Demystifying Data Queries in .NET
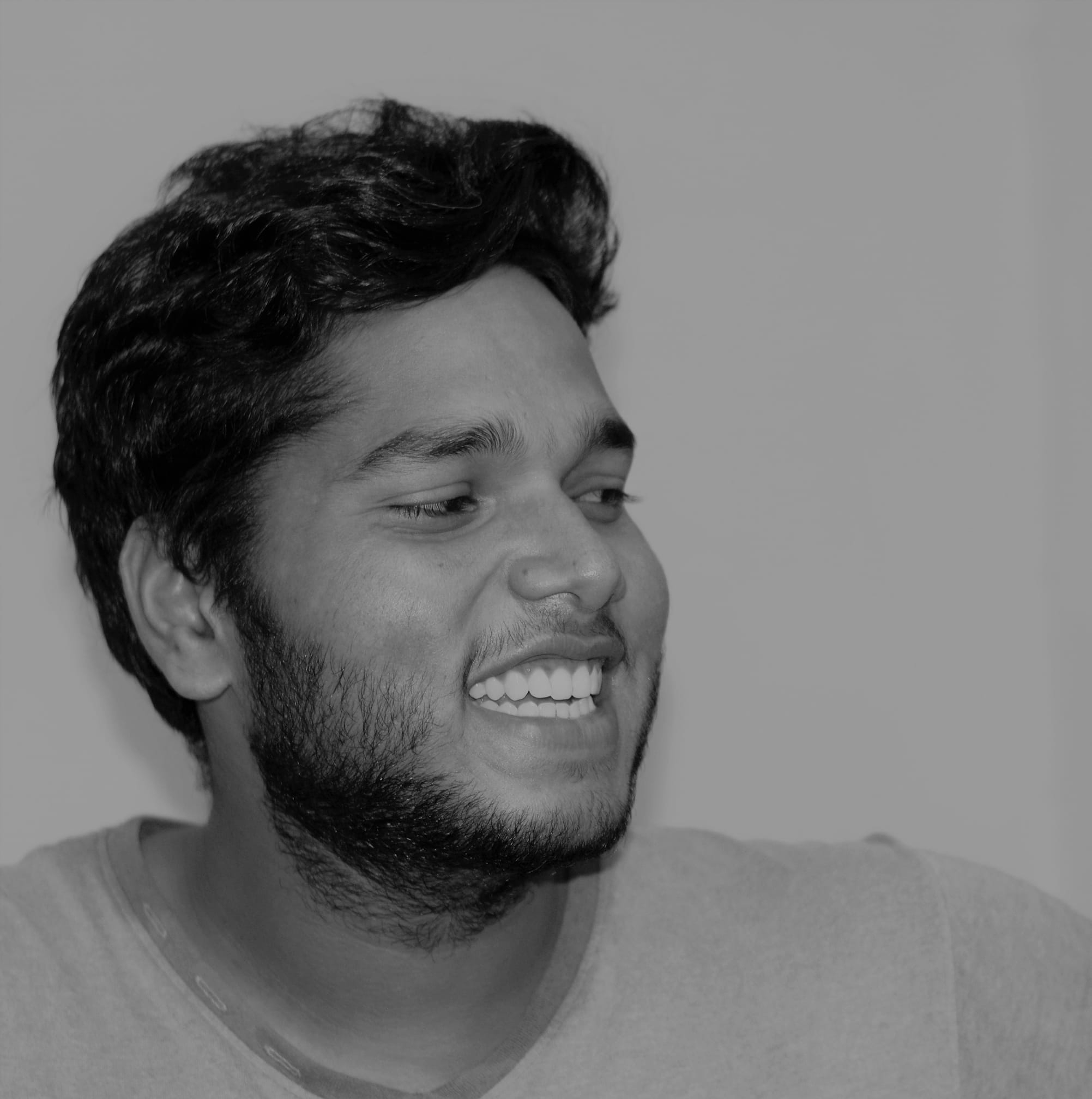
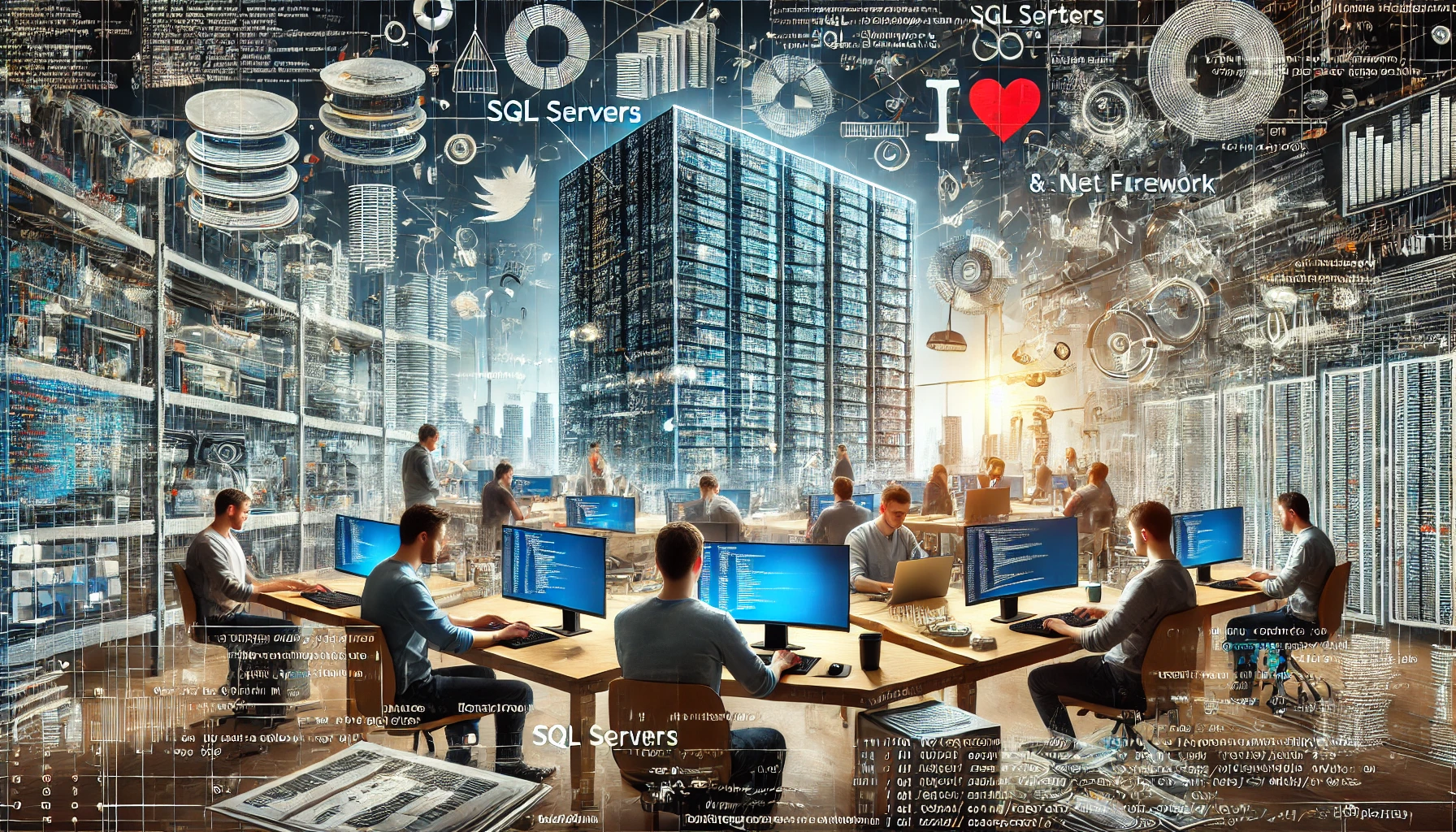
In the world of .NET development, querying data is a common task that developers handle on a daily basis. Two prominent ways to query data are using Link Query and Method Query. Despite their frequent usage, many developers often find themselves confused about the differences between these two approaches and when to use each one effectively.
Link Query and Method Query were introduced to make querying data more intuitive and powerful, allowing developers to write expressive and efficient code. This article aims to demystify these two querying techniques by explaining what they are, highlighting their differences, and providing guidance on when to use each.
By the end of this article, you will have a clear understanding of Link Query and Method Query, their respective use cases, and best practices to follow to ensure you are using the right approach for your .NET projects.
What is Link Query?
Link Query, short for Language Integrated Query, is a powerful feature introduced in .NET to simplify and streamline data querying. It enables developers to write queries directly within their programming language, making the code more readable and maintainable. Link Query is embedded within C# and Visual Basic, allowing developers to use query syntax that resembles SQL but is fully integrated with the language's type system.
Historical Context
Link Query was introduced with the release of .NET Framework 3.5 in 2007. It was designed to address the complexity of traditional data access methods and to provide a more declarative way to interact with data sources such as collections, databases, XML documents, and more.
Example of a Link Query in .NET
Here's a basic example of a Link Query in C#:
using System;
using System.Collections.Generic;
using System.Linq;
namespace LinkQueryExample
{
class Program
{
static void Main(string[] args)
{
List<int> numbers = new List<int> { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
// Link Query to select even numbers
var evenNumbers = from number in numbers
where number % 2 == 0
select number;
Console.WriteLine("Even Numbers:");
foreach (var number in evenNumbers)
{
Console.WriteLine(number);
}
}
}
}
In this example, the Link Query is used to select even numbers from a list. The syntax is straightforward and easy to read, making it clear what the query is doing at a glance.
Link Query provides a seamless way to query various data sources, leveraging the power of the .NET language and its features. Its declarative nature allows developers to express their intent more clearly and concisely compared to traditional methods.
What is Method Query?
Method Query, also known as method syntax or lambda syntax, is another powerful way to perform data queries in .NET. Unlike Link Query, which uses a declarative SQL-like syntax, Method Query utilizes method calls and lambda expressions to achieve the same results. This approach leverages the .NET framework’s rich set of extension methods provided by the System.Linq
namespace.
Historical Context
Method Query was introduced alongside Link Query with the .NET Framework 3.5 in 2007. It was designed to provide a more familiar syntax for developers who are comfortable with method chaining and lambda expressions. This method syntax is especially powerful for complex queries that require more advanced operations and transformations.
Example of a Method Query in .NET
Here’s a basic example of a Method Query in C# that mirrors the previous Link Query example:
using System;
using System.Collections.Generic;
using System.Linq;
namespace MethodQueryExample
{
class Program
{
static void Main(string[] args)
{
List<int> numbers = new List<int> { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
// Method Query to select even numbers
var evenNumbers = numbers.Where(number => number % 2 == 0);
Console.WriteLine("Even Numbers:");
foreach (var number in evenNumbers)
{
Console.WriteLine(number);
}
}
}
}
In this example, the Method Query achieves the same result as the Link Query example but uses method calls and a lambda expression. The Where
method is used to filter the numbers, and the lambda expression number => number % 2 == 0
defines the filtering logic.
Method Query syntax is often preferred by developers who like the fluent style of chaining method calls and using lambda expressions. It can also be more powerful and flexible for performing complex queries that involve multiple operations and transformations.
Differences Between Link Query and Method Query
While both Link Query and Method Query are powerful tools for querying data in .NET, they have distinct differences that can influence a developer’s choice depending on the context and specific requirements. Understanding these differences is crucial for selecting the right approach.
Syntax
Link Query uses a declarative syntax that resembles SQL, making it more readable for those familiar with SQL-like querying languages.
Example:
var evenNumbers = from number in numbers where number % 2 == 0 select number;
Method Query uses method chaining and lambda expressions, which can be more concise and flexible.
Example:
var evenNumbers = numbers.Where(number => number % 2 == 0);
Readability
Link Query is often considered more readable and intuitive, especially for those with a background in SQL. It clearly separates the different parts of the query (selection, filtering, ordering).
Method Query can become complex and harder to read with multiple chained methods and nested lambda expressions.
Flexibility
Link Query is powerful for straightforward queries but can be less flexible when it comes to more complex operations and transformations.
Method Query provides greater flexibility with its use of lambda expressions and method chaining, allowing for more advanced and intricate queries.
Performance
- Both Link Query and Method Query generally offer similar performance, as they are translated to the same underlying code by the compiler. However, the choice of one over the other can sometimes impact readability and maintainability rather than raw performance.
Use Cases
Link Query is ideal for simple, readable queries that involve basic filtering, ordering, and selection.
- Example: Fetching records from a collection where readability and simplicity are prioritized.
Method Query is better suited for more complex queries that require advanced transformations, multiple conditions, or dynamic query construction.
- Example: When performing complex data manipulations or aggregations that benefit from the flexibility of method chaining.
Comparison Table
Feature | Link Query | Method Query |
Syntax | SQL-like, declarative | Method chaining, lambda expressions |
Readability | More readable for straightforward queries | Can be less readable with complex chaining |
Flexibility | Less flexible for complex operations | More flexible for advanced queries |
Performance | Generally similar performance | Generally similar performance |
Use Cases | Simple, readable queries | Complex, flexible queries |
When to Use Link Query
Link Query is a powerful tool in .NET that excels in specific scenarios where its syntax and readability provide significant advantages. Understanding when to use Link Query can help developers write more maintainable and expressive code.
Ideal Scenarios for Using Link Query
Simple Data Retrieval
Link Query is perfect for straightforward data retrieval tasks. When you need to filter, sort, and select data in a simple and readable manner, Link Query's SQL-like syntax shines.
Example:
var highScores = from score in scores where score > 100 orderby score descending select score;
Readability and Maintainability
If the primary goal is to make the code easily readable and maintainable, Link Query’s declarative style helps by clearly defining the query structure. This is particularly useful for teams with members who are familiar with SQL.
Example:
var customersInCity = from customer in customers where customer.City == "New York" select customer;
Static Queries
Link Query is well-suited for static queries where the structure of the query does not change dynamically at runtime. It is ideal for scenarios where the query logic is straightforward and predefined.
Example:
var productsOnSale = from product in products where product.OnSale select product;
Advantages of Using Link Query
Ease of Understanding: Link Query’s syntax is intuitive and easy to understand, especially for developers with a background in SQL. This makes it simpler to read and reason about the code.
Structured and Clear: The structure of Link Query provides a clear separation of different parts of the query (such as filtering, ordering, and selection), enhancing code clarity.
Error Checking: Since Link Query integrates with the .NET language’s type system, it offers compile-time error checking, which can help catch mistakes early in the development process.
Example Use Case or Case Study
Imagine you are developing a reporting tool that extracts data from a database and presents it in a user-friendly format. The queries involved are relatively simple, such as filtering records based on specific criteria and ordering them.
Scenario: You need to generate a report of all active customers in a particular city, sorted by their last purchase date.
Link Query Example:
var activeCustomersInCity = from customer in customers
where customer.IsActive && customer.City == "San Francisco"
orderby customer.LastPurchaseDate descending
select new { customer.Name, customer.Email, customer.LastPurchaseDate };
In this scenario, Link Query’s syntax makes it easy to understand the logic at a glance, which is particularly beneficial for maintaining and updating the code in the future.
When to Use Method Query
Method Query, with its method chaining and lambda expressions, is a powerful alternative to Link Query in .NET. It excels in scenarios where more complex and dynamic querying is required. Understanding when to use Method Query can help developers leverage its flexibility and power to handle advanced data manipulation tasks.
Ideal Scenarios for Using Method Query
Complex Data Manipulations
Method Query is ideal for complex queries involving multiple operations, such as filtering, grouping, joining, and transforming data. Its method chaining capability allows for building intricate queries in a fluent and readable manner.
Example:
var groupedOrders = orders .Where(order => order.Total > 100) .GroupBy(order => order.CustomerId) .Select(group => new { CustomerId = group.Key, Orders = group.ToList() });
Dynamic Query Construction
When the structure of the query needs to be determined at runtime, Method Query provides the necessary flexibility. This is useful for scenarios where query parameters can change based on user inputs or other runtime conditions.
Example:
var query = products.AsQueryable(); if (includeOnSale) { query = query.Where(product => product.OnSale); } var result = query.ToList();
Advanced Transformations
Method Query is well-suited for performing advanced data transformations and aggregations. Its extensive set of LINQ extension methods allows for detailed and precise data manipulation.
Example:
var productStatistics = products .GroupBy(product => product.Category) .Select(group => new { Category = group.Key, TotalStock = group.Sum(product => product.Stock), AveragePrice = group.Average(product => product.Price) });
Advantages of Using Method Query
Flexibility: Method Query’s fluent syntax and method chaining provide great flexibility for constructing complex queries dynamically. This makes it ideal for applications with varied and evolving query requirements.
Lambda Expressions: The use of lambda expressions allows for concise and powerful expressions within queries. This can simplify the code and reduce the verbosity often associated with more complex querying logic.
Extensibility: Method Query can easily incorporate custom extension methods, enhancing the functionality and readability of queries.
Example Use Case or Case Study
Consider a scenario where you are building an e-commerce application that provides dynamic product filtering based on user-selected criteria. The application needs to handle various filters, such as category, price range, and availability, which can be combined in different ways.
Scenario: You need to retrieve a list of products that match user-selected filters, including category, price range, and whether the product is on sale.
Method Query Example:
public IEnumerable<Product> GetFilteredProducts(string category, decimal? minPrice, decimal? maxPrice, bool? onSale)
{
var query = products.AsQueryable();
if (!string.IsNullOrEmpty(category))
{
query = query.Where(product => product.Category == category);
}
if (minPrice.HasValue)
{
query = query.Where(product => product.Price >= minPrice.Value);
}
if (maxPrice.HasValue)
{
query = query.Where(product => product.Price <= maxPrice.Value);
}
if (onSale.HasValue)
{
query = query.Where(product => product.OnSale == onSale.Value);
}
return query.ToList();
}
In this example, Method Query's flexibility allows for the dynamic construction of the query based on user inputs. Each filter is conditionally applied, demonstrating how Method Query can adapt to complex and changing requirements.
Impact of Different ORMs on Link Query and Method Query
When working with Object-Relational Mappers (ORMs) in .NET, such as Entity Framework or LLBLGen Pro, developers might wonder if the choice of ORM affects how queries should be written. Both Link Query and Method Query can be used effectively with various ORMs, but there are some considerations to keep in mind.
Entity Framework
Support for Both Queries: Entity Framework (EF) fully supports both Link Query and Method Query. Developers can use either syntax to query the database.
Example:
Link Query:
var activeCustomers = from customer in context.Customers where customer.IsActive select customer;
Method Query:
var activeCustomers = context.Customers .Where(customer => customer.IsActive) .ToList();
Complex Queries: EF’s support for Method Query can be advantageous for building complex and dynamic queries, utilizing the full range of LINQ extension methods and lambda expressions.
LLBLGen Pro
Compatibility: LLBLGen Pro also supports both Link Query and Method Query. Developers can use the syntax they prefer, although some ORM-specific extensions might be more naturally expressed with Method Query.
Customization: LLBLGen Pro provides additional query capabilities and customization options, which can be integrated with both Link Query and Method Query.
General Considerations
Performance: The performance of queries written using Link Query or Method Query can depend on the ORM's optimization capabilities. Both queries are typically translated into SQL by the ORM, and the efficiency of this translation can vary.
ORM-Specific Features: Some ORMs may offer specific features or optimizations that are easier to utilize with Method Query due to its flexible nature and support for complex expressions.
Readability and Maintainability: Regardless of the ORM, Link Query often provides better readability for simple queries, while Method Query offers greater flexibility for complex scenarios. The choice should balance readability, maintainability, and the specific features of the ORM in use.
Key Points
Both Link Query and Method Query are supported by popular ORMs like Entity Framework and LLBLGen Pro.
Method Query can be more flexible and is often preferred for complex or dynamic queries.
Link Query tends to be more readable and is suitable for straightforward queries.
The performance and capabilities of the queries can depend on how well the ORM translates them into SQL.
Consider the specific features and optimizations offered by the ORM when choosing between Link Query and Method Query.
Best Practices
To effectively use Link Query and Method Query in .NET, it’s essential to follow best practices that ensure your code is readable, maintainable, and performant. Here are some guidelines to help you get the most out of these querying techniques.
General Best Practices
Consistency
- Choose a querying style (Link Query or Method Query) and use it consistently within your project or module to maintain readability and coherence.
Readability
Prioritize readability, especially for simpler queries. Link Query often provides a more readable syntax for straightforward queries, while Method Query can handle more complex scenarios.
Example:
// Link Query for readability var activeUsers = from user in users where user.IsActive select user;
Performance Considerations
Be mindful of the performance implications of your queries. Test and profile different query structures to ensure they perform efficiently with your data set and ORM.
Avoid using complex operations that can be offloaded to the database.
Exception Handling
Implement proper exception handling to manage runtime errors effectively, particularly when dealing with database operations.
Example:
try { var results = context.Customers.Where(c => c.IsActive).ToList(); } catch (Exception ex) { // Handle exceptions appropriately Console.WriteLine(ex.Message); }
Best Practices for Link Query
Simplicity
Use Link Query for simple and straightforward data retrieval tasks where readability is a priority.
Example:
var recentOrders = from order in orders where order.OrderDate > DateTime.Now.AddDays(-30) select order;
Avoid Nested Queries
Avoid using deeply nested Link Queries as they can become hard to read and maintain. Consider breaking down complex queries into multiple simpler ones.
Example:
var recentOrders = from order in orders where order.OrderDate > DateTime.Now.AddDays(-30) select order; var highValueOrders = from order in recentOrders where order.TotalAmount > 1000 select order;
Best Practices for Method Query
Complex Queries
Leverage Method Query for complex queries involving multiple conditions, transformations, or dynamic query construction.
Example:
var expensiveProducts = products .Where(p => p.Price > 100) .OrderByDescending(p => p.Price) .Select(p => new { p.Name, p.Price }) .ToList();
Fluent Style
Utilize the fluent style of Method Query for better readability, especially when chaining multiple methods.
Example:
var topProducts = products .Where(p => p.Rating >= 4) .OrderByDescending(p => p.Sales) .Take(10) .ToList();
Dynamic Queries
Use Method Query for constructing queries dynamically based on runtime conditions, leveraging its flexibility.
Example:
var query = context.Orders.AsQueryable(); if (includeShipped) { query = query.Where(order => order.Status == "Shipped"); } var result = query.ToList();
Common Pitfalls to Avoid
Overusing Either Query Type
- Avoid overusing either Link Query or Method Query exclusively. Choose the best tool for the task based on the query’s complexity and readability needs.
Ignoring Performance
- Don’t ignore performance implications. Test and optimize queries, especially those that will run frequently or against large data sets.
Neglecting Readability
- Don’t sacrifice readability for brevity. Ensure that your queries are understandable and maintainable by others.
By following these best practices, you can write efficient, readable, and maintainable queries using both Link Query and Method Query in your .NET projects.
Conclusion
Understanding the differences between Link Query and Method Query is crucial for .NET developers who aim to write efficient, readable, and maintainable code. Both querying methods offer powerful ways to interact with data, but each has its strengths and ideal use cases.
Link Query, with its SQL-like syntax, is perfect for straightforward and static queries, providing clarity and ease of understanding. It is especially useful when readability is a priority, making it easier for teams to maintain and understand the code.
Method Query, on the other hand, shines in scenarios requiring complex and dynamic queries. Its method chaining and lambda expressions offer greater flexibility and power, allowing developers to construct intricate queries that can adapt to varying conditions.
When working with different ORMs like Entity Framework or LLBLGen Pro, both Link Query and Method Query can be used effectively. The choice between the two often comes down to the specific needs of the project, such as the complexity of the queries and the importance of readability versus flexibility.
By following best practices, such as prioritizing readability, handling exceptions appropriately, and considering performance implications, developers can make the most of both querying techniques. Whether using Link Query for its simplicity or Method Query for its advanced capabilities, understanding when and how to use each will enhance the quality and maintainability of your .NET applications.
Subscribe to my newsletter
Read articles from Arpit Dwivedi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
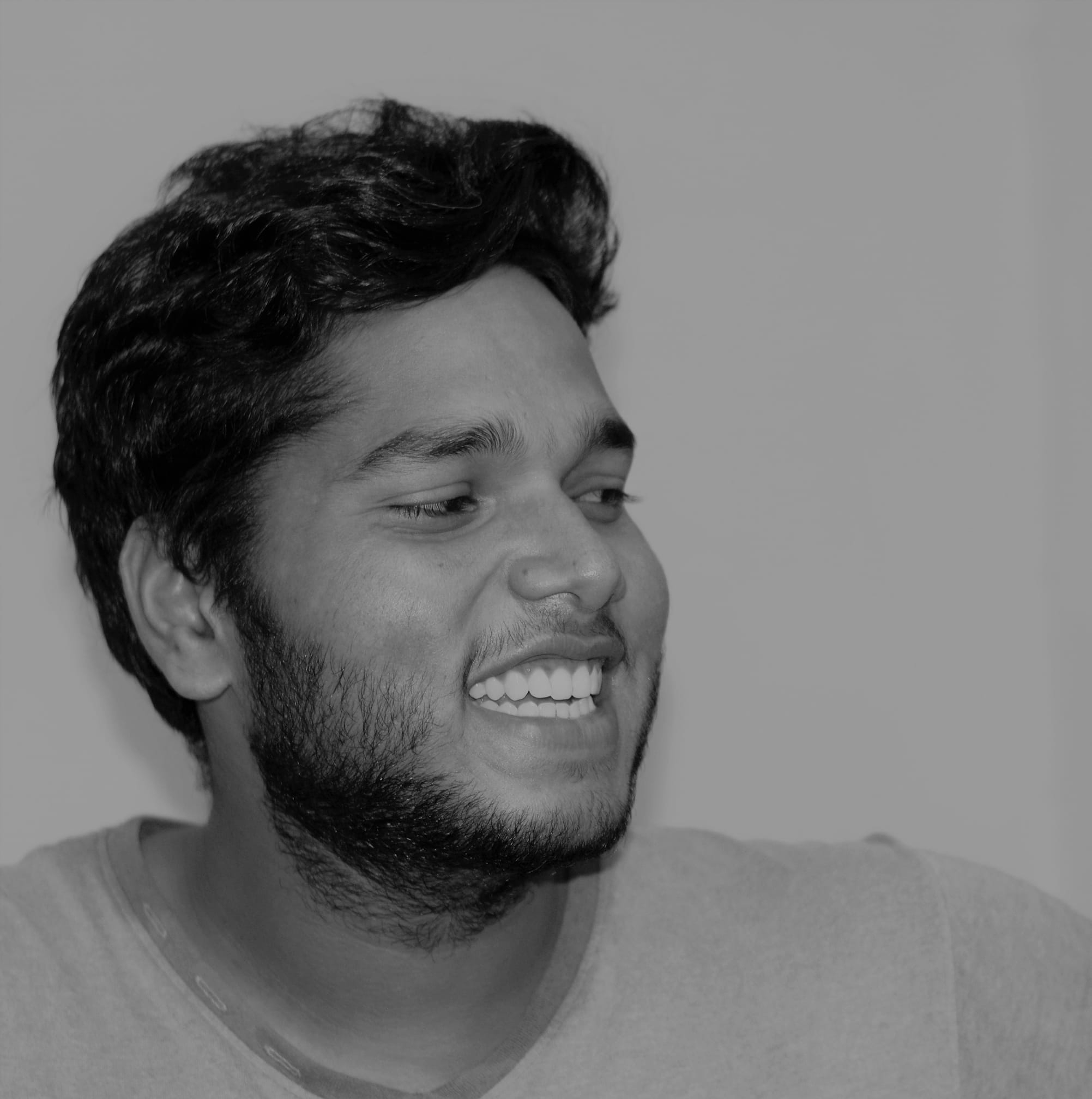
Arpit Dwivedi
Arpit Dwivedi
Crafting code with a finesse that bridges the digital divide! As a Full Stack Developer, I’ve conjured innovations at industry front-runners like Infosys and Kline & Company. With a suite boasting C#, ASP.NET CORE, Angular, and the complex dance of microservices, I’m not just programming – I’m telling stories. Beyond raw code, I'm a tech translator, dishing out insights as an avid writer, leading the charge in the open-source world, and floating on the Azure cloud. From corporates to intense tech programs, my journey’s been anything but ordinary. Curiosity? It's not just a sidekick; it's my co-pilot. Eagerly scanning the horizon for the next tech marvel. 🚀