Optimizing Web Performance with Lighthouse
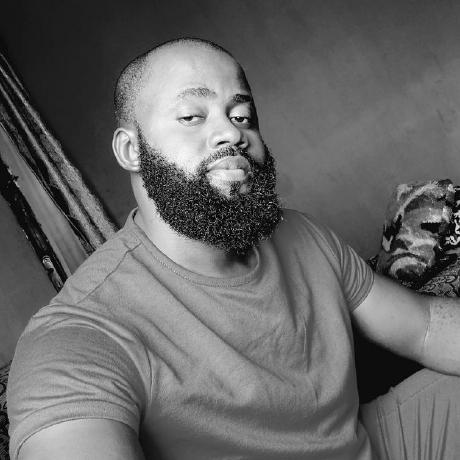
The longer it takes for a user to do something on your website, the more likely they are to give up.
Web performance refers to how quickly web pages and applications load and render in a web browser. This includes how easy it is for users to interact with the website and how reliable the site is. When users visit websites, the first thing they are likely to notice is how fast or slow it was to load the website's content. A fast-loading site allows the user to interact with its content sooner, enhancing their overall user experience. Poorly performing websites are slow to display contents and slow to respond to input. This increases sites abandonment. At its worst, poor performance causes content to be completely inaccessible. Poor web performance negatively impacts SEO rankings, as search engines, such as Google, use web performance as a metric for SEO ranking, with fast-loading and responsive websites topping the ranks for better user experience and engagement.
In this article, we will focus on Lighthouse, an open-source tool from Google used for auditing websites for performance, accessibility, SEO, and other best practices. It generates a report on how well a website performs and provides recommendations on how to improve its performance.
Google Lighthouse: What It Is & How To Use It
Google Lighthouse is a free tool used to assess and improve the overall quality and user experience of web pages and applications.
It can be used on any publicly available website. It works by performing audits in five main website optimization categories:
Performance
Accessibility
Best Practice
SEO
Setting Up Lighthouse and Running Audits
There are different ways to set up and run Lighthouse
: it can be done using Chrome DevTools, from the command line, as a Node module, as a browser extension, or through the PageSpeed Insights web page.
Run Lighthouse in Chrome DevTools
Using Chrome DevTools, audits can be run directly from the browser. Here are the steps:
Open the website to audit.
Open Chrome DevTools.
Click the Lighthouse tab.
To the left is the page that will be audited. To the right is the Lighthouse panel of Chrome DevTools.
- Click the Analyze page load button, wait for 30 to 60 seconds, and the result of the audit is displayed on the screen.
Run Lighthouse in Command Line
Install Node.js (LTS) and npm (Node Package Manager)
Install the Lighthouse command-line interface globally using npm, run the following command in your terminal
npm install -g lighthouse
- Run the Lighthouse command followed by the URL to be audited in your terminal. This will automatically generate a report in HTML format.
lighthouse https://developer.mozilla.org/en-US/
To choose the output format, use the --output
flag and specify the format. Common formats include html
, json
, and csv
.
lighthouse https://developer.mozilla.org/en-US/ --output csv --output-path ./report.csv
To see all the available options to configure Lighthouse, run the following in the terminal:
lighthouse --help
Run lighthouse programmatically as a node module
Install Node.js (LTS) and npm (Node Package Manager)
Install the required dependencies:
npm install lighthouse chrome-launcher
- Create a JavaScript file, for example,
lighthouse_report.js
, and paste this:
// Import required npm packages
import lighthouse from 'lighthouse';
import * as chromeLauncher from 'chrome-launcher';
import fs from 'fs';
// Function to run Lighthouse
async function runLighthouse(url) {
// Launch Chrome using chrome-launcher
const chrome = await chromeLauncher.launch({ chromeFlags: ['--headless'] });
// Define Lighthouse options
const options = {
logLevel: 'info',
output: 'html', // Change to 'json' or 'csv' if needed
port: chrome.port,
};
// Run Lighthouse
const runnerResult = await lighthouse(url, options);
// Save the Lighthouse report
const reportHtml = runnerResult.report;
fs.writeFileSync('lighthouse-report.html', reportHtml);
// Close Chrome
await chrome.kill();
// Log the scores
console.log(`Lighthouse scores for ${url}:`);
console.log(
`Performance: ${runnerResult.lhr.categories.performance.score * 100}`,
);
console.log(
`Accessibility: ${runnerResult.lhr.categories.accessibility.score * 100}`,
);
console.log(
`Best Practices: ${
runnerResult.lhr.categories['best-practices'].score * 100
}`,
);
console.log(`SEO: ${runnerResult.lhr.categories.seo.score * 100}`);
}
// Run Lighthouse on a specific URL
runLighthouse('https://developer.mozilla.org/en-US/');
The code above will launch Chrome in headless mode, run the audits, output the result to lighthouse-report.html
, and print the scores for the categories in the console.
Running Lighthouse on PageSpeed Insights web page
Open the PageSpeed Insights web page.
Paste the URL of the webpage to audit into the input form provided and click on the Analyze button.
Understanding Lighthouse Reports
Understanding the Lighthouse report is important for interpreting and implementing the recommendations in it. Let's take an in-depth look at the report generated across the five main categories, and how to interpret the scores for each. Each category is assigned a score from 0 to 100.
Performance Audit
This measures how quickly web pages load and become interactive to the user's input. The performance score is obtained by combining six different web performance metrics. These metrics are weighted, and as such, those with heavier weights have a greater effect on web page performance.
- First Contentful Paint First Contentful Paint (FCP) measures the time it takes for the first piece of content to appear on the page. This content can include text, images, or SVG elements. Webpages should aim for an FCP of 1.8 seconds or less. A fast FCP reassures users that the webpage is loading, reducing the likelihood of them abandoning the page.
This table shows how to interpret FCP score:
FCP time(in seconds) | Color-coding |
0–1.8 | Green (fast) |
1.8–3 | Orange (moderate) |
Over 3 | Red (slow) |
- Speed Index Speed Index (SI) measures how quickly content becomes visible during page load. The value is obtained by visually analyzing screenshots of the website during the page load process.
This table shows how to interpret Speed Index score:
Speed Index(in seconds) | Color-coding |
0–3.4 | Green (fast) |
3.4–5.8 | Orange (moderate) |
Over 5.8 | Red (slow) |
- Largest Contentful Paint Largest Contentful Paint (LCP) measures the time it takes for the largest single content element to become visible on the screen. LCP is one of Google's Core Web Vitals metrics that impacts search result ranking.
This table shows how to interpret LCP score:
LCP time(in seconds) | Color-coding |
0-2.5 | Green (fast) |
2.5-4 | Orange (moderate) |
Over 4 | Red (slow) |
- Total Blocking Time Total Blocking Time (TBT) measures the amount of time between the First Contentful Paint (FCP) and the Time to Interactive (TTI) during which the webpage is unable to respond to user input, such as mouse clicks, screen taps, or keyboard presses.
TBT time(in milliseconds) | Color-coding |
0–200 | Green (fast) |
200-600 | Orange (moderate) |
Over 600 | Red (slow) |
- Cumulative Layout Shift (CLS) Cumulative Layout Shift (CLS) measures how much the visible content of webpages shifts unexpectedly during the entire lifecycle of the page. A shift happens when visible content changes position on the page from where it was originally rendered.
Accessibility Audit
This audit focuses on how easy a website is for everyone to use, regardless of ability, such as users with visual impairments. Unlike the performance audit, each check in the accessibility category is a pass or a fail. A page does not get points for a partial pass. For example, if some images on a page have alt
attributes but others do not, the page gets zero for the "Image elements have alt
attributes" audit.
Common checks include:
Alternative text for images: This check ensures that all images have an
alt
attribute that describes their content.Color Contrast: This check ensures that the contrast between text and background is sufficient for readability
Empty Links (Links with Empty Text Content): This check ensures that links have descriptive text that tells the user where they lead.
Click here for more on the checks done during accessibility audit.
Best Practices Audit
This audit checks whether the webpage is built on modern standards of web development. It focuses on areas related to code quality, usability, performance, and security.
Common checks include:
Uses HTTPS: Ensures all resources are loaded over HTTPS for secure communication.
Avoids deprecated APIs: Checks that the page does not use outdated web APIs.
Allows users to paste into input fields: Ensures that input fields accept pasted content.
Has a
<meta name="viewport">
tag withwidth
orinitial-scale
: Ensures the page is responsive to different screen sizes.Displays images with correct aspect ratio: Verifies that images maintain their aspect ratio.
Properly defines charset: Ensures the character encoding is specified with
<meta charset="UTF-8">
.
SEO Audit
This audit analysis webpages for various factors that can affect its ranking in search engine.
Common checks include:
Page isn’t blocked from indexing: Ensures that search engines are allowed to index the page, making it eligible to appear in search results.
Document has a
<title>
element: Ensures the page includes a<title>
tag that provides a concise description of the content. The title gives screen reader users an overview of the page, and search engine users rely on it heavily to determine if a page is relevant to their search.Document has a meta description: Checks for a
<meta name="description">
tag that offers a brief summary of the page content.Page has a successful HTTP status code: Verifies that the page returns a successful HTTP status code, like
200 OK
, indicating the page is accessible.Pages with unsuccessful HTTP status codes may not be indexed properlyLinks have descriptive text: Ensures that links on the page have text that describes their destination.Descriptive link text helps search engines understand the content.
Links are crawlable: Checks that links can be followed by search engines, allowing them to discover and index the linked content.
robots.txt is valid: Verifies that the
robots.txt
file is properly configured to guide search engine crawlers.If therobots.txt
file is malformed, crawlers may not be able to understand how the website should be crawled or indexed.Image elements have
alt
attributes: Ensures all images havealt
attributes that provide descriptive text.Document has a valid
hreflang
attribute: Checks that thehreflang
attribute is used correctly to specify the language and regional targeting.
Addressing Performance Issues
Improving the performance of a website is a win-win situation. It creates a better experience for users and boosts SEO rankings, which is crucial to the website's success.
Common Performance Issues and Recommendations
Serve static assets with an efficient cache policy: When static assets like images, scripts, and stylesheets are not cached effectively, it leads to repeated downloads on subsequent visits, which makes loading slow. A long cache lifetime can speed up repeat visits to your page.
Reduce unused CSS When stylesheets contain
CSS
rules not used by any element on the page, it leads to longer load times. Stylesheets should be leaner. This reduces the amount of data transferred over the network. Also, deferringCSS
not used for above-the-fold content (content visible on the screen without scrolling) until needed improves the website's loading speed.Avoid large layout shifts Unexpected changes in the position of visible content can lead to a poor user experience. Ensure that images and video content always have width and height attributes. Ads, embeds, and late-loaded content also lead to layout shifts on websites. This can be avoided by reserving the space for them in the initial layout.
Avoid chaining critical requests A critical request chain is a series of dependent network requests important for page rendering. Long chains lead to slow load speeds, causing delays in rendering the initial content. Deferring non-critical resources when rendering the initial content and preloading critical resources like fonts or stylesheets in advance using the preload resource hint can improve performance.
Properly size images Serving desktop-sized images to mobile devices can use 2–4x more data than needed. Serve appropriately-sized images to improve load time. Use responsive image techniques (e.g.,
srcset
) to serve the appropriate image size based on the user's device.
Continuous Monitoring and Improvement
Continuous monitoring and improvement are crucial for maintaining the performance and quality of web applications over time. Lighthouse CI
is a tool that automates running Lighthouse audits on websites. A single Lighthouse report provides a snapshot of a website's performance at the time it was run. Lighthouse CI
shows what has changed over time, helping to identify the impact of a particular code change.
Setting Up Lighthouse CI
- Install Lighthouse CI:
npm install -g @lhci/cli
# or as a dev dependency
npm install --save-dev @lhci/cli
- Configuring Lighthouse CI
Create a Lighthouserc.js
file in the root directory. file will contain the configurations needed for Lighthouse CI
here is an eaxmple of a lighthouserc.js
file
module.exports = {
ci: {
collect: {
url: ['https://dandyschat.onrender.com/chat'],
startServerCommand: 'npm start',
numberOfRuns: 3,
},
assert: {
assertions: {
'categories:performance': ['error', { minScore: 0.9 }],
'categories:accessibility': ['error', { minScore: 0.9 }],
'categories:best-practices': ['error', { minScore: 0.9 }],
'categories:seo': ['error', { minScore: 0.9 }],
},
},
upload: {
target: 'temporary-public-storage',
},
},
};
The collect
section specifies the array of URLs to audit, the command to start the website's server, and the number of times to run the audit against each URL in the URL array
The assert
section defines assertions for different Lighthouse categories. These assertions set thresholds, and if they are not met, the CI process fails. Lighthouse CI supports three levels of assertions:
off
: ignore assertions warn
: displays failure messages as errors error
: displays failure messages as errors and exit Lighthouse CI with an error code.
In the upload
section, we set the location to upload the report, in this case, the temporary-public-storage
provided by Lighthouse CI.
- Running Lighthouse CI
Use the Lighthouse CI
command lhci autorun
to collect, assert, and upload the results.
Integrating Lighthouse CI into Your CI/CD Pipeline
Integrating Lighthouse CI into your CI/CD pipeline allows you to run performance audits automatically on every build or pull request.
- create a directory named
.github/workflows
, in the root directory, add this filelighthouse.yaml
. This file will hold the configuration for a new workflow.
mkdir .github
mkdir .github/workflows
touch lighthouse.yaml
- Add the following text to
lighthouse.yaml
name: Build project and run Lighthouse CI
on: [push]
jobs:
lhci:
name: Lighthouse CI
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v1
- name: Use Node.js 10.x
uses: actions/setup-node@v1
with:
node-version: 14.x
- name: npm install
run: |
npm install
- name: run Lighthouse CI
run: |
npm install -g @lhci/cli@0.6.x
lhci autorun --upload.target=temporary-public-storage || echo "LHCI failed!"
This setup contains a single job that will run whenever a new code is pushed to the repository. This job has four steps:
Check out the repository that
Lighthouse CI
will be run againstInstall and configure Node
Install required npm packages
Run
Lighthouse CI
and upload the results to temporary public storage.
- Commit and push the changes to Github, this will trigger the workflow automatically.
Conclusion
Lighthouse is an essential tool for web developers looking to optimize their website performance. Using Lighthouse leads to improved user experience, higher SEO rankings, better accessibility for all users, adherence to best practices, and continuous improvement for the webpage. We can use Lighthouse CI
to automate the process of running audits on webpages, providing us with a clear picture of the webpage performance over time.
Subscribe to my newsletter
Read articles from Madu Emelie directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
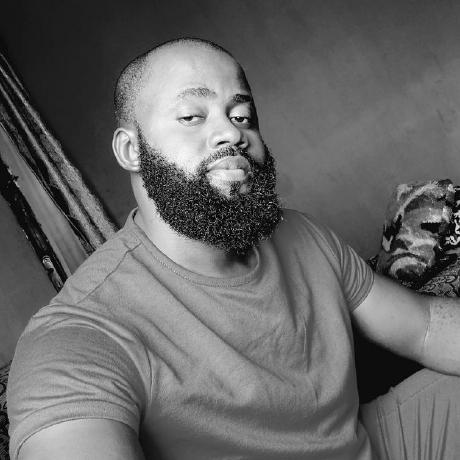