Javascript Objects and ways to create them
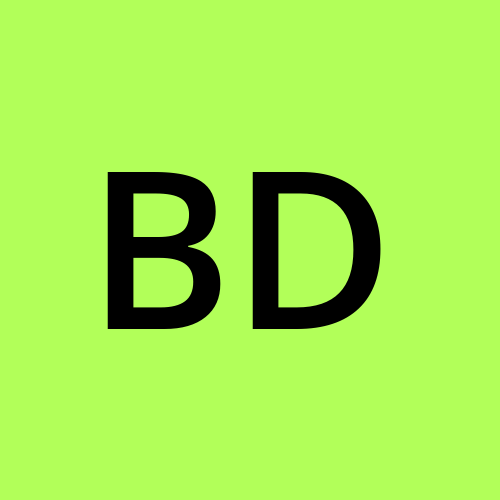
Table of contents
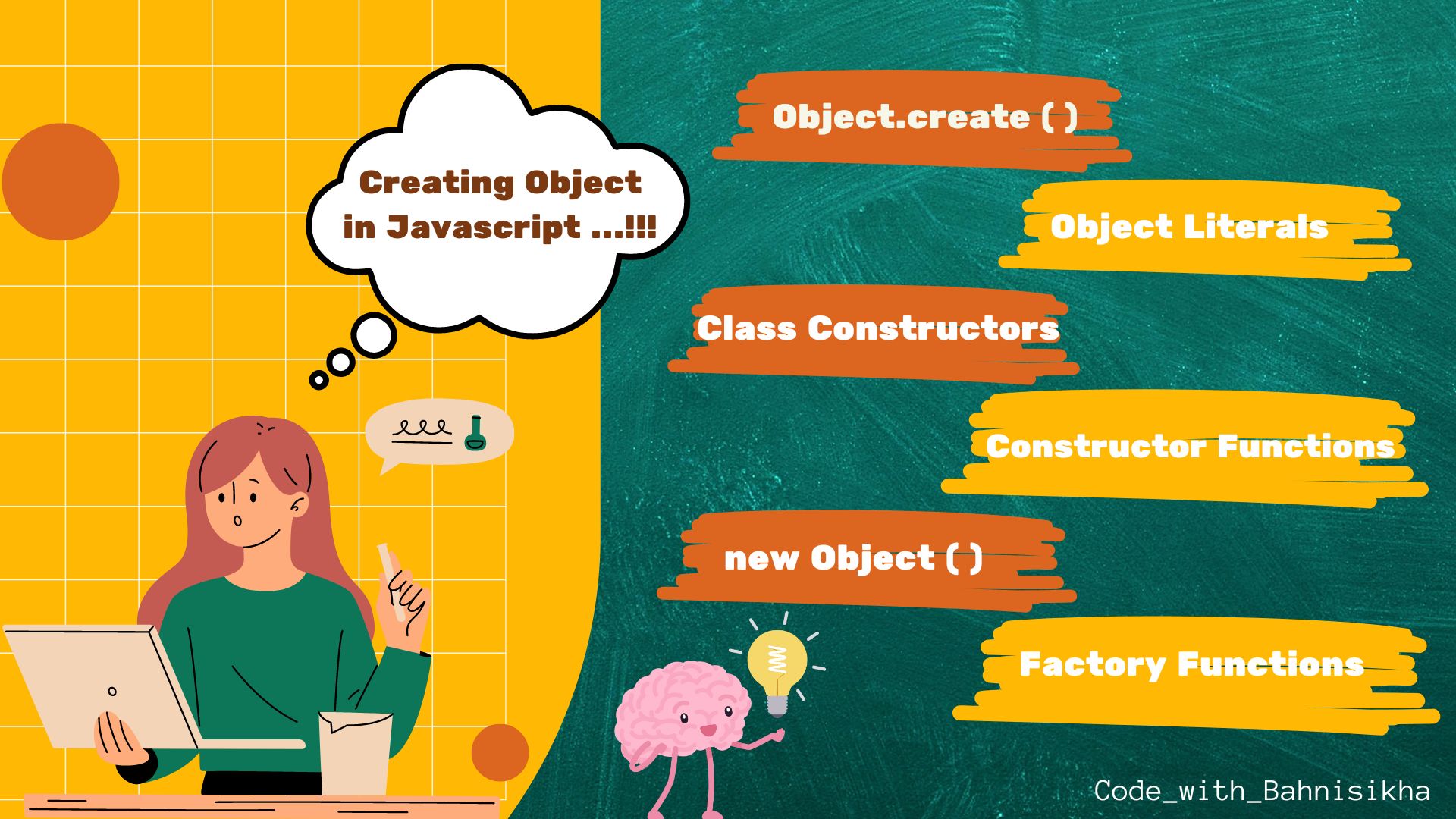
Object is a very well-known and most important data type in Javascript. Before we dive deep into creating objects in different ways, let's revise objects a bit.
What is a Javascript Object?
A Javascript object is a non-primitive
data type. We also know that the Object is of reference type
. That means, when we assign a value to an object, the value is stored in a memory location having a specific unique memory address. The object doesn't store the actual value, rather, it stores the reference of the memory address where the value is stored. An object consists of key-value pairs
where the key is of string type and the value can be of any type, it can be an object or an array or a function as well along with other primitive data types. A function as a value is known as a method
.
Now that we have revised a bit about Objects, let's start with different ways of creating them.
Ways to Create Javascript Objects
Creating Objects with Object Literals
The most common and straightforward way to create an object in JavaScript is using object literals
. This is defined as a pair of curly braces {}
and contains key-value pairs
const person = {
name: 'Bahnisikha',
year: 2024,
greet: function() {
console.log('Hello, ' + this.name + ', you are in ' + this.year);
}
};
person.greet(); // Hello, Bahnisikha, you are in 2024
console.log(person); // { name: 'Bahnisikha', year: 2024, greet: [Function: greet] }
Creating Objects with Object.create() method
The Object.create()
method creates a new object. This new object will have another object as its prototype. You can think of this prototype as a blueprint of the new object from which it will inherit properties and methods.
Note:
The newly created object itself doesn't have its own properties initially.
It only has access to the properties and methods through its prototype chain, that is, it only has inherited properties.
Here in the below code snippet we can see, that the newly created object (newPerson
) gets access to the properties and methods of the person
object but we get an empty object {}
if it is consoled (as it doesn't have its own properties or methods).
If we further add new properties or methods, that will be shown when the newPerson
object is consoled.
const person = {
name: 'Bahnisikha',
year: 2024,
greet: function() {
console.log('Hello, ' + this.name + ', you are in ' + this.year);
}
};
const newPerson = Object.create(person);
newPerson.greet(); // Hello, Bahnisikha, you are in 2024
newPerson.name; // Bahnisikha
console.log(newPerson); // {}
newPerson.place = "kolkata"; // new property added in newPerson object
console.log(newPerson); // { place: 'kolkata' }
Creating Objects with new Object()
Creating objects using new Object
syntax is less used, it's equivalent to using object literals but can be useful in certain situations. Have a look at the below code snippet...
const person = new Object();
person.name = "Bahnisikha";
person.year = 2024;
person.greet = function() {
console.log('Hello, ' + this.name + ', you are in ' + this.year);
}
person.greet(); //Hello, Bahnisikha, you are in 2024
console.log(person); // { name: 'Bahnisikha', year: 2024, greet: [Function (anonymous)] }
Creating Objects with Constructor Functions
Objects can be created using constructor functions. Constructor functions
are like normal functions and to differentiate them from normal functions, the convention which is followed is to give the name of the constructor function starting with a capital letter
.
In the below code snippet, when we call the
Person
constructor function with thenew
keyword, it creates anew object
(creates an instance of thePerson
)Sets the new object's prototype to
Person.prototype
(Prototype is something by which JavaScript objects inherit features from each other)Binds
this
inside the constructor to the new object and initializes the propertiesname
,species
and the methodgreet
on the new object.Note
: Arrow functions cannot be used as constructor functions as they do not have their ownthis
context (they inheritthis
from the parent scope at the time they are defined) and they also do not support thenew
keyword for creating new instances. On the other hand, a regular function works as a constructor function asfunction
keyword allowsthis
to refer to the newly created instance when invoked withnew
keyword.Executes the constructor function with the provided arguments
Returns the new object.
Note
: Defining the greet method within the constructor function will cause each instance to have its own copy of thegreet
method leading to increased memory usage.
function Person (name, year) {
this.name = name;
this.year = year;
this.greet = function() {
console.log('Hello, ' + this.name + ', you are in ' + this.year);
};
}
const newPerson = new Person("Bahnisikha", 2024);
console.log(newPerson); // Person {name: 'Bahnisikha', year: 2024, greet: [Function (anonymous)]}
newPerson.greet(); // Hello, Bahnisikha, you are in 2024
Note
: When we log a newly created object, the console shows the object's own properties, but it doesn't show the properties and methods inherited from its prototype chain directly.
So, in the below code snippet, as the name
and year
properties are own properties of the newPerson
object, and the greet
method is the property of the Person
prototype, so, console.log(newPerson) won't show greet in it. This is a good way to define methods as they are shared among all instances and not duplicated for each instance, making it more memory efficient.
function Person (name, year) {
this.name = name;
this.year = year;
}
Person.prototype.greet = function() {
console.log('Hello, ' + this.name + ', you are in ' + this.year);
};
const newPerson = new Person("Bahnisikha", 2024);
newPerson.greet(); // Hello, Bahnisikha, you are in 2024
console.log(newPerson); // Person { name: 'Bahnisikha', year: 2024 }
Creating Objects with Class Constructors
Another way of creating objects is using Class Constructors. These are very similar to function constructors.
In the below snippet, it can be seen that properties
are defined inside the constructor but the method
is defined outside of it because if it were defined within the constructor then each instance of it would get its own copy of the method, leading to increased memory usage and slower performance (same discussed in case of the constructor functions)
class Person {
constructor(name, year) {
this.name = name;
this.year = year;
}
greet() {
console.log("Hello, " + this.name + ", you are in " + this.year);
}
}
const newPerson = new Person("Bahnisikha", 2024);
console.log(newPerson); // Person { name: 'Bahnisikha', year: 2024 }
newPerson.greet(); // Hello, Bahnisikha, you are in 2024
Creating Objects with Factory Functions
A factory function is a special type of function specifically designed to create and return objects. Factory functions are useful for creating multiple instances of similar objects without using the new
keyword and constructors, making it a good alternative to constructor functions and classes
function createPerson(name, year) {
return {
name,
year,
greet() {
console.log("Hello, " + this.name + ", you are in " + this.year);
}
};
}
const newPerson = createPerson('Bahnisikha', 2024);
newPerson.greet(); // Hello, Bahnisikha, you are in 2024
console.log(newPerson); // { name: 'Bahnisikha', year: 2024, greet: [Function: greet] }
These are some of the ways we can create objects in Javascript. Hope you enjoyed it. Stay tuned for more such blogs ๐.
Subscribe to my newsletter
Read articles from Bahnisikha Dhar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
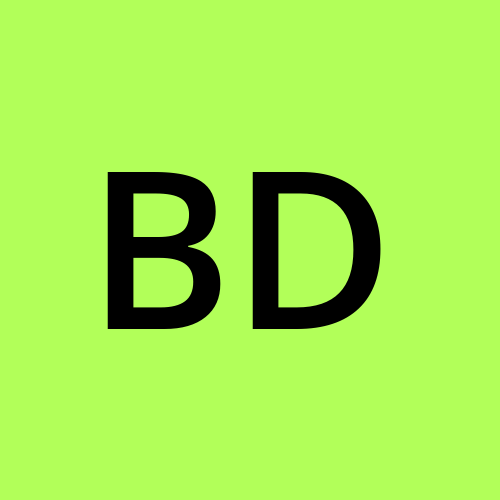