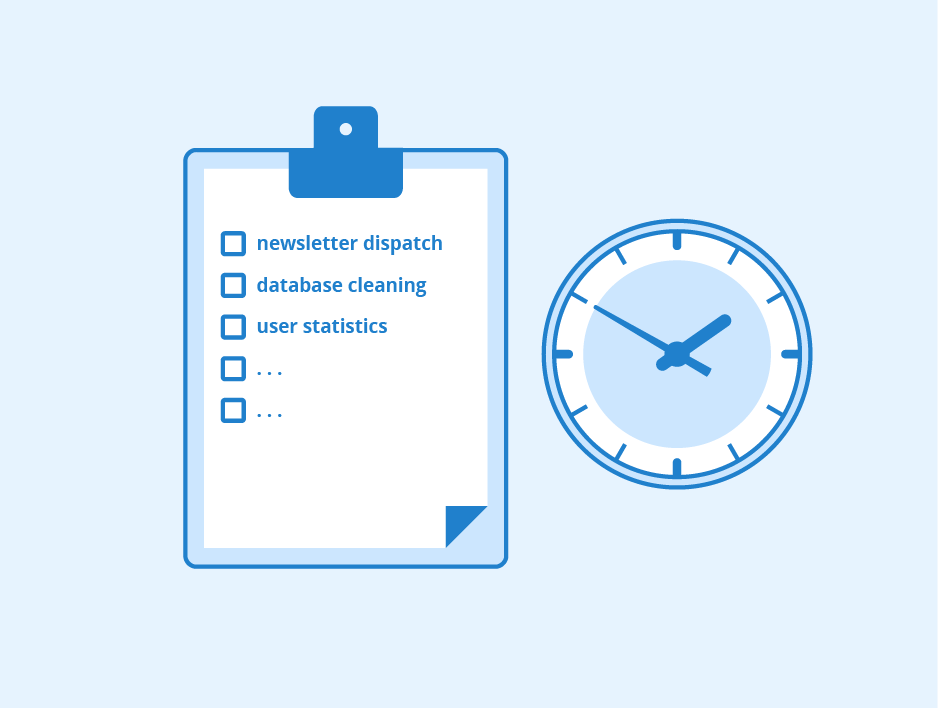
In the fast-paced world of web development, automating repetitive tasks can save significant time and effort. One common requirement is scheduling tasks, such as making API calls at specific times. In this blog post, we'll explore how to use the node-cron library in Node.js to schedule a task that makes an API call every night at 12 AM.
What is CronJob?
The cron command-line utility is a job scheduler on Unix-like operating systems. Users who set up and maintain software environments use cron to schedule jobs, also known as cron jobs, to run periodically at fixed times, dates, or intervals.
What is node-cron?
node-cron is a popular library in the Node.js ecosystem for scheduling tasks. It allows you to run functions at specified times and intervals using cron syntax, which is a standard way to define scheduled tasks.
Setting Up Your Node.js Project First
You'll need to have Node.js installed on your machine. If you haven't done this yet, download and install it from the official Node.js website.
Next, create a new Node.js project. Open your terminal and run the following commands:
create a folder cron-scheduler
cd cron-scheduler
npm init -y
2. Installing node-cron
To use node-cron, you need to install it along with axios (for making API calls). In your project directory, run:
npm install node-cron axios
3. Creating the Scheduled Task
Now, let's write the code to schedule an API call. Create a file named index.js in your project directory and open it in your favorite code editor.
const cron = require('node-cron');
const axios = require('axios');
// Define the scheduled task
cron.schedule('0 0 * * *', () => {
console.log('Running a task every midnight');
// Replace with the URL of the API you want to call
const apiUrl = 'https://api.restful-api.dev/objects';
axios.get(apiUrl)
.then(response => {
console.log('Data fetched:', response.data);
})
.catch(error => {
console.error('Error making API call:', error);
});
});
Understanding the Code
Importing Modules: We import node-cron and axios. The cron library is used for scheduling, while axios is used for making HTTP requests.
Cron Syntax: The string '0 0 *' specifies the task to run at midnight every day. This is the cron syntax, where each part represents a time unit (minute, hour, day of the month, month, day of the week).
Scheduling the Task: We use cron. Schedule to define and schedule the task. The function passed to schedule will run at the specified time.
Running Your Scheduler
To start the scheduler, simply run your script using Node.js:
node index.js
Wrapping Up
Scheduling tasks in Node.js using node-cron is a powerful way to automate routine tasks, such as making API calls or sending emails. By following this guide, you've set up a basic task scheduler that can be expanded to include more complex tasks or additional scheduling needs.
Remember, while automating tasks can greatly improve efficiency, it's essential to monitor these tasks to ensure they are functioning correctly and handling errors gracefully. Happy coding!
Got feedback? Drop them in the comment section!
Subscribe to my newsletter
Read articles from Random Dude directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
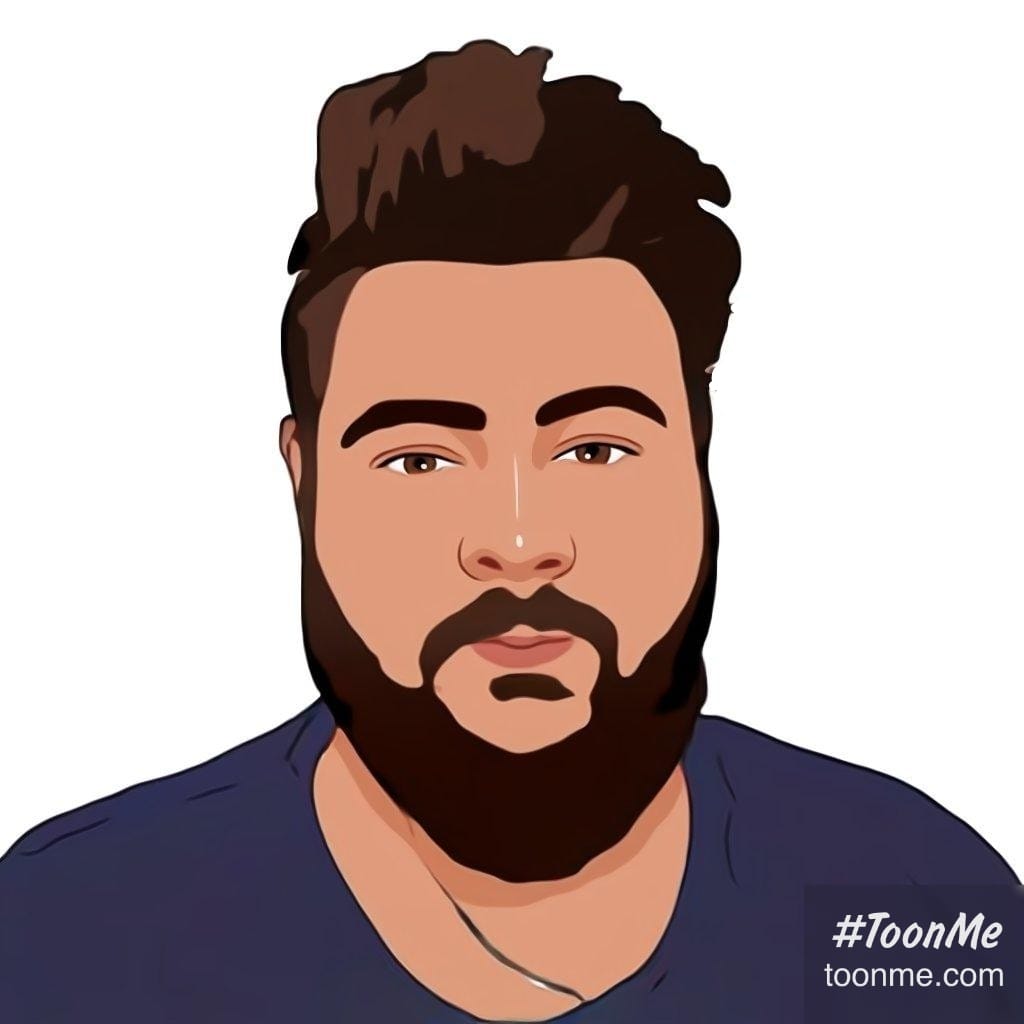
Random Dude
Random Dude
Passionate Software Engineer with 4 years of experience in developing web applications and backend systems using MERN stack. Skilled at writing clear, concise code that is easy to maintain and troubleshoot. Experienced in working with both small and large teams across multiple projects and companies. Able to work independently of remote locations or in office environments as needed by the company.