✍️Day 24 Guide: Key Docker Interview Questions to Practice
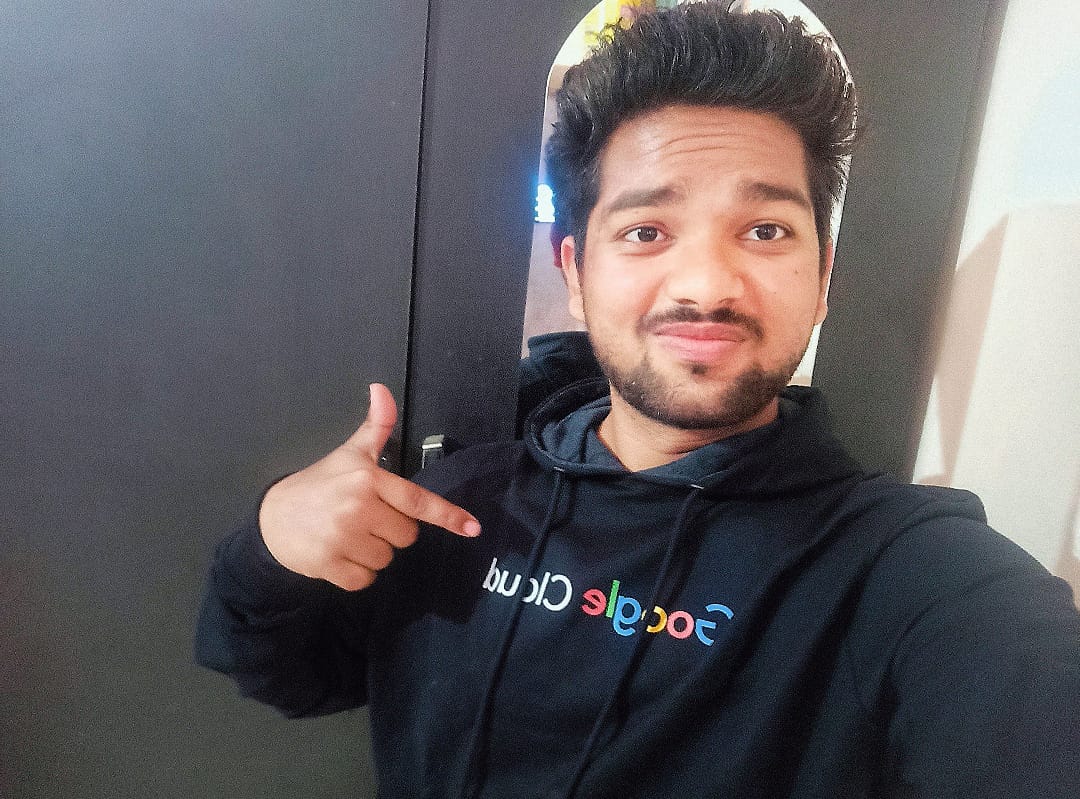
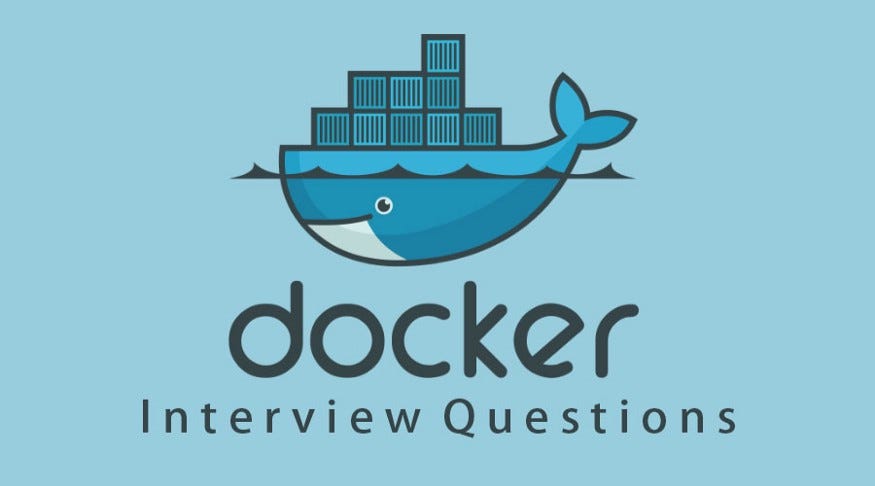
Today, I focused on practicing essential Docker interview questions that are likely to come up in interviews for DevOps roles. Mastering these questions will not only help in acing interviews but also deepen your understanding of Docker's core concepts and practical applications.
In this guide, I’ve compiled a list of key Docker interview questions along with their explanations. Reviewing and practicing these questions will prepare you for discussions about Docker images, containers, commands, and best practices for optimizing Docker images. Whether you’re preparing for an upcoming interview or looking to enhance your Docker skills, these questions are a great resource to test your knowledge.
1. What is the Difference between an Image, Container, and Engine?
Image: 🖼️ An image is a lightweight, standalone, and executable software package that includes everything needed to run a piece of software, including the code, runtime, libraries, environment variables, and configuration files. Images are read-only templates used to create containers and can be shared via Docker registries.
Container: 📦 A container is a runtime instance of an image. It is a lightweight, isolated, and portable environment that encapsulates an application and its dependencies. Containers run on a host operating system and share the host's kernel but are isolated from each other through namespaces and control groups (cgroups). Containers ensure that applications run consistently across different environments.
Engine: 🛠️ The Docker Engine is a client-server application that builds and runs Docker containers. It consists of three main components:
Docker Daemon (dockerd): A background service that manages Docker containers, images, networks, and storage volumes.
REST API: Interfaces with the daemon to instruct it what to do.
CLI (Command Line Interface): A user interface to interact with the Docker daemon using commands like
docker run
,docker build
, anddocker pull
.
2. What is the Difference between the Docker command COPY vs ADD?
COPY: 📂 COPY is used to copy files and directories from the host machine into the Docker image. It only copies local files and directories within the context of the build.
COPY <src> <dest>
: Path to the file or directory on the host.
: Path inside the image where the files or directories will be copied.
ADD: ➕ ADD is similar to COPY but with additional functionalities. It can:
Copy local files and directories.
Download files from remote URLs.
Extract TAR archives automatically.
ADD <src> <dest>
Use ADD when you need its extra functionalities (e.g., downloading a file from a URL), and use COPY for simple, straightforward file copying tasks.
3. What is the Difference between the Docker command CMD vs RUN?
CMD: 🏁 CMD specifies the default command to run when a container is started. It can be overridden by providing arguments at runtime. CMD should be used to set the main command for an image. Only the last CMD instruction in the Dockerfile is effective.
CMD ["executable","param1","param2"]
RUN: 🏃♂️ RUN executes commands during the build process to modify the Docker image. These commands are used to install dependencies, set up the environment, and configure the container. The effects of RUN commands are committed to the image as a new layer.
RUN <command>
4. How will you reduce the size of the Docker image?
- Use Multi-Stage Builds: Utilize multi-stage builds to minimize the number of layers and reduce the final image size. This allows you to copy only the necessary artifacts to the final stage.
FROM golang:alpine AS builder
WORKDIR /app
COPY . .
RUN go build -o main .
FROM alpine
WORKDIR /app
COPY --from=builder /app/main .
CMD ["./main"]
- Remove Unnecessary Files and Dependencies: Clean up temporary files and unnecessary dependencies after installation.
RUN apt-get update && apt-get install -y \
build-essential \
&& rm -rf /var/lib/apt/lists/*
- Utilize Smaller Base Images: Use smaller base images like Alpine Linux to reduce the overall image size.
FROM alpine
Optimize and Compress Files: Compress and optimize files and assets before adding them to the image.
Minimize the Number of Installed Packages: Install only the necessary packages and dependencies.
Clean Up After Each Build Step: Remove temporary files and caches created during the build process.
RUN apt-get update && apt-get install -y \
build-essential \
&& apt-get clean \
&& rm -rf /var/lib/apt/lists/*
- Use .dockerignore File: Exclude unnecessary files and directories from being copied into the image by using a
.dockerignore
file.
# .dockerignore
node_modules
dist
5. Why and when to use Docker?
Consistency Across Environments: Docker ensures that applications run the same way in different environments by encapsulating them and their dependencies in containers.
Isolation and Resource Management: Containers provide isolated environments for applications, improving security and resource management.
Microservices Architecture: Docker is ideal for adopting a microservices architecture, allowing each service to run in its own container.
Scalability: Docker facilitates the horizontal scaling of applications by running multiple instances of containers.
CI/CD Integration: Docker is used in continuous integration and continuous deployment (CI/CD) pipelines to automate the build, test, and deployment processes.
Simplified Dependency Management: Docker eliminates the "it works on my machine" problem by bundling the application with all its dependencies.
6. Explain the Docker components and how they interact with each other.
Docker Compose: 📜 A tool for defining and running multi-container Docker applications. It uses a YAML file (docker-compose.yml) to configure the application's services, networks, and volumes.
version: '3'
services:
web:
image: nginx
ports:
- "80:80"
db:
image: mysql
environment:
MYSQL_ROOT_PASSWORD: example
Dockerfile: 📝 A text file that contains instructions for building a Docker image. It specifies the base image, dependencies, environment variables, and commands to run during the build process.
FROM node:14
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 3000
CMD ["node", "server.js"]
Docker Image: 🖼️ A lightweight, standalone, and executable software package that includes everything needed to run a piece of software. It is used to create Docker containers.
Docker Container: 📦 A runtime instance of a Docker image. It is a lightweight, isolated, and portable environment that encapsulates an application and its dependencies.
7. Explain the terminology: Docker Compose, Docker File, Docker Image, Docker Container.
Docker Compose: 📜 A tool for defining and running multi-container Docker applications. It uses a YAML file (docker-compose.yml) to configure the application's services, networks, and volumes.
Dockerfile: 📝 A text file that contains instructions for building a Docker image. It specifies the base image, dependencies, environment variables, and commands to run during the build process.
Docker Image: 🖼️ A lightweight, standalone, and executable software package that includes everything needed to run a piece of software. It is used to create Docker containers.
Docker Container: 📦 A runtime instance of a Docker image. It is a lightweight, isolated, and portable environment that encapsulates an application and its dependencies.
8. In what real scenarios have you used Docker?
Microservices Architecture: Containerizing microservices-based applications for scalability and easier deployment.
Development Environment: Simplifying development workflows by providing consistent environments across development, testing, and production.
CI/CD Pipelines: Implementing CI/CD pipelines to automate the build, test, and deployment processes.
Legacy Applications: Running legacy applications in isolated containers for compatibility and security purposes.
Testing and Debugging: Creating disposable and reproducible environments for testing and debugging.
9. Docker vs Hypervisor?
Docker Containers: Docker containers share the host operating system's kernel, making them lightweight and efficient compared to traditional virtualization technologies like hypervisors.
Hypervisors: Hypervisors create and manage virtual machines (VMs), which emulate physical hardware and run guest operating systems on top of the host operating system.
Performance: Docker containers provide faster startup times, better resource utilization, and higher density compared to VMs.
Isolation: Hypervisors offer stronger isolation between virtual machines but incur higher overhead due to the duplication of the operating system.
10. What are the advantages and disadvantages of using Docker?
Advantages: ✅
Lightweight: Containers are more lightweight compared to virtual machines.
Consistent Environments: Ensures consistency across different platforms and environments.
Scalability: Facilitates scaling applications horizontally.
Deployment Speed: Faster deployment and version control.
Simplified Management: Simplified management and orchestration of applications.
Disadvantages: ❌
Security: Security concerns due to shared kernel.
Learning Curve: Steep learning curve for newcomers.
Networking and Storage: Complexity in managing networking and storage.
Legacy Support: Limited support for legacy applications.
Performance Overhead: Potential performance overhead compared to bare-metal deployments.
11. What is a Docker namespace?
Docker namespace is a hierarchical naming system used to uniquely identify Docker objects such as images, containers, volumes, networks
, and plugins. Namespaces prevent naming conflicts and provide a way to organize and manage Docker resources. Docker uses different namespaces for different types of objects, such as image namespace, container namespace, and network namespace.
12. What is a Docker registry?
A Docker registry is a centralized repository for storing and distributing Docker images. It allows users to push and pull images to and from the registry, enabling collaboration and sharing of Docker images across different environments. Docker Hub is the default public registry provided by Docker, but users can also set up private registries for internal use.
13. What is an entry point?
The entry point is a command or script specified in a Dockerfile that will be executed when a container starts. It defines the default executable for the container and can be overridden by providing arguments at runtime. The entry point is typically used to set up the container environment and start the main application process.
ENTRYPOINT ["executable", "param1", "param2"]
14. How to implement CI/CD in Docker?
Containerize the Application: Use Docker containers to package the application and its dependencies.
CI/CD Tools: Set up CI/CD pipelines with tools like Jenkins, GitLab CI/CD, or GitHub Actions.
Automate Processes: Automate the build, test, and deployment processes using Docker images and Docker Compose.
Docker Registries: Use Docker registries to store and distribute the built images.
Orchestration Tools: Integrate with orchestration tools like Kubernetes or Docker Swarm for automated deployment and scaling.
15. Will data on the container be lost when the docker container exits?
By default, data within a Docker container is ephemeral, meaning it will be lost when the container exits. To persist data between container restarts or exits, you can use Docker volumes or bind mounts to map host directories to container directories. Docker volumes provide a way to manage persistent data storage independently of the container's lifecycle.
16. What is a Docker swarm?
Docker Swarm is a clustering and orchestration tool for managing a cluster of Docker hosts and running containerized applications at scale. It provides features for service discovery, load balancing, and fault tolerance. Swarm mode allows you to create and manage a swarm of Docker nodes as a single virtual system.
17. What are the Docker commands for the following:
a) View running containers:
docker ps
b) Command to run the container under a specific name:
docker run --name <container_name> <image_name>
c) Command to export a Docker container:
docker export <container_name_or_id> > <file_name>.tar
d) Command to import an already existing Docker image:
docker import <file_name>.tar <image_name>
e) Command to delete a container:
docker rm <container_name_or_id>
f) Command to remove all stopped containers, unused networks, build caches, and dangling images:
docker system prune -a
18. What are the common Docker practices to reduce the size of Docker Image?
Start with Smaller Base Images: Use minimal base images like Alpine Linux.
Reduce the Number of Layers: Combine multiple commands into a single
RUN
statement.Eliminate Unnecessary Dependencies: Install only the necessary packages.
Optimize Dockerfile Instructions: Order instructions to leverage Docker's caching mechanism.
FROM node:14
WORKDIR /app
COPY package*.json ./
RUN npm install && npm cache clean --force
COPY . .
EXPOSE 3000
CMD ["node", "server.js"]
By practicing these Docker interview questions, you’ll be well-prepared to handle Docker-related discussions in interviews. Mastering these questions will not only boost your interview confidence but also strengthen your practical understanding of Docker.
Keep reviewing and practicing these questions to ensure you're ready for any Docker-related challenges that come your way. Good luck with your interview preparations, and stay tuned for more learning insights in the upcoming days! 🚀
Happy Learning! 😊
Subscribe to my newsletter
Read articles from Ritesh Dolare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
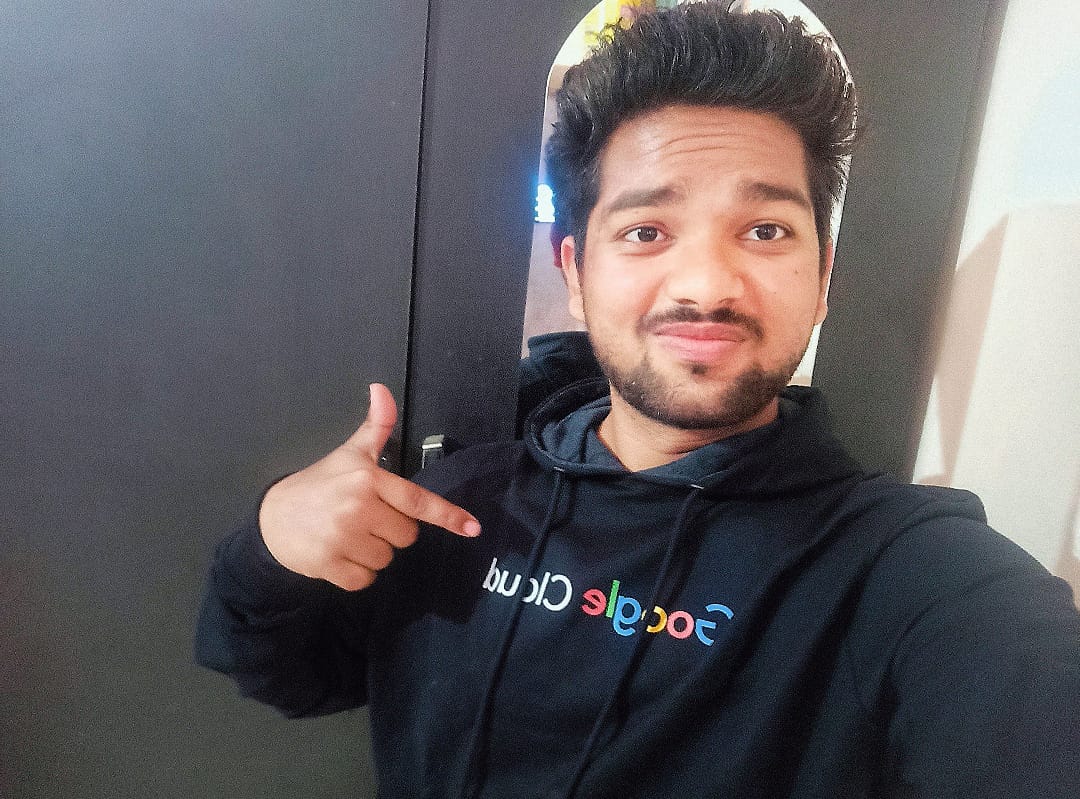
Ritesh Dolare
Ritesh Dolare
👋 Hi, I'm Ritesh Dolare, a DevOps enthusiast dedicated to mastering the art of DevOps.