ADB commands Tutorial
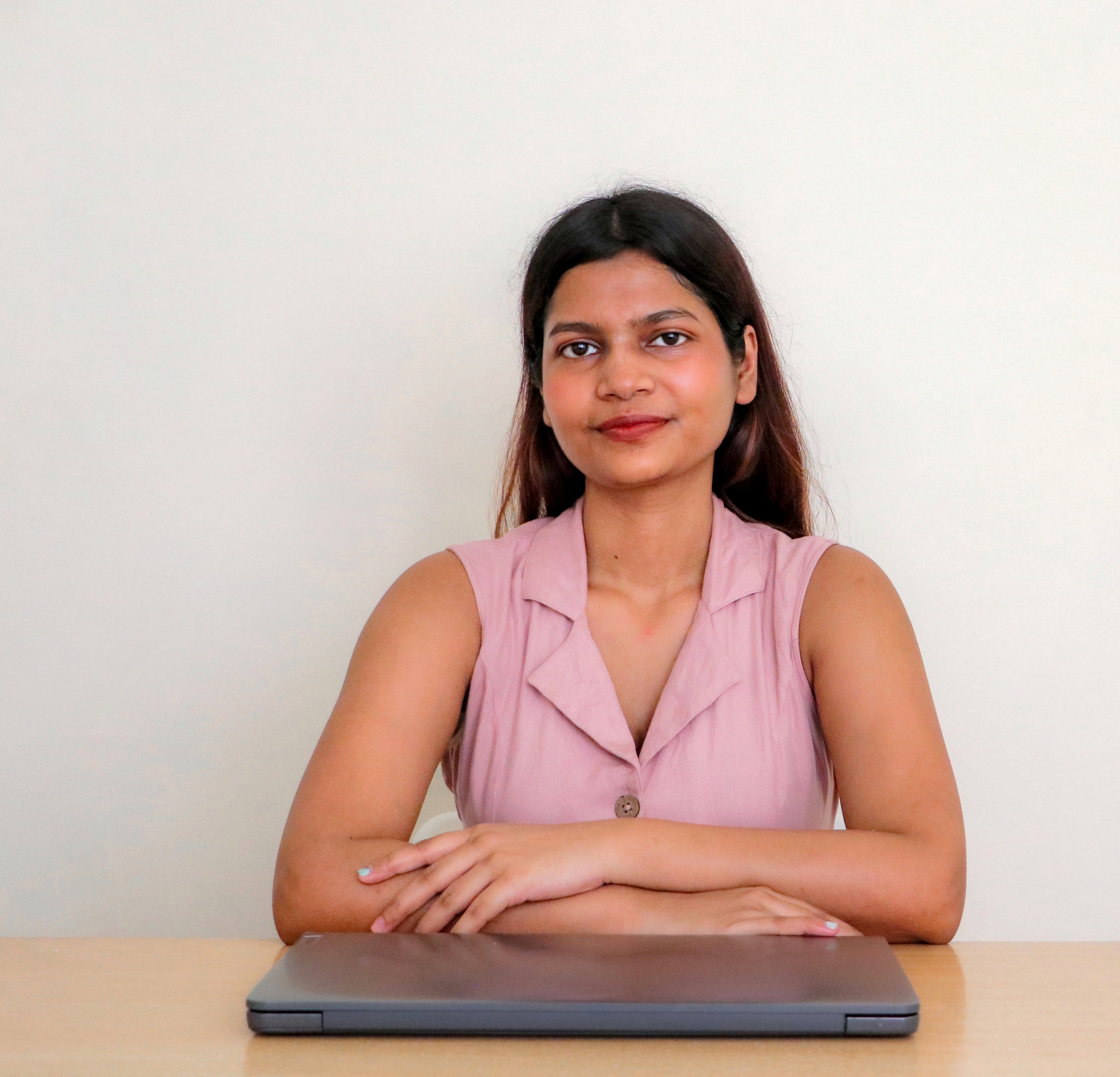
Table of contents
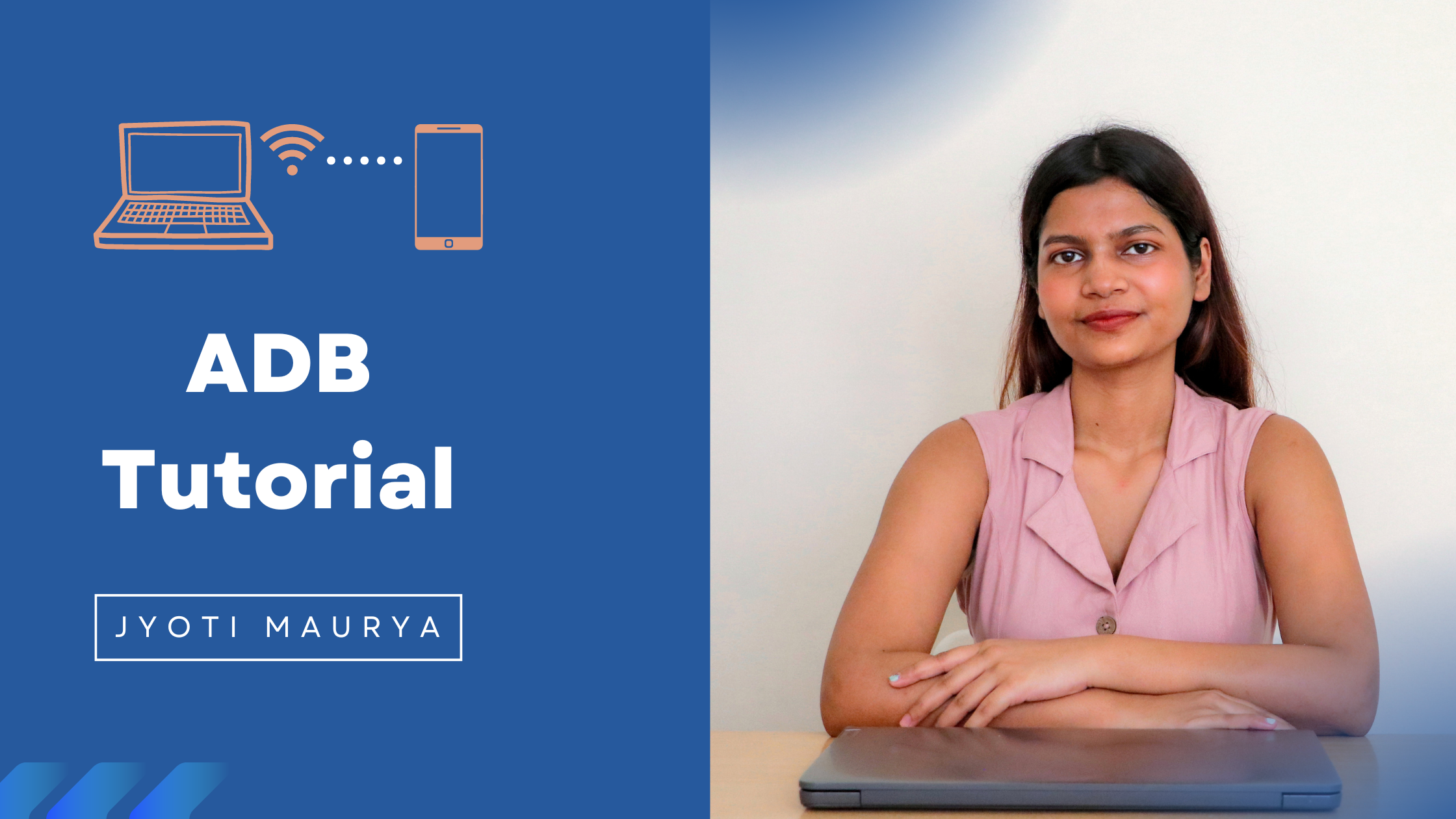
Introduction
Android Debug Bridge or ADB is a versatile command line tool that lets you communicate with your android device for debugging purposes. ADB provides access to a shell environment on your device, allowing you to run powerful commands directly on the Android system. ADB lets you transfer files between your computer and your device, similar to how you would with a USB drive.
With ADB you can install apps on your android mobile phone or tablet and run your app in debug as well as release mode.
Pre-requisites
To run ADB commands, you should have ADB on your laptop. ADB comes bundled with android studio. So, my advice is to correctly install android studio on your laptop which would automatically install ADB with it.
Before running the ADB commands make sure that you have attached the device i.e. your android testing phone or tablet to your laptop via a cable. Make sure you connect the laptop and attached device to the same Wi-Fi network. Also note down the IP address of the attached device by going to the settings > Wi-Fi, click on the arrow icon beside the Wi-Fi network you are connected to, you should see the IP address and other details.
Now head over to terminal in your VS code or any other IDE and start writing the ADB commands.
ADB Basic Commands
Start ADB Server
Start the ADB server on your laptop
adb start-server
View all Attached Devices
To view all attached devices
adb devices
To view the device with model details
adb devices -l
Run ADB in TCPIP Mode
To run adb in tcpip mode
adb tcpip 5555
Connect device over Wi-Fi
To connect device over Wi-Fi using Ip address (IPv4) of attached device (Phone/Tablet).
adb connect 192.168.1.6
See all the packages installed your attached device
adb shell cmd package list packages
Uninstall a package/app on your phone
Sometimes you would want to uninstall an app from your phone, and you can do this using the following command. yourAppName is available in your android app's Manifest.xml file.
adb uninstall com.yourAppName
There are more than one way to uninstall the app. Some of them are mentioned below
adb uninstall <app .apk name>
adb uninstall -k <app .apk name> # "Uninstall .apk withour deleting data"
adb shell pm uninstall com.example.MyApp
adb shell pm clear [package] # Deletes all data associated with a package.
Install a package on your phone
To install an app
adb -e install path/to/app.apk
Install app on all connected devices
adb devices | tail -n +2 | cut -sf 1 | xargs -IX adb -s X install -r com.myAppPackage
Update the app
Replace yourApp and filepath with the app name and filepath that you have. Also, -r means re-install the app and it keep its data on the device.
== Update app
adb install -r yourApp.apk
adb install –k filepath/yourApp2.apk
Stop the ADB server
adb kill-server
Reboot ADB
Following command is the most basic reboot command. It restarts your Android device into the normal mode you use every day. This is useful for situations like applying changes made through ADB or restarting after a system crash.
adb reboot
Another reboot command is the one that reboots your device directly into the recovery mode. Recovery mode is a separate partition on your device that allows you to perform tasks like: Installing custom ROMs (alternate operating systems), Wiping data (factory reset), Troubleshooting boot issues.
adb boot recovery
Reboots your device into the bootloader mode, also known as fastboot mode. Bootloader mode is used for advanced actions like: Flashing system images (updating firmware or installing custom software), unlocking the bootloader (on some devices, allowing more customization) and performing other low-level operations.
adb reboot-bootloader
Root Permission
adb root only assigns permissions. Rooting grants superuser access to the Android system, enabling advanced modifications but also carrying security risks.
adb root
Help
adb help
ADB Advanced Commands
You might not use them everyday but you might need them someday
Logcat
== LogCat
adb logcat
adb logcat -c // clear // The parameter -c will clear the current logs on the device.
adb logcat -d > [path_to_file] // Save the logcat output to a file on the local system.
adb bugreport > [path_to_file] // Will dump the whole device information like dumpstate, dumpsys and logcat output.
Files
== Files
adb push [source] [destination] // Copy files from your computer to your phone.
adb pull [device file location] [local file location] // Copy files from your phone to your computer.
Screenshot and Screen record
== Screenshot
adb shell screencap -p /sdcard/screenshot.png
$ adb shell
shell@ $ screencap /sdcard/screen.png
shell@ $ exit
$ adb pull /sdcard/screen.png
---
adb shell screenrecord /sdcard/NotAbleToLogin.mp4
$ adb shell
shell@ $ screenrecord --verbose /sdcard/demo.mp4
(press Control + C to stop)
shell@ $ exit
$ adb pull /sdcard/demo.mp4
Device Android Version
Get attached device android version using below command.
The following flags can be used
-d - directs command to the only connected USB device
-e - directs command to the only running emulator
-s
-p
adb shell <flags> getprop ro.build.version.release
Reset Permissions
// Reset permissions
adb shell pm reset-permissions -p your.app.package
adb shell pm grant [packageName] [ Permission] // Grant a permission to an app.
adb shell pm revoke [packageName] [ Permission] // Revoke a permission from an app.
Key Events
== Key event
adb shell input keyevent 3 // Home btn
adb shell input keyevent 4 // Back btn
adb shell input keyevent 5 // Call
adb shell input keyevent 6 // End call
adb shell input keyevent 26 // Turn Android device ON and OFF. It will toggle device to on/off status.
adb shell input keyevent 27 // Camera
adb shell input keyevent 64 // Open browser
adb shell input keyevent 66 // Enter
adb shell input keyevent 67 // Delete (backspace)
adb shell input keyevent 207 // Contacts
adb shell input keyevent 220 / 221 // Brightness down/up
adb shell input keyevent 277 / 278 /279 // Cut/Copy/Paste
Other Key events include
0 --> "KEYCODE_0"
1 --> "KEYCODE_SOFT_LEFT"
2 --> "KEYCODE_SOFT_RIGHT"
3 --> "KEYCODE_HOME"
4 --> "KEYCODE_BACK"
5 --> "KEYCODE_CALL"
6 --> "KEYCODE_ENDCALL"
7 --> "KEYCODE_0"
8 --> "KEYCODE_1"
9 --> "KEYCODE_2"
10 --> "KEYCODE_3"
11 --> "KEYCODE_4"
12 --> "KEYCODE_5"
13 --> "KEYCODE_6"
14 --> "KEYCODE_7"
15 --> "KEYCODE_8"
16 --> "KEYCODE_9"
17 --> "KEYCODE_STAR"
18 --> "KEYCODE_POUND"
19 --> "KEYCODE_DPAD_UP"
20 --> "KEYCODE_DPAD_DOWN"
21 --> "KEYCODE_DPAD_LEFT"
22 --> "KEYCODE_DPAD_RIGHT"
23 --> "KEYCODE_DPAD_CENTER"
24 --> "KEYCODE_VOLUME_UP"
25 --> "KEYCODE_VOLUME_DOWN"
26 --> "KEYCODE_POWER"
27 --> "KEYCODE_CAMERA"
28 --> "KEYCODE_CLEAR"
29 --> "KEYCODE_A"
30 --> "KEYCODE_B"
31 --> "KEYCODE_C"
32 --> "KEYCODE_D"
33 --> "KEYCODE_E"
34 --> "KEYCODE_F"
35 --> "KEYCODE_G"
36 --> "KEYCODE_H"
37 --> "KEYCODE_I"
38 --> "KEYCODE_J"
39 --> "KEYCODE_K"
40 --> "KEYCODE_L"
41 --> "KEYCODE_M"
42 --> "KEYCODE_N"
43 --> "KEYCODE_O"
44 --> "KEYCODE_P"
45 --> "KEYCODE_Q"
46 --> "KEYCODE_R"
47 --> "KEYCODE_S"
48 --> "KEYCODE_T"
49 --> "KEYCODE_U"
50 --> "KEYCODE_V"
51 --> "KEYCODE_W"
52 --> "KEYCODE_X"
53 --> "KEYCODE_Y"
54 --> "KEYCODE_Z"
55 --> "KEYCODE_COMMA"
56 --> "KEYCODE_PERIOD"
57 --> "KEYCODE_ALT_LEFT"
58 --> "KEYCODE_ALT_RIGHT"
59 --> "KEYCODE_SHIFT_LEFT"
60 --> "KEYCODE_SHIFT_RIGHT"
61 --> "KEYCODE_TAB"
62 --> "KEYCODE_SPACE"
63 --> "KEYCODE_SYM"
64 --> "KEYCODE_EXPLORER"
65 --> "KEYCODE_ENVELOPE"
66 --> "KEYCODE_ENTER"
67 --> "KEYCODE_DEL"
68 --> "KEYCODE_GRAVE"
69 --> "KEYCODE_MINUS"
70 --> "KEYCODE_EQUALS"
71 --> "KEYCODE_LEFT_BRACKET"
72 --> "KEYCODE_RIGHT_BRACKET"
73 --> "KEYCODE_BACKSLASH"
74 --> "KEYCODE_SEMICOLON"
75 --> "KEYCODE_APOSTROPHE"
76 --> "KEYCODE_SLASH"
77 --> "KEYCODE_AT"
78 --> "KEYCODE_NUM"
79 --> "KEYCODE_HEADSETHOOK"
80 --> "KEYCODE_FOCUS"
81 --> "KEYCODE_PLUS"
82 --> "KEYCODE_MENU"
83 --> "KEYCODE_NOTIFICATION"
84 --> "KEYCODE_SEARCH"
85 --> "KEYCODE_MEDIA_PLAY_PAUSE"
86 --> "KEYCODE_MEDIA_STOP"
87 --> "KEYCODE_MEDIA_NEXT"
88 --> "KEYCODE_MEDIA_PREVIOUS"
89 --> "KEYCODE_MEDIA_REWIND"
90 --> "KEYCODE_MEDIA_FAST_FORWARD"
91 --> "KEYCODE_MUTE"
92 --> "KEYCODE_PAGE_UP"
93 --> "KEYCODE_PAGE_DOWN"
94 --> "KEYCODE_PICTSYMBOLS"
Subscribe to my newsletter
Read articles from Jyoti Maurya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
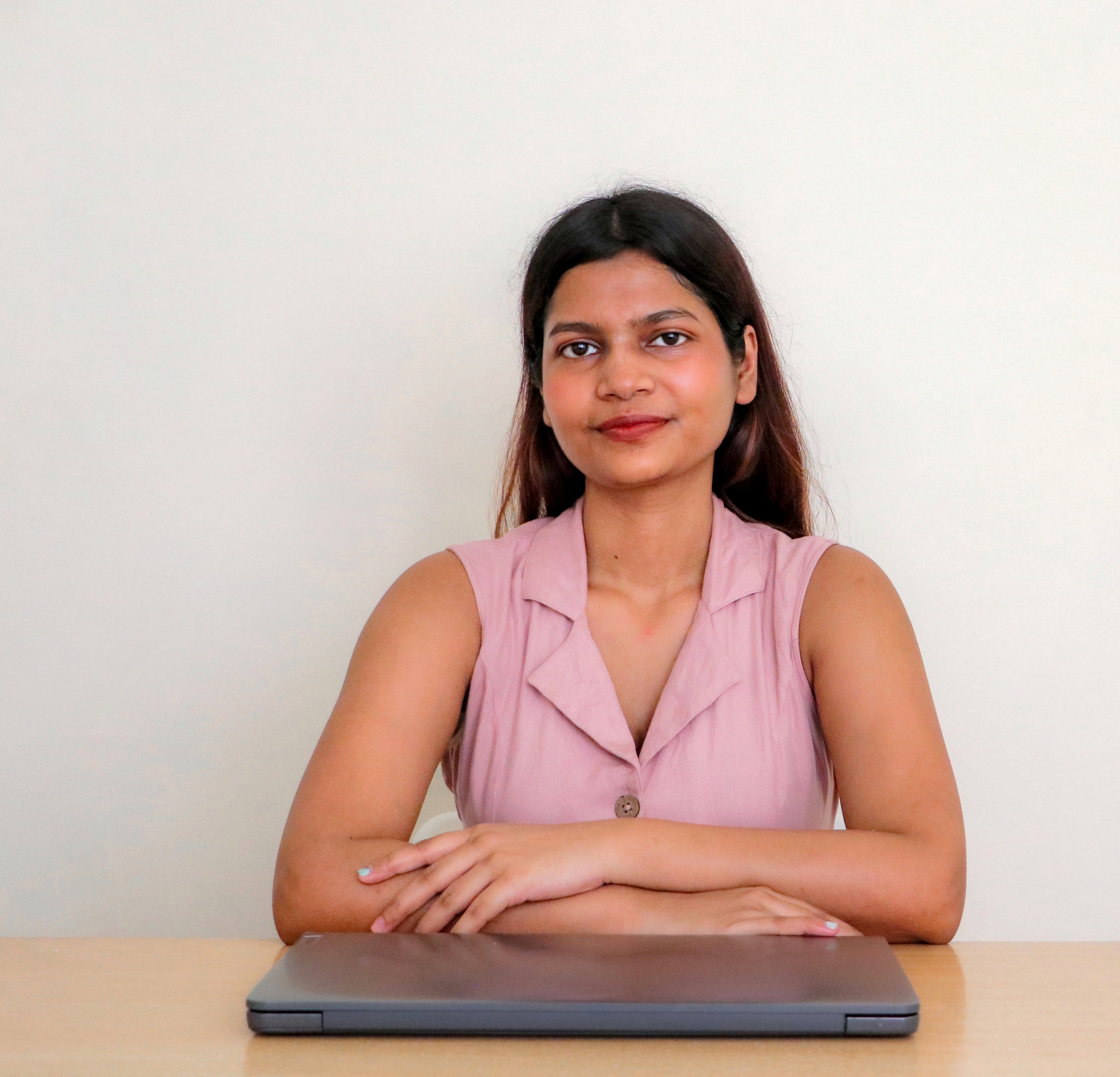
Jyoti Maurya
Jyoti Maurya
I create cross platform mobile apps with AI functionalities. Currently a PhD Scholar at Indira Gandhi Delhi Technical University for Women, Delhi. M.Tech in Artificial Intelligence (AI).