Demystifying Bubble Sort: A Simple Guide for Beginners with Implementation
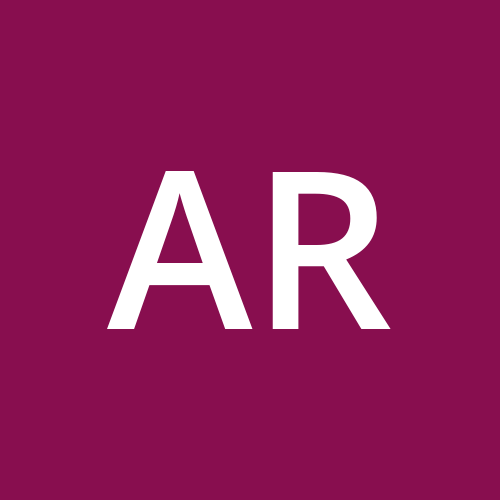
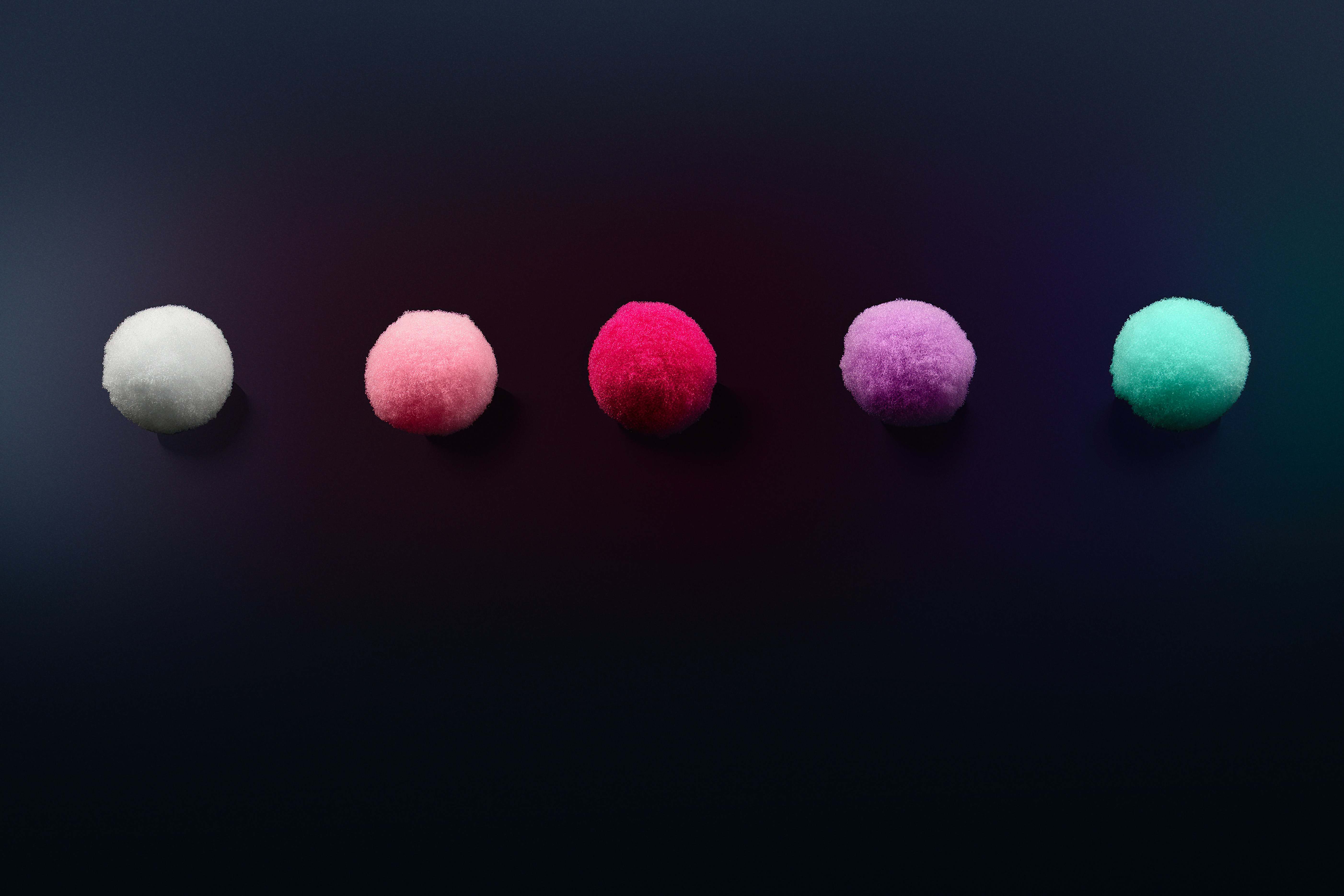
Introduction
Hey there, fellow coders! Today, we're diving into the world of sorting algorithms with one of the simplest and most intuitive ones: Bubble Sort. Sorting is a fundamental task in programming, and understanding basic sorting algorithms is essential for any aspiring coder. Let's break down Bubble Sort in a way that's easy to understand, even for beginners!
What is Bubble Sort?
Bubble Sort is a straightforward sorting algorithm that works by repeatedly stepping through the list to be sorted, comparing each pair of adjacent items, and swapping them if they are in the wrong order. The process is repeated until no more swaps are needed, indicating that the list is sorted. The name "Bubble Sort" comes from the way smaller elements "bubble" to the top of the list (beginning) with each pass.
How It Works?
Let's walk through the steps of Bubble Sort with a simple example:
Start with the First Pair:
- Compare the first two elements in the list.
Swap if Necessary:
- If the first element is greater than the second, swap them.
Move to the Next Pair:
- Move to the next pair of elements and repeat the comparison and swap if needed.
Continue to the End:
- Continue this process for each pair of elements until you reach the end of the list. This completes one pass.
Repeat Passes:
- Repeat the process for the entire list. After each pass, the largest element in the unsorted portion of the list will have moved to its correct position.
Stop When Sorted:
- Stop when a pass is completed without any swaps. This means the list is sorted.
Pseudocode Example
Let's sort the array [5, 3, 8, 4, 2]
using Bubble Sort:
First Pass:
Compare 5 and 3 -> swap ->
[3, 5, 8, 4, 2]
Compare 5 and 8 -> no swap ->
[3, 5, 8, 4, 2]
Compare 8 and 4 -> swap ->
[3, 5, 4, 8, 2]
Compare 8 and 2 -> swap ->
[3, 5, 4, 2, 8]
Second Pass:
Compare 3 and 5 -> no swap ->
[3, 5, 4, 2, 8]
Compare 5 and 4 -> swap ->
[3, 4, 5, 2, 8]
Compare 5 and 2 -> swap ->
[3, 4, 2, 5, 8]
Compare 5 and 8 -> no swap ->
[3, 4, 2, 5, 8]
Third Pass:
Compare 3 and 4 -> no swap ->
[3, 4, 2, 5, 8]
Compare 4 and 2 -> swap ->
[3, 2, 4, 5, 8]
Compare 4 and 5 -> no swap ->
[3, 2, 4, 5, 8]
Compare 5 and 8 -> no swap ->
[3, 2, 4, 5, 8]
Fourth Pass:
Compare 3 and 2 -> swap ->
[2, 3, 4, 5, 8]
Compare 3 and 4 -> no swap ->
[2, 3, 4, 5, 8]
Compare 4 and 5 -> no swap ->
[2, 3, 4, 5, 8]
Compare 5 and 8 -> no swap ->
[2, 3, 4, 5, 8]
No swaps were needed in the last pass, so the array is sorted.
Time Complexity
Best Case: ๐(๐) - When the list is already sorted.
Average Case: ๐(๐ยฒ) - Typical case with random order.
Worst Case: ๐(๐ยฒ) - When the list is sorted in reverse order.
Space Complexity
- Space Complexity: ๐(1) - Bubble Sort is an in-place sorting algorithm, meaning it requires only a constant amount of additional space.
Implementation in JavaScript
Here's how you can implement Bubble Sort in JavaScript:
function bubbleSort(array) {
let n = array.length;
let swapped;
do {
swapped = false;
for (let i = 0; i < n - 1; i++) {
if (array[i] > array[i + 1]) {
// Swap elements
let temp = array[i];
array[i] = array[i + 1];
array[i + 1] = temp;
swapped = true;
}
}
} while (swapped);
return array;
}
// Example usage
const arr = [5, 3, 8, 4, 2];
console.log(bubbleSort(arr)); // Output: [2, 3, 4, 5, 8]
Implementation in Python
And here's how you can do it in Python:
def bubble_sort(array):
n = len(array)
swapped = True
while swapped:
swapped = False
for i in range(1, n):
if array[i - 1] > array[i]:
# Swap elements
array[i - 1], array[i] = array[i], array[i - 1]
swapped = True
return array
# Example usage
arr = [5, 3, 8, 4, 2]
print(bubble_sort(arr)) # Output: [2, 3, 4, 5, 8]
Use Cases
Small Data Sets:
- It's simple and works fine for small lists where the simplicity of implementation outweighs efficiency concerns.
Partially Sorted Lists:
- Bubble Sort can be effective when the list is already nearly sorted, as it requires fewer passes.
More About Algorithms
If you enjoyed learning about Binary Search, you might also find these articles interesting:
Understanding Merge Sort: A Simple Guide for Aspiring Coders
Cracking the Code: Understanding Quick Sort Algorithm in Simple Steps
These posts will help you further explore different sorting algorithms and improve your coding skills!
Conclusion
Bubble Sort may not be the most efficient sorting algorithm for large datasets, but it's a great starting point for understanding the basics of sorting. Its simple logic and easy implementation make it an excellent tool for learning how sorting works.
If you found this post helpful, please give it a like and share it with others who might benefit from it. Happy coding!
Subscribe to my newsletter
Read articles from Artur directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
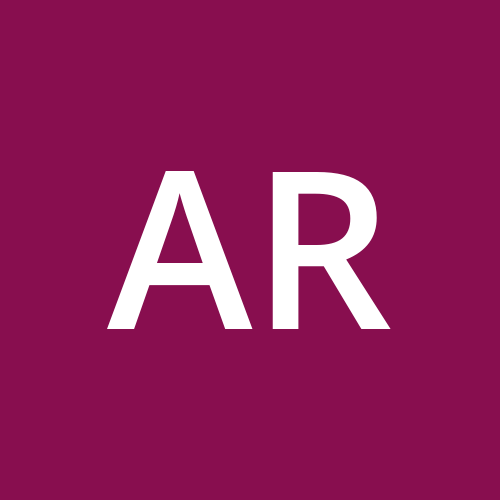